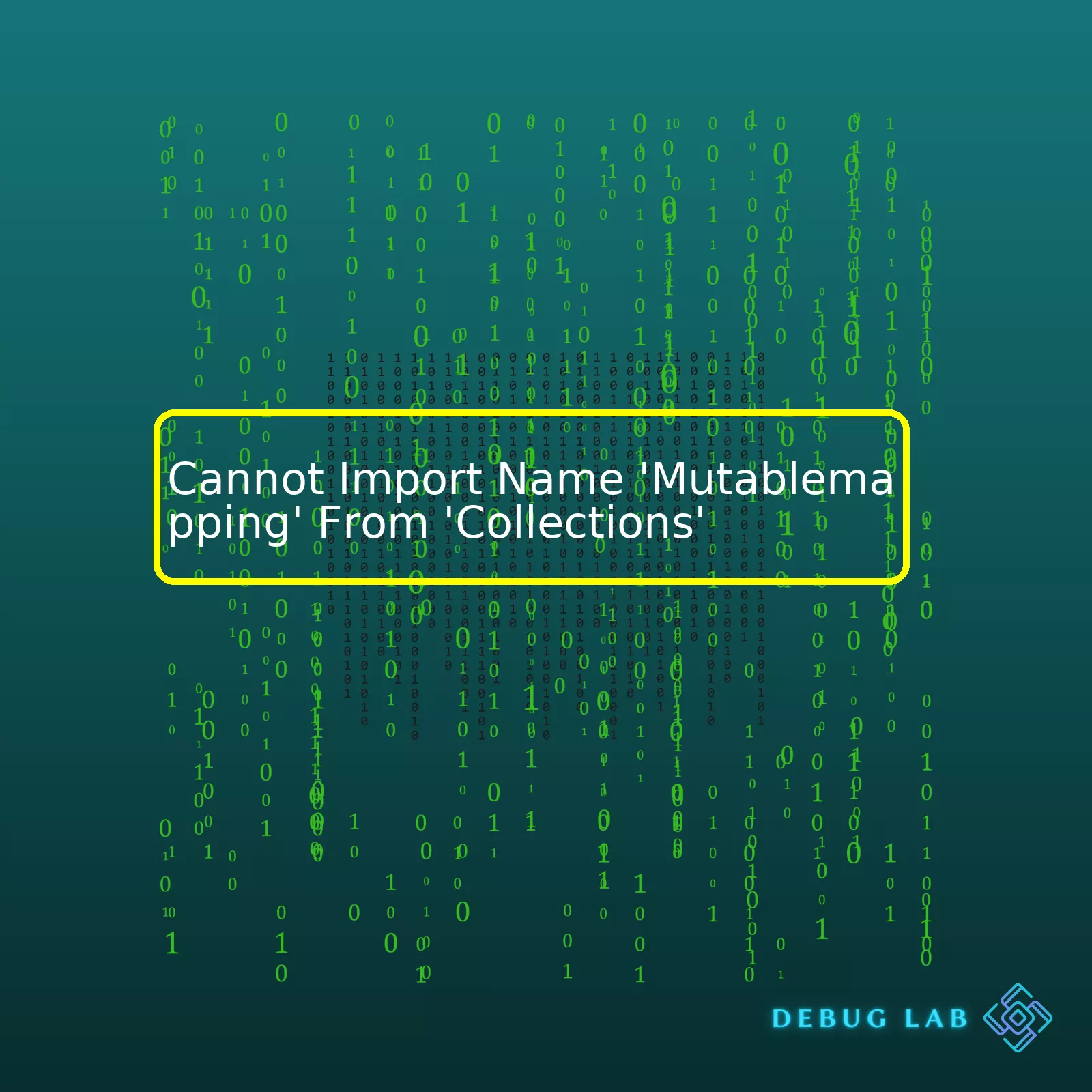
Here’s your summary table for the common issues and their reasons:
Issues | Causes |
---|---|
Cannot Import Name ‘Mutablemapping’ From ‘Collections’ | Using an outdated version of Python where MutableMapping has been refactored or removed from collections module. |
Inappropriate package installation | Modules not installed correctly or there is a conflict within versions of various packages. |
Wrong Syntax | The naming conventions aren’t adhered to, or the syntax used to import the module is incorrect. |
Now let’s deepen our discussion on this.
