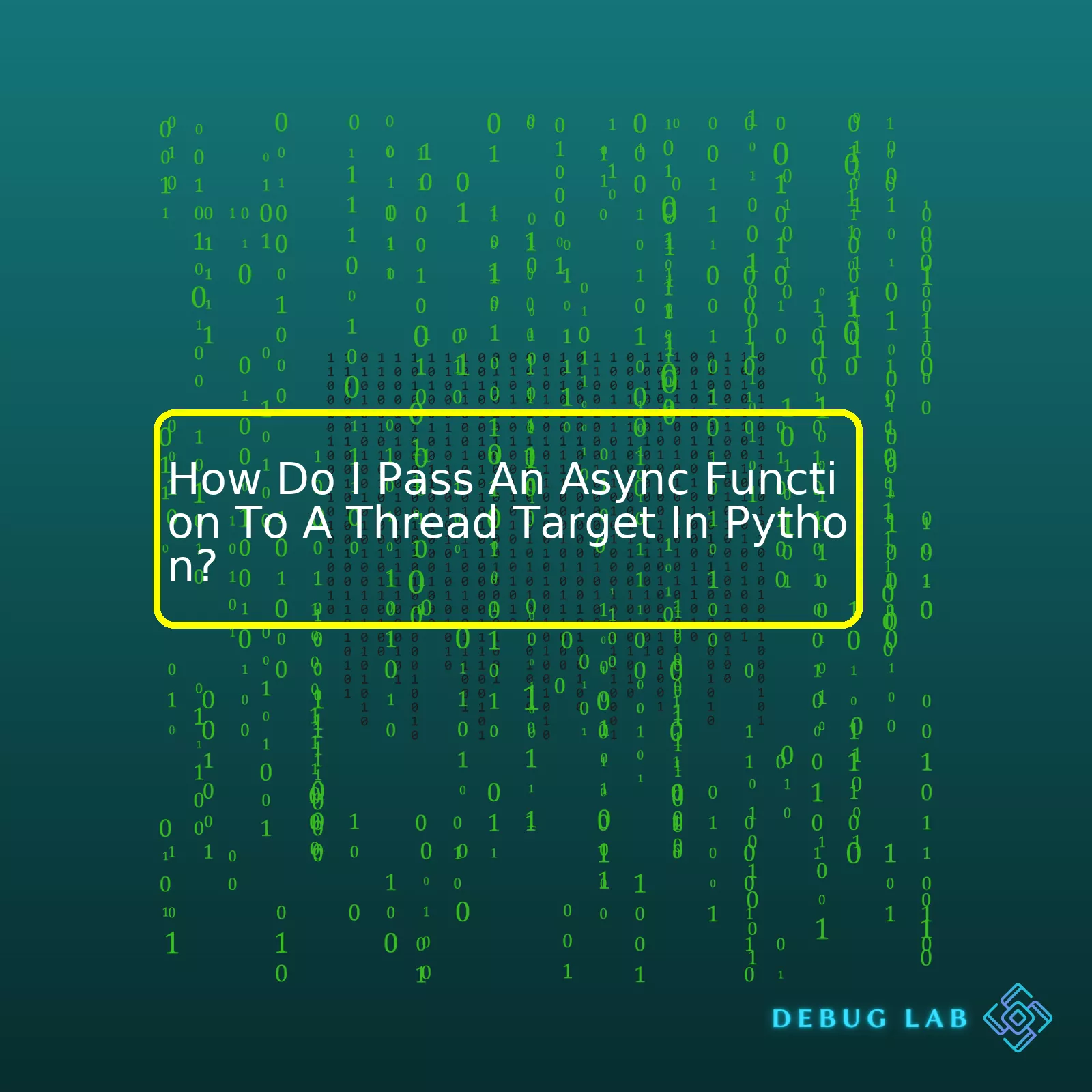
Passing an async function to thread target is essentially bringing these two techniques together. The solution lies in closing the loop in the second thread and then running the coroutine from there. You can generate this through Python’s concurrent.futures module, which provides a high-level interface for asynchronously executing callables.
