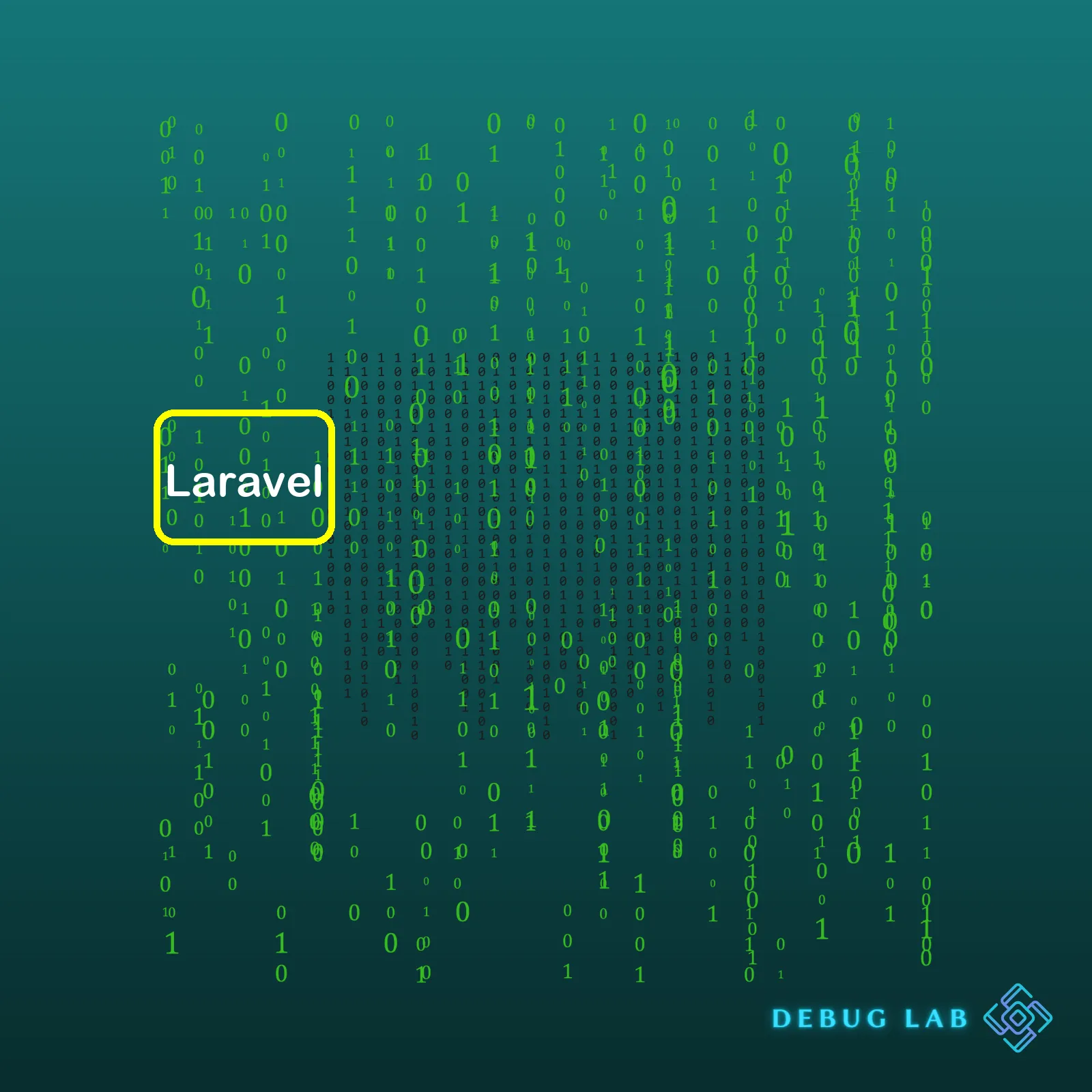
<table>
<thead>
<tr>
<th>S.No</th>
<th>Laravel Version</th>
<th>Release Date</th>
<th>PHP Version</th>
</tr>
</thead>
<tbody>
<tr>
<td>1</td>
<td>Laravel 7.x</td>
<td>3rd March, 2020</td>
<td> PHP >=7.2.5</td>
</tr>
<tr>
<td>2</td>
<td>Laravel 6 LTS</td>
<td>3rd September, 2019</td>
<td>PHP >=7.2.0</td>
</tr>
</tbody>
</table>
This HTML table gives a brief history of the Laravel framework by summarizing its versions and release dates along with the PHP version compatibility. From this summary, it is noted that Laravel 7.x was released on the 3rd March, 2020 and runs on PHP versions greater or equal to 7.2.5. The previous version, Laravel 6 LTS, was released on the 3rd September, 2019, operational on PHP versions starting from 7.2.0.
