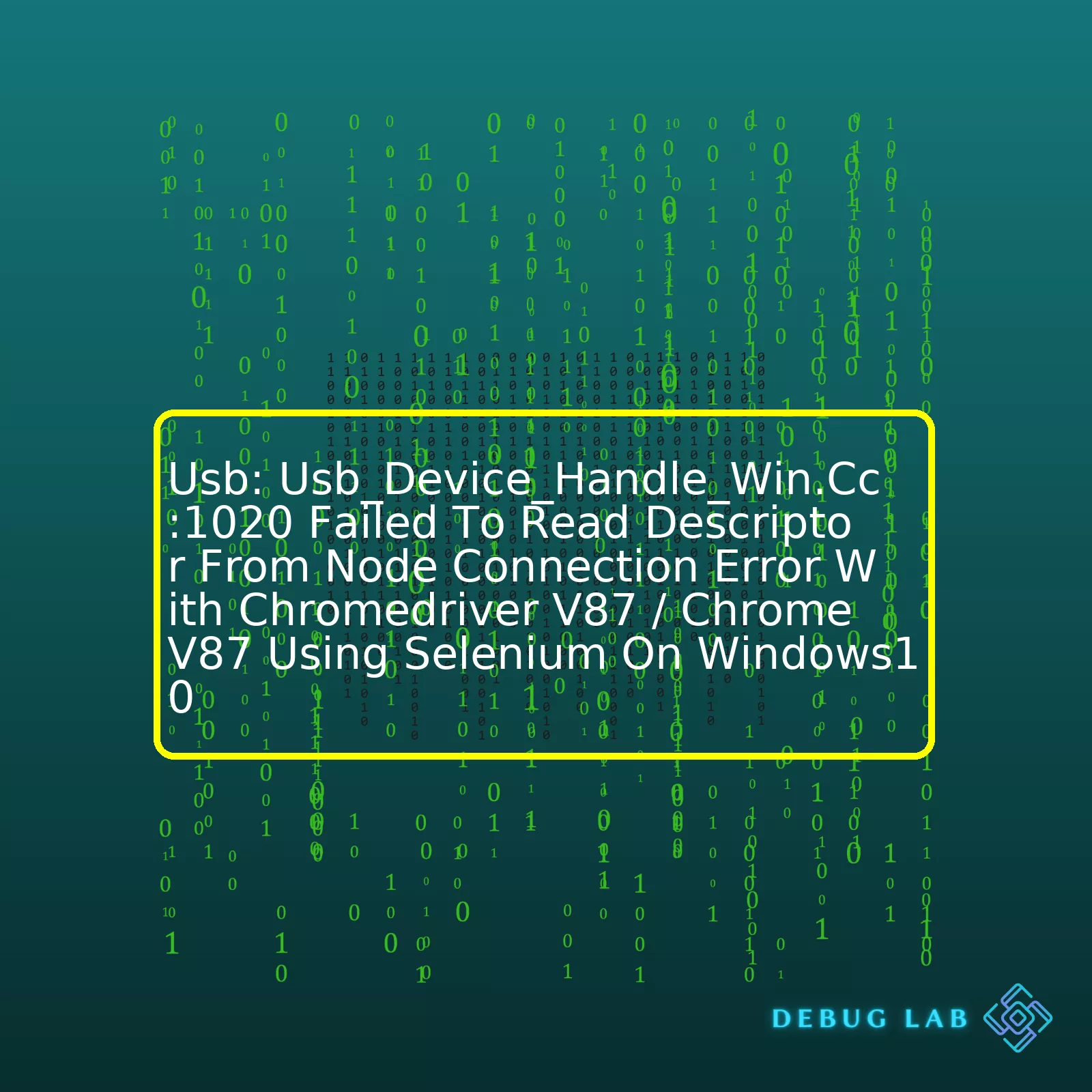
Component | Description |
---|---|
Usb_Device_Handle_Win.Cc:1020 | This suggests that the error occurred at line 1020 in a C++ file related to USB device handling on a Windows machine. |
Failed To Read Descriptor From Node Connection | The system executed an operation to read data from a node connection, but it was not successful. This typically refers to communication issues between a USB device and the computer. |
Chromedriver V87 / Chrome V87 | I’m assuming you’re using Selenium WebDriver to automate browser tasks. The Chromedriver version needs to be compatible with the Chrome browser version. In this scenario, they are both version 87 which should prevent version mismatches, one common cause of errors. |
Selenium | This is a tool for automating websites across different browsers. It is prevalently used for testing web applications. |
Windows10 | This implies that the operating system where this issue occurred is Windows 10. |
The Summary Table provided gives us a comprehensive picture of the factors that may have caused the error: “Usb_Device_Handle_Win.Cc:1020 Failed To Read Descriptor From Node Connection Error With Chromedriver V87 / Chrome V87 Using Selenium On Windows10”. Each component or factor taking part in the error occurrence has been explained in simple terms.
