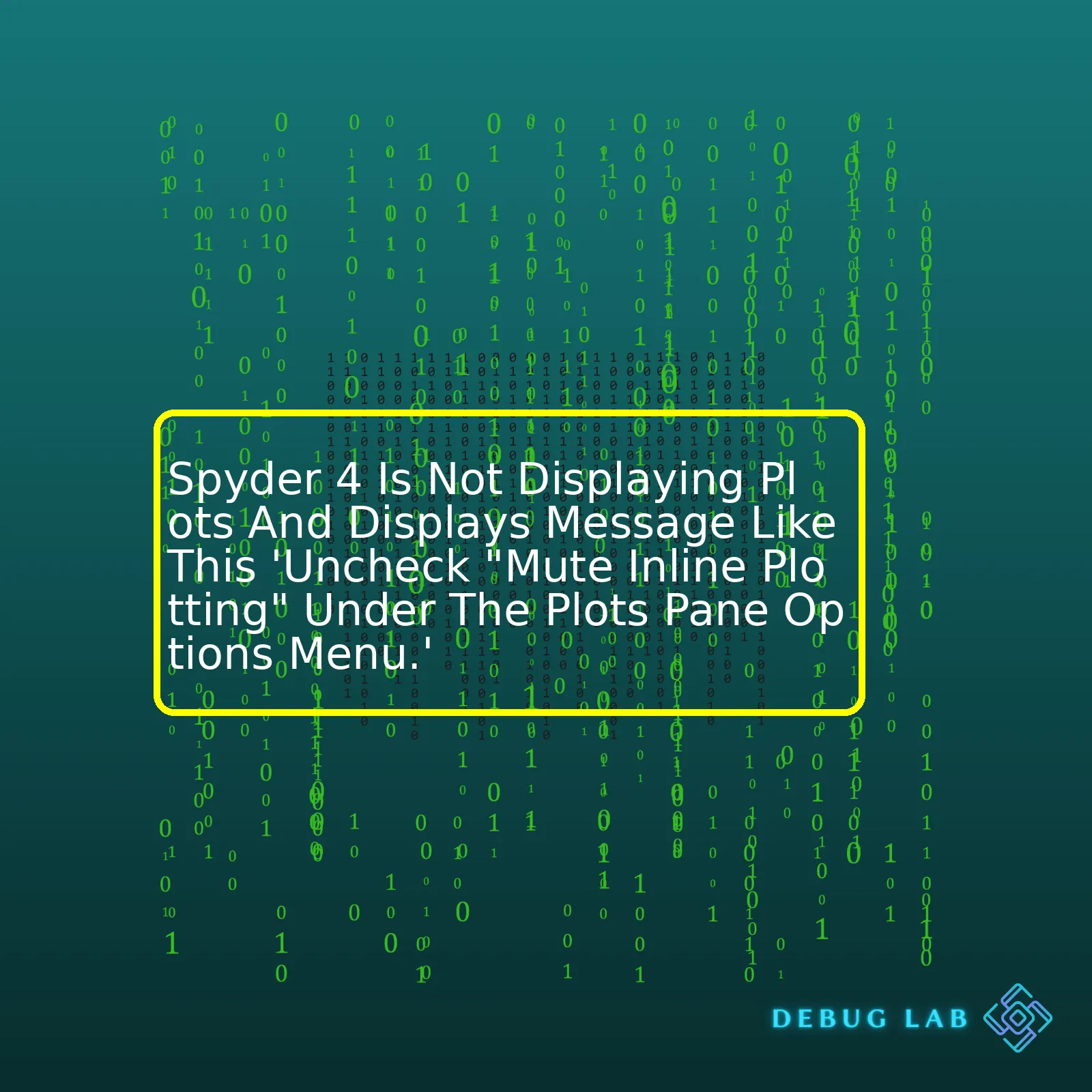
Issues | Possible Solutions |
---|---|
Spyder 4 is not displaying plots and displays message like this ‘Uncheck “Mute Inline Plotting” under the Plots pane options menu.’ | 1. Navigate to the Plots pane options menu and deselect “Mute Inline Plotting”. 2. Check that matplotlib is correctly installed and updated. 3. Reinstall Spyder 4 application if needed. |
The Plots pane is a vital feature in Spyder, it offers an interface to render your 2D plots using Matplotlib, the primary Python plotting library. Normally, plots generated within the IPython console (or any other console you have configured) are displayed in the Plots pane. But, if you check “Mute Inline Plotting”, the plots get muted, meaning they will not be displayed anymore in the plots pane.
