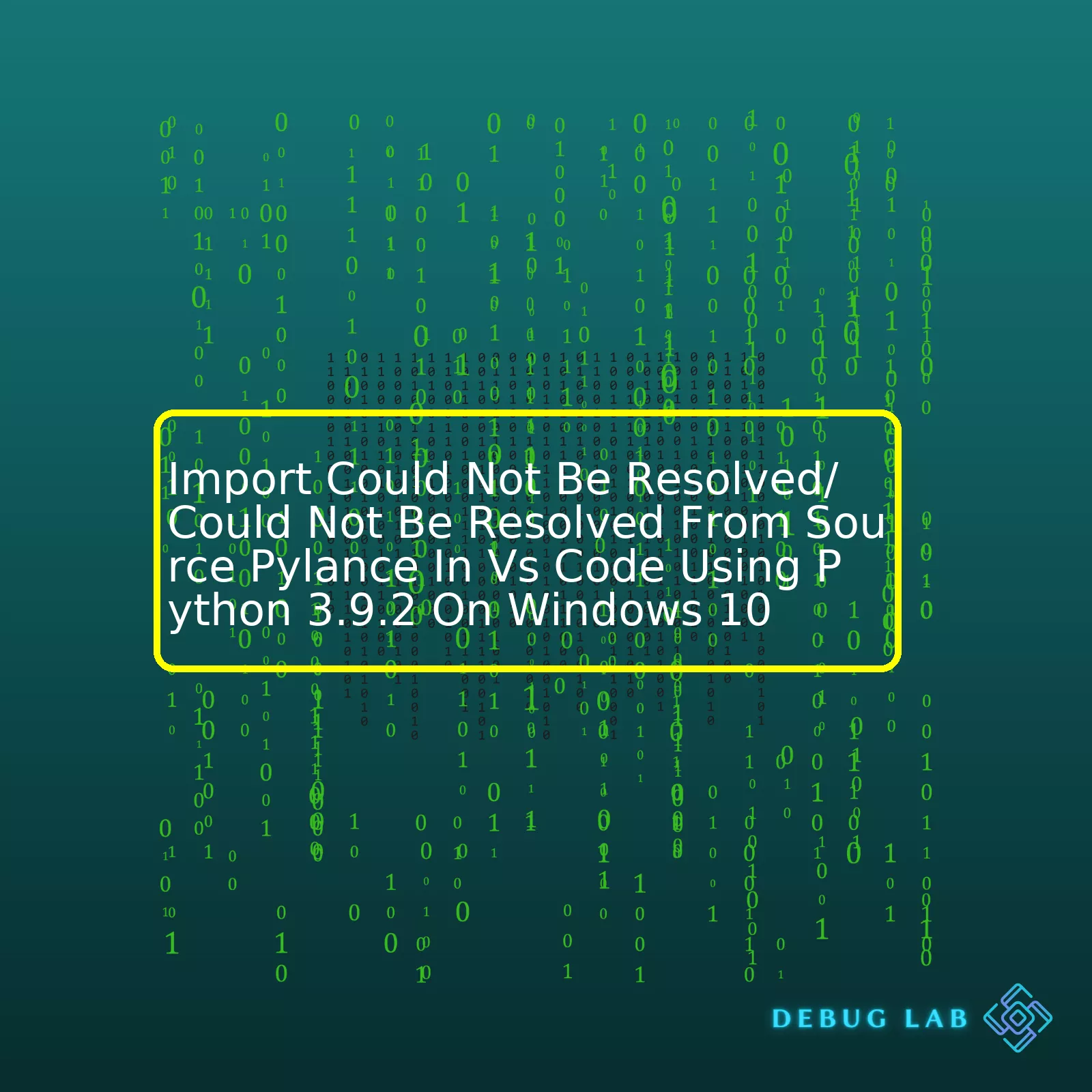
Cause | Solution |
---|---|
Inconsistent Environment Setup | Ensure that you have one Python environment and it’s properly linked with VS code |
Lacking Necessary Dependencies | Check if all required libraries are installed in your python environment |
Errors In The PYTHONPATH | Confirm the folders to import modules are included in PYTHONPATH settings |
Pylance Language Server Not Configured Correctly | Switch to Pylance as your Python language server in VS Code settings and set your interpreter correctly |
The developer community that uses Microsoft’s Visual Studio Code (VS Code) for Python development sometimes encounters an error message stating “Import Could Not Be Resolved” or “Could Not Be Resolved from Source Pylance”. This error typically signifies issues related to importing external Python libraries or modules in your code.