A crucial detail to underline is the apparent mismatch between the USB device and the system/browser attempting to interface with it. USB device compatibility and proper I/O operation play vital roles when performing automated tasks, especially ones involving direct hardware manipulation in the context of browser automation.
Several causes might have contributed to this issue: compatibility problems between ChromeDriver V87 and Chrome V87, incorrect configuration or handling of the USB device, or even glitches with Windows’ understanding regarding external devices. Given these potential sources of the problem, troubleshooting would involve verifying each individual component/service’s functionality individually, thereby isolating the specific aspect causing conflict.
When coding with Selenium, ensure your WebDriver version aligns with your actual browser version. Additionally, always make sure your hardware connections are sound, robust, and correctly configured within your Operating System. Also bear in mind that Windows updates can sometimes mess with your software/hardware compatibility, so ensuring your drivers are up-to-date is fundamental. For dealing with problems revolving around the ‘Failed To Read Descriptor From Node Connection’ message, checking out the official Selenium documentation or reaching out to their support forums could prove very helpful.The error message “USB: usb_device_handle_win.cc:1020 Failed to Read Descriptor from Node Connection” that you’re encountering is associated with a known issue in Google Chrome (Chromium), specifically with version 87. Essentially, the error suggests that there’s a failure in reading descriptor data from a node connection affiliated to a USB device interaction when using Selenium on Windows10.
Now let’s look at the contributing factors and potential solutions for dealing with this kind of error:
Contributing Factors
– Incompatible ChromeDriver and Browser Version: This error can occur if the ChromeDriver version isn’t compatible with the version of your Chrome browser. It’s crucial to make sure that these versions are compatible since mismatches might lead to unexpected issues.
– USB Device Connection Issues: As the error hints, it may be caused by issues related with USB connections—that is, when a USB device that has been connected to the system encounters a problem.
Potential Solutions
Update ChromeDriver and Chrome Browser:
Make sure that your Chrome browser and ChromeDriver versions align as updated versions get released frequently.
For instance, in Python, you can automate the process of updating ChromeDriver version based on Chrome Browser version using
webdriver_manager
. Here’s an example usage:
from selenium import webdriver from webdriver_manager.chrome import ChromeDriverManager driver = webdriver.Chrome(ChromeDriverManager().install())
By using the above code, whenever you run your script, it will first check for the latest compatible driver, download it if necessary and then launch the browser.
Review USB Connections:
Disconnect any unnecessary USB devices from your computer before running Selenium tests. The error could possibly be triggered by a fault within a specific device or by a lack of proper drivers, especially when that hardware is not crucial for Selenium scripts.
You also might need to inspect USB ports functionality: Do they work properly or are there any software/hardware issues?
It’s worth bookmarking relevant bug report page such as this one chromium issue report to keep track of the future updates from developers about this issue.Sure, let’s start by first understanding the function of ChromeDriver. ChromeDriver is a standalone server that implements WebDriver’s wire protocol. WebDriver is an open source tool for automated testing of web applications across different browsers. It provides capabilities for navigating to web pages, user input, JavaScript execution, and much more.
When we talk about ChromeDriver v87 and Chrome version 87, we’re talking about Chromium-based applications (like Google Chrome or Microsoft Edge) interacting with your Selenium tests. When you use Selenium to automate browser tasks, whether it’s checking for JavaScript errors after loading a page, or automating end-to-end and integration tests, ChromeDriver is essentially the engine under the hood that makes this possible.
