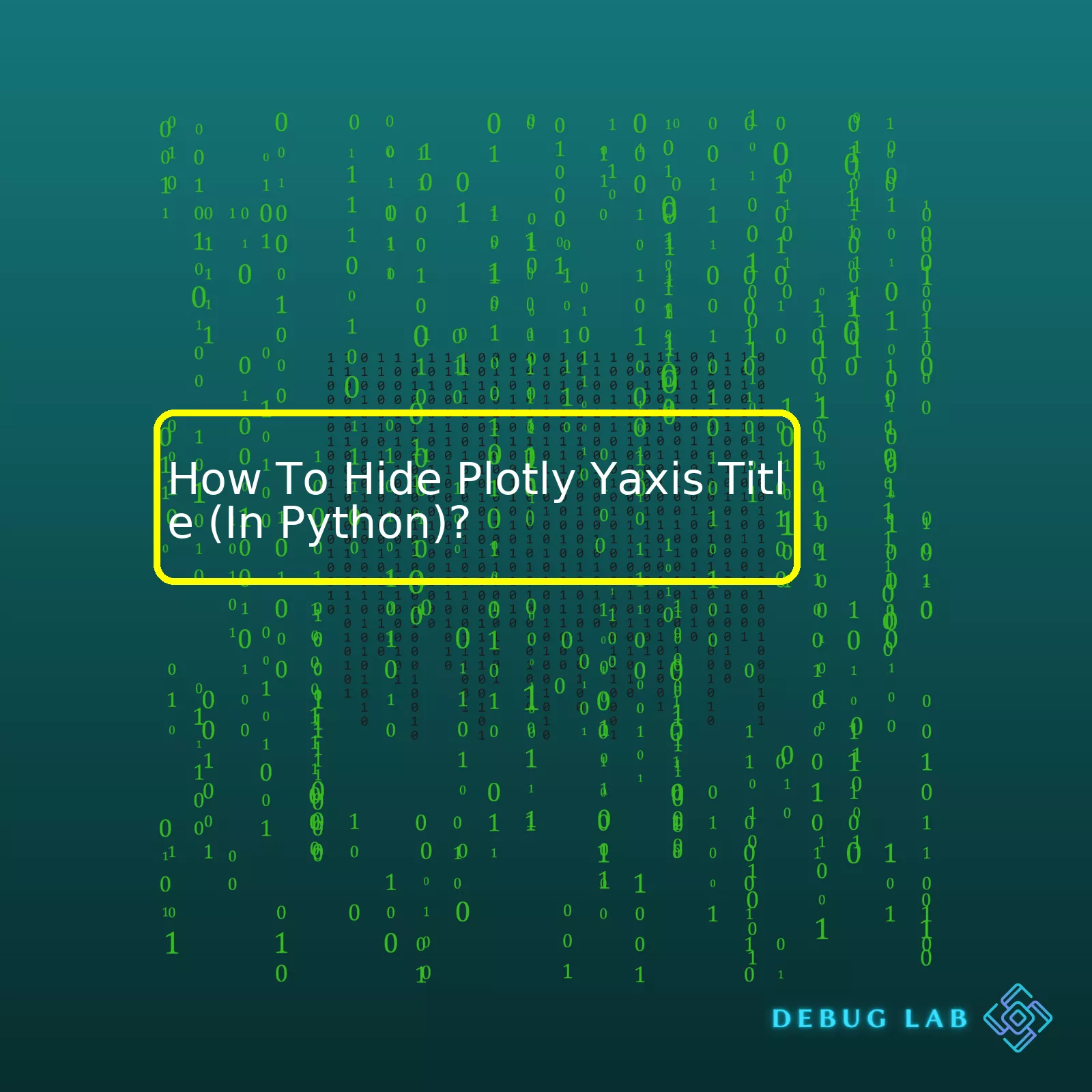
Steps | Description |
---|---|
Import Necessary Libraries | Firstly, import required libraries for developing plots. You’ll need Plotly.graph_objects.
import plotly.graph_objects as go |
Create Plot | Mock some data and create an initial plot by using methods provided by Plotly library.