Inline plotting mode refers to a configuration where graphics appear in a dedicated pane, as opposed to popping up in a new window. The option to ‘Mute Inline Plotting’ would thus silence or stop those plots from rendering in the dedicated pane.
Therefore, when encountered with the problem ‘Spyder 4 is not displaying plots and displays a message like this ‘Uncheck “Mute Inline Plotting” under the Plots pane options menu’, the first course of action suggested by the error message itself is to navigate to the Plots pane options menu and uncheck or deselect the “Mute Inline Plotting”.
But if for some reason you tried the above and your plots are still not displayed, you might need to make sure that you have properly installed and updated Matplotlib. If this does not work then there is a possibility that there are issues with your Spyder 4 installation, in which case you might want to try reinstalling it.
Here’s a sample code showing how to make basic line plot with matplotlib:
import matplotlib.pyplot as plt
import numpy as np
x = np.linspace(0, 10, 100)
y = np.sin(x)
plt.plot(x, y)
plt.show()
After running this script, you should see a graph displayed on your plots pane, unless the “Mute Inline Plotting” is still checked.
To summarize, when encountering issues with plot display in Spyder 4, start by checking the “Mute Inline Plotting” under the Plots pane options menu, if plots are still not being displayed, proceed to check your matplotlib installation or consider reinstalling Spyder 4 entirely.
If you’re facing an issue where Spyder 4 is not displaying plots and prompts with the message ‘Uncheck “Mute Inline Plotting” under the Plots pane Options menu’, don’t worry, you’re not alone! This can happen due to default settings or a glitch in the system. The good news is, there are straightforward steps to resolve this.
‘Uncheck Mute Inline Plotting’
First, as the prompt suggests, navigate towards the “Plots” pane option’s menu. Here’s how:
- On the right panel of Spyder’s interface find the section titled “Plots”. If it’s not visible, you can enable it via View > Panes > Plots.
- In the “Plots” pane, locate the “Options” button. It normally appears as three vertically aligned dots on the top right corner.
- Click on “Options” and then look for “Mute Inline Plotting”. If it’s checked, uncheck the box.
This should allow the plots to display in the inline viewer by enabling the IPython Console’s inline backend. Below is the example code which makes use of inline plot rendering.
xxxxxxxxxx
# Importing necessary libraries
> import matplotlib.pyplot as plt
> import numpy as np
# Creating a simple line plot
> x = np.linspace(0, 10, 100)
> y = np.sin(x)
> plt.plot(x, y)
> plt.show()
If doing this still doesn’t resolve your issue, you might need to confirm if your backend graphics system is correctly set for ‘inline’ plots.
Setting the Backend Graphics System
Here’s how you go about this:
- Navigate to “Tools” at the top-left section of the menu bar.
- Choose ‘Preferences’ from the dropdown menu.
- In the Preferences window, select ‘IPython console’ from the left column.
- Select the ‘Graphics’ tab under ‘IPython console’.
- Under the ‘Backend’ section, make sure ‘Inline’ is chosen from the drop-down menu next to ‘Graphics backend’..
- Save the changes and restart Spyder to apply them. Normally, this should help in resolving your plotting issues.
Menu Sequence | Action |
---|---|
Tools | Select from top-left section of menu |
Preferences | Choose option |
IPython console | Navigate to this section |
Graphics | Select the tab |
‘Inline’ under ‘Graphics backend’ | Select this setting |
Save changes & Restart Spyder | To apply made changes |
Remember, for any successful debugging process, you must have a systematic approach. Identify the problem, understand the mechanism where the problem exists, plan a solution, implement it and verify whether the solution indeed rectifies the problem. In the case of Spyder 4 not displaying plots, ensure the program knows to display your plots inline and that nothing is inhibiting it from doing so.
