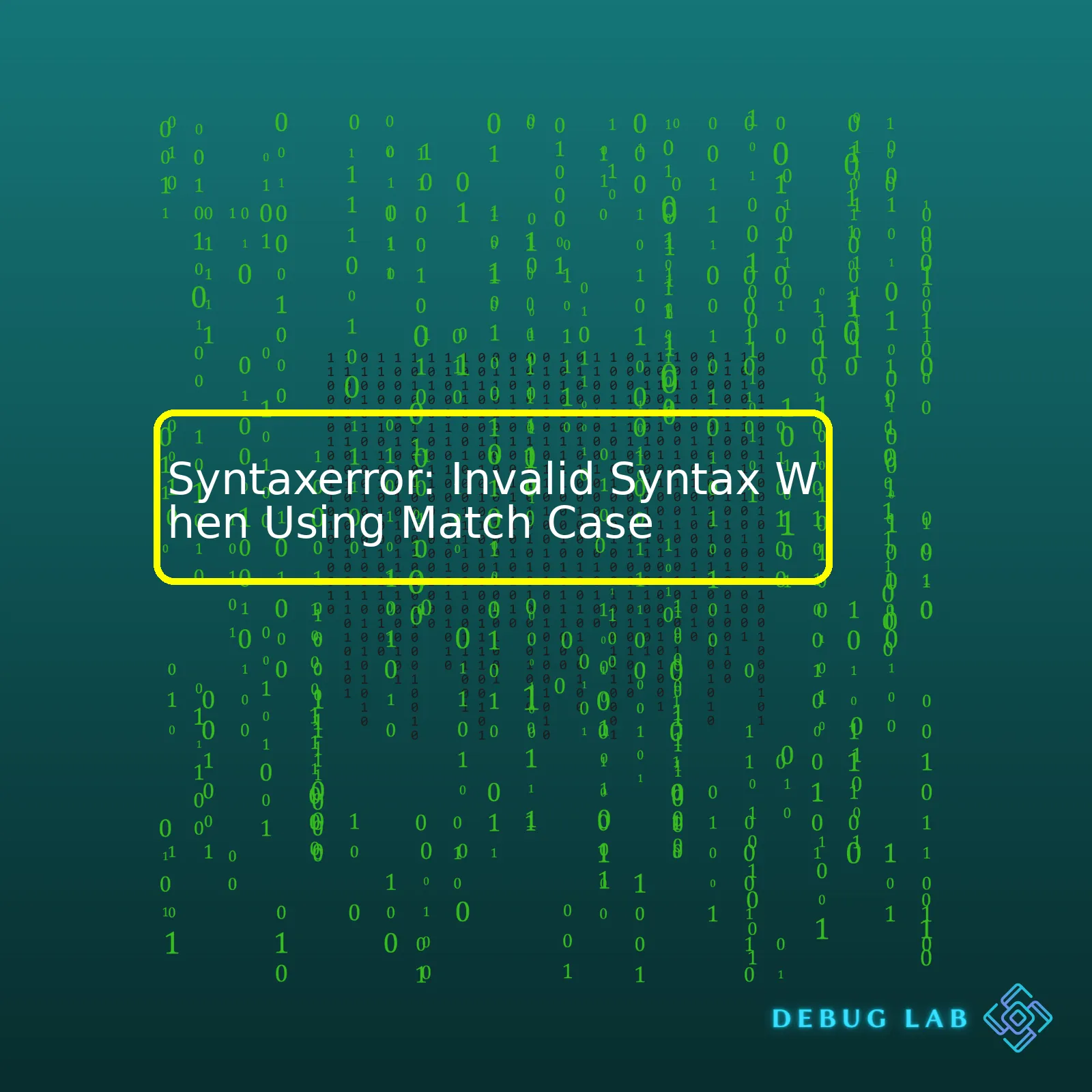
When you come across the “SyntaxError: Invalid syntax” while using match case
on Python, it is usually due to an incorrect or unrecognized grammar in your code.
