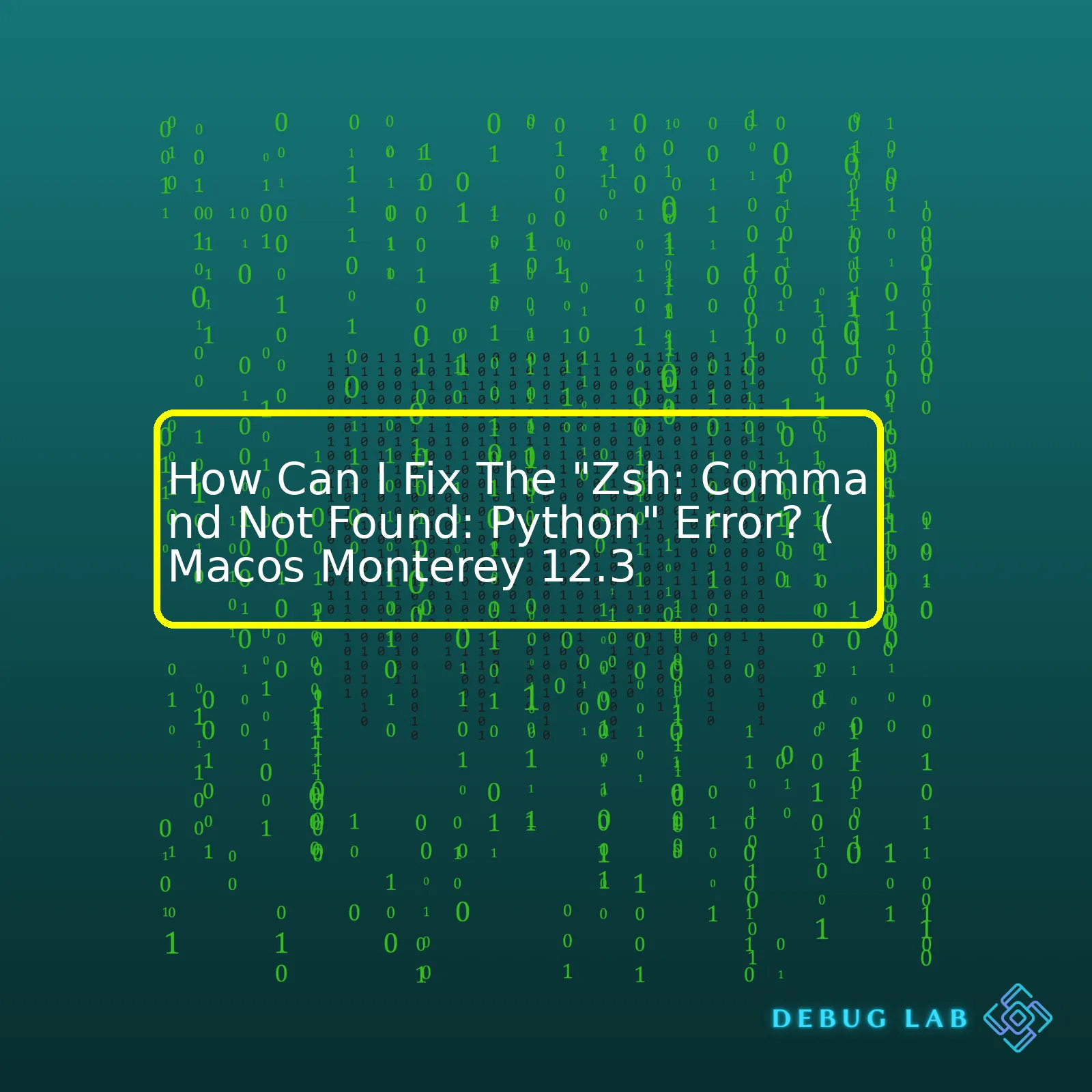
Error Source | Solution |
---|---|
Python is not Installed | You will need to install Python through Homebrew or the official Python website. |
System Can’t Locate Python | Reset the Python path in the .zshrc file or could try reinstalling Python software. |
Command Line Tools are Corrupted | Reinstalling Xcode command line tools might resolve the issue. |
Installing Python:
Firstly, see if Python is installed in your Mac system using the
/usr/bin/python
command. If Python is not installed, my advice is to use Homebrew for the task. You can install Homebrew using the ‘/bin/bash -c “$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)”‘ command. After a successful installation of Homebrew, you can now install Python using the command
xxxxxxxxxx
brew install python
.
