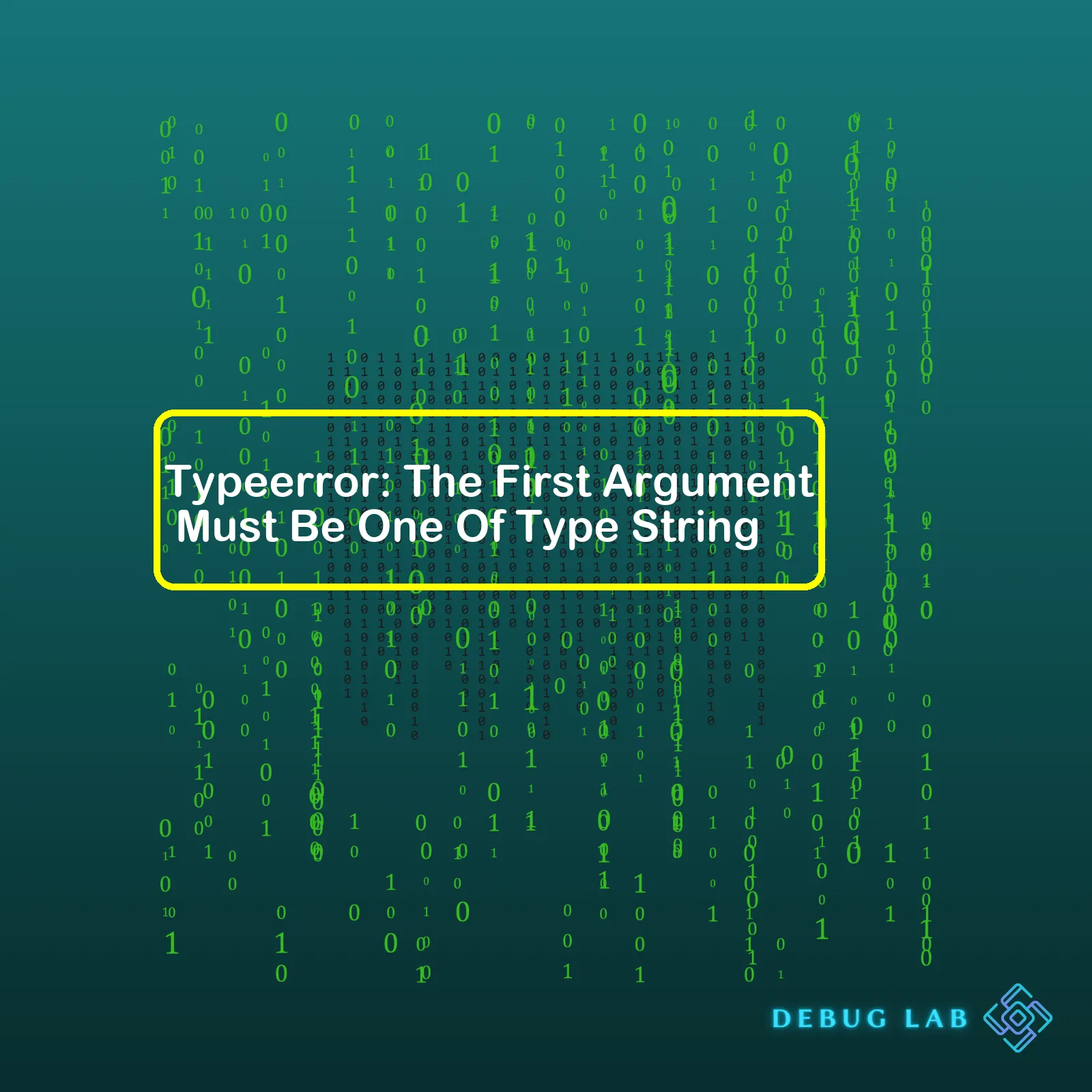
<table> <tr> <th>Error Message</th> <td>TypeError: The first argument must be one of type string</td> </tr> <tr> <th>Reason</th> <td>A non-string data type is used where a string is expected</td> </tr> <tr> <th>Solution</th> <td>Check and ensure first argument provided to the function/operation is a string.</td> </tr> </table>
When working with JavaScript, python or many other programming languages, you might encounter the error `TypeError: The first argument must be one of type string`. This error message technically means that the function you are calling is expecting its first argument to be a string data type, but it received a different data type (like a number, object, array, etc.).