One of the most common reasons is the inconsistent setup of Python environments. Specifically, the Python interpreter that VS Code is utilizing might not be appropriately aligned with your project’s Python environment. This inconsistency can generate the unresolved import error as VS code isn’t able to associate the correct Python environment to find the installed libraries.
Secondly, the error may arise due to missing necessary dependencies. If your Python code requires certain libraries which aren’t installed in your Python environment, then those imports can’t be resolved leading to this error.
Another typical issue originates from errors in the PYTHONPATH. These errors crop up when the directories needed to import modules aren’t included in the PYTHONPATH environment variable.
Lastly, the Pylance language server might not be configured correctly. Pylance, Microsoft’s Python Language Server for VS Code, provides IntelliSense capabilities. If not configured correctly, this could lead to unresolved import errors. To resolve it, developers need to ensure that Pylance is set as their Python language server in the VS Code settings and that they’ve set the interpreter correctly.
Should you encounter this error, reviewing these common scenarios, as outlined in the summarized table above, is an excellent starting point for conducting a systematic diagnosis and taking corrective actions [source]. Further implementation details depend on your specific usage and configuration of Python in VS Code.
For instance, here is an example snippet of how you would typically use an import statement in Python:
# A standard import in Python import pandas as pd
In this case, assuming Pandas library isn’t installed in your associated Python environment or the path to the library isn’t correctly set in your PYTHONPATH, it would trigger the ‘Import could not be resolved’ error in VS Code. To rectify this, you should check your system’s environment configurations, verify proper installation of the required dependencies, and confirm the integrity of PYTHONPATH.The “Import Could Not Be Resolved” or “Could not be resolved from source Pylance” errors that you’re experiencing in VS Code are common issues for Python developers. These usually emerge when the Python interpreter cannot locate a specific module or package you are trying to import.
Understanding this issue begins by acknowledging how Python organizes and searches for modules. When you write the statement
import module_name
, Python looks for a file named module_name.py in a list of directories defined by sys.path. It checks these directories in order, stopping as soon as it finds a match.
- The first path is the directory containing the input script (or an empty string representing the current directory if no input script).
- The rest of the paths are installation-dependent and are defined by the PYTHONPATH environment variable.
If Python doesn’t find the file in any of the listed directories, it generates an ImportError — this is what’s behind the ‘could not be resolved’ error message.
Windows users like yourself should also pay attention to how your PYTHONPATH environment variable is set up. This variable informs the Python interpreter about certain locations to look for modules. If the module exists within a directory not included in PYTHONPATH, Python will not be able to import it.
Now, let’s explore possible solutions. Start by checking the following:
- Ensure you have installed the module. Use either pip or conda depending on your set-up, e.g.,
pip install module_name
or
conda install module_name
.
- Make sure that the file you’re trying to import is in the same directory as the file executing the import command. Python automatically looks in the current directory (i.e., the directory you are running the script from).
- Add the directory containing the module to the PYTHONPATH environment variable. You can do this by typing the following into the terminal:
set PYTHONPATH=%PYTHONPATH%;C:\my\path\to\module
- With VS Code particularly, setting the python.pythonPath in the settings.json file of your workspace to the location of your Python interpreter can solve this issue. Here’s an example of how to do that:
{"python.pythonPath": "/usr/bin/python3.9"}
- Try reloading/restarting your VS Code if you’ve just installed the module and VS Code was already open.