Python has a unique way of writing its language, and slight deviations from that
can lead to this error. Prior to Python 3.10, match case was not supported, which could be another source of the problem.
Error Type | Common Cause | Solution |
---|---|---|
SyntaxError: Invalid Syntax | Unrecognized Python Code Syntax | Review Code for Errors |
SyntaxError: Invalid Syntax | Use of Match Case Statement in Python Version Older Than 3.10 | Update Python Environment |
To remedy this issue, the first step to take is to properly review your code to identify any place where this could have gone wrong. Are there functioning calls omitted? Or perhaps some indentation mistakes?
#Incorrect
match x:
case [1]:
print("Matched!")
#Correct
def check_match(x):
match x:
case [1]:
print("Matched!")
Also, if you are working with Python version older than 3.10 and trying to implement match-case statements, you’ll certainly face some form of ‘invalid syntax’ error since match case became part of Python syntax as of the 3.10 release. Make sure to upgrade your Python interpreter via the official Python website here.
Remember, keeping your coding environment updated is critical not only to prevent such errors but also to make use of the latest and greatest enhancements and optimizations available in modern releases of the language. Happy Coding!
When seeing the error ‘SyntaxError: Invalid syntax’ when using the match case in Python, it’s important to look closely at where and how you’re deploying this feature in your script.
Here we must understand that the match-case statement is introduced in Python 3.10. Therefore, you are likely to face the SyntaxError if you’re using an older version of Python that doesn’t support match-case statements.
So, let’s delve into what might cause such an error:
- Incompatibility Issue: The first thing one should check is the compatibility. The match-case command was introduced in Python 3.10. So if you’re using any version prior to 3.10, then the code will throw a syntax error.
xxxxxxxxxx
# A sample match-case implementation in python 3.10
def http_response(status):
match status:
case 200:
return "OK"
case 404:
return "Not found"
_:
return "Unknown status"
# This code will throw a syntax error in versions below 3.10
To get the python version, use the following code:
xxxxxxxxxx
import sys
print(sys.version)
# Sample output might be '3.8.5 (default, Jan 27 2021, 15:41:15)'
– If the version shown is older than 3.10, consider upgrading your Python package to run match-case statements.
- Coding Mistake: Python is a language that pays special attention to indentation and the correct use of punctuation like colons (:). Any neglect in these areas can cause a Syntax Error. For instance, forgetting to end a case statement with a colon :
For example:
xxxxxxxxxx
# This block of code will lead to a syntax error:
def http_response(status):
match status:
case 200
return "OK"
case 404
return "Not found"
_:
return "Unknown status"
Here, notice how the case statement has been ended without a ‘:’. This minor neglect can lead to the SyntaxError.
The correct form should be:
xxxxxxxxxx
def http_response(status):
match status:
case 200:
return "OK"
case 404:
return "Not found"
_:
return "Unknown status"
Compare the two blocks of code closely; you’ll see a difference in how colons have been applied at the end lines after the case statement. Thus, coding mistakes may also lead us to a ‘SyntaxError: Invalid syntax’ when using the match case.
By understanding the reasons and methods mentioned above, you can address the issue quickly. As a professional coder, decoding errors is all about catching clues given by the compiler and knowing the updates in the language. For more details, refer to the Python 3.10 official documentation.
The introduction of Python 3.10 brought with it the eagerly anticipated `match` statement, also known as Python’s version of a switch-case construct. However, with novel functionality comes the possibility for slip-ups and consequently, SyntaxErrors being thrown up by your code. In this segment, we will concentrate on identifying some common mistakes developers run into when implementing match-case statements, and how can these be avoided.
Let’s begin with defining a basic match-case using an example:
xxxxxxxxxx
animal = "Dog"
match animal:
case "Cat":
print("You have a cat.")
case "Dog":
print("You have a dog.")
case _:
print("Unknown animal.")
This block of code works well and is error-free, thanks to its simplicity. But once, we start dealing with complex constructs, that’s when developers tend to make mistakes, both syntactical and logical.