Here’s an example:
import asyncio
import concurrent.futures
import time
async def async_function():
print('async function start')
await asyncio.sleep(1)
print('async function end')
def thread_function(loop):
asyncio.set_event_loop(loop)
loop.run_until_complete(async_function())
executor = concurrent.futures.ThreadPoolExecutor(max_workers=3)
loop = asyncio.new_event_loop()
executor.submit(thread_function, loop)
time.sleep(2) # ensure the async task has time to complete
executor.shutdown()
This way, we start off by creating a new event loop which we pass to our threaded function. Then, inside the threaded function, we designate the new loop as the event loop for the current context, and run the async function on it until it completes. It’s important to note to give enough time for the async task to complete before shutting down the executor.
Now, below is the summary table for this concept:
Concepts | Description |
---|---|
Asynchronous Functions | Part of the asyncio library introduced in Python3.4 primarily for writing single-threaded concurrent code. |
Threading | A technique for decoupling tasks which are not sequentially dependent. Threads share the same memory space. |
Passing async function to a thread target | This integrates both asynchronous and threading techniques where you close the loop in the second thread and then running the coroutine there. |
Remember that understanding how to pass an async function to a thread target in Python ties concurrency and multithreading aspects of the programming language into a neat package. This broadens your options in creating high-performing applications.The async functionality comes in pretty handy when dealing with IO-bound operations in Python where code has to wait for resources or responses from other systems. It allows you to write asynchronous code in a synchronous manner. When API requests or database operations are waiting to complete, instead of blocking the entire execution of the code,
xxxxxxxxxx
async
enables us to run multiple tasks virtually at the same time. This greatly increases system efficiency and resource use.
To pass an async function to a thread target in Python, it’s important first to note that asyncio operates on an event loop whereas threading involves parallel work of different threads. The challenge in combining these two is about knowing that asyncio runs the event loop in a single thread, and it schedules coroutines onto this loop as required.
Let’s consider the following async function:
xxxxxxxxxx
import asyncio
async def my_async_func():
await asyncio.sleep(1)
print('Hello from async function')
There seems no direct method to pass this async function to a thread because threads don’t recognize
xxxxxxxxxx
async
functions. They don’t know how to handle coroutines. So to combine threading and asyncio, we need to do some extra steps.
The best way around is actually to create a new event loop in the target thread and then run the routine on this loop. Here’s how it can be done:
xxxxxxxxxx
import asyncio
import threading
async def my_async_func():
await asyncio.sleep(1)
print('Hello from async function')
def worker():
new_event_loop = asyncio.new_event_loop()
asyncio.set_event_loop(new_event_loop)
new_event_loop.run_until_complete(my_async_func())
target_thread = threading.Thread(target=worker)
target_thread.start()
target_thread.join()
Here, we’re:
– Creating a new event-loop using
xxxxxxxxxx
asyncio.new_event_loop()
– Setting that as the current event-loop using
xxxxxxxxxx
asyncio.set_event_loop(new_event_loop)
– Finally, use
xxxxxxxxxx
run_until_complete()
to run the coroutine `my_async_func` on this loop.
You may have heard about daemon threads. By marking the thread `target_thread` as `daemon`, you might expect the program to exit immediately after main thread completion. However, unlike regular Python functions, `async` functions contain
xxxxxxxxxx
await
within them, suspending the containing coroutine for an IO-bound operation’s response. Hence, even if `daemon` is set to True, your Python script won’t terminate until all the threads are completely executed.
Take into account the aforementioned information while passing an async function to a thread target in Python. Remember, Python’s asyncio library is undergoing changes, and certain asynchronous patterns may change significantly in the future versions, so always keep your knowledge up-to-date.Perhaps one of the best ways to understand the thread target concept in Python is by understanding how async functions are passed to a thread target. The beauty of multi-threading lies in its ability to run code in parallel, thus significantly improving the program’s efficiency, particularly when working on CPU-intensive tasks. Before we dive into threading with asynchronous functions in Python, let’s briefly touch on key concepts; threading and async functions.
Thread in Python
A thread in Python is a separate flow of execution. Information can be shared between threads, making it an effective way to pass data between functions – or in this case, using one function as an argument for another (often referred to as a callback).