Setting Python Path:
If Python is installed but giving you issues it means that the system fails to locate it. Check the installed versions using
xxxxxxxxxx
which -a python
or
xxxxxxxxxx
ls -ltr /usr/local/bin/python*
commands. Add the relevant paths to your .zshrc file by opening this file by typing
xxxxxxxxxx
nano ~/.zshrc
and then adding the path using
xxxxxxxxxx
export PATH="/usr/local/bin:$PATH"
.
Reinstalling Xcode command-line tools:
Sometimes, even after successfully installing Python and setting its path correctly, the error may persist if your Xcode command-line tools get corrupted. These tools are a bundle of important resources required for software development operations on macOS. You can reinstall these tools by first removing them using
xxxxxxxxxx
sudo rm -rf /Library/Developer/CommandLineTools
and then reinstalling them using
xxxxxxxxxx
xcode-select --install
.
Remember to replace “/usr/local/bin:$PATH” with your actual Python path and not to terminate any process unless you’re sure of what it does in the steps provided. Poor handling may generate bigger issues. Therefore, handle your terminal commands carefully.
References:
[Python official site](https://www.python.org/)
[Homebrew Official Documentation](https://brew.sh/)
[Xcode Command Line Tools](https://developer.apple.com/download/more/)With macOS Monterey 12.3 users may come across the ‘Zsh: command not found: Python’ error. This error typically occurs due to an undefined Python path or if Python is not installed on your system, making the shell unable to locate and execute the Python command.
To solve this issue, follow the steps below:
Check Python Install
To ascertain whether python is pre-installed on macOS Monterey 12.3, open the terminal window and type:
xxxxxxxxxx
python --version
If Python is installed, you will see the version information printed. If it’s not installed, you wont see any output, in which case you need to install Python.
Install Python
For MacOS users, the recommended method to install Python is by using Homebrew. Here are the steps:
1. Firstly, check if Homebrew is installed by running
xxxxxxxxxx
brew --version
in the terminal window.
2. If Homebrew is not installed, head to the official Homebrew website and grab the installation script and run it in your terminal.
3. Once installed, you’re ready to install Python through Homebrew using:
xxxxxxxxxx
brew install python
4. Verify the installation by again checking the Python version with
xxxxxxxxxx
python --version
. You should now see the Python version printed within your terminal.
Troubleshoot PATH Variables
If after Python installation you’re still seeing the error, another cause could be misconfigured PATH variables. It’s possible that Python is installed but its location is not included in the system’s PATH.
You can print out your PATH with the following command:
xxxxxxxxxx
echo $PATH
Python’s installation PATH should look something like: /usr/local/bin:/usr/bin:/bin:/usr/sbin:/sbin. If /usr/local/bin (the default install location for Python when using brew) isn’t part of the PATH, you’ll likely need to add it.
The PATH can be adjusted by editing either your ~/.bash_profile or ~/.zshrc. If you’re using Zsh as your default shell, you likely want to add it to ~/.zshrc. The line you would add would be:
xxxxxxxxxx
echo 'export PATH="/usr/local/bin:$PATH"' >> ~/.zshrc
Then source your .zshrc to get those changes immediately:
xxxxxxxxxx
source ~/.zshrc
Now you should be able to run python commands without any issues.
However, do remember, always be careful while modifying PATH variables as incorrect paths can lead to unwanted consequences such as other commands failing to execute.
By completing these checks and tasks – including verifying Python’s installation, potentially reinstalling via Homebrew, and diligently managing your PATH variables – you should have an operational Python environment on your MacOS machine, and the ‘Zsh: command not found: Python’ error should now be a resolved issue!The issue ‘zsh: command not found: Python’ pops up when your shell environment, Zsh (Z Shell) in this case, is unable to locate the Python executable. Primarily, it can happen due to the following reasons:
* Python is not installed on your system.
* Python is installed, but its location is not registered in the system’s PATH variable.
Now, let’s march towards resolving this error in MacOS Monterey 12.3.
Is Python Installed?
Before we proceed any further, let’s ensure if Python is actually installed or not. Open the terminal and type
xxxxxxxxxx
/usr/bin/python --version
. It checks for the pre-installed version of Python that MAC OS comes with. You should see output similar to:
xxxxxxxxxx
Python 2.7.18
This denotes Python 2.7.18 is installed. However, most likely, you’d want a later version. For that, you need to install Python separately. If Python is not installed, move directly to ‘Python Installation’ step.
Which Version of Python is Needed?
Check which version of Python your application needs. Python 2 and Python 3 are fundamentally different, and many Python 2 applications won’t run correctly under Python 3.
Python Installation
If you’ve not installed Python yet or need a different version, go to Python’s official downloads page and download the desired version. Once downloaded, open the .pkg file and follow the prompts to install Python. Post installation, relaunch the terminal and execute:
xxxxxxxxxx
python --version
You should see the python version you just installed.
