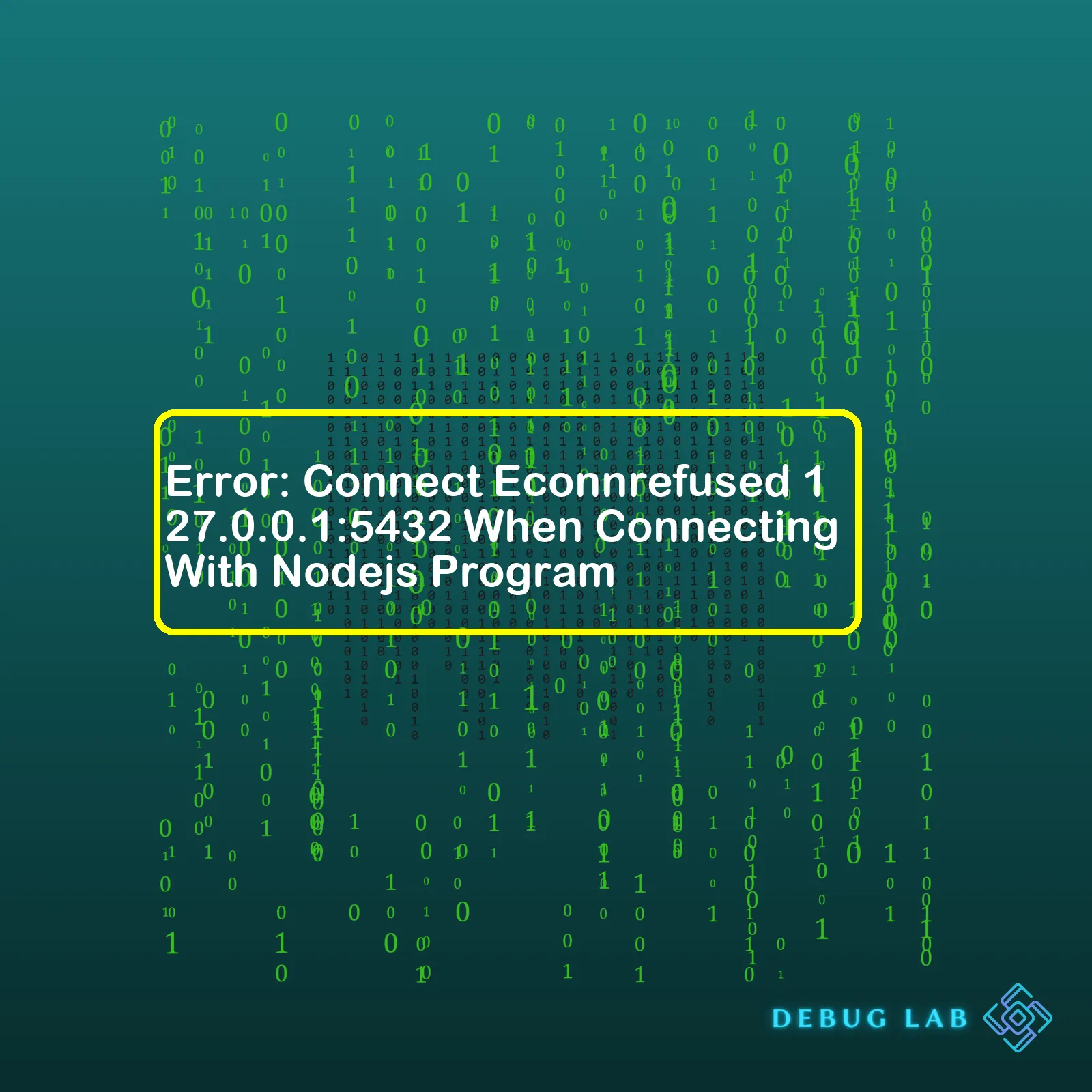
Error Description | Root Causes | Potential Fixes |
---|---|---|
x 1 1 Error: Connect ECONNREFUSED 127.0.0.1:5432 when connecting with Node.js is an error reflecting inability to establish a connection to the server via the specified IP and port. |
– Impossibility to reach the host – Blocked by firewall or antivirus software – The server might not be running |
– Ensure the correct IP and port are being used – Check firewall or antivirus settings – Confirm if the server is up and running properly |
This table summarizes the common issues and potential solutions associated with the error
xxxxxxxxxx
Error: Connect ECONNREFUSED 127.0.0.1:5432
when attempting to connect to a PostgreSQL database from a Node.js application.