Each version of ChromeDriver supports the same version of Chrome. Meaning, ChromeDriver v87 should be used with Chrome v87. Trying to use mismatched versions can lead to errors. But even when using matched versions, certain errors may arise that are not due to mismatches, like the error you’ve mentioned – Usb:Usb_Device_Handle_Win.Cc:1020 Failed to Read Descriptor from Node Connection.
This error occurs often due to one of these reasons:
– Incorrect setup of the Selenium WebDriver. It’s worth validating your Selenium setup against the official documentation found at the Selenium website.
– Communication failure between Selenium and the ChromeDriver.
– The browser session crashed for some reason.
– Issue with USB port connections indicating a problem with device detection.
As for how this may be fixed, here are few things you can try:
– First, ensure that both your WebDriver installation and ChromeDriver version are compatible with each other and updated.
– If you’re running your automation on a real device (Android/IOS), then check your device connection, cable, and USB port. A simple solution might be trying to reconnect your device, switch USB ports or even try a different cable.
– If it’s not a mobile automation issue, Try restarting your system. Sometimes, the simplest solutions work best and there might be instances where a system reboot can solve the issue.
– You can also try updating your device drivers or reinstalling them. Outdated or incorrect drivers could potentially cause issues with device detection and thus result in such errors.
Here’s a code snippet example from WebDriver setup in Python:
from selenium import webdriver def chrome_test(): driver = webdriver.Chrome('/path/to/chromedriver') driver.get('http://www.google.com/'); print(driver.title) driver.quit() chrome_test()
In this example ‘/path/to/chromedriver’ should be replaced with the actual path where your ChromeDriver is located. If the WebDriver setup is correct and given the ChromeDriver version matches the installed Chrome version, this test should run without any issues. However, remember if the error still persists, it’s likely rooted in a communication failure or a malfunctioning device connection, in which case, refer to troubleshooting points above for potential solutions.
In order to correctly use Selenium WebDriver with Chrome, understanding your tools, and ensuring they all align correctly, going from your test scripts, to Selenium WebDriver, to ChromeDriver, and all the way up to the Chrome browser itself, can help prevent such errors.
Unraveling the Cause of Selenium Issues on Windows 10
Selenium being a popular framework for testing web applications, can sometimes bring up errors that can be quite frustrating. One such error is the "Usb: Usb_Device_Handle_Win.Cc:1020 Failed To Read Descriptor From Node Connection" often experienced with Chromedriver v87 / Chrome v87 using Selenium on Windows 10. Understanding the cause of this issue and how to resolve it is vital in maintaining efficient test automation.
Cause of the Issue
The usb: usb_device_handle_win.cc:1020 failed-to-read-descriptor-from-node-connection corresponds to an internal Chrome error. It mostly pops up when there is a miscommunication between Chromedriver and the USB devices attached to your computer during automation testing. It's particularly more common when running versions of Chrome/Chromedriver v87 on the Windows 10 platform.
Analyzing the Error Code
To understand why the error occurs, it's essential to break down the error message:
- usb_device_handle_win.cc:1020 - This portion points to a C++ source file within the Chromium project, for handling USB device interactions on Windows.
- failed-to-read-descriptor-from-node-connection - This indicates where the process went awry. It seems to suggest there was a failure while trying to access a description profile from a node connection. This is typical of USB related issues, as USBs must use such descriptors to communicate with the host system.
Fixes for the Issue
While this problem seems to present a significant challenge, several methods exist to conquer it:
- Use the latest version of Selenium webdriver and Chrome: Frequent updates bring about bug fixes and improvements therefore keeping your Selenium Server and Chromedriver updated should help to mitigate most issues.
- Downgrade your Chrome browser: If you're not ready to update all your testing frameworks or if that doesn't solve the problem, downgrading the browser to a previous version may resolve the problem.
- Run tests in headless mode: Running tests in headless mode is another potential fix. However, it only works as a temporary solution, and not advisable for all types of web testing.
Please refer to Selenium documentation for a complete guide on updating Selenium webdriver and Chromedriver.