The Laravel framework is an open-source framework for developing web applications following the MVC (Model-View-Controller) architectural pattern. It offers out-of-the-box solutions to use repetitive coding tasks which simplifies the web development process and reduces development time.
One significant feature of Laravel is that it uses Composer as a dependency manager to manage its extensions, which allows easier updates and addition of extensions. Developers can keep track of all dependencies in their codebase and update these as per their requirements.
With the Laravel framework, developers are able to write cleaner, more readable codes due to its elegant syntax that makes it easier for them to use the framework. It enhances the overall development process by ensuring that the views and logic are properly separated, which in turn enables the developer to modify and maintain code quite conveniently.
Furthermore, Laravel boasts excellent documentation that assists developers in understanding the framework and its components better. This is one of the key reasons why Laravel framework is recommended for both small and large scale projects across the globe.
Additionally, Laravel has a dedicated security package that provides protection against different threats like SQL injection, cross-site scripting etc making Laravel a robust framework for developing secure web applications as well.
Altogether, the features such as high security, scalable mechanisms, easy-to-use tools and highly customizable structure make Laravel a top choice when considering PHP frameworks for web application development.
Sources utilized includes the official Laravel Documentation.Laravel, a delightful open-source PHP framework developed by Taylor Otwell, is widely acclaimed for its beautiful syntax. Laravel has redefined the way developers write PHP code. Something that makes it popular amongst developers and startups is its use of Model-View-Controller (MVC) design pattern[Wikipedia], which separates the application into three interconnected parts.
To fully understand Laravel’s MVC architecture, let’s break down what these components – Models, Views, and Controllers – are:
• Models in Laravel represent the logical structure of your data. They interact with your database tables, retrieving, inserting, and updating information. Importantly, models allow you to encapsulate business rules and data manipulation methods so that they can be reused and maintained separately from the rest of your application logic.
Example of Laravel Model:
namespace App;
use Illuminate\Database\Eloquent\Model;
class Post extends Model
{
// The table associated with the model.
protected $table = 'posts';
}
• Views handle the presentation of your application. Think of them as templates which are populated with information taken from a model and delivered to a client for display. Usually, views consist of HTML, CSS, and embedded PHP (Blade) code.
Example of Laravel View:
xxxxxxxxxx
<!-- view located in resources/views/greeting.blade.php -->
<html>
<body>
<h1>Hello, {{ $name }}</h1>
</body>
</html>
• Controllers, being part of the business layer interact with both the models and the views. Controllers handle user requests, retrieve/process data from models, load the appropriate views, and ultimately return an HTTP response to the client. Essentially, controllers act as an interface between models and views.
Example of Laravel Controller:
xxxxxxxxxx
namespace App\Http\Controllers;
use App\Post;
use Illuminate\Http\Request;
class PostController extends Controller
{
public function show($id)
{
return view('post.show', ['post' => Post::findorFail($id)]);
}
}
In essence, the MVC design pattern helps organize your application’s code, improving maintainability and scalability. It encourages separating business logic (model), data rendering (view), and user actions (controller) into different segments of the application. This separation of concerns leads to modular codebase, clean code practices, effective collaboration between developers, and better long-term project management.
In the context of Laravel’s MVC architecture, tools such as Eloquent ORM(Official Documentation) for simplified database operations on Model, Blade Templating Engine(Official Documentation) for easy creation and extension of views, and Resource Controllers(Official Documentation) for efficient route-to-controller logic mapping further enriched the productivity.