Common Pitfalls
1. Missing Colon “:”: If you forget to apply a colon right after a value in the case clause, you will get a “SyntaxError: invalid syntax”. So always remember to add a colon after each case value.
For instance, instead of writing:
xxxxxxxxxx
case "Dog"
print("You have a dog.")
Make sure to use:
xxxxxxxxxx
case "Dog":
print("You have a dog.")
2. Indentation Errors: Python heavily relies on indentation for reading blocks of code. Remember to correctly indent your code snippets under each case clause, or else a SyntaxError may be raised due to invalid identation. This holds true even in match-case statements.
For instance, rather than writing:
xxxxxxxxxx
case "Dog":
print("You have a dog.")
You should write:
xxxxxxxxxx
case "Dog":
print("You have a dog.")
3. Case Clause not Exhaustive: Another common issue arises if no case conditions are met, and no catch-all (designated by an underscore “_”) condition has been defined. The error might not be reported at runtime until the input doesn’t match any case, but it is indeed a pitfall to be aware of.
Therefore, instead of leaving out a ‘catch-all’ case:
xxxxxxxxxx
match animal:
case "Cat":
print("You have a cat.")
case "Dog":
print("You have a dog.")
Always make sure to include one:
xxxxxxxxxx
match animal:
case "Cat":
print("You have a cat.")
case "Dog":
print("You have a dog.")
case _:
print("Unknown animal.")
As with most coding practices, getting familiarized with match-case syntax and avoiding these common pitfalls takes practice. Make sure you adhere to correct Python syntax and handle edge cases effectively when working with match-case statements. By utilizing the newly introduced pattern matching capabilities in Python efficiently, you can significantly simplify your code, enhance readability and improve performance.
References: Python PEP 636 — Structural Pattern Matching Tutorial
When you’re debugging
xxxxxxxxxx
SyntaxError
problems with match cases in Python, it’s essential to follow some strategies to iron out the bugs effectively. The most common error message that is commonly encountered is ‘
xxxxxxxxxx
SyntaxError: Invalid syntax
‘ when using the
xxxxxxxxxx
match case
statement.
In Python 3.10 and newer versions, structural pattern matching was introduced with the
xxxxxxxxxx
match
statement which follows the semantics of switch-case constructs found in other high-level languages.
The SyntaxError could occur due to numerous reasons, such as:
- An invalid pattern after
xxxxxxxxxx
111case
.
- Inappropriate indentation or white spaces.
- A missing colon
xxxxxxxxxx
111:
after a case-label.
- Using the
xxxxxxxxxx
111match-case
construct in Python versions lower than 3.10.
To debug these issues, consider implementing the following strategies:
1. Check Python Version
The
xxxxxxxxxx
match case
statement is only applicable in Python 3.10 and later versions. Using this feature in prior versions will raise a
xxxxxxxxxx
SyntaxError
. Always verify the version of your Python interpreter by running
xxxxxxxxxx
python --version
in your terminal or cmd.(source)
2. Debugging using print() statement
You can temporarily integrate
xxxxxxxxxx
print()
statements to visualize the patterns being matched. (source)
3. Refer to the Line Number in Error Message
The error message gives you the specific line at which the error has occurred. Use this information to investigate issues with indentation, missing colons, etc.
4. Use match-case in an Appropriate Context
Ensure that you are employing the
xxxxxxxxxx
match case
statement within its intended scope. For instance, trying to use match case on non-literal cases would yield a SyntaxError.
For example, although match case supports various patterns like sequence patterns, mapping patterns, class patterns, it doesn’t support regular expressions or lambda function.
5. Consult Documentation
The Python documentation(source_docs) provides substantial detail about how to correctly utilize the match-case statement.
6. Utilize Python IDEs or Code Editors’ Built-in Debugging Tools
Integrated Development Environments (IDEs) like PyCharm and code editors like VS Code offer built-in debugging tools for Python. They highlight the problematic parts of your code, easing the process of debugging.(source)
Applying these strategies should help us resolve most of the common
xxxxxxxxxx
SyntaxError: Invalid syntax
errors encountered when using a
xxxxxxxxxx
match case
. Remember to always check your syntax thoroughly and upgrade to the appropriate Python version if needed.