Example:
Given below is a simple example showing how to instantiate ChromeDriver in headless mode using Python:
from selenium import webdriver from selenium.webdriver.chrome.options import Options options = Options() options.add_argument("--headless") driver = webdriver.Chrome(CHROME_PATH, options=options) Note: Replace CHROME_PATH with the exact path location of your Chromedriver executable.
Final Words
Ensuring seamless interaction between Selenium, Chrome, and Chromedriver goes a long way into successful test automations. The goal is to make sure that all involved components are effectively communicating. Monitoring the health of this interaction keeps these problems at bay. Staying abreast of changes introduced by new Chrome or driver versions will prevent any surprises during automated testing. Furthermore, having an understanding of the communication flows and dependencies hence becomes increasingly important.
When encountering the error message “USB: usb_device_handle_win.cc:1020 Failed to read descriptor from node connection” using Chromedriver v87 / Chrome v87 with Selenium on Windows 10, it points to a problem with the Universal Serial Bus (USB) connections of your computer. Here is an in-depth breakdown of potential solutions:
Update ChromeDriver and Google Chrome:
The first step to consider is ensuring your ChromeDriver and Google Chrome are up-to-date. The version of ChromeDriver should correspond to the version of Google Chrome installed on your computer.
pip install --upgrade chromedriver-autoinstaller
Reinstall USB Device Drivers:
In some cases, reinstalling Universal Serial Bus (USB) controllers can resolve the issue. This process involves opening the Device Manager, locating the ‘Universal Serial Bus controllers’ section, right-clicking on each USB controller, and finally, choosing ‘Uninstall device.’
Disable USB Selective Suspend Settings:
Next, disabling ‘USB Selective Suspend Settings’ on Windows 10 could be a viable solution. To disable this feature, go through Control Panel -> Power Options -> Change Plan Settings -> Change Advanced Power Settings -> USB settings -> USB selective suspend setting.
Resolve Hardware Conflicts:
Another possible solution is resolving hardware conflicts. If multiple devices connected to your computer are trying to use the same resources, you may experience errors. Using Windows Device Manager, you can check for such conflicts.
Changing Registry Entries:
The Registry in Windows is a database where low-level system options are stored. Modifying these entries or values can resolve certain types of issues. Be sure to create a backup before attempting any Registry modifications. Here’s an example of how one might disable USB power management.
Windows Registry Editor Version 5.00 [HKEY_LOCAL_MACHINE\SYSTEM\CurrentControlSet\Control\usb] "DisableSelectiveSuspend"=dword:00000001
The fact that you’re encountering this error while using Selenium suggests that the issue could be related more specifically to ChromeDriver. Potential solutions can include:
Providing Full Path to ChromeDriver:
Ensure that you provide the full path to the ChromeDriver executable when initializing WebDriver. For instance:
from selenium import webdriver driver = webdriver.Chrome(executable_path=r'C:\path\to\chromedriver.exe')
But remember, even though these approaches can help, there’s no silver bullet for debugging issues because systems can vary dramatically. Stay patient, keep testing different options, and don’t be scared to ask the coding community for advice if needed.When updating your ChromeDriver for better compatibility, there are a few steps you’ll want to follow. The reason why some users might encounter the
Usb: Usb_Device_Handle_Win.Cc:1020 Failed To Read Descriptor From Node Connection Error With Chromedriver V87 / Chrome V87
on Windows 10 could be due to version compatibility issues between Selenium WebDriver and Google Chrome browser or an outdated ChromeDriver.
Step One: Check Your Version of Chrome
You’ll first need to verify the current version of your Google Chrome. This is easy to do by simply dropping the URL chrome://version/ into your browser’s address bar.
The top line – ‘Google Chrome’ provides the version number of your Chrome browser.
Example:
<p>Google Chrome: 87.0.4389.82</p>
Step Two: Download the Correct ChromeDriver
Once you have determined that our Chrome version is at least 87 or above, the next step is to download the compatible ChromeDriver from the ChromeDriver downloads page. Remember, compatibility between Selenium WebDriver and ChromeDriver is crucial for functional test automation using Selenium. So, always ensure they’re up-to-date.