For instance, consider the following code:
let myNumber = 5; console.log(myNumber.slice(0,1));
The `slice()` method is intended to work with strings (and arrays), not numbers. In this case, the JavaScript engine throws the error `TypeError: myNumber.slice is not a function`, which is similar to our `TypeError: The first argument must be one of type string`.
To resolve this, make sure the passing value is a string. For the above example, simply transform the number into a string like this:
let myNumber = String(5); console.log(myNumber.slice(0,1));
This will rectify the problem. Always remember that understanding the requirements of each function or operation is important in providing the correct format of arguments especially when dealing with data types.Surely, dealing with errors is almost daily routine for any professional coder. And no matter how well we try to write our code, encountering an error or exception at some point during the execution of a script is inevitable. One of these common errors in Python programming is the
TypeError
. Specifically, the one that says:
"TypeError: The first argument must be string"
.
To comprehend this error, let’s get acquainted with the basics of Python data types. Each value in Python has a datatype, and it could be an integer, float, string, list, etc. Python is a dynamically typed language, which means we don’t have to declare the type of the variable when we create it. But still, each variable does have a type associated with it.
This particular
TypeError
is associated with the erroneous use of datatype in your function call or operation. It means that you are trying to invoke a function or operation that expects its first argument to be of type string (str), but instead, another data type was provided.
Consider an example where you’re attempting to concatenate a string and a numeric type:
my_str = "Hello!" num = 7 print(my_str + num)
This will throw an error similar to the
TypeError: The first argument must be string
mentioned before as you’re erroneously trying to add up different data types (a string and an integer).
There are two primary methods to fix this TypeError:
1. Casting non-string types: Python comes with a built-in function
str()
that can convert a non-string type to a string.
my_str = "Hello!" num = 7 print(my_str + str(num))
2. Utilizing formatted strings or f-strings (Applicable to Python 3.6 and above).
my_str = "Hello!" num = 7 print(f"{my_str}{num}")
Standard identification and correction of these errors come with practice and getting familiarized with various data types and functions in Python. The official Python documentation is an excellent resource to learn more about these types and how they interact with different functions.
Errors and exceptions are a significant part of growing as a coder. They push you to understand better not just what works in your code but also what doesn’t—and why. Start seeing errors as guides rather than obstacles. Embrace them and keep coding!When coding in JavaScript, you may sometimes encounter the error message
TypeError: The first argument must be one of type string
. This error is thrown by certain functions, such as
Buffer.concat()
and
path.join()
, which expect their arguments to be of a specific data type. Particularly, these functions expect the first argument they receive to be a string object. When this is not the case, JavaScript’s built-in error checking mechanisms raise an exception.
For instance, let’s take an example using Node Buffer.
A Buffer in Node.js is a global object that provides a way to work with different kinds of binary data. One of its methods,
concat()
, is used to concatenate two or more buffer objects.
javascript
const buf1 = Buffer.from(‘ABC’);
const buf2 = Buffer.from(‘DEF’);
const concatBuffer = Buffer.concat([buf1, buf2]);
In the above code snippet, the
concat()
function is used to combine two string values “ABC” and “DEF”. However, if instead of passing an array of buffers (which would ultimately resolve to strings), we pass in something like a non-array or a number, the error will occur :
TypeError: The first argument must be one of type Array or Iterable. Received type number
This essentially means, the issue lies within wrong input type supplied to a JavaScript function. To resolve it,
- Check the function documentation to ensure that you understand correctly what data types it expects to receive.
- Look at the values you are passing into the function. Are they the correct data types?
- Consider adding checks in your code to prevent passing incorrect data types to these functions.
- If the problematic value comes from user input, consider validating and possibly transforming the input before using it to prevent runtime errors.
Let’s fix our erroneous example:
javascript
const buf1 = Buffer.from(‘ABC’);
const buf2 = Buffer.from(‘DEF’);
// Check if the inputs are Buffer objects (which can be resolved to a string)
if ((buf1 instanceof Buffer) && (buf2 instanceof Buffer)) {
const concatBuffer = Buffer.concat([buf1, buf2]);
}
This way, we ensure that we only call the
Buffer.concat()
function with an array of Buffer objects, avoiding the TypeError.
