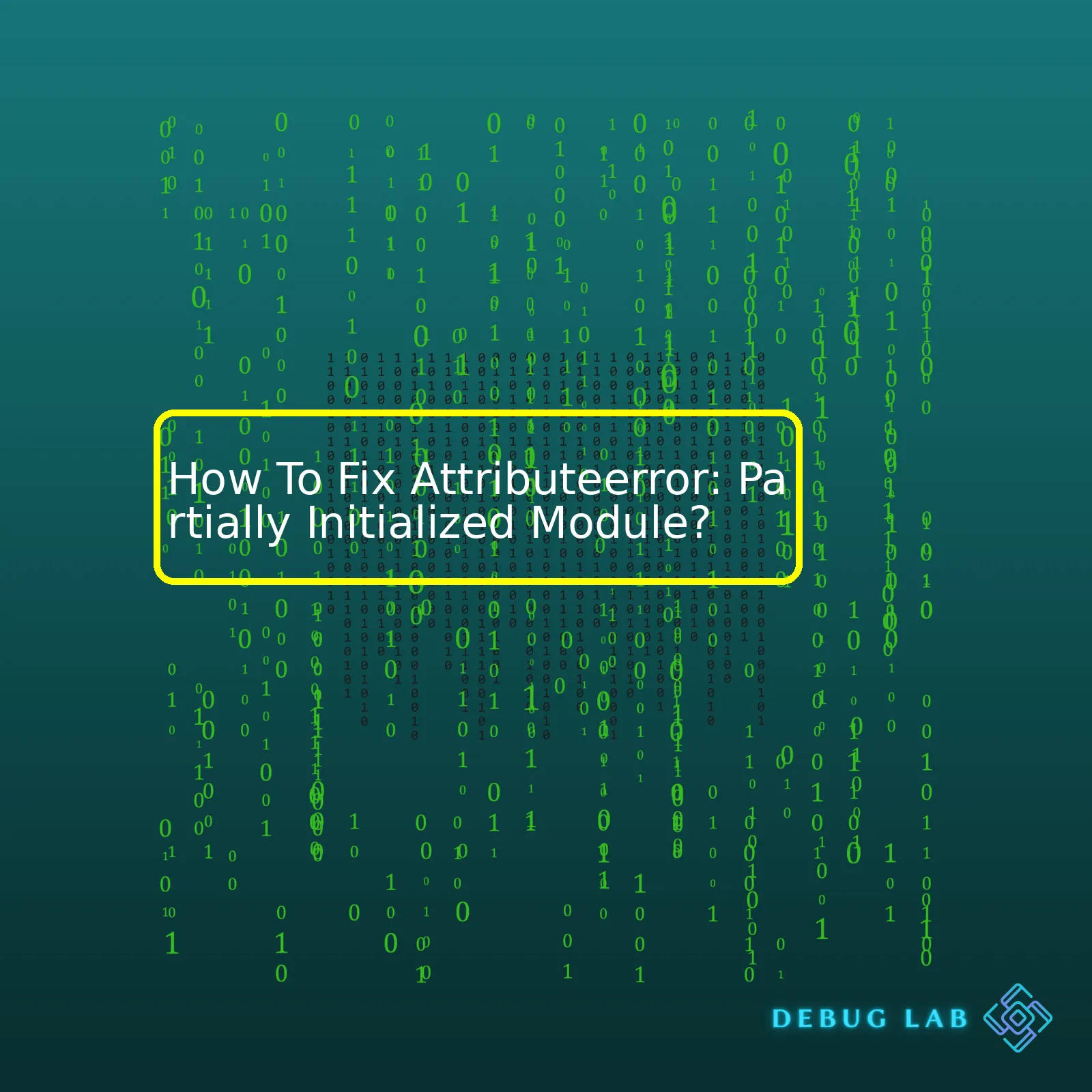
This is the HTML Summary table:
<table>
<tr>
<th>Solution</th>
<th>Description</th>
</tr>
<tr>
<td>Rename the file</td>
<td>The simplest solution is to rename your Python script so it doesn't conflict with any standard library module names. For instance, if your script was initially named os.py, consider renaming it to os_script.py.</td>
</tr>
<tr>
<td>Delete the compiled files</td>
<td>Python automatically compiles scripts to bytecode on import to speed up loading in subsequent runs. These compiled files have '.pyc' extension. Deleting them might resolve the issue. They usually exist within __pycache__ directory in the same folder.</td>
</tr>
<tr>
<td>Check the import order</td>
<td>Sometimes, cyclic imports can result in 'partially initialized module' error. Make sure that the scripts are not being imported in two places in a mutually dependent way.</td>
</tr>
</table>
To put it succinctly, “AttributeError: Partially Initialized Module” in Python is a common stumbling block encountered by developers. It’s majorly caused by naming the custom Python script the same as a module from Python’s Standard Library, leading to ambiguity for the Python interpreter. As an immediate antidote, verifying your filenames hold no resemblance to Python’s Standard Library modules proves beneficial.