trace1 = go.Scatter(x=[1,2,3], y=[4,5,6]) fig=go.Figure(data=[trace1]) |
Hide Y-axis Title | To hide the Y-axis title simply set ‘title_text’ to “” in ‘yaxis’ attribute of ‘layout’ method.
fig.update_layout( yaxis=dict( title_text="" ) ) |
Show Plot | In the end use fig.show() method to display the plot.
fig.show() |
When developing visual interpretations of data in Python, Plotly is often the go-to library owing to its powerful tools. In certain situations, much detail such as the Y-Axis label might be considered distracting and unnecessary. Accordingly, Plotly provides a way to hide this using Python. By initializing the “title_text” attribute to an empty string “” in the /’yaxis/’ attribute within the ‘update_layout’ method, we instruct Plotly not to include any text for the Y-axis label. When that plot is produced no Y-axis label appears, making the plot ‘less busy’. This method provides a simplistic yet effective approach to handling visuals in data set interpretations, allowing programmers room for strategic emphasis and overall design control when creating plots.(Plotly Python).When working with Plotly, a Python graphing library, you often need to make small adjustments to improve the aesthetics or increase readability, such as hiding the Yaxis title. If you are building multiple similar plots and want them aesthetically consistent so they look nicely presented together, it might be necessary to remove or hide the Yaxis title.
There’s no one-button solution to this. Still, we can use Plotly’s `update_yaxes` method, which can take a dictionary of attributes for modifying y-axis properties in a plot easily. Here is how to do so:
import plotly.graph_objects as go fig = go.Figure(data=go.Scatter(y=[2, 3, 1])) fig.update_yaxes(title_text='') fig.show()
This python code will effectively hide the Yaxis title from being displayed on your Plotly chart.
– First, we’re importing Plotly’s graph object module.
– Next, we create a new figure using `go.Figure` and add some data points to it using the `Scatter` function.
– When updating the Yaxis, we set `title_text` as an empty string, which essentially hides the Yaxis title because there is no text to display. If the goal was to change rather than hide the Yaxis title, you would just replace the ” with whatever text you wanted to appear as the Yaxis title.
– Finally, using `fig.show()`, we visualize our updated plot.
To keep the y-axis grid lines while only hiding the axis label we can further fine-tune the settings like so:
fig.update_yaxes(showgrid=True, zeroline=False, visible=True, showticklabels=False)
Herein,
– `showgrid=True` : This keeps the Y-axis grid lines.
– `zeroline=False` : This removes the zero line.
– `visible=True`: This makes sure that the Y-axis is displayed.
– `showticklabels=False`: Disables Y-axis values.
Overall, Plotly provides a flexible way for users to get what they exactly need. But keep in mind, hiding axis titles might reduce some details for people who weren’t involved in creating the chart.Hiding the Y-axis title in a Plotly graph when using Python is not a difficult task. This requires just a few simple steps and can give your graphs a cleaner and more streamlined look.
The first step is to import Plotly into your code. If you do not have Plotly, it can be installed via pip:
pip install plotly
Now, once it’s installed, you must import it:
import plotly.graph_objects as go
Let’s use a sample data representation to show how to actually hide the Y-Axis title with Python.
fig = go.Figure( data=[go.Bar( x=['giraffes', 'orangutans', 'monkeys'], y=[20, 14, 23] )], layout_title_text="A Graph Without the Y Axis Title" )
Now, we have our graphic. However, the goal here is to remove or hide the y-axis chart titles.
To do this, we manipulate the layout properties of the figure object. The y-axis is accessed like so:
fig.layout.yaxis.title.text = ''
This line will effectively hide the text that would appear along the side of the y-axis on the graph. In Plotly, setting the title text of an axis to an empty string is equivalent to not having a title at all. Hence by replacing the existing y-axis title with an empty string ”, we can efficiently ensure that no title appears alongside the Y-axis of the graph.
