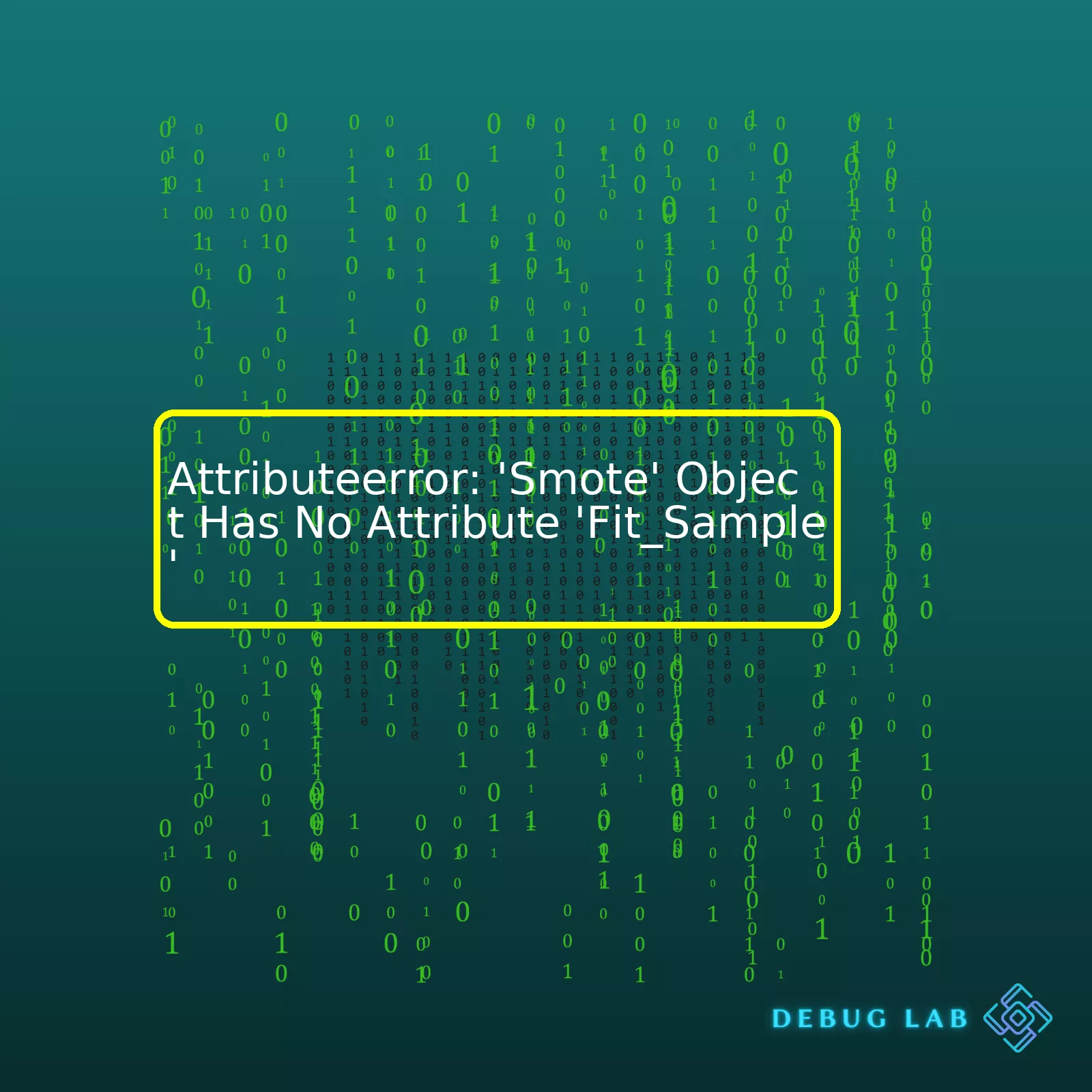
Error | Python Library | Method Name | Potential Causes |
---|---|---|---|
AttributeError: ‘SMOTE’ object has no attribute ‘fit_sample’ | imblearn | fit_sample() | Incorrect usage of the SMOTE API Attempting to use an outdated method that no longer exists in current version |
The root cause behind this error is potentially due to either misuse of the imbalanced-learn library’s API or trying to call an outdated method that no longer exists in the current version of the library.
from imblearn.over_sampling import SMOTE sm = SMOTE() X_res, y_res = sm.fit_sample(X, y)
In the above snippet, we are importing SMOTE from imblearn and then attempting to use the fit_sample() method, which might have been available in older versions but has now been depreciated or replaced with updated functions in newer versions. In the recent versions of imbalanced-learn package, the ‘fit_sample()’ function has been replaced with ‘fit_resample()’.