Async Functions
Async functions, short for asynchronous functions, is a paradigm used to manage long-duration tasks that would otherwise block the application from processing other tasks. Async functions mitigate against blocking behavior by creating an illusion of concurrency through using either callbacks, promises, or async and await.
Now, let’s combine these two concepts – how do you pass an async function to a thread target in Python?
In Python, the typical syntax for initiating a new thread would look something like this:
xxxxxxxxxx
import threading
def some_function():
# some task
thread = threading.Thread(target=some_function)
thread.start()
But what if we have an asynchronous function and we want to use it as our thread target? Here’s an example async function:
xxxxxxxxxx
async def async_func():
# some task
Passing this function to the thread target may seem straightforward, but there’s a twist. Normal function calls are blocking. This means they complete their execution before yielding control back to the caller, but async functions are designed to be non-blocking, meaning they are able to yield control back to the caller without having completed.
To successfully pass an async function to a threading target, we would need to create an event loop in the targeted function, like so:
xxxxxxxxxx
import threading
import asyncio
async def async_func():
# perform some async operation
def thread_target(loop):
asyncio.set_event_loop(loop)
asyncio.run(async_func())
def main_function():
loop = asyncio.new_event_loop()
thread = threading.Thread(target=thread_target, args=(loop,))
thread.start()
# perform other tasks concurrently here
thread.join()
if __name__=="__main__":
main_function()
In the above script, thread_target takes an event loop object as an argument and sets it as the current event loop used by this thread. After setting the event loop, it runs our ‘async_func’. We can then safely start our thread knowing that our async function will correctly run in a non-blocking fashion while taking advantage of threading.
I should note that while this setup will allow you to execute an async function within a new thread, threading and async IO serve different purposes are usually not mixed. Threading allows multiple parts of your program to run concurrently (which is useful when each part is waiting on a different resource such as disk, network, cpu, etc), whereas async IO specifically helps you deal with high-latency, typically network-based operations without blocking.
References:
1. Python Threading Library Documentation
2. Python asyncio Library Documentation
It’s critical to have a clear understanding of both concepts and how they fit into asynchronous programming. Even though it might appear a bit overwhelming at first, mastering these paradigms would certainly catapult your Python skills to a whole new level.Having a firm grip on concepts relating to multithreading and asynchronous programming is one surefire way to write efficient Python applications. According to the Python 3 official documentation, you have at your disposal tools such as the ThreadPoolExecutor or ProcessPoolExecutor classes housed within the `concurrent.futures` module to manage a pool of worker threads or processes respectfully.
But a common question bereaves many when threading intersects with async functions in Python: How do you pass an async function to a thread target? It’s vital to remember that threading is designed for I/O-bound tasks, while async programming aims to manage multiple tasks simultaneously-especially when waiting for other tasks to complete.
For starters, this code snippet demonstrates creating a new thread using the threading.Thread class with a given target function:
xxxxxxxxxx
import threading
def sync_func():
print("This is a synchronous function")
task = threading.Thread(target=sync_func)
task.start()
The execution begins with the creation of a new Python Thread by invoking the `start` method on the `threading.Thread` instance. The specified `target` is the function that’ll be called in the context of the new thread.
However, problems arise when we substitute a regular function with a coroutine function (or simply, async function) because these functions are meant to be run within an event loop courtesy of the asyncio library. So, if you unilaterally make a change as shown below…
xxxxxxxxxx
import threading
import asyncio
async def async_func():
print("This is an async function")
task = threading.Thread(target=async_func)
task.start()
…execution culminates into a rather unwelcome SyntaxError! This happens due to the fact that an async function doesn’t implicitly start running without an event loop-oscillating alongside other tasks on a turn-by-turn basis to give an illusion of concurrency happening.
To successfully schedule an Asyncio coroutine from a different Python thread, you must establish a new event loop inside the separate thread just like the code snippet below:
xxxxxxxxxx
import threading
import asyncio
async def async_func():
print("This is an async function")
def worker(async_func):
loop = asyncio.new_event_loop()
asyncio.set_event_loop(loop)
loop.run_until_complete(async_func)
loop.close()
task = threading.Thread(target=worker, args=(async_func(), ))
task.start()
After spinning up a new event loop using the `asyncio.new_event_loop` and subsequently setting it as the current event loop in the context of the current OS thread via the `asyncio.set_event_loop`, the `loop.run_until_complete` is called to execute the async_func() until completion.
