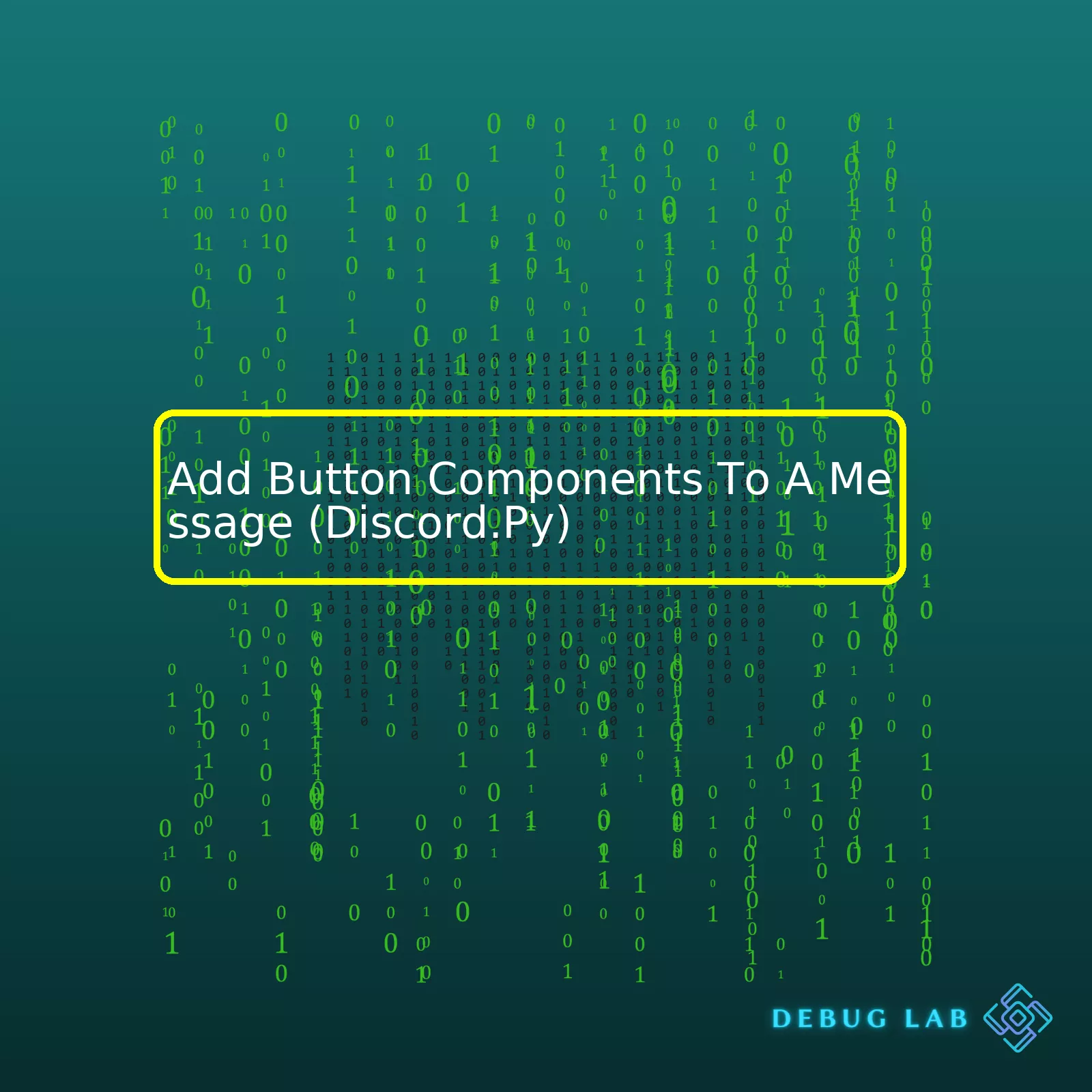
discord.py
library to add button components. This is one of the most popular python libraries for creating bots on Discord.