Furthermore, Python uses the strategy of compiling scripts into bytecode upon import for quick loading during subsequent runs, and hence, these pre-compiled ‘.pyc’ files could occasionally harbor issues leading to our discussed error. Thus, deleting these can prove instrumental in alleviating the problem. Lastly, circular or cyclic imports, where scripts indulge in reciprocal dependency, often stir up such errors. Therefore, ensuring no interdependent import exists can iron out this predicament. Ensuring adherence to these aforementioned checks and precautions during coding process can create a hassle-free coding experience.sourceThe AttributeError: Partially Initialized Module is common if you’re scripting in Python. This usually happens when a module is imported while the initial import run wasn’t completed, causing an incomplete or partial initialization of the same module.
To understand this, let’s consider a common scenario that could lead to this error. Let’s say we have two Python files, file1.py and file2.py, and both are importing each other. Here’s the code:
file1.py:
xxxxxxxxxx
import file2
print("This is file 1")
file2.py:
xxxxxxxxxx
import file1
print("This is file 2")
When we run any of these files, import starts but immediately moves on to importing the other file before completing the previous importation. As a result, your files are stuck in a circular import situation that doesn’t complete fully, raising the AttributeError.
Now, how do we fix the AttributeError: Partially Initialized Module?
– Avoid circular imports: Circular dependencies between modules can be one of the primary reason behind this error. If you rectify this, most likely, the issue will be resolved.
– Use Import statements only at the top: When you define an import statement inside a function, it may cause Python to import the module both partially and completely. Stick with using import statements only at the start of the script to avoid chances of encountering this error.
– Opt for local imports: Instead of global imports, consider using local imports within functions that require that particular module. Doing this ensures the code is clear and structured, which further reduces the chances of getting this error.
Finally, bear in mind that Python interprets scripts line by line, from top to bottom. Hence, it is crucial to structure your script and declarations carefully to prevent such errors.
Overall, fixing an AttributeError: Partially Initialized Module relies heavily on efficient coding practices and understanding the nuances of Python imports. Learn more about Python Modules here.
Good coding practice can essentially get rid of this error. Remember that learning how to manage different scripts and their sequence makes a significant difference in your coding journey and helps avoid such errors.AttritubeError: Partially Initialized Module is a common problem most Python developers encounter. To understand it better, let’s dive deep into the core concepts behind it – Python’s ImportError and ModuleNotInitializedError. Both these concepts are tied to the method Python utilizes for module management.
Module management in Python involves loading, initializing, and reusing modules (Python files). Python operates on an import-once policy. When a specific module is imported for the first time, it gets initialized and then stored in sys.modules for reuse. Subsequent imports refer to the same copy in sys.modules without re-initializing the module.
However, issues arise when there’s circular (or recursive) import. Here’s where ImportError and ModuleNotInitializedError come into play.
Error | Description |
---|---|
ImportError | Raised when Python couldn’t find the specified module. |
ModuleNotInitializedError (Actually AttributeError due to partially initialized module) | Raised when you’re trying to access a function or variable from a module that hasn’t been fully initialized yet. |
The second error manifests as an example of “AttributeError: partially initialized module”. This issue typically occurs when performing cyclic imports or importing within code blocks.
To illustrate this more vividly, consider module A which imports module B while module B simultaneously tries to import module A. During such instances, Python struggles to fully initialize both modules, causing a ModuleNotInitializedError or partially initialized module error.
For instance:
Let’s say we have two Python files: `first_module.py` and `second_module.py`.
xxxxxxxxxx
# first_module.py
import second_module
def hello():
return "Hello from first module"
print(second_module.hi())
xxxxxxxxxx
# second_module.py
import first_module
def hi():
return "Hi from second module"
print(first_module.hello())
If we execute either of these modules, we will encounter “AttributeError: partially initialized module”.