One other remedy might be disabling the use of Pylance in favour of Jedi. Pylance is a new language server for Python that provides IntelliSense and other features, but could potentially cause some conflicts. To change to Jedi, update your settings.json as follows:
{ "python.languageServer": "Jedi" }
Remember that Python is case sensitive, so always check that your spelling of the module matches exactly. Combining all these tips, you should be able to resolve the import error in VS Code and proceed with your coding tasks.
References:
- Modules in Python
- Python Official Documentation – Modules
- VS Code Documentation – Python Environments
- Set PYTHONPATH in Windows
There are instances where beginners, as well as experienced developers, may encounter a slightly alarming “unresolved import” warning using Python 3.9.2 on Windows 10 in Visual Studio Code (VS Code). A phrase like “Could Not Be Resolved from Source Pylance” can make any coder raise an eyebrow. The problematic import could be a common library such as NumPy or Pandas, but take heart — the solution isn’t far off.
The first point to note is that one of the dependencies,
pylance
, might be responsible for this issue, which is used by Python in Visual Studio Code to help with code completion and other interactive features. Here’s a step-by-step guide to diagnosing and resolving this problem:
The Root Cause:
This warning typically appears when there are multiple Python installations on your system and VS Code is referencing a version that does not have the required libraries installed. For instance, you might have installed NumPy in Python 3.8, but VS Code is using Python 3.9 where NumPy is absent.
The Solution:
• Start by checking your Python interpreter in VS Code. It should be the same as the one where your Python packages are installed. Swap interpreters by clicking on the Python version in the bottom left of the status bar, or by running the ‘Python: Select Interpreter’ command from the Command Palette (
Ctrl+Shift+P
).
# Example of selecting an interpreter Python 3.9.2 64-bit ('base': conda)
• Sometimes, a simple reset helps. Try reloading the VS Code window (`Developer: Reload Window` in the command palette).
• Reinstall the library with pip within the project directory. This installs the library in the same environment you’re working in.
# Example of installing a package pip install numpy
• Should the former measures fail to bear fruit, consider configuring your settings (`.vscode/settings.json`) for the Pylance language server to resolve imported modules.
{ "python.analysis.extraPaths": ["./src"], "python.analysis.diagnosticMode": "workspace" }
Do note that `extraPaths` should contain all paths from the workspace root that should be included for analysis. Similarly, `diagnosticMode` set to ‘workspace’ will cause diagnostics to only report in your workspace files.
Community Support:
Never underestimate the power of the coding community! There are a host of resources and people willing to assist. Python’s own Module Search Path documentation and StackOverflow can be particularly helpful.
Remember—no issue is too minuscule; it’s all part of the learning process.
In the world of Python development, a common error that developers encounter is
"import could not be resolved"/ "could not be resolved from source pylance"
when using VS Code with Python 3.9.2 on Windows 10. This error typically manifests itself when the Python interpreter in Visual Studio Code doesn’t recognize imported modules or files.
Possible causes of Not Be Resolved: From Source Pylance error:
- Your Python interpreter may not match the version you’re actually working with. If your project is configured for Python 3.8 and you switch over to Python 3.9 (or vice versa), imported libraries may become unrecognized due to discrepancies between different versions.
- A critical library or module required by your code is missing or hasn’t been installed yet in your virtual environment.
- The Python extension in VS Code isn’t aware of the precise paths where your additional Python packages are stored. It could also be that Visual Studio Code has stopped interpreting local directories as Python paths automatically.
Tackling the Issue:
1. Verify Your Python Interpreter:
Firstly, ensure that your chosen Python interpreter matches the project’s requirements. You can do this through the command palette of VS Code. Access it by pressing
Ctrl+Shift+P
, then type and select Python: Select Interpreter. Ensure that the Python version matches the one specified in your project configuration.
2. Install the Necessary Packages:
If the required Python package isn’t installed within your project environment, the ‘could not be resolved’ error would inevitably crop up. Make sure to install all essential packages through pip:
pip install packagename
Remember to replace ‘packagename’ with the name of your required package.