Commands | Description |
---|---|
xxxxxxxxxx 1 1 1 button() |
This function creates an instance of Button Component. |
xxxxxxxxxx 1 1 1 add_component(component) |
This method adds a component to the message (like button). |
xxxxxxxxxx 1 1 1 set_components(*components) |
This method sets the components for the message (like button). |
xxxxxxxxxx 1 1 1 send(content=None, *, tts=False, embeds=None, file=None, files=None, delete_after=None, nonce=None, allowed_mentions=None, reference=None, mention_author=None, view=None) |
This method actually sends the message with all defined components to the Discord server. |
Now, it’s time for the paragraph elaborating these commands in detail:
To send messages with components like buttons in Discord using
xxxxxxxxxx
discord.py
, we make use of several functions. Starting with
xxxxxxxxxx
button()
. It is a class that creates an instance of the Button Component. Buttons are structures that represent a Discord message Button component. They have label, style, and custom id fields that define how they look and behave.
But a Button Component requires a Message to be assigned on, that’s why other methods come into play. Python-Discord delivers a well-designed function
xxxxxxxxxx
add_component(component)
. It’s a function responsible for adding a button (Button Component) to the message to be sent to the server which gives us the ability to interact with users in different and creative ways.
We can also replace existing message components in bulk using
xxxxxxxxxx
set_components(*components)
where components parameter signifies the iterable of components to set into the message.
After the preparation of the message with buttons, we turn on to the main function for execution which is
xxxxxxxxxx
send()
. It’s a coroutine, which handles the actual sending of the message to the Discord server; with content as string variable for the message,
xxxxxxxxxx
tts
for text-to-speech control,
xxxxxxxxxx
embeds
for rich content display, and others. The
xxxxxxxxxx
view
parameter accepts inputs instance of View to add interactive components to the message.
This overall process with
xxxxxxxxxx
discord.py
makes the interaction with Discord bots much more engaging and fun. Practices on sending buttons Components with messages will not only enhance your bot’s functionality but also elevate user engagement.In order to fully comprehend the button components in Discord.Py and how to add these to a message, it is essential to first understand what Discord.Py is. Discord.Py is essentially a modern, easy-to-use, feature-rich, and async-ready API written in Python for Discord. It allows you to integrate your Python application, website, or service with Discord’s bespoke features.
Button components are recently introduced elements that allow developers to create interactive buttons on their discord channels. These buttons can enhance the user experience by providing interactive responses for each button click.
In the latest version of Discord.Py (2.0), the library has introduced support for these button components. Therefore, let’s deep dive into understanding how to add button components to a message using Discord.Py.
We start by setting up a bot command that will send an embedded message with a button component when invoked.
xxxxxxxxxx
from discord.ext import commands
import discord
bot = commands.Bot(command_prefix="!")
command() .
async def button(ctx: commands.Context):
embed = discord.Embed(title="My Title", description="My Description")
button = discord.ui.Button(label='Press me!', style=discord.ButtonStyle.blurple)
view = discord.ui.View()
view.add_item(button)
await ctx.send(embed=embed, view=view)
First, we build the Embed object ’embed’ with a title and description of our choice. The magic then happens – we construct a Button instance where we define the content of the button. We define its label and a design style. In this case, the ‘style’ option makes our button have a blue color.
Secondly, we need to create a View object ‘view’ that will serve as a container for our button. We take our interactive item (button) and add it to the view via its ‘add_item()’ method.
Finally, we call the ‘send()’ method from the ‘Context’ class which will broadcast our message along with our view (containing button).
Keep in mind that a standalone button doesn’t do anything upon being clicked. To make it respond to clicks, we need to write a callback inside our button instance. Between the ‘button =’ and ‘view =’ entries, add the following code line:
xxxxxxxxxx
click .
async def click_interact(self, interaction: discord.Interaction):
await interaction.response.send_message('Hello, Discord!')