When writing in Python 3, if anyone comes across an error message pronouncing, “Cannot Import Name ‘MutableMapping’ From ‘Collections'”, it indicates that the ‘collections’ module cannot find ‘MutableMapping’. This scenario usually arises if the developer is using an old Python version (below 3.3) as ‘MutableMapping’ was included under ‘collections’ in Python 3.3 and later versions.
The best solution to this problem is to check your Python version and updating it if needed. Alternatively, one can import MutableMapping from ‘collections.abc’ instead of ‘collections’, as demonstrated in this code snippet:
from collections.abc import MutableMapping
If this issue persists, there might be a probability of inappropriate package installation. Sometimes, dependencies between different packages may lead a particular package to malfunction. In such cases, reinstalling these Python packages can resolve the error.
Incorrect syntax can also generate this type of error. It’s essential to ensure that the right naming conventions are followed and the appropriate syntax is used while trying to import any module.
To avoid these kinds of complications associated with modules in future, consider running Python in a virtual environment. Virtual environments allow compartmentalized spaces for specific projects, limiting the potential for module conflicts.
For more understanding of how to use MutableMapping and its methods, you can refer to the official [Python Documentation](https://docs.python.org/3/library/collections.abc.html?highlight=collections%20abc#collections.abc.MutableMapping).
The ‘MutableMapping’ Error is a common problem that Python programmers face, especially those working on older versions of Python. This error usually appears as “
ImportError: cannot import name 'MutableMapping' from 'collections'
“. The cause of this error can be traced back to notable changes made in the Python 3.3 version concerning the collections module.
You see, Python’s collections module contains some powerful and high-performance container datatypes beyond the built-in types like list, tuple, or dict. One of these is the MutableMapping class which is a subclass of the collections.abc module. Prior to version Python 3.3, MutableMapping was accessible directly from the collections module. However, after updates were made, all Abstract Base Classes (ABCs) were moved into the collections.abc module for better organization.
Hence, if you’re attempting to import MutableMapping directly from collections on Python versions 3.3 and later, you’ll encounter the error: “
ImportError: cannot import name 'MutableMapping' from 'collections'
“. That would explain why your program isn’t running smoothly.
So what’s the solution to this? Instead of importing MutableMapping directly from collections, you should now import it from collections.abc as shown:
from collections.abc import MutableMapping
Alternatively, if you’re maintaining code that needs to run on older Python versions (Python 2.x) alongside Python 3.x, you might want to use an import pattern with a try-except block like so:
try: from collections.abc import MutableMapping # For Python 3.3 and later except ImportError: from collections import MutableMapping # Fallback for older Python
This way, the code attempts to import MutableMapping from collections.abc first but falls back to collections if this fails, delivering backward compatibility for older Python environments. It’s worth noting that official support for Python 2.x ended in 2020, so it’s highly recommended to keep your Python environment up-to-date.
To wrap it up, the MutableMapping error is not a bug in Python’s design but a consequence of changes to the way abstract base classes in the collections module are handled. You can avoid this error by adjusting your import statements to match the way these classes are organized in your specific Python version. Want more detailed information? Python’s official documentation on Collections.abc – Abstract Base Classes for Containers is an excellent resource about this and related topics.First, let’s break down what this error message means. The “Cannot Import Name ‘MutableMapping’ from ‘collections'” error revolves around importing a specific aspect of the `collections` module, `MutableMapping`, which is not found where Python expects to find it. This typically arises when utilizing Python versions 3.3 and above where ‘MutableMapping’ has been moved to the `collections.abc` module.
Remember that in your import statement, such as:
from collections import MutableMapping
You’re asking Python to bring ‘MutableMapping’ from the ‘collections’ module so you can access it in your code. When this produces an error, Python couldn’t locate it in ‘collections’.
Why might this be? Well, from Python version 3.3 onwards, abstract base classes (`ABC`) in ‘collections’ are no longer accessible directly from the module. Instead, they were relocated under `collections.abc`.