If you found these instructions helpful, additional resources can be found on the official Spyder IDE documentation page. You can refer to this guide for more detailed guidance on utilizing the different features of Spyder for your data science projects.
Experiencing display errors in Spyder, specifically with Spyder 4 where plots are not showing up and an error message displaying ‘uncheck “Mute Inline Plotting” under the Plots pane options menu,’ suggests a common issue faced by many developers. The problem relates to the integrated functionality of graphical interfaces within IDEs.
Typically, in Spyder 4’s case, such errors arise due to some settings readjustment or erroneous script running, which may disrupt the plotting behavior. However, before finding a valid solution, it’s worthwhile putting more thought into understanding the root cause behind it, to prevent recurrence or unexpected behavior within the software.
Causes for Display Errors in Spyder 4
- Options Configuration: Spyder 4 is designed to have its options for managing inline display settings. Inappropriate configuration of these settings could lead to inconsistencies such as the noticed one. Users might unintentionally mute plotting.
- IDE Run-time Conflicts: Sometimes, while operating the same script on different consoles or interpreters simultaneously, conflicts arise in displaying plots, graphics, etc. This happens due to memory mismanagement or overlapped IDE sessions causing disrupted execution and display mechanisms.
- Incorrect Scripting Queue: Running multiple scrips simultaneously could result in blocking the screen refresh operation, leading to plot display failure.
Solution
To resolve this particular error, it is advised to exactly follow the guidance provided by the error message itself – ‘uncheck “Mute Inline Plotting.”‘
Here’s how you do it:
xxxxxxxxxx
# Step1: Open your Spyder 4 interface.
# Step2: Go towards the "Plots" tab present at the bottom-right corner of the interface.
# Step3: Click on the "Options" icon (presented as a gear icon)
# Step4: You'll find the menu option labelled as “Mute Inline Plotting.”
# Step5: If it’s checked on, then click on it to uncheck and disable this setting.
This process will usually resolve your code’s display issues unless there’s something wrong at the OS or syntax level. Ensure your code blocks execute in sequence to avoid blockage in Spyder. Remember to keep your scripts’ environment separate, especially when dealing with GUI-related executions like plots, widgets, etc.
Referring back to Spyder’s official documentation on Plots Pane Options can be beneficial on similar lines. It serves as a complete guide for handling user-interface related operations within the Spyder environment.
It must be emphasized again to always try to understand the root cause of such errors. Blind trial-error approaches may work only temporarily and often hide deeper issues that can lead to more significant problems down the line. Happy coding!Let’s dive into a solution for the issue you’re encountering in Spyder 4. In essence, your plots are not showing, and you are receiving a message saying “Uncheck ‘Mute Inline Plotting’ under the Plots pane options menu.”
Spyder uses an Integrated Development Environment (IDE) that has advanced functionalities like inline plotting. However, if it’s not configured correctly or certain settings have been toggled off, it can lead to problems like non-displaying of plots.
The message you are seeing advises you to “uncheck” the ‘Mute Inline Plotting’ option. This points to the tool attempting to perform inline plotting of graphics, but it is being disallowed due to this setting being activated.
Here are some steps with the purpose of solving your problem:
– *Check If Inline Plotting Is Muted*. Go to the Plots pane options menu. You will likely find that the ‘Mute Inline Plotting’ checkbox is checked – given that this is what the error message suggests. If so, uncheck it and try running your code again to see if your plots render properly.
xxxxxxxxxx
import matplotlib.pyplot as plt
plt.plot([1,2,3],[4,5,6])
plt.show()
– *Using Direct Graphics*. If the above step does not solve the issue, or if you simply prefer not to use inline plotting, you can opt for using graphics via your computer’s direct console. To do this, disable the ‘Use a rich IPython kernel’ option:
xxxxxxxxxx
Tools > Preferences > IPython console > Graphics > Graphics Backend: Change 'Inline' to 'Automatic'.