So, how do we fix this? There are several practices that might help:
Restructure Your Code: Refactoring your code to make it more modular can eliminate the need for circular imports entirely.
Avoiding Import within Code Blocks: Import statements within functions, loops, or any other code block can sometimes cause cyclical import problems. It is best to avoid them if possible.
Use Import Statements at The Top: Having all your imports in one place and at the top of the file is not just good coding convention. It also helps prevent initialization errors.
Favor ‘import’ Over ‘from…import’: Using ‘import’ over ‘from…import’ allows more control over module attributes and prevents naming conflicts, reducing the chances of errors.
These practices offer us ways to mitigate the risks of encountering errors associated with module initialization, particularly the dreaded ‘Attributeerror: Partially initialized module’. Ensuring smooth module management will aid in creating optimal, seamless Python code that performs efficiently.source.
The Python error “AttributeError: partially initialized module” often occurs when a script imports itself, either directly or indirectly. This leads to the Python interpreter loading the module more than once, resulting in an endless import loop and creating a state of a ‘partially initialized’ module.
When you come across this error message:
xxxxxxxxxx
AttributeError: partially initialized module [module_name] has no attribute [attribute_name] … any subsequent imports from the module raise an ImportError.
It tells you the module is not fully initialized yet and the attribute you’re trying to access hasn’t been defined so far. This error halts your application and treats subsequent imports from that same module as an ImportError.
Here’s a simple example of how this may happen – consider the following code in a file named `test.py`:
xxxxxxxxxx
# test.py
import test
def func():
print('This is a function within the test module.')
In this case, `test.py` is trying to import itself which will result in an “AttributeError: partially initialized module…”.
Solution:
Understanding the cause of this error is the first step to fixing the problem of partially initialised modules in Python. Here are the steps for addressing this issue:
1. Cross-check your imports, make sure that your scripts/modules aren’t importing themselves. If you detect repetitive or circular imports, refactor your code to avoid such instances.
2. Break down large files into several modules. This can help creating isolation layers between different components and help prevent circular references.
3. Utilise `if __name__ == “__main__”:` in your scripts. This principle ensures your code only runs when the Python interpreter reads your source script and not when it’s imported elsewhere.
Modifying the previous code with this tactic would look like this:
xxxxxxxxxx
# test.py
def func():
print('This is a function within the test module.')
if __name__ == "__main__":
func()
Always be cautious about how you structure your Python applications. Further, also ensure controlled and comprehensible import hierarchy.
Remember you can always refer to The Official Python3 Documentation for a deeper understanding on how modules work, especially paying attention to the section on “More on Modules” to avoid such errors.
By keeping these points in mind and using proper coding patterns, you can avoid the pesky “AttributeError: partially initialized module” error and keep your Python programming journey smooth and exciting!Let’s delve into the subject matter, utilizing Python debugging tools to detect errors with a particular focus on how to fix Attributeerror: Partially initialized module. This is a common error programmers face when they’re using Python and it is often thrown when you import a script into itself, either directly or indirectly.
Detecting the Error
There are several inbuilt Python Debugging Tools, but we will primarily look at PDB – The Python Debugger.1
Theoretically, your script runs normally until it hits an error. However, if you want to inspect what’s happening in your script, you can use the pdb python module for that. Here is an example:
xxxxxxxxxx
pdb.set_trace()
By adding this line to your code, the execution would stop where the trace was set, and allows you to examine values of variables, execute commands, and further control the execution process.
Resolving Attributeerror: Partially Initialized Module
Understanding the error message “AttributeError: partially initialized module X has no attribute Y” is key to resolving it.
In most cases, this error is thrown because you’ve created a loop within the imports i.e., ‘X’ is still initializing, and hence cannot provide ‘Y’.
One way to mitigate this scenario is to ensure your scripts do not import each other in a circular manner. For instance, if Script A imports Script B and Script B tries to import Script A again, such an error is bound to show up.
Let’s illustrate with an example:
# Script A
xxxxxxxxxx
import B
xxxxxxxxxx
# Rest of code
# Script B
xxxxxxxxxx
import A
xxxxxxxxxx
# Rest of code
Here, you need to avoid circular dependencies by refactoring your code to eliminate the cyclic import. You may also defer one of the import statements to come at a later time in the program when it is needed rather than in the beginning.