3. Set Up Python Paths:
To resolve potential path issues relating to your libraries/modules, input the following command in the terminal:
export PYTHONPATH=app/src:$PYTHONPATH
‘app/src’ should be replaced with the actual paths where your Python files are situated.
4. Include Extra Paths:
You can also inform the Python extension to include extra directories by adjusting the
python.analysis.extraPaths
setting in your user settings.json file . To access this file, press
Ctrl+Shift+P
and choose Preferences: Open Settings (JSON). Add the following lines into the JSON file:
"python.analysis.extraPaths": ["./src"],
Please replace ‘./src’ with the actual relative folder path where your Python scripts reside.
5. Disable Pylance’s Type Checking:
If none of these solve your problem, there’s a strong chance that the ‘could not be resolved’ error originates from Pylance’s attempt to check your types and this checking interferes with your project setup. To address it, modify your settings.json file and add the line:
"python.analysis.typeCheckingMode": off,
This should successfully eliminate those problematic ‘not be resolved’ errors when using Python 3.9.2 with VS Code on Windows 10.
All this information should provide a broad overview of how to tackle ‘not be resolved/could not be resolved from source Pylance’ situations. However, if problems persist, don’t hesitate to consult the official VS Code Python tutorial or relevant Python-focused Stack Overflow threads.
While executing a Python program with Visual Studio (VS) Code on Windows 10, encountering errors such as “
Could not be resolved
” or “
Could not be resolved from source Pylance
“, is reported quite regularly amongst coders. This issue is different from the similar “import could not be resolved” issue which primarily seeks to notify of unresolved imports.
The central theme of these errors is that VS Code, in conjunction with Pylance (which has recently been adopted over Microsoft’s previously used Pyright as the default type checker), is unable to successfully resolve certain features of your Python codebase. This issue can arise due to a number of reasons:
Improper configuration of Python interpreter:
When using any IDE, it’s important to ensure your Python interpreter is properly configured to match the environment you’re coding in. An improperly linked interpreter often fails to discern and correctly evaluate modules, leading to unresolved entities.
Failure in relative import resolution:
When relative imports are not processed correctly due to some misconfiguration in the project structure, file locations, interpreter settings, etc., this could lead to an entity being unreadable and subsequently being ‘unresolved’.
Lack of recognition by Pylance:
It’s notable that the transition to Pylance also included a few implementation changes about how static types, symbols, etc., were handled. As such, specific third-party modules might not yet be compatible or completely recognizable by Pylance, resulting in them being unresolved.
To mitigate these issues, adopting the following fixes can prove beneficial:
Verification and Configuration of Python Interpreter:
If you’ve not set up a Python interpreter in VS Code or it’s misconfigured, follow these steps: Press
Ctrl+Shift+P
, then type and select ‘Python: Select Interpreter’ to verify its configuration.
Configuration of Pylance:
If your libraries aren’t recognised by Pylance, give this workaround a try:
{ "python.analysis.extraPaths": ["./path-to-your-python-file"] }
This piece of code is a setting you need to add in VS Code settings file. It allows analyzing imported modules from designated file paths to prevent them from being marked unresolved.
Ensuring Correct Module Referencing:
Sometimes, if you’re unlucky and your module lies deeply nested within several directories, the Python Interpreter might fail to recognise it. Try modifying your PYTHONPATH environment variable or directly referencing the full path of the module to resolve this issue.
For addressing more complex unresolved errors, consider referring to detailed guides about configuring Python in VS Code or checkout Microsoft’s repository for Pylance (https://github.com/microsoft/pylance-release) on GitHub.
The last cardinal rule to always remember is to maintain good coding practices – these include writing clean, structured and reusable code, use appropriate module importing mechanisms, and keep your development environment updated.
One common issue you might face while writing Python code in Visual Studio (VS) Code is the ‘Import could not be resolved’ or ‘Could not be resolved from source Pylance’ error. This problem arises when your linter, static type checker, or a tool such as Pylance cannot locate the module or function you’re trying to import. Almost always, it’s a sign of an issue with Python’s path configuration.