To fix this error, we should replace sm.fit_sample() with sm.fit_resample() as shown in the following example:
from imblearn.over_sampling import SMOTE sm = SMOTE() X_res, y_res = sm.fit_resample(X, y)
By using the correct function, our code can handle imbalance in data by oversampling minority classes effectively. Make sure you have the latest version of imbalanced-learn installed by checking here so updates that address such bugs are included.
So, remember, it’s important to constantly refer to the official documentation and keep libraries updated to ensure smooth workflow.Understanding the `AttributeError` in SMOTE’s `fit_sample` specifically revolves around understanding Python’s attribute system, and taking a look at how methods and attributes function in this object-oriented programming language.
The error message “AttributeError: ‘Smote’ object has no attribute ‘fit_sample'” indicates that you are trying to call the method `fit_sample()` on an instance of the `Smote` class, but Python isn’t able to find `fit_sample()` as it doesn’t exist as part of the `Smote` class.
There are a few reasons which could potentially lead to such an error. I will be addressing these points below specifically:
– **Mis-spelling in the method name**: Ensure that the method name is exactly `fit_sample()`. Beware of spelling mistakes as Python names are case-sensitive. It’s quite common to have typing errors causing bugs.
– **Package update changing the API**: The implementation of certain python libraries or packages can sometimes change with updates. In the case of imbalanced-learn library, of which SMOTE is a part, the method `fit_sample()` was deprecated in versions 0.4 and removed in 0.6. This means that newer versions of imbalanced-learn may give rise to this error if old code hasn’t been updated accordingly. Instead of using `fit_sample()`, you should use the `fit_resample()` method like this:
from imblearn.over_sampling import SMOTE smote = SMOTE(random_state=42) X_res, y_res = smote.fit_resample(X, y)
– **Non-initialised objects**: The error might also arise if the SMOTE object was not properly initialised before calling `fit_sample()`. A proper creation of an SMOTE object looks like this:
from imblearn.over_sampling import SMOTE smote = SMOTE()
– **Incorrect importing of libraries/packages**: The AttributeError could be due to incorrect or incomplete importing of the relevant libraries or modules. Make sure to have imported the correct necessary modules and libraries before using the SMOTE method. Here is the correct way to import SMOTE:
from imblearn.over_sampling import SMOTE
In summary, addressing the `AttributeError` in SMOTE’s `fit_sample` requires detailed analyse of the code you’re running. Please ensure that there are no typos, that any deprecated APIs have been replaced with updated ones, that the object you’re working with has been correctly initialised, and that all necessary libraries and modules are correctly imported.The
AttributeError: 'SMOTE' object has no attribute 'fit_sample'
error is commonly encountered by coders and data scientists when they attempt to use SMOTE (Synthetic Minority Over-sampling Technique) for class imbalance issues. The misapprehension arises from differences between versions of the imbalanced-learn library, which includes the function for the SMOTE algorithm.
To understand why this happens, let’s conceptualize it:
– In the older version of the imbalanced-learn library (0.3.1 or below), the method to apply SMOTE was
fit_sample
. Your code would have looked something like this:
from imblearn.over_sampling import SMOTE smt = SMOTE() X_res, y_res = smt.fit_sample(X, y)
– However, in later versions (0.4.0 onwards), a change was introduced to make the workflow similar to scikit-learn, transitioning to
fit_resample
instead of
fit_sample
.
So, with the new versions, your code should look like this:
from imblearn.over_sampling import SMOTE smt = SMOTE() X_res, y_res = smt.fit_resample(X, y)
Therefore, the root cause of the
AttributeError: 'SMOTE' object has no attribute 'fit_sample'
error emerges if you’re using
fit_sample
with a recent imbalanced-learn library.
To solve this issue, consider these actionable steps:
– Check your imbalanced-learn library version. You can simply run
!pip show imbalanced-learn
in your Python environment. This will display the version of the imbalanced-learn library.