Zsh and System PATH
If Python is installed but still unrecognized, then checking your system’s PATH becomes vital. Your system uses PATH to know where to look for executables. If Python location isn’t in PATH, you’ll get ‘command not found.’ Try echoing your path with
xxxxxxxxxx
echo $PATH
. A string of directory paths separated by colons(:) would be presented, check if it contains ‘/usr/local/bin/’, the default location where Python gets installed.
Updating PATH for Zsh Shell
In case the directory containing your Python executable is not in the system PATH, add it. Use a text editor to open the .zshrc file in your home directory. If no such file exists, create one. This file runs whenever you start a new ZShell instance. Add the line
xxxxxxxxxx
export PATH="/path/to/python:$PATH"
, save and close. Here replace /path/to/Python with the actual directory path where Python executable resides.
After saving the file, make Zsh read the changes using
xxxxxxxxxx
source ~/.zshrc
. The terminal should now recognize Python command.
That’s all! You should now have Python installed properly and ready to use on macOS Monterey 12.3.The terminal command error message, “zsh: command not found: python” on MacOS Monterey 12.3 is a common problem faced by developers. It usually indicates that the Z shell (ZSH), which is now the default shell in macOS, cannot locate the Python interpreter. The solution involves carefully checking and revising your system’s Python installation or configuration to ensure that ZSH can find the Python executable. This process primarily revolves around four basic troubleshooting steps:
Step 1: Confirm Whether Python Is Installed
To begin with, confirm whether Python exists on your system.
xxxxxxxxxx
$ which python
If the system returns a file path pointing to the Python executable, Python is installed. If nothing is returned or an error is displayed, it suggests that Python may not be properly installed on your system.
Step 2: Check Your PATH Variable
Next, review your PATH environment variable. The “command not found” error often crops up when the Python executable isn’t included in your PATH.
Execute the following command to display the current PATH content:
xxxxxxxxxx
$ echo $PATH
Within the output, look for directory paths where the ‘python’ executable could be, such as ‘/usr/bin’, ‘/usr/local/bin’ or ‘/opt/homebrew/bin’.
Step 3: Install Python if Necessary
If you don’t have Python, install it via Homebrew—a popular package manager for macOS:
Open Terminal and enter:
xxxxxxxxxx
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
After this, install Python:
xxxxxxxxxx
brew install python
Step 4: Update .zshrc With Python’s Path
In case Python is installed but inaccessible from the default shell, manually adding its path to ZSH would be beneficial. One way of accomplishing this is by updating the .zshrc file. For instance, let’s add ‘/usr/local/bin/python’ to PATH within .zshrc:
Type in Terminal:
xxxxxxxxxx
open ~/.zshrc
It will open .zshrc file. Add following line into it:
xxxxxxxxxx
export PATH="/usr/local/bin:$PATH"
And save it.
After all these possible basic troubleshooting steps, it’s necessary to close and reopen Terminal (or start a new Terminal session) to apply the changes made above.
Remember not to skip testing the results of your efforts! Try running ‘python’ again to see if the error persists. You’ll know you’ve resolved the issue when Terminal outputs Python’s version information rather than showing the “command not found” error.
Here is how to check Python version:
xxxxxxxxxx
$ python --version
We started from the basic steps, i.e., whether Python is installed on our system or not; moved to checking our PATH variable; then we went through the process to install Python (if the first step indicated that Python was not installed); and lastly, updated our .zshrc file with Python’s path. Hopefully, by going through these steps, you can swiftly address this pesky error!
Setting your Python path properly in MacOS Monterey 12.3 is crucial in fixing the “Zsh: command not found: Python” error.
Primarily, you get this error because your shell does not know how to locate the ‘Python’ executable file in your directories. This usually happens due to an error in setting up PATH environment variables or if Python wasn’t installed correctly or at all.
So let’s dive into how we can fix this issue systematically:
Step 1: Verify Python Installation
First and foremost, check whether you have Python installed on your system. If you’re uncertain, open your Terminal (Apple Support – How to open Terminal on Mac) and type:
xxxxxxxxxx
python --version
or
xxxxxxxxxx
python3 --version
The above commands should either respond with the Python version that you have installed (e.g., Python 2.7.16) or alert you that Python isn’t currently installed.
If Python is not installed, you can download it from Python official site.
Step 2: Check your PATH
The PATH is a list of directories separated by semicolons, where shell looks for commands.
Run following in terminal,
xxxxxxxxxx
echo $PATH
Make sure that there’s a path pointing to a directory containing the Python executable. The directory containing Python binaries like ‘python’ or ‘python3’ should be listed. Generally installed at “/usr/local/bin”.