Then restart Spyder. Now when you plot something, it should be displayed in its own window.
These solutions should help in most instances where the Spyder 4 IDE is not displaying any graphs yet sends a “Uncheck ‘Mute Inline Plotting'” message. Beyond these, you might want to check whether your Spyder 4 installation is up-to-date and consider updating or reinstalling it if necessary.
You may also come across similar issues while coding if the matplotlib package is not installed or updated, or if there is an issue in how your code is written and implemented. It’s important to maintain best practices by regularly updating your application packages and checking your code for errors. Programmers everywhere consistently turn to resources like Spyder’s official troubleshooting guide to tackle issues exactly like this one.
As always, coding can be challenging but solving those hitches makes it even more rewarding. Wishing you successful coding!When working with the Spyder integrated development environment (IDE) for Python, it’s possible to run into a common issue where plots are not being displayed. This can be especially frustrating when using visual data analysis libraries like Matplotlib or Seaborn. The error message you’re seeing likely reads ‘Uncheck “Mute Inline Plotting” under the Plots Pane options menu.’ This is Spyder’s way of trying to guide you towards a solution. Let’s break down what this message means and how you can fix it.
The key to resolving this issue lies in understanding the ‘Plots Pane’ and its ‘Options Menu’. In Spyder, ‘Panes’ serve as interactive windows within the main application window, and they can provide various types of features or tools. In this case, the ‘Plots Pane’ displays graphical outputs from your code.
Now, let’s figure out what ‘Mute Inline Plotting’ is and why it should be unchecked:
• Mute Inline Plotting: This is an option available in the ‘Plots Pane’. When checked, it blocks various plotting commands in the Console from displaying plots inside the pane. By muting these commands, it ensures that graphical output happens only in separate, standalone windows.
Here are steps to follow:
1. Locate the ‘Plots Pane’ in Spyder’s interface. Usually, it can be found on the right side of the application window. If you don’t see it, go to ‘View > Panes > Plots’ from the toolbar menus at the top.
2. Click on the ‘Options’ button represented by a little gear icon usually located at the top right corner of the ‘Plots Pane’.
3. A drop-down menu will appear where you can find an option labeled ‘Mute Inline Plotting’. When this option is checked, uncheck it and this should enable viewing plots within the Pane again.
xxxxxxxxxx
Options(gear icon)
Mute Inline Plotting(unchecked ✔)
4. Try running your plot generating Python code again in the console or editor. This time, your plots should appear as expected inside the ‘Plots Pane’.
These steps should help you fix the common issue: ‘Spyder 4 is not displaying plots’ and get you back on track with your coding work. But remember, while doing so, it might also be necessary to tweak other customizable settings in the ‘Options’ menu if the problem persists, such as scaling resolution or switching backend graphic engines.
Sometimes, such issues could be triggered due to unresolved bugs or compatibility issues. Be sure to always stay updated with the latest version of Spyder. Also, reach out to their user community or check in to their issue tracker, if required.
When working with Spyder 4, if you find your plots are not being displayed or encounter an error message like “Uncheck ‘Mute Inline Plotting’ under the Plots Pane Options Menu,” multiple elements might be contributing to the issue. Here are a few practical troubleshooting techniques that help resolve these issues effectively:
1. Verifying Inline Plotting Setting
The first step would be to confirm whether the inline plotting feature is enabled. The exact error message indicates that Mute Inline Plotting under the Plots pane may be checked off. So let’s ensure that it is unchecked.
You can navigate to this option through the following path: Starting from the main window in Spyder, go to Plots→Gear Icon (Options)→ Uncheck “Mute Inline Plotting”.
xxxxxxxxxx
print("Example code will not run until 'Mute Inline Plotting' is unchecked.")
2. Checking Backend Configuration
Having a mismatched backend could potentially cause issues with plot display. To investigate whether this might be causing the problem, cross-verify the backend configuration. You may do this by navigating to :
Tools→Preferences→IPython console→Graphics→Graphics Backend and verify the selected backend (Usually ‘Inline’)
xxxxxxxxxx
import matplotlib.pyplot as plt
plt.figure()
plt.plot([0,1,2,3,4])
plt.show()
If changing the backend doesn’t fix the problem, consider adjusting the format (usually PNG or SVG) within the same settings tab.