Step Three: Replace the Old ChromeDriver
After downloading the correct ChromeDriver, replace the old one in its location. Simply go to the folder path where your previous ChromeDriver was installed and replace the old driver with the new one.
Example:
<p>C:\Users\\AppData\Local\Temp\
If you’ve already taken these actions and still encounter errors, it’s possible there may be other issues interfering. Here are a couple of additional tips that can help troubleshoot:
Frequent Debugging: Regular testing throughout the update process is key. Continually check your code to make sure it’s functioning properly at each step.
Monitoring the Action Log: Monitoring your action logs and error messages closely will give you insight into what exactly is happening when you run the code.
So, fundamentally, ensuring consistency and synchronization among Selenium WebDriver, Google Chrome, and ChromeDriver versions should eliminate the common
‘Failed To Read Descriptor From Node Connection’ error faced while using Selenium on Windows10.Browser automation is quite common in today’s development practices and Selenium plays a crucial role in that. However, while running your automation scripts using Chromedriver V87 with Chrome V87 on Selenium, you may encounter the error related to USB: Usb_Device_Handle_Win.Cc:1020 Failed to read descriptor from node connection. This error typically pops up when you’re leveraging Selenium for browser automation with Chrome.
This USB related error can be attributed to the internal way Google Chrome handles automated tasks. It’s important to understand that even though this appears as an error, it generally does not interfere with your automation tasks. That is to say, while this might be logged as an error, in practice it shouldn’t obstruct or misroute any of your automated tasks. So, as far as your Selenium automation testing is concerned, you are most probably safe but logging an error like this isn’t reassuring, so let’s look into possible solutions or workarounds.
options = webdriver.ChromeOptions() options.add_experimental_option('excludeSwitches', ['enable-logging']) driver = webdriver.Chrome(options=options)
This snippet of python code manipulates ChromeOptions to exclude ‘enable-logging’. Essentially, it suppresses the manifestation of the error by disabling the verbose logging which Chrome typically defaults to.
Here also comes another piece of a workaround that could be helpful, usually looking something like this:
capabilities = DesiredCapabilities.CHROME capabilities.add_argument("--disable-web-security")
By setting the DesiredCapabilities.CHROME and adding argument “–disable-web-security” could also lead to avoiding the same issue.
Again, these measures are more about suppressing the error message than fixing a functional problem, since this error does not seem to affect the functionality of Selenium tests in a meaningful way.
Preemptively figuring out such potential quirks and having a ready arsenal of methods and approaches ensures you can facilitate clean execution logs that ease debugging. While understanding the specifics of low level errors like this is somewhat outside the scope of typical Selenium usage since it involves the intricacies of how Google leverages Windows APIs under the hood, ensuring there are no hindrances to the execution of automation test plans makes for smooth deployment pipelines and quicker debugging.
However, if this message does end up being indicative of a larger issue or appears along with other failures, then a full bug report including system specifics, error logs, and relevant code snippets would need to be submitted to the ChromeDriver community for further assistance.
Remember, learning about testing challenges and effective workarounds is paramount for every coder involved in test automation and it’s what keeps us going amidst potential quirkiness that automation frameworks often surface.When a USB device fails to connect, it can be a frustrating experience. There are various reasons why a USB device may not connect properly, including driver issues, hardware problems, or software incompatibility. Specifically concerning Selenium users who use ChromeDriver version 87 and Google Chrome v87 on Windows 10, they might encounter a common error message: “usb_device_handle_win.cc:1020 Failed to read descriptor from node connection.” Let’s focus on what could potentially cause this issue and offer suggestions for troubleshooting it effectively.
Understanding the Problem:
The mentioned error is generated by an underlying Chromedriver or Chrome problem rather than Selenium itself. After analyzing this log snippet, we identified that this is more of a diagnostic message suggesting an attempt and failure to read something regarding USB—that by a piece of OS-level code in Chromium dealing with USB communication. This in itself does not signify a specific problem with Selenium automation scripts but may lead to unexpected behaviors.
Moreover, as a developer working on automation projects using Selenium, you normally wouldn’t deal directly with USB devices, which makes the appearance of usb-related messages perplexing.