So, there you have it! You can well imagine how adopting Laravel’s MVC architecture will drive the developer’s experience towards writing more expressive, readable, and less redundant PHP code while keeping the essentials intact.
Okay, let’s dive into Laravel routing, arguably one of the main reasons why Laravel is so widely accepted in the PHP community. Laravel offers an explicit and intuitive API over the URL scheme of your application.
In a fresh Laravel installation, a `routes` directory containing various files where you can define all of the route definitions for your application is included. Out of these,
xxxxxxxxxx
web.php
is primarily where we’ll focus on for this article.
So, what exactly are routes?
Routes lay down the path between your users’ request and the logic that responds to their needs; basically which URL links to what part of your code.
Say, for instance, you want your application to respond to a user accessing the URL www.yourwebsite.com/about by displaying “About Us”. A simple way to do this would be:
xxxxxxxxxx
Route::get('/about', function () {
return 'About Us';
});
In the simplest form, there are two parts to this:
1. A HTTP Verb: This defines how one interacts with the resource, most commonly used methods being GET, POST, PATCH/PUT, DELETE. In our example, we used get.
2. Route URI: This will be appended to our website’s base URL.
Now, while inline closure based routes are fine for very small apps and prototyping, when building larger applications we generally don’t want to have the entire application logic inside route closures.
Let’s consider using Controllers which are efficient way of linking a route to a class method. According to MVC (Model-View-Controller) architecture, Controllers hold the business logic of your application hence making them ideal to handle incoming requests. Let’s modify the previous /about route:
xxxxxxxxxx
Route::get('/about', 'App\Http\Controllers\AboutController@index');
The string after the @ symbol corresponds to the method on the given controller that’ll be executed when the route is accessed. So, if you visit www.yourwebsite.com/about, the index() method of AboutController class will be invoked.
Remember, Laravel also allows other types of routing methods such as post(), put(), patch(), delete(), options() along with any() and match(). You can further have more than one route pointing to the same controller or even a single method depending on your needs.
Finally, I’d like to discuss a powerful feature; Route Parameters. Web applications typically use dynamic URLs to output uniquely generated pages. For example, you might need to get a specific user by their identifier. See the below code:
xxxxxxxxxx
Route::get('/user/{id}', 'App\Http\Controllers\UserController@show');
Here the {id} part is a variable parameter means it could be anything; 1, 15, cart, john. Whatever this variable gets hold of, will be passed as argument to our controller’s method.
All said and done, this only scratches the surface of the flexible and efficient routing system of Laravel. Topics such as named routes, route groups & middleware, sub-domain routing among others form part of the deeper world of Laravel Routing. For more detailed information about Laravel Routing, please refer to the official Laravel documentation here.
Laravel is an outstanding PHP framework that offers a simple, yet robust way of developing web applications. One of its defining characteristics is its built-in authentication system, designed with security in mind. In this brief write-up, we will delve into how Laravel developers can leverage the framework’s authentication features to enhance the security of their applications.
To begin with, Laravel provides out-of-the-box support for user registration, login, and password resets – what it refers to as its Authentication scaffolding. This means you don’t have to write these features from scratch, and instead, you get them just by running a command, namely:
xxxxxxxxxx
php artisan make:auth
Once the above command is run within your Laravel project directory, certain routes related to authentication are automatically generated. Furthermore, the views for login and registration pages are likewise produced, thus saving you valuable coding hours.
Other than simplifying the development process, Laravel also prioritizes data protection via hashed passwords. It utilizes the Bcrypt hashing algorithm, a secure method of storing passwords in your database. Here is an example of how you might use Laravel’s built-in Bcrypt function to generate a hashed password in one of your controller classes:
xxxxxxxxxx
$user = new User;
$user->password = Hash::make($request->input('password'));
$user->save();
In the code snippet above, the user’s password input is being hashed using Laravel’s Hash facade, which ensures that even if someone were to gain unauthorized access to your database, they wouldn’t be able to see your users’ plain text passwords.
Another effective way Laravel helps protect your application is through its built-in solution for protecting against Cross-Site Request Forgery (CSRF) attacks. A CSRF Token makes sure that it is actually your website sending requests to your server and not some hacker trying to take advantage of your user’s session.