The advent of Python 3.10 introduced a new principle to the Python world, the match-case pattern matching syntax. Unfortunately, many seem to encounter `SyntaxError: invalid syntax` upon using it. When you see this error message, it’s bewildering, because your code seems to follow the appropriate syntax for the design feature.
However, it can be boiled down to a simple explanation – The reason you’re encountering this error is likely that the Python version you’re employing doesn’t support the match-case control method as the feature was only introduced in Python 3.10.
Ensure You’re Using Python 3.10 or Higher
First off the bat, ensure you are working with a Python version that supports the structured pattern matching mechanism. This will save you from SyntaxError-related headaches. Confirm your Python version with the following command line input:
xxxxxxxxxx
python --version
Now, if your Python version is 3.10 or above, let’s consider some best practices to employ while using the match case keyword in your programming:
Structuring Your Match Patterns
The effectiveness of match case lies in its pattern structure (also known as patterns), which you can use to match different cases. Patterns come in a variety of forms but the primary way would be:
xxxxxxxxxx
match variable:
case pattern1:
...
case pattern2:
...
Patterns can be literals, sequences, mappings, class instances, among others. Optimal utilization of these varieties will bring more value to your code.
Undertake Precise Matching by Including Additional Conditions
This makes Match Case an advanced switch statement. Certain types of pattern matching only allow you to check if value equals to specific constant values but with match case, you can include certain conditions against each pattern for more precise matching.
xxxxxxxxxx
match object_expression:
case Classname(x) if x.condition == 'value':
print('Condition met!')
case _:
print('No match')
Wildcard Pattern (_) for The Default Case
Python Documentation suggests the use of `_` in match case pattern acts as a `default case`. Meaning, if no pattern matched, the statements inside case `_` block will be executed. It’s akin to the `else` clause in most conditional structures.
xxxxxxxxxx
match number:
case 0:
print("Zero")
case _:
print("Not zero")
Safeguarding Your Code Against Malformed Data
To protect your code, include fallback cases that handle unexpected data. These robust checks prevent your applications from breaking when they receive inputs that do not conform to their expected formats. For example, we could implement error handling mechanisms like so:
xxxxxxxxxx
match expression:
case Expectation(args):
case _:
You cannot undermine the importance of debugging and validating your code. Attack those bugs before they lay eggs! With these helpful tips and practices in mind, you should be able to effectively use the match case structures and eliminate invalid syntax errors.
When programming, syntax errors like
xxxxxxxxxx
SyntaxError: invalid syntax
are common issues we encounter. Such an error occurs when Python’s parser can’t understand a line of code. An interesting scenario arises when using the newly incorporated
xxxxxxxxxx
'match case'
construct in Python 3.10 which may spawn error messages similar to this if attempted on older versions.
The Impact of Syntax Errors
Syntax errors, including
xxxxxxxxxx
SyntaxError: Invalid Syntax
, have several impacts on programming:
- Break in code execution: When a syntax error arises in a script, it halts the program. Further lines of code aren’t executed causing disruption in functionality.
- Time consumption: Identifying and resolving syntax errors takes time, particularly in larger projects. This diverts programmers from building or enhancing the necessary functionalities.
- Impaired user experience: If not properly handled, syntax errors can cause applications to break abruptly, leading to a poor user experience.
- Misinterpretation of Code: In some cases, syntax errors might cause certain parts of the code to function unexpectedly, resulting in incorrect program output.
Understanding The match-case Construct
Introduced in Python 3.10, the
xxxxxxxxxx
match-case
statement is a switch-like construct that allows for better matching and pattern checking. It offers more expressive matching syntax compared to traditional if-elif-else blocks.