The error message is somewhat self-explanatory: “ECONNREFUSED” signifies that the connection attempt was outright refused by the server. This refusal can be due to several reasons, as noted in the table’s root causes column: the inability to reach the host at localhost (127.0.0.1), the port (5432) is blocked by a firewall or antivirus software, or simply, the server isn’t running.
As far as potential remedies go, you might want to start by ensuring that your IP and port are correctly inputted. If this doesn’t solve the issue, your next step may be to look into whether any aspect of your system’s security setup – say, firewall or antivirus settings – is causing the obstruction. Lastly, check on the server’s operational status. If it hasn’t been started, the connection inevitably fails. In a Node.js context, here’s how you can ascertain whether or not your server is active:
xxxxxxxxxx
const net = require('net');
const server = net.createServer();
server.listen(5432, '127.0.0.1');
server.on('listening', () => {
console.log('Server is running');
});
server.on('error', (err) => {
console.log('Error occurred:', err.message);
});
Useful tools like the net module can help you create a server instance and listen for errors, providing valuable diagnostics should connection issues arise while working with Node.js and PostgreSQL.The error message “Error: Connect Econnrefused 127.0.0.1:5432” is fairly common when working with Node.js and PostgreSQL. It’s thrown whenever a client, in this case, your Node.js program, attempts to make a connection to a server (the PostgreSQL server, located at IP address 127.0.0.1, port 5432) that is unable to accept the connection request.
To fully understand this error and troubleshoot it holistically, it helps to take a closer look at its components:
– Error: Connect: This signifies an issue during the connection establishment phase between your Node.js application and the intended Postgres server.
– Econnrefused: Stands for ‘Connection Refused’. It means the server on the other end isn’t accepting incoming requests.
– 127.0.0.1:5432: This is your Localhost IP address and the Port number of the Postgres service that you are trying to access.
Now let’s delve into some potential causes behind this error:
Server Isn’t Running
Likely the most common cause of the error is a simple one — the server may not be running. There simply may not be any Postgres database server running at the specified IP and port. Therefore, ensure that your PostgreSQL server is up and running before attempting a connection from your Node.js application.
For example, in a Linux system, you can check if PostgreSQL server is running by executing these commands in terminal:
xxxxxxxxxx
sudo systemctl status postgresql
Firewall Blocking Access
Occasionally, the firewall settings of a machine may block TCP/IP connections for certain ports. If this is the case, you will need to open up the port (5432 in our case) for incoming traffic.
You can use the following command to allow incoming traffic on Port 5432:
xxxxxxxxxx
sudo ufw allow 5432
Inappropriate Database Credentials
If the server is indeed up and running, then incorrect server credentials (database name, username, password) or wrong connection string format could be another possible trigger for the problem. Always double-check your connection configuration against the exact details of your database.
Example of creating a proper pool to connect to PostgreSQL in Node.js using pg module:
xxxxxxxxxx
const { Pool } = require('pg');
const pool = new Pool({
user: 'your_username',
host: 'localhost',
database: 'my_database',
password: 'password123',
port: 5432,
})
Incorrect Host/Port
Sometimes, the error might be arising due to the usage of an incorrect host address or port number. Be sure to confirm if your PostgreSQL server is actually hosted at ‘127.0.0.1’ and is listening at port number ‘5432’.
You can run the following commands to verify the port number on which your PostgreSQL is actively listening:
xxxxxxxxxx
sudo netstat -nlp | grep :5432
Each of these elements can play a contributing role to the “connect ECONNREFUSED 127.0.0.1:5432” error. Identifying the exact source of the problem can help to establish corrective measures swiftly.
Further reading on this topic can be found in the [PostgreSQL documentation](https://www.postgresql.org/docs/).