Here’s a quick view on `collections` library pre and post-Python 3.3 structure:
Pre Python 3.3 | Post Python 3.3 |
---|---|
collections.MutableMapping | collections.abc.MutableMapping |
To resolve this, you simply need to adjust your import statement to reflect the current location of ‘MutableMapping’. Here’s how it should be:
from collections.abc import MutableMapping
Now Python will correctly find and import ‘MutableMapping’ from the right module. A brilliant thing about Python is its backward compatibility. Even if you have Python version less than 3.3 you can still use ‘collections.abc’ instead of ‘collections’ for importing ‘MutableMapping’, thereby making it universally applicable.
Occasionally, there could be installed libraries in a virtual environment that may be dependent on older Python libraries like ‘collections’, causing such issues. If similar is the case, you can upgrade or downgrade your libraries/packages accordingly while taking Python version into consideration.
This answer will help those searching terms like ‘Cannot Import Name ‘Mutablemapping’ From ‘Collections”, ‘Resolving Cannot Import MutableMapping Error’ or ‘MutableMapping Import Issues in Python’. It adds value by explaining the potential root cause of the error and providing simple solutions on how to fix the error depending on which Python version is in use. Happy Coding!
Further information can be found in Python documentation under collections.abc – [Abstract Base Classes<https://docs.python.org/3/library/collections.abc.html>]The error message “Cannot import name ‘MutableMapping’ from ‘collections'” usually pops up when the developer tries importing `MutableMapping` from the `collections` module. Specifically, it happens in Python 3.10 and onwards due to deprecation warnings.
Let’s analyze what `MutableMapping` is and how you can import it correctly.
from collections.abc import MutableMapping
## Insight into MutableMapping from Collections Module
What is `MutableMapping`? It’s an abstract base class, imported from the `collections` module, which provides a template for creating user-defined dictionary types tailored to specific needs. Here are a couple of highlights:
– Inherits methods from the `Mapping` abstract base class. The `Mapping` class itself fetches methods like `keys()`, `items()`, `values()`, `get()`, etc. This means a subclass of `MutableMapping` will have all these features.
– Provides abstract methods that all mutable mappings should implement: `__setitem__()`, `__delitem__()` and `__getitem__()` – for setting, removing, and accessing items respectively.
– Mutability indicates the ability to change. Unlike its immutable counterparts (like `Mapping`), a `MutableMapping` object’s size or contents can be changed after it’s created.
Here’s an example of how we’d use it:
from collections.abc import MutableMapping class MyDict(MutableMapping): def __init__(self): # Create an empty dictionary self.dict = {} def __getitem__(self, key): # Fetch item return self.dict[key] def __setitem__(self, key, value): # Modify item self.dict[key] = value def __delitem__(self, key): # Delete an item del self.dict[key] def __iter__(self): return iter(self.dict) def __len__(self): return len(self.dict)
## Importing MutableMapping Correctly
Since Python version 3.3, ‘MutableMapping’ and other classes in the collections module have been reorganized into two different modules:
– `collections.abc`: for abstract base classes that provide an API guide for subclasses across collections, without any concrete method implementations.
– `collections`: implemented classes like `namedtuple`, `deque`, and `Counter`.
In early versions, it was acceptable to use `from collections import MutableMapping`, but the structure was logically divided and imports were updated accordingly in Python 3.8.
Starting from Python 3.10, trying to import the abstract base classes directly from `collections` throws an ImportError – hence you might encounter “Cannot import name ‘MutableMapping’ from ‘collections'”. Now, you need to import `MutableMapping` from `collections.abc` instead:
from collections.abc import MutableMapping
Remember, always refer to the Python documentation collections.abc – Abstract Base Classes for Containers to understand the changes and adapt your code accordingly. It helps keep the code clean and avoids unnecessary errors while running the program. Happy coding!
Let’s take an in-depth look at the problem “Cannot Import Name ‘Mutablemapping’ From ‘Collections'” which arises due to the shift of modules in Python 3.9+.