Here is an example of what you can do with a match-case statement:
xxxxxxxxxx
def case_example(x):
match x:
case 0:
print("Zero")
case 1:
print("One")
case _:
print("Unknown number")
In older Python versions, attempting to implement this feature will lead to a
xxxxxxxxxx
SyntaxError: invalid syntax
. This is because Python 3.9 and below don’t recognize the
xxxxxxxxxx
match-case
syntax.
A hypothetical attempt to run such code could look like this:
xxxxxxxxxx
def case_example(x):
match x:
case 0:
return "Zero"
case 1:
return "One"
case _:
return "Unknown number"
print(case_example(2)) # Outputs SyntaxError: invalid syntax
To avoid these syntax errors and effectively use the
xxxxxxxxxx
match case
construct, ensure you’re running Python 3.10 or later. Simply check your python version by executing the command
xxxxxxxxxx
python --version
in your terminal.
Remember that syntax forms the basis of any programming language and correct implementation ensures seamless code execution and an optimum coding experience. Understanding the basics of syntax rules as well as updated constructs like
xxxxxxxxxx
match-case
in newer Python versions can help evade errors such as
xxxxxxxxxx
SyntaxError: invalid syntax
.
For a detailed understanding of Python match-case construct, you can refer to PEP 634 – Structural Pattern Matching.
The Match Case statement is a powerful feature introduced in Python 3.10 that extends the capabilities of conditional statements. However, one frequent error developers encounter when using Match Case is ‘SyntaxError: Invalid Syntax.’ This error presents itself due to a range of reasons from incorrect Python version to syntactical errors.
Understanding the Match Case Statement
Before delving into the specific error, it is essential to look at the structure of the match case statement:
xxxxxxxxxx
match expression_to_match:
case pattern_1:
action_1
case pattern_2:
action_2
...
case pattern_n:
action_n
Here,
xxxxxxxxxx
expression_to_match
is what you want to match against, each
xxxxxxxxxx
pattern_i
is what you’ll match against, and each
xxxxxxxxxx
action_i
specifies what happens when a match occurs. Your patterns can be as simple as literal values or complex like sequence matching depending on your use case.
For example,
xxxxxxxxxx
def get_fruit_color(fruit):
match fruit.lower():
case "apple":
return "red"
case "banana":
return "yellow"
case _:
return "unknown"
In this code snippet, we have a function which takes a fruit name as an argument and returns the color of the fruit. If the fruit isn’t an apple or banana, it defaults to returning ‘unknown’.
Common Reasons for SyntaxError: Invalid Syntax When Using Match-Case:
Knowing the correct structure and utilization of the Match case command, let’s delve into potential reasons why you may see the ‘SyntaxError: Invalid Syntax’ error:
Using an Older Python Version
Python 3.10 introduced the structural pattern matching syntax. If you attempt to use Match Case in Python 3.9 or older, you’ll encounter a syntax error.
To upgrade Python, you might find this helpful guide on Python’s official site.
Incorrect Pattern Definition
One of the crucial aspects of a Match Case statement is correctly specifying the pattern. Any errors or oversights in the design of the pattern can trigger a SyntaxError.
Misuse of Reserved Keywords
Moreover, be cautious while naming variables and other identifiers because using reserved keywords incorrectly can cause syntax errors.
Here’s an erroneous example;
xxxxxxxxxx
match x:
case True when _:
print("x is true")
But the correct form is:
xxxxxxxxxx
match x:
case True:
print("x is true")
Where
xxxxxxxxxx
_
stands for don’t care value for structural pattern matching and shouldn’t succeed
xxxxxxxxxx
when
.
Conclusion:
Whenever encountering the ‘SyntaxError: Invalid Syntax’ error with Match Case statement, dig into these common issues discussed here. By doing so, you’re more likely to resolve it.Certainly, troubleshooting an invalid syntax error when using
xxxxxxxxxx
Match Case
in Python can be a bit challenging. Lucky for you, I have handy tips to resolve this issue effectively.
First off, it is essential to understand the context of this syntaxerror problem. The `match case` statement, also termed as a Structural Pattern Matching (SPM), is a recent addition to Python 3.10 and beyond. If you encounter an invalid syntax error while using
xxxxxxxxxx
Match Case
, it could happen because your Python interpreter version doesn’t support this feature.