Below represents csrf field added in form :
xxxxxxxxxx
@csrf
Application-level security is only as strong as you make it. Thus, employing Laravel’s built-in tools for authentication and protection against threats like CSRF attacks aids in enhancing the security of your application. As a professional coder, I’d highly recommend leveraging Laravel’s powerful, out-of-the-box security features to safeguard your data and uphold your system’s integrity.
To decode the Eloquent Object-Relational Mapper (ORM) in Laravel, we need to understand that it’s an implementation of the Active Record pattern which provides an easier way to interact with your database. The central principle is that the database table correlates to an Eloquent model, allowing interaction with your tables by invoking corresponding methods on instances of your models.
An everyday example of using Eloquent ORM in Laravel can be seen when running a basic query for selecting all records from a table:
xxxxxxxxxx
$users = App\Models\User::all();
foreach ($users as $user){
echo $user->name;
}
In this code snippet,
xxxxxxxxxx
App\Models\User::all()
retrieves all users from the database into an array of User objects and then prints out each user’s name.
Moreover, Eloquent provides a broad range of capabilities to perform operations such as:
1. **Inserting & Updating Models** – To create a new record in the database, simply create a new model instance, specify attributes and call the save method.
xxxxxxxxxx
$user = new App\Models\User;
$user->name = 'John Doe';
$user->email = 'john@example.com';
$user->save();
2. **Deleting Models & Soft Deleting** – Deleting a model is as simple as calling delete() on the instance. Laravel also supports “soft deletes,” enabling retrieval and restoration of deleted models.
3. **Query Scopes** – Scopes allow for encapsulation of query constraints into manageable, reusable methods, notably including the local scope and global scope.
4. **Events** – Events provide a way to hook into various parts of Eloquent’s lifecycle with performant event listeners.
Eloquent also supports relationships used in relational databases like one-to-one, one-to-many, many-to-many, polymorphic relations (source). These enable clean coding without messing up with direct SQL queries.
In the end, Laravel’s Eloquent ORM combines convenience with functionality and ease of syntax to make working with databases seamless, capturing the essence of Laravel’s philosophy of making development tasks enjoyable for the developer without sacrificing functionality.
For more depth understanding, take a look at the Laravel documentation on Eloquent ORM (source). Eventually, you will find that Eloquent ORM is an extremely influential piece of the Laravel framework, offering powerful features that can significantly speed up the process of building sophisticated applications.
Laravel, the comprehensive, expressive, and elegant web application framework crafted for web artisans, comes inherently equipped with several features to ease your software development journey – one of such is middleware. Before we delve into Laravel middleware and requests, let’s understand what middleware in a software application truly means.
Middleware acts as a series of “checkpoints” or “filtering mechanism” in software applications, particularly in web application frameworks like Laravel. These could be depicted as intermediaries that stand between client request and server response, tweaking them for varied purposes such as user authentication, logging, caching, and so on.
Laravel Middleware
In Laravel, Middleware operates as a bridge connecting a request and a response. Its core job consists of analyzing, transforming, and filtering HTTP requests entering your application. For instance, Laravel includes middleware for maintenance mode, validation of X-CSRF-TOKEN, sanitization of inputs, etc. To create a new middleware, you would use Laravel’s artisan command line tool:
xxxxxxxxxx
php artisan make:middleware TestMiddleware
This creates a new file in
xxxxxxxxxx
app/Http/Middleware/TestMiddleware.php
, where you can define logic that runs before your application handles the incoming HTTP request.
Handling Requests With Middleware
Middlewares are stacked upon each other in a way that form a layer around the app route handling mechanism. Whenever an HTTP request enters the application, it has to pass through each of these middleware layers before finally reaching the location it was intended to.
To apply a middleware to every request handled by your router, use the global array variable in
xxxxxxxxxx
app/Http/Kernel.php
:
xxxxxxxxxx
protected $middleware = [
\App\Http\Middleware\TestMiddleware::class,
];
Now the TestMiddleware will run on every HTTP Request made to your application. If required, you can also assign middleware to specific routes.
xxxxxxxxxx
Route::get('/admin', function () {
//
})->middleware('TestMiddleware');
It’s just like putting a security guard (middleware) on gate for checking (processing) everyone (request) who wishes to go inside a building (route).