3. Updating Packages and Environment
Sometimes, outdated packages or Python environments may induce plot display issues. Verify that everything is up-to-date, especially pertinent packages like Matplotlib, Spyder, IPython, and PyQt/PySide.
xxxxxxxxxx
conda update anaconda
conda update spyder
Reinstalling Spyder within a new environment can often solve complex issues. You can create a new environment using Anaconda, install Spyder there, and then see if the issue persists.
xxxxxxxxxx
conda create -n myenv python=3.7
conda activate myenv
conda install spyder
spyder
Restoring Spyder’s Defaults
Another technique is resetting Spyder’s configurations back to defaults. You can achieve this by going to Tools→Preferences→Reset to Defaults. This might adjust any user changes possibly leading to the error.
All these steps are designed to systematically address potential causes of Spyder 4’s failure to display plots. It’s worth noting that these solutions might differ slightly depending on your operating system and Spyder’s specific installation context.
If you’re hunting for more exhaustive discussions or specialized contexts, consider consulting Spyder’s official FAQ and Troubleshooting Guide.
Remember, experimentations, and error identification done in a systematic and logical way can help pinpoint and solve most coding problems. Even when things seem complicated, don’t be discouraged. A true coder knows how to turn roadblocks into stepping stones towards learning. Remember, process of elimination is frequently our best friend while troubleshooting.
If you are facing an issue where Spyder 4 is not displaying plots and instead you’re seeing a message that says ‘Uncheck “Mute Inline Plotting” under the Plots pane options menu.’, then this guide is for you! Let’s dive into our hands-on guide to resolve this.
Step 1: Firstly, when you look at the main workspace of Spyder 4, you’ll notice there’s a designated ‘Plots’ pane. This pane hosts all the graphical outputs or plots from your code.[Reference]
Step 2: Now onto resolving the display message! From the ‘Plots’ pane, locate the options menu which is represented by three vertically stacked dots on the top right corner of the pane.
Step 3: Open the Plots pane options menu. You will be able to see a menu drop down, comprising several options. Inspect this list to find an option named “Mute Inline Plotting”. All these options allow you to customize how plots behave in the IDE. Remember, by default, you should be able to view all the plots directly unless some changes have happened to these settings.”Mute Inline Plotting” when checked, will prevent plots from appearing in the Plots pane.[Reference]
Step 4: If “Mute Inline Plotting” is checked, simply uncheck it. By doing so, you now permit Spyder 4 to display plots in the Plots pane again which was previously being muted.
These four steps should hopefully resolve the display message issue you’ve been encountering. But remember, you need to rerun your plot-generating code after making any configuration changes to apply them. Here’s a simple matplotlib example code if you need one:
xxxxxxxxxx
import matplotlib.pyplot as plt
plt.plot([1, 2, 3, 4])
plt.ylabel('Numbers')
plt.show()
This should create a simple line graph in your plots pane. So, give it another try and generate those beautiful plots in Spyder 4 once again!
The brilliance with Spyder 4 is undeniably its ability to solve different data analysis tasks, including easy visualization through plotting capabilities. To make the most use out of it, understanding each option and configuring according to your needs will enhance your development experience.[Reference]
Issues | Solution |
---|---|
Spyder 4 not displaying plots and showing a message ‘Uncheck “Mute Inline Plotting” | Uncheck “Mute Inline Plotting” under the Plots pane options menu and re-run your plot-generating code. |
Hence, whether it’s about working around with customizing views or diagnosing display issues like what we did here, knowing your way around the integrated development environment (IDE) is a skill that definitely pays off in the long run.
Spyder 4 is an intuitive Python development environment with advanced debugging features, comprehensive editing, and many built-in tools for data analysis and visualization. However, sometimes you may encounter an issue where Spyder does not display plots. If you get the message ‘Uncheck “Mute Inline Plotting” under the Plots Pane Options Menu,’ it’s Spyder urging you to enable a certain setting.