Above, we use a decorator ‘@button.click’ to designate a coroutine that will be called when the button is clicked. Inside this method, we use the ‘send_message()’ function from the ‘InteractionResponse’ class to send a response on the channel saying ‘Hello, Discord!’.
Once set up correctly, your discord.Py bot should now be able to send proactive messages with interactive button components. Happy coding!
You may want to refer to the official documentation for more insight into working with components in Discord.Py.
Sure! When it comes to setting up your environment for button implementation, specifically for adding button components to a message in Discord.py, be prepared to engage in some Python coding. The Discord API is comprehensive and well-documented, but getting those buttons functioning perfectly may require some initial groundwork.
Prerequisites
Ensure that your development environment has:
– Discord.py installed: this Python library allows you to interact with the Discord API.
– A connected bot on a server: you will need to have an existing bot set up on a Discord server. This doesn’t just mean creating a bot account, but also having it ‘online’ within a server.
Use pip to install the discord package:
xxxxxxxxxx
pip install -U discord.py
Importing Necessary Libraries
First things first, import the necessary libraries. In order to add buttons to a message, you are going to require at least the following:
xxxxxxxxxx
import discord
from discord.ext import commands
Setting Up Your Discord Bot Client
Afterwards, setup your Discord bot client:
xxxxxxxxxx
client = commands.Bot(command_prefix = "!")
This will create a new instance of a bot with the command prefix being “!”. You can change the prefix to anything you’d like.
Create Message With Buttons
Adding button components requires use of the discord UI module, `discord.ui`. The Button component can be found inside this module. Here is a simple example:
xxxxxxxxxx
class Confirm(discord.ui.View):
def __init__(self):
super().__init__()
self.value = None
ui.button(label='Yes', style=discord.ButtonStyle.green) .
async def confirm(self, button: discord.ui.Button, interaction: discord.Interaction):
self.value = True
self.stop()
ui.button(label='No', style=discord.ButtonStyle.grey) .
async def cancel(self, button: discord.ui.Button, interaction: discord.Interaction):
self.value = False
self.stop()
The code above represents two buttons labeled ‘Yes’ and ‘No’. ‘Yes’ button with green color and ‘No’ with grey. The `discord.ui.button` decorator is used to convert the corresponding methods into buttons.
Incorporate them as part of a view into a message. Below the method where your bot sends a message, try using the following lines of code:
xxxxxxxxxx
view = Confirm()
await ctx.send('Are you sure?', view=view)
# wait for the View to stop listening for input...
await view.wait()
if view.value is None:
await ctx.send(f'Timed out.')
elif view.value:
await ctx.send(f'Confirmed.')
else:
await ctx.send(f'Denied.')
In this example, `ctx.send` refers to the context in which the message is sent.
Remember that implementing buttons and other Discord UI features involves managing two-way communication between your bot and users: when they click the button, the bot needs to respond.
Note: While incorporating button components like these in your bot, keep it at the back of your mind to always conform to Discord’s guidelines regarding bots. Misuse or abuse could lead to your bot user token being revoked.Sure, I’d be glad to explain how to add button components to a message through Discord.py.
Discord recently unveiled Message Components, which now feature buttons. The interaction with these embedded buttons are referred everything as “components”. There’s now a `Components` class under the interactions package in discord.py. For your convenience and quick access, you can find all the fascinating new details in the discord.py docs.