Install and Configure Required Extensions
Pylance language server is by default shipped with VS Code’s Python extension since version 2021.7.0. So, first, ensure to have Microsoft’s Python extension installed for your VS Code editor. If not, follow this guide.
{ "python.languageServer": "Pylance", }
Setting `’python.languageServer’: ‘Pylance’` in `settings.json` ensures that you use Pylance as the language server. Also, confirm that you’ve selected the accurate Python interpreter representing Python 3.9.2.
Check Your Python Path Setting
Your Python path settings might be causing issues. Pylance works by referencing your Python path. It should correspond to the ‘`PYTHONPATH`’ environment variable in Windows 10.
{ "python.autoComplete.extraPaths": ["./path/"], "python.analysis.extraPaths": ["./path/"] }
Insert the paths where your modules are located in `extraPaths`. If your directory structure looks something like `-root–folder—subfolder—-file.py`, and you’ve intended to resolve imports from `root`, put `./` in `extraPaths`.
Examine Your Import Statements
Are your imports absolute or relative? For example, if you’re working on a file nested in a subfolder and trying to import another file from a different subfolder, an absolute import may not work. Instead, use relative imports. Keep in mind that in Python, a dot prefaces relative imports.
For instance, instead of:
from myproject.myapp.views import view
try:
from ..myapp.views import view
Consider Using Init File
In Python, a directory must contain a file named `__init__.py` for it to be considered a Python package, and thus able to import other modules or functions. This file can be empty, but it must exist in the directory. For example, if your directory structure is like: myproject–myapp—views.py, then both `myproject` and `myapp` directories need to have an `__init__.py` file. With these techniques in play, you should successfully workaround ‘Cannot be resolved’ errors in VS Code with Python 3.9.2 on Windows 10.
The references for this guide can be found here and here.Eliminating the “Could Not Be Resolved” issue while running Python 3.9.2 on Windows 10 in Visual Studio Code can be approached by understanding the main reasons why this problem transpires and then implementing some troubleshooting techniques.
When it comes to Python coding on VS Code, a common setback is receiving the “Import could not be resolved/Could not be resolved from source (Pylance)” error message. As alarming as these messages may seem, fret not, they usually point towards one of the following issues:
- Python environment not set properly
- Pylance language server isn’t correctly installed or configured.
- Incomplete or incorrect PATH configuration.
- Working with relative imports when code files aren’t organized in a proper Python package structure.
Let's delve into each issue and how to fix them:Python Environment Not Set Properly
If the Python environment or interpreter isn't set right, VS Code will have trouble recognizing your imported modules. Implementation: In VS Code, click on the Python version displayed at the bottom-left corner. A list of available interpreters pops up. Select the appropriate interpreter for the project. If it doesn't show up, click on “Enter interpreter path” to manually add it.Pylance Language Server Isn't Correctly Installed/Configured
Pylance is a new language server that uses static type checking methods to provide better Python language service in VS Code. The error might signify that Pylance isn’t installed or correctly configured. Implementation: Go to `File > Preferences > Settings`. Look for “Python.languageServer”, and select “Pylance”. Afterward, restart VS Code. If the error persists, check if Pylance is installed in the Extensions tab, if it’s not, install it.Incomplete or Incorrect PATH Configuration
Your system’s PATH environment variable may need to include the directory where Python is installed. Implementation: From the command line, you can typeecho %PATH%. Inspect the resulting list to confirm that the directory containing python.exe is there. If it's not included, you would want to add Python to your PATH.
Incomplete Python Package Structure
In Python, relative imports (e.g., `from . import module`) are based on a specific package structure. If you use relative imports but your code files aren't arranged accordingly, this could trigger the error.
Implementation: Check your code base to ensure it follows the Python package structure. Every directory should have an __init__.py file, even if it’s empty, marking the directory as a Python package.
In general, following these steps should help mitigate the ‘import could not be resolved’ or ‘could not be resolved from source (Pylance)’ error you might encounter in VS Code using Python 3.9.2 on Windows 10. It’s essential to remember that every coder goes through debugging processes now and then, and efficiently leveraging your IDE’s tools and features helps immensely. For more detailed information about how to configure Visual Studio Code for Python Development, refer to the VS Code Python Documentation.