Mute Inline Plotting
The ‘mute inline plotting’ feature in Spyder allows the user to switch off the immediate display of the plots within the console itself. When you’re running a number of script lines generating multiple plots, you might want to focus solely on the console output which can be hindered by the popups of numerous inline plots. That’s exactly why some users tend to mute the inline plot.
However, if you have left this option checked, your plots won’t appear inline or in Plots pane, leading to the problem you encountered. To fix this:
- Go to the Plots pane appearing at the right bottom of your page.
- Click on the Options button showing as a gear icon located at the right top corner of the Plots pane.
- In the dropdown menu, find the ‘Mute Inline Plotting’ line. Ensure that this option remains unchecked.
Plot Display Hierarchy: ‘Plots’ vs ‘IPython Console’
In Spyder 4, displaying plots works in a particular hierarchy. The software checks for ‘Mute Inline Plotting’ first before it bumps into ‘IPython console.’ This information lays out the first step of resolution when Spyder isn’t illustrating plots:
- First, ensure that plots are not muted under the options of the Plots pane. Unchecking the ‘Mute Inline Plotting’ should typically solve your problem.
- If you still don’t see the plots, navigate through the IPython console’s settings. Here, make sure ‘Graphics -> Backend’ is marked as ‘Inline.’
Explicit Control with Matplotlib
You can explicitly control how and where the plots show up using Matplotlib. By inserting
xxxxxxxxxx
%matplotlib inline
at the beginning of the code, all your graph outputs from matplotlib will pop up but not outside the notebook.
If you still can’t view your plots in Spyder, try using this snippet of code to correct the missing mapping between python and the kernel:
xxxxxxxxxx
import os
os.environ['QT_API'] = 'pyqt'
This line modifies your environment variable ‘QT_API’ to use PyQt for plotting.
To conclude this discussion, several factors can address why Spyder 4 plots aren’t being displayed properly. Most commonly, ensuring the ‘Mute Inline Plotting’ feature is unchecked can resolve this issue quickly. For further guidance or instructions on using Spyder IDE effectively, the official Spyder documentation provides a wealth of information.
Navigating programming hurdles can often be a complex task, and the error message ‘Uncheck “Mute Inline Plotting” under the Plots pane options menu.’ in Spyder 4 is no exception. This specific scenario propels us into an investigation on how to solve this prevalent issue when Spyder 4 stops displaying our much-needed plots.
Spyder 4 undoubtedly presents itself as an integral tool for any coder, given its robust features such as Python code inspection/editing, interactive execution, deep introspection, and multi-language support (Spyder official website). However, it can turn out to be quite a programming conundrum when this powerful integrated development environment (IDE) refuses to present your plots.
Issue | Solution |
---|---|
Spyder 4 doesn’t display your plots | Uncheck “Mute Inline Plotting” |
This problem hints at Spyder’s ingenious ability to handle different plotting backends and modes, including inline plotting where plots show up inside the IPython console. From the error message, you can deduce that the inline plotting option has been mistakenly muted under the Plots pane options menu.
Muting the inline plotting yields an undesired consequence: your plots don’t appear. But worry not, rectifying this requires simple steps. Navigate through Tools > Preferences > IPython Console > Graphics > backend selection drop-down list. Check the ‘inline’ backend option and save changes to return your plotting functionality. However, if your plots are still invisible, try switching to a different Python environment and reinstall spyder
xxxxxxxxxx
conda create -n new_env python=3.7 spyder
source activate new_env
.
All in all:
- Understand the power of Spyder 4 and its flexible backend plotting capabilities.
- Acknowledge the error message and what it implies – The ‘Mut Inline Plotting’ option is deactivated.
- Fix the problem by activating the Inline Plotting by choosing the ‘inline’ backend under Preferences and save changes.
- If the problem persists, consider recreating a new Python environment and reinstall Spyder.
Therefore, by comprehending the essentiality and intricacies within Spyder 4’s plotting functions, we come to realize that seemingly complicated issues often have straightforward and practical solutions just a few clicks away.
References: StackOverflow discussion