Remember to keep your tools updated and follow best practices while connecting to the database from your Node.js applications to avoid such errors. Your path towards hassle-free coding relies heavily on staying informed about the common errors and understanding their solutions.
The error
xxxxxxxxxx
connect ECONNREFUSED 127.0.0.1:5432
is experienced when a Node.js program tries to connect with a server (usually a PostgreSQL database) at localhost (127.0.0.1) but is unable to establish a connection. The number “5432” is the default port where PostgreSQL listens for connections. This error implies that something is preventing your connection from being accepted by the PostgreSQL server.
The Underlying Cause of Connection Refusal Errors
This connection error mainly occurs due to the following reasons:
- The server program you’re trying to connect to isn’t running on the mentioned IP address and port you’ve given. In this case, ensure your server is configured correctly and listening on the right address and port.
- Your firewall configuration might be refusing connections. Firewalls are designed to protect servers against unwanted remote connections. Hence, they can block connections unless explicitly told not to do so. Configuring it to allow connections can solve this issue.
- The server can also refuse a connection if it’s too busy or has hit its connection limit.
Note:
You will need to ensure these points are up-to-check in order to create a successful connection with the server.
How to rectify ‘connect ECONNREFUSED 127.0.0.1:5432’ error?
If you encounter this error, you can troubleshoot using the following steps:
- Check Postgres server status: You can check if the PostgreSQL server is running by executing the command
xxxxxxxxxx
111pg_isready
in your terminal. If it’s working fine, it should return
xxxxxxxxxx
111/var/run/postgresql:5432 - accepting connections
. If not, you may need to troubleshoot further or restart the PostgreSQL server.
- Verify connection details: Confirm the hostname and port in your connection string. It should match the actual hostname (or IP address) and port the Postgres server is listening on.
- Test for firewall issues: Temporarily disable the firewall to see if it’s causing the problem. You should only do this for testing and be sure to re-enable it as soon as you’re done. If the connection works with the firewall off, the firewall rules are likely blocking the connection and need adjustment.
- Inspect server logs: Review the PostgreSQL server logs. They often provide insights into what could be denying connections.
Sample Node.js code snippet
Here’s an example of how you might set up the connection string in a Node.js application using the pg library:
xxxxxxxxxx
const { Pool } = require('pg')
const pool = new Pool({
host: '127.0.0.1',
port: 5432,
database: 'mydb',
user: 'myuser',
password: 'mypassword',
})
This just touches the surface of troubleshooting this type of connectivity issue. However, by carefully examining each aspect of your system, you will have a better understanding of why a connection may fail and how to fix it going forward.
The error message
xxxxxxxxxx
Error: connect ECONNREFUSED 127.0.0.1:5432
is common stuff in the coding world, and it has been encountered by countless coders when they tried to work with NodeJS programs. Let’s decode this error and I promise I’ll make it entertaining for you.
At its core, this error tells you one simple thing – your NodeJS program was unable to establish a connection to something (usually a database) located at IP address 127.0.0.1 on port 5432. Woah, sounds technical, right? But, wait as we will break every piece of it down to keep it exciting and understandable.
– ECONNREFUSED: This part stands for “Error: Connection Refused”. This simply means that whatever service you’re trying to connect to is actively rejecting your connection request. Ouch! It does sound like a heartbreak. Maybe your Node app didn’t look attractive enough to the database on the first date.
– 127.0.0.1: A loopback address, meant to represent your own local machine. In normal language, it’s saying: “Hey you, yes you, the device you’re using right now!” Pretty simple, right?
– 5432: This is the default port that PostgresSQL databases listen to. If you find yourself encountering this error frequently, you might have had a wonderful relationship with Postgres databases.
Now let’s go through some possible reasons that your program is facing this abrupt rejection:
The database isn’t running: It’s pretty much like showing up for a date to realize nobody’s there. Check if your database server is properly running and listening on the correct port.