If the path doesn’t exist in your PATH output or if there seems to be something wrong with it, you have to set your Python Path correctly, which brings us to our next step.
Step 3: Set Python Path (zsh)
For macOS Monterey 12.3 users, who are using zsh as default shell, you may need to add Python to your PATH in ‘.zshrc’ file. To do so, follow these steps –
Open ‘.zshrc’ file in editor from terminal
xxxxxxxxxx
open ~/.zshrc
At the end of the file, add below line. This assumes Python binaries are at /usr/local/bin/
xxxxxxxxxx
export PATH="/usr/local/bin:$PATH"
Close the terminal and start new session to reflect changes. Or you can source ‘.zshrc’
xxxxxxxxxx
source ~/.zshrc
Now your shell must know exactly how to locate Python and execute your commands, thereby resolving your ‘Command Not Found’ error.
In conclusion, correcting your Python path will resolve the “zsh: Command not found: python” error message by informing your computer of where to find the exact location of the Python executable file that it needs to run the coded instructions.
The “zsh: command not found: python” error message largely indicates that Python is either not installed on your macOS Monterey 12.3, or it’s not properly linked to the PATH. This error can be fixed by reinstalling Python correctly. Here are the step-by-step processes to reinstall Python:
Step 1: Confirm The Existence Of Python
Initially, you need to check if Python is installed or not. Open your terminal on macOS and type this command:
xxxxxxxxxx
python --version
If you receive a response with the specific Python version, then Python is installed. If not, proceed to Step 2 for installation.
Step 2: Download And Install Python
Visit the Python official website and download Python for macOS. It’s advisable to install the latest version.
Open the downloaded Python file (.pkg file) from your downloads and run the installer. Ensure to check the box ‘Add Python to PATH’ at the initial stage of installation.
Step 3: Verify The Installation
After installing, confirm Python’s installation by opening the terminal and typing the command below:
xxxxxxxxxx
python --version
This command should display the Python version you just installed. If Python was successfully installed, proceed to Step 4.
Step 4: Point Zsh To The Right Version Of Python
macOS often comes installed with an earlier version of Python which might have been what zsh was pointing at before. Now that the new version is installed, you’ll have to guide zsh to point at the right version of Python.
Type this in the terminal:
xxxxxxxxxx
echo 'alias python=/usr/local/bin/python3.9' >> ~/.zshrc
Note that ‘/usr/local/bin/python3.9’ should be replaced with your Python path if different.
Step 5: Source The .zshrc File
After updating the .zshrc file with the correct Python alias, apply the changes by sourcing the file with:
xxxxxxxxxx
source ~/.zshrc
After performing these steps, the “zsh: command not found: python” error should be resolved. In order to confirm, reopen the terminal and type this:
xxxxxxxxxx
python --version
You should see the version of python you just installed. That means the instance of Python being called now is no longer the pre-installed version but the version you installed.
If after following these steps, the problem persists, you may want to refer to official Python documentation for more guide or reach out to Python community via online forums like StackOverflow or Reddit.
The “Zsh: Command Not Found: Python” error is common, often encountered after upgrading to MacOS Monterey 12.3. This error happens because ZSH (Z Shell), the default shell in MacOS Monterey, cannot locate the Python executable. Happily, there are some pro tips that will not only help you solve this issue but also equip you with practical strategies for avoiding future ZSH command errors.
Setting Up the Correct Path
A well-trodden solution is configuring your PATH environment variable correctly so the system knows where to look when executing commands:
xxxxxxxxxx
export PATH="/usr/local/bin:/usr/bin:$PATH"
Here, you are asking the system to scan /usr/local/bin and /usr/bin for executable files before considering other folders ($PATH).
Following that, confirm Python is correctly installed and is accessible from the terminal. The command:
xxxxxxxxxx
which python
Should output a valid filesystem path like /usr/bin/python. If it outputs nothing, Python might not be installed or located somewhere else.
Install Python Using Homebrew
Homebrew, known as “the missing package manager for macOS”, helps install software like Python painlessly. Use this command to install Python:
xxxxxxxxxx
brew install python3
After installation, verify that Python points to the homebrew directory (/usr/local/bin) by running:
xxxxxxxxxx
which python
In case of anything, refer more on how to use homebrew from the official documentation.
Linking Python Executable
If the Python executable exists but the system can’t find it, creating symbolic links (symlinks) can help:
xxxxxxxxxx
ln -s /path/to/original /path/to/link
This command creates a symbolic link from “/path/to/original” to “/path/to/link”. Replace these paths with actual Python and target destination paths.