Dealing with Middleware parameters
The beauty of Laravel’s middleware architecture is it gives developers the freedom to pass parameters. Instead of only dealing with HTTP requests and responses, your middlewares can now interact with custom parameters defined by you. Below is a snippet of how you may implement this:
xxxxxxxxxx
public function handle($request, Closure $next, $role)
{
if (! $request->user()->hasRole($role)) {
// Redirect...
}
return $next($request);
}
You’re simply feeding 3rd Parameter (
xxxxxxxxxx
$role
) to the middleware. You can call it on the route like:
xxxxxxxxxx
Route::put('/post/{id}', function ($id) {
//
})->middleware('role:editor');
Middlewares & Request Life-Cycle in Laravel
Once the Laravel app receives a request (HTTP), the process begins with unserialization of the URL and ends with sending back the HTTP response. In this whole journey, the part of request reception and response dispatch is surrounded by various Middleware.
Stage | Description |
---|---|
Pre-Processing Stage | When request first hits, the Middleware you’ve placed globally handles it. Any general ruling applied gets checked here. |
Routing Stage | If your request specifies a route-based Middleware, it’s tackled in this stage. |
Post-Processing Stage | After your app has catered to the request by generating a response, all Middleware specified within terminable middleware get their chance to work on the response before sending it back. |
By fully understanding and effectively utilizing Laravel’s middleware and its request life-cycle handling capabilities, developers can deliver highly scalable, customizable, maintainable, and full-fledged web applications. Despite its simplicity, this pattern allows for powerful modifications and extensions, which makes Laravel a highly flexible and extensible PHP web application framework.
For more learning refer to Laravel Middleware documentation.
Queues in Laravel are used to defer the processing of time-consuming tasks, such as sending an email, until a later time which significantly speeds up web requests to your application. The queue configuration file is stored in
xxxxxxxxxx
config/queue.php
, where you can find connection configurations for several queue drivers.
Laravel Queues
Laravel Queue system offers a unified API across multiple different queue backend systems like Beanstalk, Amazon SQS, Redis, or even a relational database.
Create Job:
To generate a new job, you may use the
xxxxxxxxxx
make:job
Artisan command:
xxxxxxxxxx
php artisan make:job ProcessPodcast
Dispatch the Job:
Once you have written your job, you may dispatch it using the
xxxxxxxxxx
dispatch
function:
xxxxxxxxxx
$podcast = App\Podcast::find(1);
ProcessPodcast::dispatch($podcast);
Laravel Jobs
A job in Laravel is the unit of data that you’re pushing into the queue. This is usually done via a simple PHP class which implements Laravel’s job contract.
Create and Execute Job Inline:
You can also create jobs on the fly:
xxxxxxxxxx
use App\Jobs\ProcessPodcast;
use Illuminate\Support\Facades\Queue;
$data = ['string' => 'Hello'];
Queue::push(new ProcessPodcast($data));
Laravel Schedules
Laravel has a powerful task scheduling mechanism, which allows your application to accomplish tasks at specified intervals. Some common tasks includes executing console commands, dispatching Laravel jobs, or running a piece of arbitrary code.
To schedule tasks, you need to write them in the method
xxxxxxxxxx
schedule
of the class
xxxxxxxxxx
App\Console\Kernel
.
Schedule a Command:
xxxxxxxxxx
$schedule->command('inspire')->hourly();
Laravel handles the heavy lifting when dealing with queues, jobs & schedules. Providing developers with powerful tools to help manage things that might otherwise slow down their applications. The extensive documentation available at Laravel site provides clear guidance on how to set up and use these features.
Laravel’s Artisan CLI (Command-line interface) is a vital asset to developers. This tool offers a range of beneficial effects, from backend system automation tasks to driving the framework’s behavior.