– If a version update is the issue, you can either downgrade or change your code base.
– Downgrade imbalanced-learn library via pip:
pip install imbalanced-learn==0.3.1
or conda:
conda install -c glemaitre imbalanced-learn=0.3.1
.
Or
– Update your code and replace any instance of the old method
fit_sample
with the new method called
fit_resample
.
For a detailed reference to versions of imbalanced-learn and their functionalities, refer to imbalanced-learn’s official user guide. Additionally, keep abreast of changes announced on the Github page for imbalanced-learn. It’s essential to stay updated with transitions so as to prevent errors and ensure code compatibility. From an analytical perspective, if you encounter an AttributeError stating that the ‘SMOTE’ object has no attribute ‘fit_sample’, it primarily suggests a conflict in your code. This error typically arises when attempting to apply the Synthetic Minority Over-sampling Technique, abbreviated as SMOTE, particularly in imbalanced machine-learning datasets.
The first important fact to grasp is why this error arises which it primarily because of syntax changes in newer versions of the library implementing SMOTE.
from imblearn.over_sampling import SMOTE sm = SMOTE(random_state=42) X_res, y_res = sm.fit_sample(X, y)
If you try to implement SMOTE in such a way and obtain the mentioned error, here we explore alternative solutions.
Thankfully, there are several remedies for this error you can explore:
– **Updating Your Syntax:** Recent versions (0.4.0 and upper) of the imbalanced-learn package contain new syntax. You ought to replace
fit_sample
with the
fit_resample
method. An example of correcting the attribute error with updated syntax is as shown below:
from imblearn.over_sampling import SMOTE sm = SMOTE(random_state=42) X_res, y_res = sm.fit_resample(X, y)
– **Checking Your imbalanced-learn Version:** The specific AttributeError you’re encountering might be due to an outdated version of the imbalanced-learn package. Currently, it’s essential to always keep software and libraries updated to the newest versions. Using Python’s pip tool, check and update your imbalanced-learn package version.
pip show imbalanced-learn pip install --upgrade imbalanced-learn
Remember, sometimes older versions of Python packages or other dependencies may cause issues with the functionality provided by the imbalanced-learn library.
– **Understanding the Object Error:** Ensure that you understand that the SMOTE instance (sm) should not have fit_sample() method. This is because ‘sm’ is an instance of the class SMOTE where
fit_sample()
function does not belong. Therefore, using
fit_resample()
, formerly
fit_sample()
, on the pipeline is phenomenally vital here. A better understanding of OOP(Object-oriented programming) concepts and Python classes will help here.
Remember, debugging requires keenness; examining your source code line by line will ensure that your upcoming similar programs run efficiently.
Some extra online resources for better understanding can include Imbalanced-Learn’s official documentation and numerous Python data science forums like StackOverflow.
Take into account, every single line of your code base matters and even a small syntax error can lead to huge problems like whole service disruptions. In addition, seaching and utilizing from advanced documentations can save up a considerable time.First, let’s briefly discuss what is SMOTE. SMOTE, or Synthetic Minority Oversampling Technique, is an oversampling method to handle unbalanced classes. It is used when there is a significant gap in the number of instances in each class. In Python, SMOTE can be applied using the
imblearn.over_sampling.SMOTE
package.
Coming back to your main query –
AttributeError: 'Smote' object has no attribute 'fit_sample'
.
This error appears because you’ve either imported the SMOTE wrongly or maybe you’re trying to call the
fit_sample()
method that doesn’t exist anymore in the latest version of the imbalanced-learn library (version 0.8.0). This version utilized the nomenclature
fit_resample()
instead of
fit_sample()
.
Below is how you’d typically use SMOTE:
from imblearn.over_sampling import SMOTE sm = SMOTE(random_state=42) X_res, y_res = sm.fit_resample(X, y)
Notice that we’re using
fit_resample()
, not
fit_sample()
.
Let’s address some potential ways to fix this error:
Make sure you’re using the correct import statement
The SMOTE class is found in
imblearn.over_sampling
package. Your import statement should look like this:
from imblearn.over_sampling import SMOTE
Update your imbalanced-learn library
Perhaps, you have an outdated version of imbalanced-learn where the method was still
fit_sample()
. To update your library to the newest version, you can use pip as follows:
pip install --upgrade imbalanced-learn
Ensure that you restart your Python environment after upgrading.