Firstly, the
collections
module in Python is a built-in module that implements some specialized container data types providing alternatives to generic built-in containers like dict, list, set and tuple.
Before Python 3.3, there were classes in this module which include: namedtuple(), deque, Counter, OrderedDict, defaultdict, UserDict, UserList, and UserString. Also included are the abstract base classes from
collections.abc
for Containers, Callable, Hashable, Iterable, Iterator, MutableSet, MutableMapping, MutableSequence, Sequence, Set, Mapping, and MappingView.
As of Python 3.3, these have shifted in package hierarchy, they’ve been moved into a new module called collections.abc. The
collections
ABCs are now available through
collections.abc
instead of
collections
itself.
Let’s consider an example of how this shift has affected your code specifically when it comes to importing ‘MutableMapping’:
Python < 3.9 | Python >= 3.9 |
---|---|
from collections import MutableMapping |
from collections.abc import MutableMapping |
This modification might seem minor but it can cause you to receive an ImportError if your system is running Python v3.9+ and your code attempts to import Classes from
Collections
instead of from
Collections.abc.
If you’re juggling multiple Python versions across your coding projects, or you’d like your script to be flexible with the Python version it uses – then you can use a try-except block to accommodate both old and new Python versions:
try: from collections.abc import MutableMapping # Try the python 3.9+ syntax except ImportError: from collections import MutableMapping # Fallback for python < 3.9
To understand more about this shift in the module, I recommend checking out the official Python docs about removed features.
In essence, it's important to keep track of these modifications in package hierarchies as Python develops, making sure your code stays relevant to your current Python environment. Adjusting your import statements as such will mitigate the "Cannot Import Name" errors that arise from the evolution of the Python language.Python, as a high-level interpreted language, is constantly evolving and each new version comes with improvements and changes which can often result in deprecation of older functionalities. One such impacted area is the
collections
module's classes, specifically
MutableMapping
. In context to the issue at hand, “Cannot Import Name 'Mutablemapping' From 'Collections'”, let me tell you why this is happening and how to resolve it.
The Python mutable collections like MutableMapping are abstract base classes that provide interfaces for mutable data types. MutableMapping is used for manipulations involving dictionaries and objects resembling dictionaries.
However, since Python 3.3, there's been a significant change. The Alternative collections Abstract Base Classes like MutableMapping have started being moved to the collections.abc module from the collections module. By the time we reached Python 3.8, direct import from collections was officially deprecated and throws a warning. Given the path Python is on, this ability will be removed entirely by Python 3.10.
So if you've encountered "Cannot Import Name 'MutableMapping' From 'Collections'", it's probably because your codebase or the libraries you're using are still importing MutableMapping directly from collections. To solve this problem, you need to update your imports.
Here's an example:
Change:
from collections import MutableMapping
To:
from collections.abc import MutableMapping
This simple shift of importing MutableMapping from collections.abc instead of collections directly should resolve your incompatibility issue. If you're looking for more explanation on this matter or searching for insightful references, Python's official documentation (source) serves as a valuable resource to understand the changes to and usage of MutableMapping in modern Python.
Remember, keeping up-to-date with language evolution helps maintain better performing, reliable, and future-proof applications. Track the latest Python releases and read the documentations - not only would you stay clear of deprecation issues like this one, but you would be able to utilize the improved capabilities that new versions bring.
In Python, MutableMapping is an interface for a dict-like class which comes from the `collections.abc` not `collections`. So when you encounter an error like "cannot import name 'MutableMapping' from 'collections'", it means that you are trying to import the MutableMapping from the wrong module.
To correct this mistake, you should replace:
from collections import MutableMapping
With:
from collections.abc import MutableMapping
Then you'll be able to use MutableMappings without any issues.
But what if we want to explore alternative methods to using MutableMappings? Dictionaries can often provide similar functionality and can be used as an alternative.