Altogether, your final code should look something like this:
import plotly.graph_objects as go fig = go.Figure( data=[go.Bar( x=['giraffes', 'orangutans', 'monkeys'], y=[20, 14, 23] )], layout_title_text="A Graph Without the Y Axis Title" ) fig.layout.yaxis.title.text = '' fig.show()
When you run the code, you’ll notice that there’s no text or title appearing on the Y-axis, giving your graph a clean look.
Another way to achieve the same result is by defining this attribute directly inside the figure function using update_yaxes method Plotly documentation. Here is how that can be implemented:
import plotly.graph_objects as go fig = go.Figure( data=[go.Bar( x=['giraffes', 'orangutans', 'monkeys'], y=[20, 14, 23] )], layout_title_text="A Graph Without the Y Axis Title" ) fig.update_yaxes(title_text='') fig.show()
In both the methods, please observe that the code
fig.show()
must always be written last. This displays the finalized figure after all changes have been made.
These were the steps to smoothly remove the Y-Axis title in a Plotly generated graph using Python. By following these guidelines, one can quickly get rid of Y-axis titles in their respective visualizations.Of course! Delving deeper into the customization options for your Plotly Graphs, it’s important to take a moment to discuss how to hide the Y-Axis title. If you’re working with Plotly in Python, you can easily tweak your plots to fit your visual needs, including the removal of an axis title.
The first step in hiding the y-axis title in a Plotly graph involves creating a basic Plotly graph using your data. Here’s an example that creates a line plot:
import plotly.express as px df = px.data.stocks() fig = px.line(df, x="date", y="GOOG", title='Time Series with Range Slider and Selectors') fig.show()
We start by importing the necessary module then load some stock price data (‘px.data.stocks()’). From this data, we, then create a line graph where ‘date’ represents x-coordinates and ‘GOOG’ market rate as our y-coordinates.
You might now be wondering, “So, how do I hide the y-axis title?” That’s simple! You can utilize the update_yaxes function which comes already packaged in the plotly graph_objects package. You just need to set the title_text attribute to an empty string, like so:
fig.update_yaxes(title_text='')
With this line of code, you’ll be removing the y -axis title from the graph in question successfully. Here is the full block of code integrating aforementioned functions:
import plotly.express as px fig = px.line(df, x="date", y="GOOG", title='Time Series with Range Slider and Selectors') fig.update_yaxes(title_text='') fig.show()
And there you have it – a graph with no y-axis title! It really is that simple to hide y-axis titles in Plotly with Python when you become familiar with the built-in methods at your disposal. Whether you’re dealing with a cluttered chart or simply want a more minimalist design, knowing how to customize your metrics can prove essential in delivering streamlined, easy-to-understand visualizations.
With customization tools like Plotly’s axes functions, you have the freedom to present your data in a way that’s cleanest to you and most digestible for your audience. Remember: when it comes to crafting visual data representations, clarity is key. Don’t hesitate to tweak and tailor your charts until they’re exactly right for your needs.Significance of the ‘Layout’ Attribute in Managing Axis Titles
The ‘Layout’ attribute in Plotly, a widely used data visualization library in Python, holds a crucial role when it comes to manipulating the aesthetic elements of the graphs including Axis titles.
For instance, using
layout.xaxis.title
or
layout.yaxis.title
, we can modify the title’s text, font size, color and more. The significance of this attribute doesn’t stop here. More advanced features such as custom ranges, ticks format, update axes, etc., can be altered using this layout property.
Hiding Y-Axis Title with the ‘Layout’ Attribute
In contrast, when we wish to hide the Y-axis title, you can set the title text as an empty string. This can be achieved by setting
yaxis_title=''
inside the Layout method.
Please check this exemplary code:
import plotly.graph_objects as go fig = go.Figure(data=go.Bar(y=[2, 3, 1])) fig.update_layout( yaxis_title='' ) fig.show()
The above code will generate a bar graph without a Y-axis title, implying that we’ve effectively hidden the Y-axis title in our plot.