One primary functionality of Artisan CLI with Laravel includes generating boilerplate code for new controllers, migrations, and models. It can also be useful in database seeding and scaffolding. To do this, you’ll use the ‘make’ command.
xxxxxxxxxx
php artisan make:controller UserController
Through the above command, we are creating a new controller named UserController.
Another key feature of Artisan CLI is handling database migrations. Migrations, they’re like version control for your database, allowing you to modify your database structure.
To create a new migration, you can use the ‘make:migration’ command:
xxxxxxxxxx
php artisan make:migration create_users_table --create=users
The ‘–create’ option indicates that the migration will be creating a new table, and ‘users’ is the name of the table.
After creating migrations, you can apply them using the ‘migrate’ command:
xxxxxxxxxx
php artisan migrate
Caching configuration plays a crucial role in performance boosting, as well. The ‘config:cache’ command provides this benefit by compiling all configuration files into a single file, reducing computational overhead during runtime.
xxxxxxxxxx
php artisan config:cache
A few other noteworthy functionalities include tinker mode, environment management, and task scheduling.
– **Tinker Mode**: A REPL (Read-Eval-Print Loop) utility provided with Laravel allows you to interactively try out Laravel’s features or check how certain operations work in the Laravel environment.
xxxxxxxxxx
php artisan tinker
– **Environment Management**: Artisan CLI helps manage different environments for your application like ‘testing’, ‘staging’, or ‘production’. By merely updating the ‘.env’ file, users can switch between environments.
– **Task Scheduling**: Laravel’s task scheduler abstracts away the complexities of setting up task scheduling via CRON jobs, making it easy to schedule tasks right within the Laravel application.
xxxxxxxxxx
php artisan schedule:run
Artisan CLI makes Laravel simpler and more user-friendly. Code generation, database migrations, configuration caching – all these functions mean less manual labor for developers. Remember, for any question on a specific command, just type ‘php artisan help [command_name]’. For a general list of commands, use ‘php artisan list’.
Learn more about Artisan CLI and its functionalities on the official Laravel documentation page: Laravel Artisan CLI.
Accordingly, learning to use Artisan CLI efficiently might turn crucial while working with Laravel. Leveraging these features wisely can lead to rapid and astounding improvements in your Laravel development experiences.Laravel, being a web application framework with expressive, elegant syntax, has evolved as an integral tool in today’s programming world. By simplifying tasks that are commonly used in developing major web projects, such as routing, sessions, caching, and authentication, it naturally provides developers a breath of relief as well as the productivity boost they need.
One notable feature Laravel excels at is its beautiful, simple ActiveRecord implementation for working with your database – Eloquent. The Eloquent ORM included with Laravel, enables a wonderfully simple ActiveRecord implementation for working with your database, including quick pagination tools. This involves writing straightforward PHP to interact with your database, yet another point Laravel scored for developer-friendliness.
xxxxxxxxxx
class User extends Eloquent {
protected $table = 'users';
public function phone()
{
return $this->hasOne('Phone');
}
}
Here, the method name ‘phone’ in the User model class reveals the model naivety. Now you can access Phone records from User model objects:
xxxxxxxxxx
$phone = User::find(1)->phone;
Additionally, Laravel comes with a powerful template system called Blade, which is driven by template inheritance and sections. See an example below creating a view file in Laravel:
xxxxxxxxxx
// View placed at resources/views/greeting.blade.php
Hello, {{ $name }}
Regarded highly for its clean architecture, Laravel provides developers a way to use a simple syntax structure; while making a relatively small footprint on resources, thus increasing efficiencies.
Further to this, Laravel has a unique architecture, where it possibly holds all related logic within one folder (called MVC). This helps Laravel provide better performance, features, and scalability.
Moreover, extensive support from the community corresponds to an easy-to-understand, always-available pool of knowledge, consisting of variously detailed tutorials and blog posts, promoting swift learning and problem resolution for novice to seasoned developers.
For more details, refer to the official Laravel documentation.
The modern PHP-based Laravel, loaded with prime and above-explained traits like simplicity, elegance, conciseness, flexibility, readability, and maintainability, often becomes an imperative asset, that significantly facilitates the complex processes involved in the effective web development life-cycle.