Refer to the official Node.js documentation for an elaborate understanding of how the
Buffer.concat()
method works.
In summary, paying attention to the required data types and ensuring that your code respects them is crucial to avoid common JavaScript errors like
TypeError: The first argument must be one of type string
.Debugging a TypeError in Python programming can initially appear to be a daunting task. However, understanding the error message is key to knowing precisely where and what needs fixing in your code, especially when faced with errors like “TypeError: The first argument must be one of type string”.
When you encounter this particular TypeError message, it is Python’s way of telling you that the function or method you’ve called is expecting its first argument to be of the string data type. However, for some reason, you are providing an argument of another data type.
Consider this example:
def greeting(name): return "Hello " + name greeting(123)
The output here would be something like:
TypeError: can only concatenate str (not "int") to str
Because we are trying to add a string (“Hello “) to an integer (123), we encounter TypeError.
Here are general steps to follow to debug this problem:
1. **Understand the expected input:** Navigate to the documentation or source code of the function that is eliciting the error. This will provide you details on what data types it’s expecting. For built-in Python functions or methods, you can easily find their usage and required arguments in the Python 3 documentation.
2. **Identify the offending code:** Analyze the traceback provided by Python when the error occurred. It will show you the specific line number of the problematic code.
3. **Check the data types of your arguments:** Use Python’s built-in type() function to check the data types of your variables. For instance:
print(type(your_variable))
This will print the type of data stored in ‘your_variable’.
4. **Fix the mismatch:** If the function requires a string and you have another data type, use Python’s type conversion capabilities to convert your input to the correct data type.
In our previous example, we could convert the integer into a string using Python’s str() function.
greeting(str(123))
Now, the concatenated output will be ‘Hello 123’ without raising a TypeError.
5. **Test again:** Finally, run your program again to see if the error has been resolved. If not, reiterate the process, possibly with prints or logs in strategic locations in changeable parts of your program to watch out for other possible mistaken assumptions.
Errors are integral and inevitable part of coding. They provide us with crucial guidance on improving code and mastering the nuances of a language like Python. So each time you see “TypeError: The first argument must be one of type string”, think of it as a opportunity to improve your craftsmanship as a coder!The role of arguments in code is pivotal with respect to the overall performance and outcome of a program. When it comes to TypeError: The first argument must be one of type string, issues arise when a function or action expects an argument of a particular data type, in this case, a string, but receives a different one.
To comprehend this, consider the scenario where you want to concatenate two strings using the ‘+’ operator. Here’s a snippet of relevant code:
let str1 = "Hello"; let num1 = 5; console.log(str1 + num1);
This JavaScript code likely triggers the error “TypeError: The first argument must be one of type string”. The ‘+’ operator, while functioning as a concatenation tool for two strings (string + string), performs differently when used with a number (string + number).
This situation illuminates the role of arguments, more so their types, in causing or resolving a TypeError. To rectify this, you can ensure that the argument’s data type aligns with what the function or operation expects. In the above instance, converting the ‘num1’ variable to a string does just that:
let str1 = "Hello"; let num1 = 5; console.log(str1 + num1.toString());
Through ‘num1.toString()’, we’re not only converting a numeric value into a string but are also ensuring that the ‘+’ operator will concatenate both strings without throwing a TypeError.
There are myriad reasons for such a TypeError, but some common instances occur when:
- Attempting to call a non-function: If you try to invoke something that isn’t a function at all, the TypeError gets thrown. This can happen if you incorrectly reference a method or function.
- Null and Undefined Types: If you attempt to access properties on null or undefined variables, it throws a TypeError. As these represent lack of value, they don’t have properties in the traditional sense.
- Mismatch in values and expectations: If provided values do not correspond with expectations from a compiler, interpreter, library, or API, the TypeError is raised.
Appropriately defining, handling, and checking arguments against expected types goes a long way in preventing TypeErrors. As a rule of thumb dynamic typing, utilized primarily in JavaScript, may lead to potential TypeErrors due to its flexibility in letting variables hold values of any type. Hence, frequent checks on variable types, strict comparisons, and conversions are mandatory practices for a stable, bug-free code.