Although working around async and threading can appear cumbersome initially, the knowledge you garner will propel you further on your journey to mastering Python concurrency-the underlying concept behind most high-performance Python applications. Navigate carefully through the concurrency model jungles though as they’re fraught with potential issues such as synchronization and resource contention, as wisely recommended by Guido Rossum, the creator of Python himself.When working with Python, running asynchronous functions on a separate thread can be a delicate task. This is because Python’s standard library doesn’t directly support passing async functions as a thread’s target function. But don’t worry! By using concurrent features from the asyncio package, such as event loops and tasks, you’ll implement this functionality.
To perform concurrent operations asynchronously, Python provides built-in libraries – threading and asyncio. In our case here, you’d want to pass an async function (also known as coroutine) to a Thread instance. However, threading package does not inherently support coroutines, because it uses system-level threads that aren’t designed for async I/O operations.
So, let’s use asyncio to create an event loop within the run_in_thread function, which will start a new system-level thread:
xxxxxxxxxx
import asyncio
from threading import Thread
async def my_async_function():
# Place your async code here
print("Asynchronous function is running...")
def run_in_thread(async_func):
loop = asyncio.new_event_loop()
task = loop.create_task(async_func)
loop.run_until_complete(task)
my_thread = Thread(target=run_in_thread, args=(my_async_function(),))
my_thread.start()
my_thread.join()
In this code snippet:
• We define an async function, my_async_function.
• The function, run_in_thread, sets a new event loop, submits the async function to the event loop, and then runs tasks on the event loop until complete.
• We provide run_in_thread as the target function to the thread initializer and pass my_async_function() as an argument to the target function.
• my_thread.start() begins the execution of the thread, and my_thread.join() ensures the termination of the thread before the main thread finishes.
It’s critical to note that all IO-bound operations should be non-blocking within async functions. It processes IO-bound operations alongside computational tasks, thus eliminating waiting times observed with blocking operations. So, make sure to utilise asyncio’s non-blocking counterparts for any IO-bound operation when working with async functions.
This method applies not just to single-thread scenarios but also to multi-thread scenarios where multiple asynchronous operations need to be performed parallelly in different threads. Just remember that threading in Python has its limitations. If your primary concern is CPU-bound tasks rather than IO-bound tasks, consider using multiprocessing instead for native parallelism to overcome Python’s Global Interpreter Lock mechanisms.
Remember- if you’re planning to use asyncio libraries with threads, it is recommended that each thread has only one event loop running at any given time.
Conclusively, the combination of asyncio with threading allows you to leverage both asynchronous programming paradigms and Python’s concurrent programming capabilities. The approach offers excellent utility when dealing with a mix of CPU-bound and IO-bound tasks or when you have to interface with code demanding explicit coroutines or callbacks.To pass an async function to a thread target in Python, you will first need to understand both how threading works and how async functions behave. Threading is a processing feature that allows for concurrency when executing certain tasks. On the other hand, async functions in Python form part of the asyncio library – this library is used for writing single-threaded concurrent code using coroutines, multiplexing I/O access over sockets and other resources, running network clients and servers, and other related primitives.
In most cases, an async function needs to be wrapped with its event loop, then directly called or run as a task within the same thread. However, passing an async function directly to a threading module might cause runtime unexpected errors because threads are not aware of Python asynchronous functions.
Python’s AsyncIO
Asyncio is a standard Python library used for writing single-threaded concurrent code via coroutines while concurrently handling many connections. It was designed to use syntax closely resembling that of synchronous coding, thus making it simpler and more straightforward to write asynchronous code.
Below is a typical example of an async function:
xxxxxxxxxx
import asyncio
async def async_function():
print('start')
await asyncio.sleep(1)
print('end')
asyncio.run(async_function())
The key part about asyncio is that all async functions must be called from other async functions. If you try to call an async function from a regular function, it won’t do anything.