First things first, to use the button features using discord.py, you need to have at least discord.py v2.0 installed. Here’s a helpful command line snippet that updates your version to this requirement:
xxxxxxxxxx
pip install git+https://github.com/Rapptz/discord.py
Having sorted that out, let’s dive into what you’re waiting for – incorporating the much sought after Buttons through the discord.py library. The general steps will include:
– Setting up an instance of the `ButtonStyle`
– Using an instance of `Button` with your desired label and ButtonStyle
– Pushing these buttons into an ActionRow
– Finally, sending them off inside the components keyword argument
Here’s an illustrative example:
xxxxxxxxxx
from discord import Button, ActionRow, ButtonStyle
async def button_send(ctx):
row = ActionRow(Button(style=ButtonStyle.red, label="Button Label!"))
await ctx.send("This is a new message with a button!", components=[row])
In the code above, an instance of the `ButtonStyle` class is created with the red style. A `Button` instance is made specifying this particular style and a label. Then the aforementioned button is shoehorned into an `ActionRow`. This row is later included into a `message` through components keyword argument which uses an array.
With this, you’ll be able to send a brand new message that comes attached with a clickable button. Clicking on these buttons sends an interaction event which you can use for further facilitating user-bot communication.
It’s important to note here that while the above example is simple enough, the buttons provided by Discord come in many variants. There’s `Link button`, more color options through `Button Style`, and the option of including `emojis`.
Don’t forget, Buttons are not limited to just new messages but can also be added to previous messages through message editing.
Overall, adding components like buttons adds another dimension to interaction within Discord. It might seem complicated at first glance, but with practice, you’ll get the hang of it. By looking at examples, exploring the official Discord.py documentation for more information about individual classes and methods and experimenting with different options, you’ll be well equipped to utilize the full scope of this exciting update to extend possibilities of Discord.py.Sure, I’d love to! When we’re using the
xxxxxxxxxx
discord.py
library, we know that messages in Discord can have multiple components. These components cover different functions, such as content, embeds and files, reactions, mentions, and more recently, buttons.
Add Button Components To A Message (Discord.Py)
New additions like button components are taking the interaction capabilities of Discord to another level. We generally categorize these components, most notably buttons, into a specific namespace known as the message component context in
xxxxxxxxxx
discord.py
. If we talk about buttons specifically, they come under ActionRow inside the discord module’s codebase.
Before getting into the coding part, I should mention that to utilize this feature of adding buttons, you need to use a fork of discord.py as the original one discontinued further updates before Discord introduced buttons. The most popularly used fork is ‘nextcord’.
Here’s an example on how you can attach button components:
xxxxxxxxxx
from nextcord import Message, Interaction, Button, ButtonStyle, ActionRow
async def send_button_message(ctx):
row_of_buttons = ActionRow(
Button(style=ButtonStyle.green, label='Click Me!')
)
await ctx.send('Hello, world!', components=[row_of_buttons])
event .
async def on_button_click(interaction: Interaction):
if interaction.component.label == 'Click Me!':
await interaction.response.send_message('Button clicked!')
In this initial code snippet, we’ve created a row with a single green button labeled ‘Click Me!’. The
xxxxxxxxxx
send()
method sends the button along with the message.
The subsequent button click event is fired whenever any button attached to the bot’s message is clicked. Depending upon the text present on the clicked button (interaction.component.label), we’re sending back an appropriate response.
For more information, please refer to the [Nextcord API Reference](https://docs.readerbench.com/nextcord/api/nextcord.html) for comprehensive guidance on the Nextcord library.
It’s important to note that the way you interact with these new features might vary slightly based on your particular version of ‘discord.py’ or its forks such as ‘nextcord’ or ‘hikari’.
Be rest assured that, despite some discrepancies, the core idea remains the same – we’ve got additional methods to create interactions within our messages, making our Discord bots more dynamic and engaging!Let’s delve into the step-by-step guide on how to add button components to a message using discord.py, which is a Python library for interacting with Discord’s API.
Firstly, you’ll need to make sure that your version of discord.py supports button interactions. Button interaction was introduced in the discord-interactions version of the library, which can be installed with
xxxxxxxxxx
pip install discord-py-interactions
The following steps outline the process of adding buttons to messages using discord.py:
Create A Button
To create a button, you’ll use the class
xxxxxxxxxx
ButtonStyle
from the discord-py-interactions library. Each button has a style (like primary or danger) and a label. For example:
xxxxxxxxxx
from discord_components import *
button = Button(style=ButtonStyle.blue, label='Press Me!')