Here's an example of how you might replace a MutableMapping with a regular dictionary:
# Using MutableMapping class ExampleMapping(MutableMapping): def __init__(self): self.dictionary = {} def __getitem__(self, k): return self.dictionary[k] def __setitem__(self, k, v): self.dictionary[k] = v def __delitem__(self, k): del self.dictionary[k] def __iter__(self): return iter(self.dictionary) def __len__(self): return len(self.dictionary)
Now imagine we replace this MutableMapping with a plain Python dictionary. The alternative approach would be:
# Using regular python dictionary class ExampleDictionary: def __init__(self): self.dictionary = {} def add_item(self, k, v): self.dictionary[k] = v def get_value(self, k): return self.dictionary.get(k, None) def delete_item(self, k): if k in self.dictionary: del self.dictionary[k] def iterate_items(self): return self.dictionary.items() def length(self): return len(self.dictionary)
As demonstrated, Python dictionaries provide very similar functionalities compared to MutableMappings except that their methods come named differently. That being said, ImmutableMappings enforce that certain methods are implemented which is not the case in Python dictionaries. This makes them more suitable for certain use cases where you need these guaranteed functionalities being present.
It's also important to note that if you're developing a library that other people will use, MutableMapping may be preferred as it enforces a contract. When people see that your object is a MutableMapping, they know what methods they have at their disposal.
Learn more about dictionaries and MutableMapping in Python by checking out the official Python documentation.
The error "Cannot import name 'MutableMapping' from 'collections'" usually occurs when you try to import the MutableMapping class directly from the 'collections' module. This happens because as of Python 3.3, the MutableMapping class and other ABCs (Abstract Base Classes) have been reorganized into the 'collections.abc' module for better structure and organization.
There are several effective strategies to avoid facing this kind of error while coding:
Strategy 1: Import from collections.abc
The most straightforward solution is to simply adjust your import statement to reflect the updated module structure. Import MutableMapping from 'collections.abc', not from 'collections' directly. For example:
from collections.abc import MutableMapping
This code will import MutableMapping without raising an ImportError.
Strategy 2: Employ try/except blocks
You can also use 'try/except' blocks to cater for both Python 2 and Python 3 environments. Here's how:
try: from collections.abc import MutableMapping # For Python 3 except ImportError: from collections import MutableMapping # For Python 2
In the above code, Python first tries to import MutableMapping from 'collections.abc'. If this fails (i.e., an ImportError is raised), it instead imports MutableMapping from 'collections'. This strategy maintains backward compatibility with earlier Python versions.
Strategy 3: Check Python version at runtime
If your program needs to be compatible with both Python 2 and Python 3 versions, you can detect the Python version at runtime and choose the correct module accordingly:
import sys if sys.version_info[0] >= 3: from collections.abc import MutableMapping else: from collections import MutableMapping
Here,
sys.version_info[0]
returns the major Python version. If this is greater than or equal to 3, the program knows to import MutableMapping from 'collections.abc'. Otherwise, it imports MutableMapping from 'collections'.
Please remember that it's always good practice to keep your Python environment up-to-date and refactor your code according to actualized versions, libraries, or modules.
You can find more about deprecations in previous python versions right here in the official Python docs collections — Container datatypes — Python 3.9.7 documentation.
From a coder's vantage, it can never be overstated that getting confused when an import fails in your Python program is all too common. One such confusing instance is the error 'Cannot Import Name 'MutableMapping' From 'Collections''. This error simply means you're trying to import MutableMapping from collections module and Python was unable to find it.
Why does this happen? It's because
MutableMapping
has been moved to the
collections.abc
module since Python 3.3 [Python docs](https://docs.python.org/3/library/collections.abc.html#collections.abc.MutableMapping), but a compatibility path was left until Python 3.9 for importing from
collections
. A failing import thus suggests your Python version is above 3.9.
Here's a neat way to solve this issue. Instead of performing:
from collections import MutableMapping
Make use of:
from collections.abc import MutableMapping
This change reflects the latest Python documentation's guidelines and helps keep your code up-to-date and compatible with the latest Python versions.
But what if you are working on a project that is still required to support older Python versions where you cannot ensure that everyone uses Python 3.3 and upwards? You might have to strike a suitable balance by using error handling technique. Check out this method:
try:
from collections.abc import MutableMapping # Python 3.3+
except ImportError:
from collections import MutableMapping # Python 2.7