Running Async Functions in Threads
Threads can be created in Python by using the built-in threading module. A new thread is started by creating an instance of the Thread class and calling its start() method. Here’s an example:
xxxxxxxxxx
import threading
def my_function():
print('Hello World!')
thread = threading.Thread(target=my_function)
thread.start()
thread.join()
Note that the name of the function my_function has been passed as the target argument to the Thread constructor, without parentheses. This tells Python to execute the function in a new thread.
Bringing it Together
In order to pass an async function to a thread target in Python, you’ll have to embed the coroutine in the thread’s run method.
Let me illustrate with an example,
xxxxxxxxxx
import threading
import asyncio
class MyThread(threading.Thread):
def __init__(self, loop, *args, **kwargs):
self.loop = loop
super().__init__(*args, **kwargs)
def run(self):
self.loop.run_until_complete(self._target(*self._args, **self._kwargs))
async def async_function():
print('start')
await asyncio.sleep(1)
print('end')
loop = asyncio.get_event_loop()
thread = MyThread(loop, target=async_function)
thread.start()
thread.join()
print('finished')
In the example above, we defined a MyThread class which inherits from threading.Thread. We gave it an event loop, which knows how to handle and run asyncio functions. Then, we run the intended async function (async_function) in the context of the event loop.
This way, your async function is run concurrently along with the rest of your synchronous Python code. Keep in mind that while this code will work, Python’s async and await syntax can provide a beautiful, native feeling API around handling these sorts of operations. They are designed to make handling such operations cleaner and easier to reason about.
With this practical example, you should be able to pass an async function to a thread target in python in an elegant and effective manner. Do make sure to test the changes you make to ensure they work as expected. Happy coding!
When you want to pass an async function to a thread target in python, it can be quite tricky. This is because threads and asynchronous programming are two fundamentally different paradigms, and Python is not designed natively to support mixing them seamlessly. So, if you’re running into issues while trying to do this, let’s dig a bit deeper to understand why these problems are arising and how we can mitigate or work around the blockers.
A common mistake — and that could be your case — is simply passing an async function as the thread target, like:
xxxxxxxxxx
import threading
import asyncio
async def async_function():
....
t = threading.Thread(target=asyncio.run, args=(async_function(),))
t.start()
Although this might look correct, it will throw an exception. The issue lies in the lines “t = threading.Thread(target=asyncio.run, args=(async_function(),))”. Here, async_function() is called on the main thread instead of the new thread, which is not what we want. Moreover, asyncio.run() should be given a coroutine, but not in the executed form, so directly passing async_function() is also incorrect.
The problem with using threads and asynchronous scripts is they have different event loops by design. This separation between the loops prevents threads from sharing states, thus complicating the process. However, don’t worry; we can still surpass the hurdle by handling the event loop and async functions correctly.
Firstly, we have to ensure that our async function is defined within the main body of the new thread as below:
xxxxxxxxxx
import threading
import asyncio
async def async_function():
...
def thread_function():
asyncio.run(async_function())
t = threading.Thread(target=thread_function)
t.start()
In this way, we bound the async function inside another regular function, which becomes the new thread’s main body, giving us control over the script’s flow and allowing us to fully utilize the benefits of both threading and asynchronous features.
However, remember that all threads have their own event loop, and these cannot be shared between threads unless explicitly assigned. Therefore, take caution when orchestrating multiple threads containing event loops – without proper synchronization, this can result in race conditions or unexpected behavior.
To merely use asyncio functionalities within threads, consider using concurrent.futures module from standard library to create a thread pool and run the tasks asynchronously within the thread pool.
Remember that the beautiful Python language is continually evolving, with major enhancements made to help programmers like us cross these boundaries in future versions. Still, until then, we’ll have to stick to these creative methods for routing around potential challenges inherent in blending synchronous with asynchronous programming modalities.
Always keep these insights in mind when you’re aiming to distribute resource-intensive tasks across system resources optimally!
Asyncio and threading are two powerful components within Python for managing concurrent code execution. Asyncio takes the control of the event loop, whereas threading manages the system threads invoking them concurrently.
However, it’s vital to understand the nuances between async functions (or coroutines) and threads in Python before trying to run async functions via threading.
Understanding the distinctions:
– Multithreading means that multiple threads occur concurrently, excellently managing CPU-bound tasks.