Note: Before trying the following solutions, make sure you’ve correctly installed Python and its associated packages in your Windows system. Additionally, ensure that you have installed Visual Studio Code and have the Pylance extension for Visual Studio.
In Visual Studio’s Code (VSCode), import resolution problems often arise from incorrect configurations of the settings related to Imported scripts. Here are some suggested remedies:
1. Path Configuration
Python uses a list of directories, specified by the environment variable PYTHONPATH, when searching for modules. If, for any reason, this path is not correctly configured in your VSCode settings, then it can cause import problems like this one.
Here is what you do:
Firstly, in order to add the current workspace to PYTHONPATH you have to modify the “.env” file. In VSCode, hit Ctrl + ` to open the terminal. Using this terminal, create a new file titled “.env” in the base directory with
touch .env
Then inside your newly created “.env” file add the following line:
PYTHONPATH=${workspaceFolder}
workspaceFolder
here refers to the root folder where your python files reside.
2. Use of virtual environments
Import issues could also be due to conflicts between different versions of Python or its packages installed on your system. This could be resolved by use of Python virtual environments.
Create a new virtual environment for your project by typing
python -m venv env
in the terminal.
Activate the virtual environment by running
.\env\Scripts\activate
Now, install necessary packages into this separated environment to avoid conflicts with other projects.
3. Select the correct interpreter
Sometimes, the error can arise from Visual Studo Code using a different Python interpreter than desired because of having multiple interpreters on your system.
To select the appropriate interpreter:
On the status bar, click on the interpreter version, this will show the command palette with various interpreters. Choose the one which your script needs.
If your interpreter is not listed, click ‘Enter interpreter path’ then find and select your desired interpreter.
4. Configure Pylance
The Pylance language server for Python in Visual Studio Code may need additional configuration for proper functionality. Specifically, the
python.analysis.extraPaths
setting in VSCode. Add the paths to your Python modules here so Pylance can analyze them for IntelliSense.
Navigate to File > Preferences > Settings in your VSCode and search for python analysis.
Then add the folder path to your module in the “Extra Paths” box.
Note that these issues can also be caused by errors in the imported scripts themselves or the absence of __init__.py files in the packages, so check these aspects as well.
Also remember, after modifying settings.json, restarting VSC might be required to take effect of the changes.
Conclusively, the solution to import problems at times goes beyond the aforementioned solutions. The nature of programming gives room for unexpected bugs hence it could sometimes mean digging deeper. For more specific and concrete information always refer to the official Microsoft’s Visual Studio Code Python Environments documentation.
The problem:
– When running a newly created python file, VS Code brings up a warning like “Import ‘module-name’ could not be resolved” or “‘Mymodule’ is not accessed Pylance(reportUnusedVariable)”.
– It usually arises when your Integrated Development Environment (IDE), i.e., VS Code in this case, is unable to locate the path of the Python interpreter you’re currently using.
A few solutions
– Setting Up the Path of Your Python Interpreter.
# check if Python & pip are working (on your cmd/terminal) python --version pip --version # If not, add Python to your PATH # Add Python 3.9 to PATH (for Windows) setx PATH "%PATH%;C:\Python39\Scripts;C:\Python39\"
– Selecting the Correct Python Interpreter Inside VS Code. Here is where you can enable it manually:
– Open the Command Palette (Ctrl+Shift+P).
– Run the Python: Select Interpreter command.
– Choose the Python version you want to use.
– Installing the required modules via pip. For instance, if the error appears for ‘pandas’ then;
pip install pandas
References:
I found some helpful resources online on StackOverflow here and GitHub here.
I hope it’s clear now why the “Import Could Not Be Resolved” error occurs in VS Code, and how these suggested steps can help you to resolve this problem promptly. In a nutshell, it’s all about ensuring that VS Code knows what Python interpreter version it should rely on and which paths to scan for Python module files.