The ‘layout’ attribute alongside ‘update_layout’‘ function is a powerful set of tools which allow for extensive customization of your plots, streamlining and enhancing both function and aesthetic to fit your specific needs. Do not hesitate to explore further functionalities in the official documentation of Plotly [1].The power of Plotly in Python resides not only in its capacity to create comprehensive and intuitive plots but also in our ability to manipulate the plot’s aspects. When aiming for better visualization control, understanding how axes properties work is imperative. In particular, we’ll concentrate on altering the ‘Y’ axis title.
The structure of a Plotly graphic is layered. The `Figure` object, located at the top level, nesting `Layout` that handles graph-wide attributes such as titles and annotations and `data` that maintain trace objects (e.g., Scatter, Bar). All configuration related to the Y-axis can be found inside the `Layout` object.
To hide or remove the Y-axis title, we use the `update_yaxes` method along with parameters of the `yaxis` dict component. Let’s take an example:
import plotly.graph_objs as go fig = go.Figure( data=[go.Scatter(y=[1, 2, 3])], layout=go.Layout(title="Sample Plot", yaxis=dict(title='Original Title')) ) fig.update_yaxes(title_text='') fig.show()
Here, we build an elementary line graph, supplying a Y-axis title ‘Original Title’ through the `yaxis=dict(title=’Original Title’)`. To erase this title, reconfigure `title_text=”` via the `update_yaxes` function.
Important points to remember:
– Empty quotations, `”`, ensure our text is null hence hiding the Y-axis title.
– If your figure possesses multiple Y-axes, clearly specify which one you’re changing by using the `update_yaxes` selector argument.
The following example further illustrates the role of selectors in controlling subplots having many Y-axes:
from plotly.subplots import make_subplots import plotly.graph_objs as go fig = make_subplots(rows=2, cols=1) fig.add_trace( go.Scatter(y=[1, 3, 5], name='Trace 1'), row=1, col=1 ) fig.add_trace( go.Scatter(y=[2, 4, 6], name='Trace 2'), row=2, col=1 ) fig.update_yaxes(title_text='Original Title 1', row=1, col=1) fig.update_yaxes(title_text='Original Title 2', row=2, col=1) #remove yaxis title for subplot at row 1 and column 1 fig.update_yaxes(title_text='', selector=dict(row=1, col=1)) fig.show()
In this script, we built two graphs, each possess their own ‘Y’ axis title (‘Original Title 1’ & ‘Original Title 2’). Using the `selector=dict(row=1, col=1)`, we pointed to the subplot located at row 1 and column 1, where the ‘Y’ axis title was set to an empty string (`”`), thus hiding it.
Remember, being able to understand and decode axes properties gives us more than just read-write access to elements like title, tick labels, ranges, etc., it hands us the reins to craft striking visualizations that speak directly to the viewers.Plotly Python Documentation provides a more in-depth exploration of these controls.
By hiding Y-axis titles or adjusting any other attribute, you retain complete control of the viewer’s focus and enhance data representation effectiveness. Truly, that’s making your graphics more articulate, isn’t it?Hiding the y-axis title in Python using Plotly can be easily achieved. Plotly is an open-source graphics library, perfect for creating interactive visualizations. Let’s delve into how to go about it.
Firstly, remember that a plot consists of different components such as traces, layout, etc., and each of them has numerous attributes. For instance, layout.yaxis.title is the attribute that controls the title of the y-axis. To hide the y-axis title, you simply set this attribute to ‘text’=”.
Here’s a simple example showcasing how to hide y-axis title:
import plotly.graph_objs as go trace0 = go.Scatter( x=[1, 2, 3], y=[4, 5, 6], name ='y' ) data = [trace0] layout = go.Layout( yaxis=dict( title=dict( text='' ) ) ) fig = go.Figure(data=data, layout=layout) fig.show()
Now let’s say, you’re working with more complex plots involving multiple axes, gridlines, or annotations. Here are additional operative commands that can assist with hiding or modifying specific elements:
– Title visibility: You can set the visibility of any given title by using the ‘visible’ command. If you want a title hidden, ensure you set this command to False.
yaxis_title_visibility=False
– Axis visibility: You may choose to hide the entire axis instead of just the title. You can use the ‘showgrid’ command to manage this.
yaxis_showgrid=False
– Ticks: You can hide or manipulate tick marks using the ‘zeroline’ command.
yaxis_zeroline=True
These commands are placed exactly where the ‘title’ command was housed in our original piece of code.