Here’s how to do it:
1. Check Your Python Interpreter Version:
You need to first ensure that Python 3.10 or above is installed on your workstation. To verify your Python version, run the below command in your terminal:
xxxxxxxxxx
python --version
If the version displayed is lower than 3.10, considering updating your Python Interpreter to the latest one would be an intelligent step.
2. Ensure Correct Syntax:
In Python, even the minutest syntax error can cause significant conflicts. The correct syntax format for utilizing the match-case control flow structure is:
xxxxxxxxxx
match expression:
case pattern1:
action1
case pattern2:
action2
...
Remember, Python’s match case is similar to switch statements found in other programming languages but offers more complex and expressive patterns.
3. Safeguard against Syntax Errors:
Syntax errors usually arise from not closing parentheses or not following indentation properly. It’s advisable to use the Integrated development environment (IDE) that points out these errors to safeguard against such issues. Also, don’t forget to pay attention to the details predicted in the error message, which may assist in spotting the ill-structured code.
Take an example:
xxxxxxxxxx
name = 'Alice'
match name:
case 'Bob':
print("I found Bob!")
case 'Charlie':
print("Found Charlie.")
case _:
print("This is someone else.")
4. Put Default Case at Last:
The order in which the cases are matched follows the top-to-bottom rule. So putting the default / catch-all case (`case _:`) at last ensures that all other specific cases are tested before the code settles for the default action.
By taking these factors into account, resolving syntax errors when using
xxxxxxxxxx
Match Case
becomes undemanding!
Remember, coding is about patience and persistence. Regular practice and focusing on small details will improve writing cleaner, error-free codes. Happy Coding!
For additional references, Python Official Documentary on Match Statement is a great place to start exploring the power of Python’s match-case feature.Whilst diving deeper into Python’s coding system, we may occasionally encounter the “SyntaxError: Invalid Syntax” message. This error typically appears when running “match case” statement in an environment that doesn’t support it due to an outdated Python version. If you’ve experienced this issue, you’re definitely not alone.
Let me offer a closer look at how this issue surfaces:
Firstly, the culprit is almost always your Python version. Herein lies the key – match-case statements are a fairly recent addition to Python and is only available starting from Python 3.10 onwards. So, if your development environment is utilizing any version below that, a SyntaxError will inevitably come up when trying to use match-case statements.
Here is what a typical match-case expression would look like:
xxxxxxxxxx
match object_to_match:
case pattern1:
# do something
case pattern2:
# do something else
But if you run this code on Python 3.9 or below, it would throw:
xxxxxxxxxx
SyntaxError: invalid syntax
Breaking down the error message simplifies the problem drastically. A SyntaxError arises when the Python parser encounters a situation where the provided code does not conform with the language structure, i.e., its “syntax”. So when ‘Invalid Syntax’ pops up, it essentially means that the code isn’t written according to rules and norms Python recognizes.
So how do you navigate this tricky path? You will need to upgrade your Python interpreter, aiming for minimally Python 3.10 if you wish to make use of this concise and expressive construct. Upgrading lets you solve the issue and fully explore match-case constructs, harnessing its potential in creating more compact and readable codes. However, be aware of potential dependencies and backward compatibility issues when upgrading.
In some situations, if upgrading Python is not feasible or practical, rewriting your code to avoid the use of match-case would be the safest alternative. In place of match-case expressions, using traditional if-elif-else conditional statements provide compatibility across all versions of Python. Like so:
xxxxxxxxxx
if condition1:
# do something
elif condition2:
# do something else
else:
# default "case"
The inevitable solution to our SyntaxError problem, therefore, lies in awareness of version compatibility during coding. The evolutionary nature of programming languages – with constant updates and improvements – mandates careful attention to version controls. Thankfully, the Python community is unfailing in their efforts to better the language’s efficiency and running power – which means we can look forward to smoother coding experiences as we move forward. (source)