Troubleshooting Tips:
The first step towards resolving this issue involves considering the following:
– Chrome / ChromeDriver Compatibility: A concise way to combat the error is ensuring the compatibility between the Chrome browser and the ChromeDriver. To ascertain they align, your ChromeDriver must correspond to the version of your Chrome Browser. It’s beneficial to frequently check the ChromeDriver download page for updates so your version doesn’t lag. You can even utilize WebDriverManager, which aids in managing WebDriver versions automatically.
from webdriver_manager.chrome import ChromeDriverManager from selenium import webdriver driver = webdriver.Chrome(ChromeDriverManager().install())
– Highest Admin Privileges: Run your automation cases as an administrator or user with the maximum privileges. On Windows, typically a right-click on the script file followed by ‘Run as Administrator’ should suffice.
– Clear Browsing Data: Sometimes, the accumulation of browser data can create unwarranted issues. Clear your browsing data by going to ‘Settings > Privacy and Security > Clear browsing data’ and wipe out ‘Cookies and other site data’ as well as ‘Cached images and files’.
– Update Operating System: Even though this issue arises mainly due to Chrome / Chromedriver, keeping your operating system up-to-date is always advisable. An update will rectify any bugs, enhance performance and speed, and mend security holes in the current installed OS.
Beyond these primary solutions, if you’re still experiencing discomfort from the shared error message, you could disable verbose logging. Although this method won’t resolve the underlying concern (as it’s a Chrome defect), it would refrain from displaying the error in your console logs. Use the below Python snippet to demonstrate this:
from selenium import webdriver options = webdriver.ChromeOptions() options.add_experimental_option('excludeSwitches', ['enable-logging']) driver = webdriver.Chrome(options=options)
Finally, remember that the key to resolving this kind of error requires maintaining up-to-date software, ensuring you have the most fitting permissions set up, and clearing out redundant browser data. By taking these measures, you can overcome similar issues and guarantee smooth operations when conducting automated testing with Selenium.The USB Device Handle Win Cc error is primarily caused by incompatibility issues between Chromedriver v87 and Chrome v87 on Windows 10, subsequently resulting in failure to read the descriptor from the node connection while utilizing Selenium. Well, this can be quite a head-scratcher for both novice and experienced coders dealing with the Selenium framework.
$ (DesiredCapabilities capabilities = DesiredCapabilities.chrome(); WebDriver driver = new ChromeDriver(capabilities););
Bear in mind that when using Selenium along with Chrome browser version 87 on Window 10 operating system, the ideal situation would be matching it with Chromedriver version 87. This goes a long way into ensuring smooth synchronization and operation, thereby eliminating chances of encountering the USB Device Handle error at all.
Now you might be asking yourself, “Alright! But what if i still encounter this error?”
– Check Your Browser and Chromedriver Compatibility: Ensure that your Chromedriver’s version matches that of the Chrome browser you’re using. Mismatched versions often trigger such issues. You can manually set the property for Chrome’s path using
System.setProperty()
System.setProperty("webdriver.chrome.driver", "/path/to/chromedriver"); WebDriver driver = new ChromeDriver();
– Update Software Regularly: Besides having compatible versions, always ensure both your Chrome browser and Chromedriver are up-to-date. Selenium operates optimally with latest software versions.
– Influence Path Variables in Code Directly: If handling environment variables gets chaotic, you have an option of influencing or setting the path of chromedriver directly in your code. Like so:
System.setProperty(“webdriver.chrome.driver”, “/path/to/directory/");
This way, you bypass the need for PATH variable environment setup and reduces the scope for errors.
But there’s something more than meets the eye. In reality, the common ground that bridges all these factors together is where stackoverflow comes inStackOverflow Guide. Forums like these make coding easier, they’re a treasure trove of guidelines, solution scripts, troubleshooting techniques and much more.
Let’s wrap this up now! Everything comes down to being attentive to chromium compatibility and regular updates. With conscious efforts in managing versions and understanding underlying errors, we could prevent USB Device Handle Win Cc error from reoccurring. So, dig deeper, learn more, and level up!