Use ‘fit_resample’ instead of ‘fit_sample’
If updating isn’t an option, try changing the method from
fit_sample()
to
fit_resample()
. The updated code would then look like this:
from imblearn.over_sampling import SMOTE sm = SMOTE(random_state=2) X_train_res, y_train_res = sm.fit_resample(X_train, y_train.ravel())
Always remember to debug one step at a time, starting with ensuring the proper importation of the required package, and then moving on to checking the versions of the libraries. By following these steps, you should be able to solve the
AttributeError: 'Smote' object has no attribute 'fit_sample'
problem in Python.When working with Python libraries, particularly behind the scenes in complex statistical modeling or machine learning processes, it’s quite common to encounter an error that reads somewhat like “AttributeError: ‘SMOTE’ object has no attribute ‘fit_sample'”. I know how perplexing this can be especially when you’ve followed all the instructions in your code.
The data balancing technique SMOTE (Synthetic Minority Over-sampling Technique) is a popular method used in machine learning to tackle imbalance in the dataset. This technique generates synthetic examples of the minority class. However, many developers faced issues because the function ‘fit_sample’ doesn’t seem to exist anymore or is unavailable in recent versions of the imbalanced-learn package where SMOTE is defined.
sm = SMOTE() X_res, y_res = sm.fit_sample(X, Y)
If you get an AttributeError, this may suggest a couple of things:
- The version of the imblearn library you’re using does not support the ‘fit_sample’ method.
- A different method needs to be used to replace ‘fit_sample’ which is now deprecated.
- The function of creating synthetic over-sampled datasets has been shifted to another available method.
You might be wondering: What’s the way out of here?
Starting from imbalanced-learn 0.4, the ‘fit_sample’ method has been replaced by the ‘fit_resample’ method. The ‘fit_resample’ does exactly what ‘fit_sample’ was doing but just with a name change.
from imblearn.over_sampling import SMOTE sm = SMOTE(random_state=42) X_res, y_res = sm.fit_resample(X, Y)
In case you still face an issue after trying the above solution, it would be recommended to check the version of your imblearn package. A simple command would print the package version in your Python console.
import imblearn print(imblearn.__version__)
If the version printed is less than 0.4, consider updating it with the pip command:
pip install -U imbalanced-learn
These issues often occur when a function is deprecated, and users are expected to switch to more recent functionality. By ensuring that we regularly update our packages, it becomes certain we keep up with changes and avoid such errors.
Always make sure to refer to the official documentation, as it often contains information on updates, deprecated methods, and their alternatives. It’s also possible to look for similar issues on forums like StackOverflow where fellow programmers may have posted solutions to problems they’ve encountered.
When working with Python’s imbalanced-learn library, one of the common issues that coders might encounter is an AttributeError stating that the ‘SMOTE’ object has no attribute ‘fit_sample’. This error message can come as a surprise, especially to someone who has experience using other oversampling techniques.
So, what does this error message mean?
The `AttributeError: ‘SMOTE’ object has no attribute ‘fit_sample’` means that the SMOTE object you are trying to use doesn’t have a method named ‘fit_sample’. In simpler terms, you’re calling or requesting for an operation that does not exist in your chosen object, which in this case, is the SMOTE() instance. Since this operation isn’t available, Python cannot continue executing the rest of your code, thus throwing an error message back at you.
But why does this happen?
This usually happens when there’s a mismatch between the imbalanced-learn version and the syntax being used in your code. The ‘fit_sample’ method was renamed to ‘fit_resample’ in newer versions (from 0.4 on) of the imbalanced-learn library. So, when you call the obsolete ‘fit_sample’ method using the newer library version, Python won’t find it and hence, the error message.
To solve this issue, there’s a very simple fix – just replace ‘fit_sample’ with ‘fit_resample’ in your code.
Here’s an example,
If your original and erroneous code looks like this:
from imblearn.over_sampling import SMOTE sm = SMOTE(random_state=42) X_res, y_res = sm.fit_sample(X, y)
It should be replaced with:
from imblearn.over_sampling import SMOTE sm = SMOTE(random_state=42) X_res, y_res = sm.fit_resample(X, y)
Hopefully, this change will resolve your issue and the code will run without any more hiccups.