Create Message With Button
After creating a button, add it to a message by including it in your bot’s
xxxxxxxxxx
send
method like this:
xxxxxxxxxx
await ctx.send("Hello World!", components=[button])
Handle Button Clicks
When a user clicks the button, the bot needs to listen for this interaction and respond. This is done with the
xxxxxxxxxx
on_button_click
event.
xxxxxxxxxx
event .
async def on_button_click(interaction):
await interaction.respond(content='Button Pressed')
Error Checking
In programming, error handling is critical. It is especially important here because if an error occurs during interaction (e.g., invalid arguments), the message send will fail.
xxxxxxxxxx
event .
async def on_error(event, *args, **kwargs):
print(f'Error on event {event}, {args}, {kwargs}')
Now, combining everything together, you have a simple bot that sends messages with buttons and responds when those buttons are clicked.
Source Code Example:
xxxxxxxxxx
from discord.ext.commands import Bot
from discord_components import ComponentContext, Button, ButtonStyle
bot = Bot(command_prefix="!")
command() .
async def send_button(ctx):
await ctx.send(
"Hello, World!",
components=[
Button(style=ButtonStyle.blue, label="A Blue Button")
]
)
event .
async def on_button_click(res: ComponentContext):
await res.send(content=f'{res.component.label} was clicked!')
event .
async def on_error(event, *args, **kwargs):
print(f'Error on event {event}, {args}, {kwargs}')
bot.run('your-token-goes-here')
As a professional coder, always thoroughly test new features and keep an eye on the community documentation and resources. The official GitHub page of discord.py offers examples and guides which can be very useful. Happy coding!Assuming you’re already familiar with the basic usage of discord.py, let’s delve straight into adding button components to a message.
First thing first, the Discord API recently introduced the concept of Message Components, including buttons. These allow you to create interactive messages which can be presented to your users in an engaging way. In discord.py, these features are enabled by using ActionRow and Button classes.
Let’s start off with addition of buttons to messages:
To add buttons to your text, use `ActionRow` and `Button` classes, part of the discord.py module. According to the official documentation, `ActionRow` is a list component that can hold up to 5 `Button`s or `SelectMenu`s within it.
xxxxxxxxxx
from discord import Button, ActionRow
row_of_buttons = ActionRow(Button(style=ButtonStyle.primary, label='Button 1'),
Button(style=ButtonStyle.danger, label='Button 2'))
await ctx.send('Here are some buttons:', components=[row_of_buttons])
In this example, we created a row containing two buttons with different styles. We have the ‘primary’ style for Button 1 and the ‘danger’ style for Button 2. Then we add this row to our message using the ‘components’ attribute during the send operation.
Let’s highlight styles used in the snippet above. The `ButtonStyle` class in discord.py defines multiple color-coded styles, each representing different level of prominence or type of user interaction. Some of the styles include :
- “primary” corresponds to a blue colored button,
- “secondary” indicates a grey button,
- “success” introduces a green colored button,
- “danger” shows a red button,
- “link” is for button containing URL linking outside of Discord.
xxxxxxxxxx
from discord import ButtonStyle
button_with_link = Button(style=ButtonStyle.link, label='Link Button', url='https://www.google.com')
In this code block, a link button is created using `ButtonStyle.link`. A URL is also provided, so clicking the button would lead a user to the specified web address.
Remember, discord.py has extreme flexibility and provides endless possibilities to moderate your community in a seamless and intuitive manner while providing users with an interesting engagement experience. Experimenting with these structures allows you to build a more dynamic and interesting bot, significantly enhancing its value for your users. I encourage you to check out further reading on button interactions and message component interactions in discord.py on their official API documentation.When it comes to adding button components to a message using Discord.py, there are several common errors that developers often encounter. Below are a few examples and solutions to these problems:
1) Exception Raised: You might encounter an exception being raised such as the command not found error. This may be due to you trying to add a button to a message in a way that Discord.py does not understand. In this case, ensure you are adding the button following the correct format.