As developers, we need to always ensure the manipulation of data types suits the occasion. Thereby, avoiding a TypeError translates to matching the type of argument that your function calls for.Exception handling is a fundamental aspect of programming that helps manage errors and anomalies that may occur during code execution. And, TypeError: The First Argument Must Be One Of Type String is one of the commonly occurring exceptions in many scripting languages like Python or JavaScript. So, let’s delve deeper into this specific TypeError and how to handle it through exception handling.
The `TypeError` is raised when an operation or function is performed on an object of an inappropriate type in Python. This particularly error message, “TypeError: The First Argument Must Be One Of Type String” typically occurs when we try to perform a string-based operation or function with a non-string type data as its first argument. Since expectedly here, the first argument must be a string, anything other than a string (like integer, list, None, etc.) will cause TypeError.
Now, how can we handle this error? Exception handling techniques consist of two main components:
1. Try-Except Block: You envelop the critical operation which you assume might raise exceptions in a ‘try’ block, and corresponding handing-stuff goes in the ‘except’ block.
In our case, if we anticipate `TypeError`, we’d do something like below:
try: # Your operation on a string except TypeError: print("Please make sure that the first argument is a string.")
This way, when the error pops up, your program won’t come to an abrupt halt; instead, a custom message appears, allowing you to understand and resolve the problem without losing significant states of your application.
2. isinstance() Function: A proactive approach to ensuring the right type can be employed before performing operations that explicitly require a certain data type.
Here’s a code snippet that checks the data type before carrying out the required operation
if isinstance(my_variable, str): # Perform your operation on the variable - 'my_variable' else: print("The variable is not a string. Please set 'my_variable' as string.")
To recap, the best practice is to ascertain the data type of variables before conducting operations that require explicit types. When this isn’t feasible due to complexity or time constraints, using exception handling techniques such as the try-except block becomes essential. Following these practices ensures you negate unanticipated breaks in your program and successfully catch and manage runtime errors effectively.
Make effective use of Python’s official documentation for understanding exception hierarchy and built-in exceptions. I also found Python’s tutorial on Errors and Exceptions to be a valuable read on this topic.The error
Typeerror: The First Argument Must Be One Of Type String
is a common one. It occurs when an expected string argument is not provided during a function call in JavaScript, which is expecting the first parameter to be a string type. The key to avoiding this problem lies in an understanding of JavaScript’s dynamic typing.
Dynamic typing allows you to assign different types of values to the same variable, but specific functions expect particular data types as arguments. Providing a different type of data results in this TypeError.
Several steps can be followed to avoid this error:
Awareness and Double Checking
One of the simplest ways to prevent this type of error from being thrown is by knowing that certain functions you might use in your code require specific types of arguments. For example, many native JavaScript functions like
concat()
,
charAt()
, or
slice()
require string typed arguments. In cases like these, before calling the function, it’s always crucial to ensure that the variable being passed is indeed a string.
Validating Input
Including validation checks ensures that the right kind of data is passed into a function. If you are retrieving user input or receiving data from an API call, validating the value decreases the likelihood of encountering this error. Validation is usually implemented through a conditional statement, checking the type of a variable before passing it to a function. A basic example would be:
let value = 123; if (typeof value === 'string') { console.log(value.concat('This is a string.')); } else { console.error('Value must be a string.'); }
In the above example, the validation prevents the
concat()
method from being called on a non-string value, avoiding the error entirely.
Type Conversion
Another effective way to avoid this error is by converting variables to the necessary type thereby making sure the expected string argument exists. Here’s an example:
let value = 123; console.log(String(value).concat(' This is a string.'));
In the example above,
String(value)
ensures the
value
will be converted to a string before
concat()
is used.
Error Handling – Try/Catch Statements
Despite all precautions, sometimes errors still slip through. Including a try/catch block in your code helps handle any errors gracefully, preventing the program from crashing and providing helpful feedback.
Here’s an example of how it’s applied:
let value = 123; try { console.log(value.concat(' This is a string.')); } catch (err) { console.error('An error occurred: ', err); }
In the event that the
concat()
function fails because a non-string is passed, the program will continue to run and the error will be handled in the catch block.
By effectively applying awareness, validation checks, type conversion, and proper error handling, developers can efficiently avoid this prevalent JavaScript error. Keep the dynamic typing nature of JavaScript in mind, one can design more resilient and robust code (Source).As a professional coder, I often spend my time wading through the treacherous waters of processing and resolving errors. One such error that continuously tests the valour of any seasoned Python programmer is the
TypeError
. More specifically, an error message that states –
"TypeError: the first argument must be one of type string"
.