– Async I/O, on the other hand, is for tasks that are largely I/O bound or wait for an external process like network requests — these types can be put together in an event loop where they yield control back to the loop whenever they have to wait.
Now, focusing on how could we mix these two—passing an asyncio coroutine to a thread as the target function implies running that coroutine right away—but async functions (or coroutines) don’t work that way.
Here’s a quick example demonstrating this:
xxxxxxxxxx
import threading
import asyncio
async def foo():
print('Hello')
t = threading.Thread(target=foo)
t.start()
t.join()
This won’t work because foo() isn’t executed; instead, it gives a coroutine object that needs to be run with asyncio’s event loop. This leads us to the conclusion that async functions cannot be passed like regular sync functions to Python threads.
But there is a way to achieve this; you need to make sure the new thread has its asyncio event loop to manage the coroutines. Below is the updated version of the example using asyncio.run(), which runs the asyncio event loop until the given asynchronous task completes.
xxxxxxxxxx
import threading
import asyncio
async def foo():
print('Hello')
def start_loop(loop):
asyncio.set_event_loop(loop)
loop.run_until_complete(foo())
new_loop = asyncio.new_event_loop()
t = threading.Thread(target=start_loop, args=(new_loop,))
t.start()
t.join()
Here, the coroutine
xxxxxxxxxx
foo
is run by the new event loop in a different thread.
However, performance implications arise out of this setup.
– First, the context switching between threads brought by multithreading leads to overhead, decreasing the efficiency of the system resources.
– Second, running async functions through threads squanders the primary advantage of asyncio library – the ability to handle large amounts of I/O bound tasks through cooperative multitasking without the need for threading’s heavy context-switching mechanisms.
– Lastly, most asyncio libraries are not designed to be thread-safe, leading to various potential race conditions when used across threads unless explicitly handled.
For extensive use of threads and async tasks, consider using libraries like Tornado, renowned for enabling both efficiently.
Remember, performance optimization in programming is all about leveraging the toolset given by the language in cognizant ways suitable for your specific application. Select concurrency methods that align well with your application’s requirements for optimal results. Use tools like Python’s debug and profiling functionalities during development to identify any performance bottlenecks early on.
Takeaway: While Python permits us to mix async I/O with multi-threading, it is advisable to do so only after thorough consideration of your task nature, plus understanding threading and asyncio concepts appropriately.
As we delve deeper into the realm of Python coding, it’s clear that threading and asynchronous functions are integral components of efficient programming. Particularly in Python, you may find yourself wondering how to pass an async function to a thread target. Despite your initial impressions, it might not be as straightforward due to the unique characteristics of these functions and threads.
Functions | Threads |
---|---|
Can have return values | Runs independently |
Can handle exceptions | Can run concurrently |
The crux of the challenge lies within the async definition itself – these types of functions are designed for cooperative multitasking, which means they are meant to run concurrently but not necessarily at the same time. On the other hand, threads are seen as separate flows of execution that can indeed run simultaneously.
Understandably, one cannot simply pass an async function to a
xxxxxxxxxx
Thread(target=async_function)
, because an async function must be handled inside an event loop. Here is how you can accomplish it:
xxxxxxxxxx
import asyncio
import threading
async def async_function():
print("Running async function")
def thread_target(loop):
asyncio.set_event_loop(loop)
loop.run_until_complete(async_function())
event_loop = asyncio.new_event_loop()
t = threading.Thread(target=thread_target, args=(event_loop,))
t.start()
t.join()
The key to the solution lies in invoking the async functions inside their dedicated event loops, which then gets passed as an argument to the thread target.
To keep everything running smoothly, async functions should ideally be run from an event loop based on how they were designed, but when necessary, the above method allows them to be utilized via separate threads while staying true to Python’s mechanics and syntax rules.
There is much to discover with Python’s capacity for asynchronicity and concurrent programming. As you continue your journey towards mastery of Python, it pays to deepen your understanding of threading and asynchronous functions, and most importantly, to keep coding.
If you’re interested, here’s a link to Python’s official documentation:
Python’s Official Documentation on Coroutines and Tasks, which is a treasure trove of learning materials about things like asyncio, async/await syntax, event loops, and tasks.