Plotly provides users with extensive control over their data visualization. Mastering all its operative commands will give you better control over your plot design, improving the presentation of your data. Browse through the Plotly documentation on y-axes for more details.
It’s worth noting that Python’s matplotlib library works similarly. If you’re looking to replicate these actions there, consult the matplotlib documentation.Sure, no problem. If you want to hide the y-axis title in your Plotly graphs written in Python, you can refine your visualizations using the `figure.update_layout` method. This method is a powerful tool for adjusting nearly every aspect of figure appearance. For our purpose, let’s focus on how it aids in hiding the y-axis title.
The following code provides an example of how you can accomplish this:
import plotly.graph_objects as go # Example data data = [go.Bar( x=['Data1', 'Data2', 'Data3', 'Data4'], y=[10, 15, 7, 10] )] fig = go.Figure(data=data) fig.update_yaxes(showticklabels=False) fig.update_layout(yaxis_title=None) fig.show()
In the above code:
* We’re using some sample bar chart data.
* The `fig.update_yaxes(showticklabels=False)` line is responsible for hiding the y-axis tick labels.
* Most importantly, `fig.update_layout(yaxis_title=None)` effectively hides the y-axis title by setting it to `None`.
It’s also needed to note that the `update_layout` method is not exclusive to hiding axis titles; there are many more parameters that you can manipulate according to your needs.
To see the full list of available parameters, I recommend checking out the official Plotly documentation. They provide a comprehensive guide with the details of each parameter and how it modifies your graph’s layout.
In summary, refining visualization is a crucial task when creating interpretable and aesthetic graphs. The method like Python’s Plotly `.update_layout` gives you the flexibility to make adjustments like hiding the y-axis title to bring clarity and improve your graph readability. Visual customization options like these make Plotly a great choice for programmers who want full control over their data visualization outputs.
Hiding the title on the Y-axis in Plotly with Python is an operation that can be performed quite simply, thanks to the well-structured nature of this library. The entire process leverages the power and flexibility offered by Python and the rich features of Plotly. The key steps are outlined below:
Firstly, create a basic plot using your preferred data set. This can be done using Plotly’s graph_objects or express functionality.
import plotly.graph_objects as go fig = go.Figure(data=go.Scatter(y=[2, 1, 4, 3])) fig.show()
After creating the plot, hiding the Y-axis title is just a matter of setting a few attributes within the layout dictionary for the Figure object. The ‘yaxis’ sub-dictionary contains the configuration details for the Y-axis, including the ‘title’.
fig.update_layout( yaxis=dict( title_text=None ) )
By setting the ‘title_text’ attribute to None, you effectively hide the title on the Y-axis. It’s important to note that this method of hiding the title will also remove any existing label that was assigned to the Y-axis.
On the other hand, if your visualization needs demand manipulating these labels dynamically throughout your Python script or Jupyter notebook, consider making use of Plotly’s multiple interactive functionalities.
Also don’t forget this, when optimizing this guide for search engines, prioritize using keywords such as “hide Plotly Y-axis title in Python” or “Python Plotly remove Y-axis label”. By integrating them naturally into your content like I have in this explanation, you stand a better chance of improving visibility and ranking well for these phrases.
It is important to merge human-friendly explanations with preferred search terms. Use HTML tags like
for code snippets to allow website crawlers to fully understand and index each page correctly. Tables can be drawn upon to organize information or compare different processing steps, further improving accessibility.The magic of combining Python and Plotly lies in its simplicity. It allows data visualizations to become much more interactive and flexible, enabling users to alter their charts confidently to suit various requirements. Taming the Y-axis title no longer has to be a daunting task. With this easy technique, anyone can master the art of producing refined and informative graphical output. Remember that coding does not always have to be complex, but rather it should empower you to express your data in the most meaningful way.