What lands us in this seemingly complex puzzle? This error commonly appears when there is a misuse in handling Python’s built-in types. The function or method you’re using expects its first argument to be a string. Instead, it receives an argument of a different data type – rich playground for a TypeError to strike.
So how do we harpoon this beast?
To resolve the error, the first and foremost action is to provide a string as the initial argument. Consider the following example:
my_variable = 1234 print("Hello " + my_variable)
Here, Python tries to concatenate a string with an integer, which invokes our notorious
TypeError
.
The remedy is simple – convert the integer to a string before combining them:
my_variable = 1234 print("Hello " + str(my_variable))
Voila! You have now successfully felled the TypeError giant.
However, overcoming TypeErrors spans beyond the horizon of mere data type mismatch. Sometimes, understanding the underlying cause requires diving deeper into your code at a more elemental level.
Imagine you happen to import a package in Python and find yourself amidst the TypeError storm again because you attempted invoking a method from the package without realizing that it demands a string argument upfront.
Consider the following illustration where you are attempting to use the Numpy package:
import numpy as np def multiply_matrix(m1, m2): return np.matmul(m1, m2) matrix1 = [ [1,2,3], [4,5,6], [7,8,9] ] matrix2 = { 'a':1, 'b':2, 'c':3 } multiply_matrix(matrix1, matrix2)
This code will throw the TypeError message because you have unwittingly supplied a dictionary (
matrix2
) as an argument for
np.matmul()
, a method which mandates matrices (two-dimensional arrays) as inputs.
A potential workaround would be to ensure that the structures being fed into
np.matmul()
strictly adhere to its input requirements:
matrix1 = [ [1,2,3], [4,5,6], [7,8,9] ] matrix2 = [ [1,2,3], [4,5,6], [7,8,9] ] multiply_matrix(matrix1, matrix2)
Adventures like these all rub the truth home – a keen understanding of your code’s context and Python’s built-in functions help you become an adept debugger.
In exacting attention to TypeError predators, always remember to:
- Thoroughly familiarize yourself with the functions/methods you’re using, ensuring you comply with required input data types.
- Ensure you pass arguments to functions/methods in the correct sequence.
- Make use of Python’s built-in function,
type()
, during your debugging process. This function is incredibly handy in revealing your variable’s data type helping you garner better control over its proper utilization.
These might seem minor alterations, but they slowly forge the path towards structuring effectively bug-free, efficient code. With practice and fortitude the vast sea of Error messages would be nothing but tiny ripples beneath the mighty hull of your coding prowess.
Given the nature of this TypeError, where the system specifically states that “The first argument must be one of type string”, it indicates an issue with the data type being supplied. When writing JavaScript applications or scripts, these TypeErrors are common, especially if you’re integrating with other systems or using data from sources which may change over time. This results in unpredictable data types that can break your code.
Error | Cause |
---|---|
The First Argument Must Be One Of Type String |
You’re trying to pass a value which is not a string to a method that expects a string as its first argument. |
In order to debug something like this TypeError, the very first thing you need to do is examine what you’re passing to the function and confirm whether it’s of the correct type or not. You can use the built-in typeof operator in JavaScript to check the type of a given object:
console.log(typeof myVariable); // should prints: string
If the type printed isn’t string, then you’ve identified the cause of the error. In such cases, ensure that your variables are receiving the right type of value. When the variables aren’t strings but another type—one could force them into string by invoking their toString() methods before passing them on to the function that requires args of type string.
// when variable is not of string type if (typeof myVariable !== 'string') { myVariable = myVariable.toString(); }
Developing a deep understanding of how JavaScript handles data types can also assist you in preventing these kinds of errors. Remember, knowledge of how to manipulate JavaScript data types properly will significantly enhance your abilities in resolving TypeError issues akin to “The first argument must be one of type string.” As such, mastering JavaScript type conversions is highly recommended for any professional coder to automate and streamline their debugging process.
Always make sure to keep an eye out on those tiny details that might lead to massive hitches within your code because every detail accounts—for instance, ensuring proper data typing will prevent from running into this typical type-related JavaScript error.