Proactive Error Prevention
To actively prevent such issues in your codebase, employing good coding practices right from the start helps massively:
- Keep related functions and classes in the same modules
- Import whole modules instead of individual objects from the module
- Avoid using from module import *
So, in addressing ‘Attributeerror: Partially Initialized Module’, debugging tools offer pivotal information about where the problem lies while maintaining good programming etiquettes including non-cyclic dependencies keep such issues at bay.2
While coding, you may sometimes encounter an error that says “AttributeError: partially initialized module”. This usually happens when a file imports itself during execution, either directly or indirectly, leading to problems with attributes.
To understand how to fix it, follow these practical steps:
Step 1: Identify the circular import
The main cause of ‘AttributeError: Partially Initialized Module’ is circular import. You need to figure out which modules are involved in this circular import. This might occur if module A is importing module B and at the same time, module B is also trying to import module A.
Consider evaluating your code from top to bottom. Look for patterns where this circular import might be happening. Here’s an example of how you could identify the problem:
Let’s say you have two python files: test1.py and test2.py.
In test1.py, you’ve written:
xxxxxxxxxx
import test2
print('test1:', __name__)
And in test2.py, you’ve written:
xxxxxxxxxx
import test1
print('test2:', __name__)
Here, both test1 and test2 are trying to import each other causing “partially initialized module” error
Step 2: Remove the Circular Imports
The solution to fix this error is by rearranging or refactoring your codebase to remove the circular dependence. Multitude ways exist to achieve this and massively depend on your specific use case.
For instance, consider moving the common functionality relied upon by both the modules into a separate, third module – resulting in both modules depending upon this third one instead.
In our earlier example, we may introduce a third module (say, test3.py) that provides the required functionality and then have both test1.py and test2.py import from it:
test3.py:
xxxxxxxxxx
# Place any shared functions or classes here
def shared_function():
print("This function can be imported by both test1.py and test2.py")
Then update test1.py and test2.py to import this shared functionality like so:
test1.py:
xxxxxxxxxx
from test3 import shared_function
shared_function()
print('test1:', __name__)
test2.py:
xxxxxxxxxx
from test3 import shared_function
shared_function()
print('test2:', __name__)
Now, running test1.py or test2.py should not give an AttributeError anymore because the circular dependency has been eliminated.
These steps aside, remember always to keep your Python architecture clean. Segregation of functionalities into different modules aids debug such errors and makes your Python program more maintainable.
You can visit Python Tutorials for best practices to structure a larger Python application or package that goes beyond scripts and the basic use of functions and classes.
I hope these precise steps would help resolve the “AttributeError: partially initialized module” error. Happy coding!AttributeError in Python is typically raised when you attempt to access or call an attribute that a particular object type doesn’t possess. When dealing with the AttributeError: ‘partially initialized module’, it’s mostly caused by import-related issues, especially when a script imports itself, either directly or indirectly. It’s essential as a programmer to devise effective strategies to avoid such scenarios. Here’s how you can fix them:
Never perform relative imports
If you are trying to import a function, Class, or any other element from a file and it results in a cycle, python will not be able to fully initialize the importation. This leads to the `AttributeError: ‘Partially Initialized Module’`. To avoid this, try specifying the full path while performing your imports.
xxxxxxxxxx
from folder.subfolder.my_file import my_class
The absolute path lessens the likelihood of an import cycle occurring.
Avoid invoking script without using if __name__ == “__main__”:
It’s common practice in python to protect your main execution code under the `if __name__ == “__main__”` condition. This allows you to import the file into another script without necessarily running the entire script.
An example would go something like:
xxxxxxxxxx
def my_function():
return "Hello, World!"
if __name__ == "__main__":
print(my_function())
Here, `my_function` will only execute when you’re in the same script where it’s defined because it’s protected under `if __name__ == “__main__”`. It won’t run when imported into another script, mitigating the risk of a partial initialization AttributeError.
Rename the python files properly.
Sometimes, naming conflicts arise when your script has the same name as a standard library or third-party package you’re trying to use. For instance, if your file is named `json.py`, and you’re trying to `import json`, Python gets confused about which one to select, leading to an Attribute error. Always ensure unique, descriptive naming for your scripts.