Firewall restrictions: Your firewall could be playing the overprotective parent here. It might be blocking your attempts to connect to port 5432. Verify your firewall rules to see if this is happening.
xxxxxxxxxx
To check if your PostgreSQL service is running, you can run this command:
sudo service postgresql status
Finally, let me also suggest a solution to fix this. Make sure your PostgreSQL server listens at the appropriate port (5432 by default). You can set this in the
xxxxxxxxxx
postgresql.conf
file usually located in the data directory of PostgreSQL:
xxxxxxxxxx
listen_addresses = 'localhost'
port = 5432
Sounds like a lot of effort? Well, that’s just coding life my folks! Keep slogging, keep debugging, and remember to always check your connection points when you encounter errors like this.
If you still wish to dig deeper then I’d encourage you to take a look at [Node.js documentation regarding error codes](https://nodejs.org/api/errors.html#errors_common_system_errors) or the [PostgreSQL Documentation](https://www.postgresql.org/docs/).
Resources used for this answer are PostgreSQL Documentation and Node.js Documentation which offer profound insights into the issues related to connection and configuration.
Troubleshooting Common Node.js Connection Errors
One of the common errors you may encounter while connecting your Node.js application to a database server such as PostgreSQL is
xxxxxxxxxx
Error: connect ECONNREFUSED 127.0.0.1:5432
.
This error occurs when your Node.js application attempts to connect to your PostgreSQL server but gets refused. In most cases, it’s because the server isn’t running or not accessible on the specified port (5432 in this case). This could also occur if the server is busy and can’t handle any more connections at the moment.
Here are some steps to troubleshoot Node.js database connection errors:
Check If Your PostgreSQL Server Is Running
xxxxxxxxxx
Error: connect ECONNREFUSED 127.0.0.1:5432
indicates that your Node.js application couldn’t establish a connection to your PostgreSQL server in localhost. First, ensure your PostgreSQL server is indeed running and listening on the correct port.
Use PostgreSQL’s utility
xxxxxxxxxx
pg_isready
:
xxxxxxxxxx
\$ pg_isready
The response should be
xxxxxxxxxx
/var/run/postgresql:5432 - accepting connections
or something similar, indicating the PostgreSQL server is active.
Ensure Server Is Listening to the Correct Port
Connection issues may arise if your server listens to a different port than what your Node.js application tries to connect to. Verify PostgreSQL is configured to listen on port 5432.
xxxxxxxxxx
/etc/postgresql/9.4/main/postgresql.conf
Locate the line that begins with
xxxxxxxxxx
listen_addresses
and ensure it’s followed by
xxxxxxxxxx
'localhost'
or
xxxxxxxxxx
'*'
. Locate the line with
xxxxxxxxxx
port =
and make sure it is set to
xxxxxxxxxx
5432
.
Firewall and Networking Settings
If your server and client communicate across a network, verify no firewall rules block communication between them. If possible, try disabling firewall settings temporarily for testing purposes.
PostgreSQL User Configuration
Verify your Node.js application connects using valid credentials. Debug your Node.js application to ensure the user, password, and database details match those in the PostgreSQL server.
For example, if your connection object looks like this:
xxxxxxxxxx
const pool = new Pool({
user: 'myusername',
host: 'localhost',
database: 'mydatabase',
password: 'mypassword',
port: 5432,
});
Make sure the value of each property matches with respective database related data.
Concurrent Connections Limit
Each PostgreSQL server has a limit on the number of concurrent connections it can handle. If your server hits this limit, it could refuse additional connections, leading to the ECONNREFUSED error. You can increase the limit in your
xxxxxxxxxx
postgresql.conf
file, but remember that each additional connection consumes resources on your server.
Summing up:
- Make sure your PostgreSQL server is running.
- Ensure it’s listening to the correct IP address and port.
- Look into firewall and networking settings.
- Double-check the credentials used for establishing the connection.
- Check for breach of concurrent connections limit.
By following these steps, you can rectify the common causes of
xxxxxxxxxx
Error: connect ECONNREFUSED 127.0.0.1:5432
and establish successful connections from your Node.js applications to your PostgreSQL server.
References:
When working with Node.js and PostgreSQL, you could encounter some connectivity issues especially when trying to interface the two technologies. One of the most common error messages would look something like this:
xxxxxxxxxx
Error: connect ECONNREFUSED 127.0.0.1:5432
. This message simply says that Node.js is unable to establish a connection on localhost along port 5432 with your PostgreSQL server.