Here’s an example of how to correctly implement:
xxxxxxxxxx
from discord_components import *
command() .
async def button(ctx):
await ctx.send(
"Click on the Button",
components=[
[
Button(label="Click me", style=ButtonStyle.green)
]
]
)
On the contrary, attempting to use ‘TextButton’ or ‘ImgButton’, which are not recognized by Discord.py, would raise exceptions.
2) Incompatibility issues: Your version of Discord.py might not support buttons. Remember, support for buttons was introduced from discord-components v2.0.0. You need to upgrade your library if the version doesn’t match.
Install/upgrade with pip:
xxxxxxxxxx
pip install discord-components
3) Lacking permissions: Sometimes the bot user does not have enough permission settings to interact or perform certain commands. For instance, ‘Manage Message’ permission is required for adding buttons, ‘Read Message History’ for getting message responses.
To solve such issues, analyze your bot’s permissions in your server and adjust those as needed.
4) Missing interaction response: If everything seems fine but your buttons don’t seem to react then you’re probably missing an interaction response. When a user clicks a button, webhooks send Interaction payloads to your app for processing. Failing to respond to these within 3 seconds results in the action failing silently without any sort of feedback to the user.
Look at this simple interaction response snippet for reference:
xxxxxxxxxx
event .
async def on_button_click(res: ButtonContext):
await res.respond(content=f"{res.component.label} clicked!")
With the correct response system in place, you should see notification on pressing the button.
These are some of the common pitfalls developers encounter while integrating message buttons to Discord bots using discord.py. Feel free to refer to the official [Discord.py documentation](https://discordpy.readthedocs.io/en/stable/api.html) or community [help forums](https://discordpy.readthedocs.io/en/stable/discussion.html) to find more specific solutions to other error codes or scenarios.*
As an innovative developer, it’s crucial to understand the power of interactive communication tools like Discord. Adding the button component to a Discord message using Discord.py brings your bot to life by creating interactive experiences for users. The Python package `discord_buttons_plugin` significantly simplifies this process.
– First, let’s start with installation:
xxxxxxxxxx
pip install discord-buttons-plugin
Once installed, we can import it into our bot code:
xxxxxxxxxx
import discord
from discord_buttons_plugin import *
bot = Bot(command_prefix="!")
command() .
async def button(ctx):
buttons = [
Button(style=ButtonStyle.blue, label="Blue"),
Button(style=ButtonStyle.red, label="Red"),
Button(style=ButtonStyle.grey, label="Grey"),
]
await ctx.send("Buttons:", buttons=buttons)
bot.run("token")
Let’s dissect the code snippet above:
– We import necessary tools; namely discord and discord_buttons_plugin.
– Next, we reference a bot command which is named exactly: button.
– The list ‘buttons’ demonstrates examples of three different types of buttons available. Each has its own unique style (color) and label (name).
– In the end, the bot sends a message that is accompanied by these defined buttons with
xxxxxxxxxx
ctx.send()
.
Lastly, we run our bot with token, replacing “token” with your actual bot token. For security reasons, never share or upload your token publicly as it provides access to your bot.
This interactive conversation capability enhances user engagement and interactions significantly. Advanced user interaction, appeals to various learning styles and personal preferences, thus potentially growing your bot’s user base.
Check out this Github Repository for more information and usage examples on Discord Buttons Plugin.
Coding is not just about churning out lines of code, it’s about making things easier, better, and more engaging for the end-user. Make sure to follow good SEO practices when adding content to promote your Discord bot so it gets seen by potential users – use relevant keywords and phrases, employ a natural and readable style, and provide valuable, informative content. This will ensure your work is searchable, appealing, relevant, and ultimately successful! Keep iterating, keep innovating!