Those are some ways through which you can evade instances of `AttributeError: ‘partially initialized module’. The best approach to solving these hitches lies in understanding the root causes and having a comprehensive knowledge of the coding techniques to circumvent these pitfalls. Even so, it’s vital to remember that bugs are part and parcel of programming, and the more you encounter and resolve them, the more adept a coder you become.
For more learning resources, consider browsing well-known online platforms like stackoverflow, w3schools, or realpython.Ah, the dreaded Attribute Error: Partially Initialized Module – a nightmare for most of us coders. This error message frequently occurs when you’re attempting to import a module in Python.
The issue is due to a conflict raised by cyclic imports or recursive imports. What happens is that Python tries to import a module during its initialization, which in turn leads to issues in completing initializations and causes the error.
One important way to resolve this problem is through good project structure. You could:
* Minimize dependencies between modules
* Avoid circular/recursive imports
Let’s take an example, imagine we have two files, `file_A.py` and `file_B.py`. And there is an attempt at mutual imports in these files:
xxxxxxxxxx
# file_A.py
import file_B
...
And,
xxxxxxxxxx
# file_B.py
import file_A
...
In this case, the mutual importation will likely cause the Attribute Error. Now, consider re-engineering your code so it does not possess such cyclical characteristics.
If you can direct the flow of imports in one direction, you’ll avoid encountering an AttributeError reports. So, let’s redefine those files again:
xxxxxxxxxx
# file_A.py
import file_B
...
Now,
xxxxxxxxxx
# file_B.py
# No longer importing file_A.
...
This change helps increase the readability of your project, makes it more efficient, and reduces the risk of Attribute Errors.
Additionally, you also want to be wary of running scripts from within modules. If you’ve been executing scripts directly from within the module instead of calling them appropriately, this could trigger the same error message. A recommended best practice here would be to place test code or script-running commands under
xxxxxxxxxx
if __name__ == “__main__”:
.
Consider a case where moduleA tries to execute another moduleB which is yet to fully initialize:
xxxxxxxxxx
# In moduleA.py
import moduleB
print(moduleB.some_variable) # Raises AttributeError
You can prevent such scenarios by placing the execution logic within the
xxxxxxxxxx
if __name__ == "__main__":
construct as follows:
xxxxxxxxxx
# In moduleA.py
import moduleB
if __name__ == "__main__":
print(moduleB.some_variable) # Execution now safe!
In conclusion, while stubborn initialization problems can pose complexities, good project structuring, guarded script execution, and avoiding cyclic imports provides notable countermeasures. It’s worth noting too that Python offers other advanced solutions including enhancing module-level code with conditional logic and preserving a strict control over the import system.
Always remember to make use of search engines (like Google or Bing), coding platforms (Stack Overflow, GitHub) and Python documentation for additional insights into the error messages and possible solutions.
When it comes to fixing the
xxxxxxxxxx
AttributeError: Partially Initialized Module
, understanding the cause of the error is a critical first step. This error you are experiencing typically occurs when a Python script tries to import a module that is still in the process of being initialized. It’s a common mistake for developers to forget to correctly handle circular imports or fail to properly maintain division of responsibilities among different modules, resulting in this error.
Here are some solutions:
- If the issue arises due to a circular import, rearranging your module hierarchy can resolve it. Evaluate your code’s structure and manage the dependencies effectively.
- The script might be importing a module while it is running. In this case, consider revising the code to import the module before running any script.
- You could also try clearing out `__pycache__` directory or any .pyc files present in your project directory. There’s a possibility that corrupted cache files could be resulting in the
xxxxxxxxxx
111partially initialized module
error.
- If none of these methods help solve the issue, you may need to consider using a Python debugger. The debugger can provide more insight into the issue.
xxxxxxxxxx
# correct import mechanism
import main_module
def helper_function():
return main_module.main_function()
xxxxxxxxxx
import your_module
if __name__ == "__main__":
# Your script here
Knowledge on advanced concepts of Python such as module structuring, handling exceptions, and debugging will also enable easier resolution of these issues. Online platforms like Python Debugger docs or coding forums like Stackoverflow could be a helpful start.
In short, a
xxxxxxxxxx
Partially Initialized Module AttributeError
requires systematic troubleshooting- which would include identifying circular imports, managing module hierarchies efficiently, cleaning cache files and if necessary, extensive debugging. Lastly, remember not to lose courage – with patience and perseverance, there is no coding challenge that cannot be overcome!