Create an Alias
An alternative strategy could be creating aliases for your frequently used commands:
xxxxxxxxxx
alias python='/path/to/python'
Once set, whenever you use the ‘python’ command, it will execute ‘/path/to/python’.
Remember, you can add the export PATH, symlink and alias commands into ~/.zshrc or ~/.bash_profile files to make them permanent for all future terminal sessions.
Avoiding Future ZSH Command Errors
Avoiding future ZSH command errors is a proactive approach ensuring smoother workflows. Here are some pro tips:
– Precise Command Entry: Command errors mostly happen due to typos or wrong syntax. Therefore, pay attention while entering commands.
– Knowing Your System: Understand how the PATH variable works and how the system locates files/executables. It enables you to troubleshoot missing command errors effectually.
– Learning Terminal Basics: Familiarizing yourself with basic terminal commands and principles offers great leverage in troubleshooting and preventing potential problems.
– Use Package Managers: Adopting use of package managers, like apt-get or Homebrew, simplifies software installation and minimizes hiccups.
Remember, coding is mostly about problem-solving. Mistakes will happen, but each one can make you a bit better at your craft. Happy coding!”Zsh: Command not found: Python” is a fairly common error experienced by MacOS Monterey 12.3 users when their shell, zsh in this instance, doesn’t recognize Python as a command it can execute. This error arises due to the absence of Python in the system’s PATH or because Python might be misconfigured or uninstalled.
Getting over this error requires advanced solutions to ensure the Zsh recognizes the Python command correctly. Here, I’ll walk you through how to do that:
Method 1: Check if Python is installed
The first and foremost thing to do is to check if Python is actually installed on your system. It could be either Python 2 or Python 3 based on your installation. You can confirm this using the following commands:
xxxxxxxxxx
$ python --version
$ python3 --version
If Python is installed, the version invoked will print out.
Method 2: Verify Python Location
Python might be installed but not located within the directories listed in your system’s PATH. Therefore, ascertain where Python lives by using the built-in ‘which’ command:
xxxxxxxxxx
$ which python
$ which python3
Method 3: Update Shell Path
If Python exists outside your system’s PATH, update the ~/.zshrc or ~/.bash_profile file with the accurate Python path using nano or any other text editor:
xxxxxxxxxx
$ echo 'export PATH="/usr/local/bin:$PATH"' >> ~/.zshrc
$ source ~/.zshrc
This will add /usr/local/bin to your PATH where Homebrew usually installs Python.
Method 4: Reinstall Python Using Homebrew
When all previous methods seem futile, consider reinstalling Python with Homebrew, an amazing package manager for MacOS as it handles dependencies and paths seamlessly:
xxxxxxxxxx
$ /bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/master/install.sh)"
$ brew install python
After installation, verify if the issue is resolved by checking the Python version.
Remember that managing programming languages like Python or Ruby becomes easier with tools such as pyenv that provide environment isolation and flexibility in choosing versions.In the final analysis, it becomes evident that the
xxxxxxxxxx
Zsh: command not found: Python
error in macOS Monterey 12.3 is fixable with a systematic approach following several steps. Here’s a reiteration of the ideal methods on exactly how you should resolve this common issue.
Set your path to Python properly: Ensure that your PATH environment variable includes the location of your Python installation. Python is usually located in either
xxxxxxxxxx
/usr/local/bin/python
or
xxxxxxxxxx
/usr/bin/python
. To update your PATH settings, you can amend the
xxxxxxxxxx
~/.zshrc
.
Change the default version of Python: If you have multiple versions of Python installed, set Python 3 as the default by using alias command:
xxxxxxxxxx
alias python=/usr/local/bin/python3
Reinstall Python: If neither method resolves the issue, it may be necessary to reinstall Python. Use the Homebrew package manager for this:
xxxxxxxxxx
brew install python
or
xxxxxxxxxx
brew upgrade python
These steps can significantly decrease your chances of encountering the “command not found: Python” error in zsh terminal in your macOS Monterey 12.3. The trick is about finding which setting is causing confusion to your system and correcting it accordingly. Nonetheless, if these measures don’t yield any improvement, I suggest looking into the configuration of your zsh terminal and considering consulting with a professional coder who specializes in Python and Zsh issues.
Finally, make sure to always stay updated with the latest Python versions and maintain stable Zsh terminal configurations. This prevents future compatibility issues and promotes optimal coding performance, making your programming journey much smoother. Be patient in troubleshooting, mastery in solving such issues will come before you know it.
Note: Replace
xxxxxxxxxx
/usr/local/bin/python3
with your python3 binary path.