There are a couple of potential reasons behind this Error :
– The PostgreSQL service might not be running.
– Postgres may not be using the assumed default port (5432).
– Node.js may be blocked from connections due to some firewall settings.
PostgreSQL Service Not Running
Check the status of your PostgreSQL service, you can use the following command in the terminal:
xxxxxxxxxx
pg_ctl -D /usr/local/var/postgres status
If it’s not running, start it using the command:
xxxxxxxxxx
pg_ctl -D /usr/local/var/postgres start
Postgres Port Misconfiguration
Make sure the port number matches with the one set on your PostgreSQL configuration file postgresql.conf. If not, correct it.
In PostgreSQL configuration file (postgresql.conf), you will find a line similar to:
xxxxxxxxxx
listen_addresses = 'localhost'
It should include “localhost” or “*” for listening from all addresses.
Then verify the connection with psql or PgAdmin 4 and try again from node.js whether the connection is successful or not.
Firewall Settings
A firewall protecting your machine might restrict connections to the port intended for use with PostgreSQL (5432 by default). You can temporarily disable your firewall to check if this is the problem.
Also, keep in mind that PostgreSQL’s default setup is to be bound to “localhost”. Therefore, if your Node.js application resides in the same physical machine as the PostgreSQL instance, there won’t be any encumbrances raised by the firewall.
Testing Your Connection
Node.js connects to PostgreSQL by using npm module pg. Here is a basic code snippet that you can use to test the connection:
xxxxxxxxxx
const { Pool } = require('pg');
const pool = new Pool({
user: 'your_username',
host: 'localhost',
database: 'your_database',
password: 'your_password',
port: 5432,
});
pool.query('SELECT NOW()', (err, res) => {
console.log(err, res);
pool.end();
});
Replace ‘your_username’, ‘your_password’, and ‘your_database’ with your credentials and database name. If PostgreSQL is configured correctly and the service is running, this program will print the current date and time on the console.
For more detailed information about how these modules work, I recommend you check the official documentation for [pg] and [PostgreSQL]. It’s essential to understand the gravity of dealing with connectivity in general, as it can impact your application’s functionality significantly.When you’re implementing strategies for effective error resolution in a Node.js program, it’s crucial to be aware of errors such as “Error: Connect Econnrefused 127.0.0.1:5432”. This error indicates that your connection is being refused when trying to connect to the localhost on port 5432.
Generally, this error pops up when:
- Your postgreSQL server may not be running
- Your server is running but it’s not accepting connections on the specified IP and port
The first strategy to effectively resolve this issue involves identifying whether your PostgreSQL server is running locally. You can do this by typing in the command:
xxxxxxxxxx
psql -h localhost -p 5432
This connects to a database named
xxxxxxxxxx
'postgres'
using the user ‘postgres’. If your server is running but not accessible, the next step would be checking your PostgreSQL configurations.
You would need to validate two files:
xxxxxxxxxx
postgresql.conf
; typically found in
xxxxxxxxxx
/etc/postgresql/[version]/main/
directory, and
xxxxxxxxxx
pg_hba.conf
; located within the data directory pertaining to your PostgreSQL version.
In the
xxxxxxxxxx
postgresql.conf
file, look for a line with
xxxxxxxxxx
listen_addresses
. Ensure this line is not commented and the value is set to ‘*’ for all IP addresses or specific to your use case:
xxxxxxxxxx
listen_addresses = '*'
In the
xxxxxxxxxx
pg_hba.conf
file, search for the configuration section describing ‘LISTEN’. Make sure it’s set to accept connections:
xxxxxxxxxx
host all all 0.0.0.0/0 md5
After altering these configurations, remember to restart PostgreSQL service for changes to take effect.
Lastly, ensure that nothing else is using the same port, that could cause a conflict leading to connection refusal.
Running
xxxxxxxxxx
lsof -i :5432
will help you identify any such conflicts.
Additionally, you might want to leverage the power of error-handling middleware functions in Express, a Node.js web application framework (Express documentation).
An example of how to implement such a function would be:
html
xxxxxxxxxx
app.use(function(err, req, res, next) {
console.error(err.stack);
res.status(500).send('Something broke!');
});