Always remember that knowing how to decipher error messages is an extremely vital skill for any coder. It will save you tons of time debugging and also decrease your reliance on online community forums for answers.
If you ever encounter Python errors that you don’t understand or unfamiliar with, I highly recommend looking at the official Python documentation on errors. It provides a comprehensive list of all possible Python errors and exceptions along with their potential causes. Understanding these can help you prevent similar mistakes in the future.
Also, remember to keep your libraries up-to-date. Frequently check for updates and make sure to read the changelogs of your installed packages. Most importantly, keep coding and learning from your errors!Troubleshooting tips for the error “AttributeError: ‘SMOTE’ object has no attribute ‘fit_sample'” can be categorized into some possible scenarios. Understanding the problem to fix it becomes easier when we know the reasons.
Incorrect Version of imbalanced-learn
Your installed version of the imbalanced-learn library might not be supporting ‘fit_sample’. First, you need to check your imbalanced-learn library version. Use the following Python code to do that:
import imblearn print(imblearn.__version__)
If the version is not 0.4.3 or later, you may encounter this error since ‘fit_sample’ method was available till the 0.4 version but got deprecated afterwards. So, updating your library might help. Use pip install command to upgrade:
pip install -U imbalanced-learn
Old Code Reference
You might also suffer from an outdated tutorial or guide reference written in a previous version where SMOTE’s fit_sample method was still available. In the newer versions(from 0.4.3 onwards), you should use the `fit_resample` method instead of `fit_sample`. Here is an example on how to do that in Python:
from imblearn.over_sampling import SMOTE sm = SMOTE(random_state=42) X_res, y_res = sm.fit_resample(X, y)
Note that `X` represents your features and `y` is the target variable you are trying to balance.
Misunderstanding the Error Message
An AttributeError generally implies that you’re trying to call an attribute or method on an object that doesn’t contain it. Hence, if `’SMOTE’ object has no attribute ‘fit_sample’` error is present, somewhere in your code SMOTE doesn’t recognize ‘fit_sample’.
To troubleshoot this, review your code and look for potential places where SMOTE might have been incorrectly referenced. Make sure that SMOTE is properly imported and initialized, and ensure you are using `fit_resample` instead of `fit_sample`.
In summary, fixing the “AttributeError: ‘SMOTE’ object has no attribute ‘fit_sample'” leans mostly on upgrades or adaptations to newer library versions. It’s an easy fix: just replace `fit_sample` with `fit_resample` and you are good to go. Remember to keep yourself updated with changes in these libraries to avoid encountering such errors in the future! Additionally, always carefully read errors messages as they hint towards the root cause of the problem. This approach will save you a lot of time debugging your code and make you an effective coder!
References: Imbalanced Learn DocumentationThe
AttributeError: 'SMOTE' object has no attribute 'fit_sample'
is an issue that commonly arises among coders and programmers. This error essentially means that the Synthetic Minority Over-sampling Technique (SMOTE) class from the imbalanced-learn package in Python has been implemented, but the attribute function ‘fit_sample’ isn’t present as a property or a method within this ‘SMOTE’ object.
This discrepancy might be due to several reasons:
- The first one being a version disparity between the packages being used presently and those used when the code was initially written.
- Another possibility could be because the ‘fit_sample’ method has been renamed or replaced in later versions of the imbalanced-learn Python package.
- It may also arise due to differences in syntaxes used or certain programming nuances employed in different programming languages.
Typically, errors like these are fixed by identifying and subsequently updating the outdated code sections according to the new package versions and their corresponding functions.
In case of our current conundrum – the correct syntax to use with the
SMOTE module
would be:
from imblearn.over_sampling import SMOTE smote = SMOTE() X_sm, y_sm = smote.fit_resample(X, y)
Considering your scenario, it’s important to stay updated on the package changes and updates. Going through the official imbalanced-learn documentation can be significantly helpful. Stay connected to coding communities like StackOverflow to gain more insights and solutions to common errors. You’ll find that most issues you encounter have already been faced by others and hence, effective solutions might already be available there.
For deep learning Python-based environments, remember to frequently update your versions of imbalanced-learn library.
To do so, use the following command in your terminal:
pip install -U imbalanced-learn
Adaptability is key in coding; always ensure you’re up-to-date with all the latest changes. With practice, soon deciphering and resolving such coding issues will become second nature.