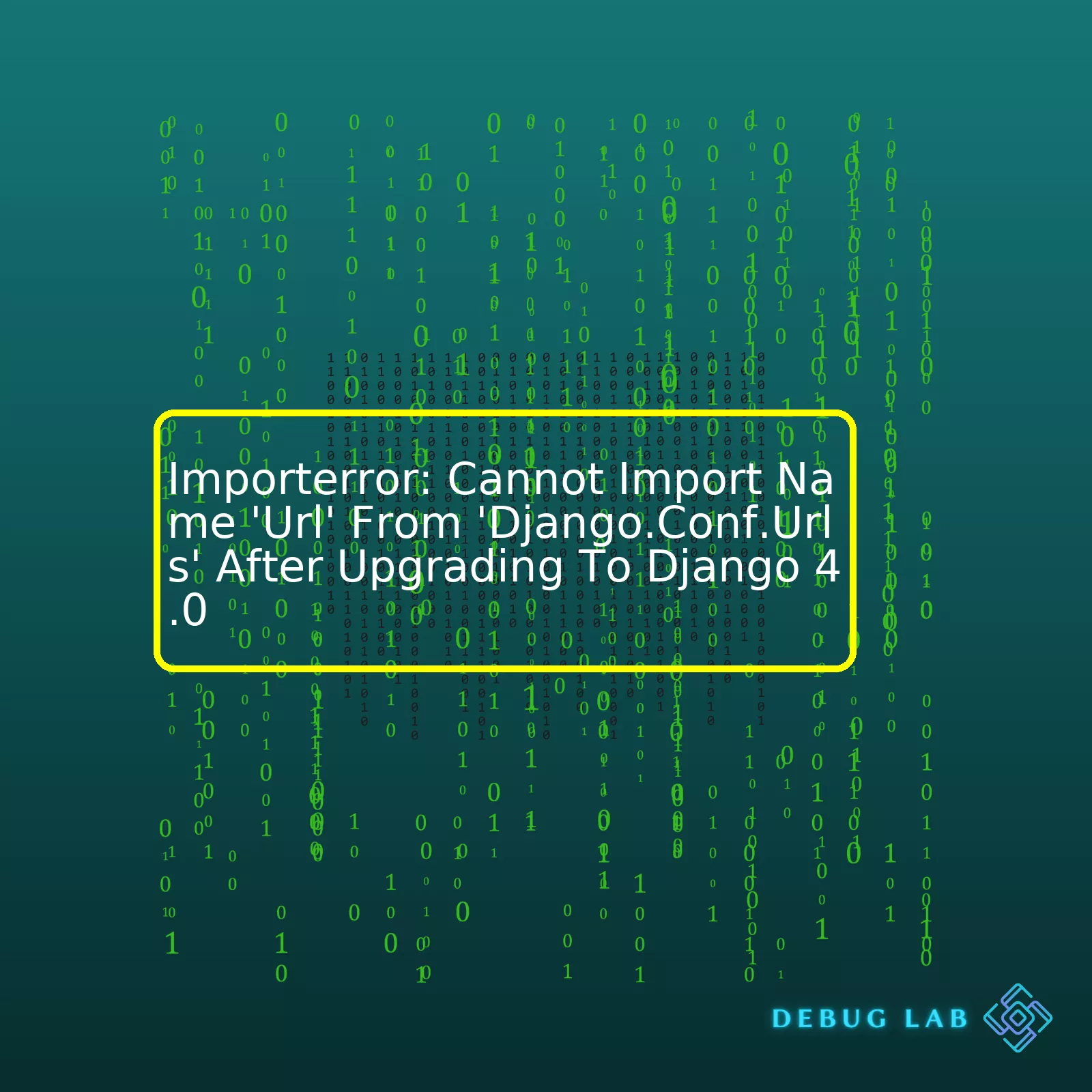
Essentially, the issue is due to a change in how url routing works with the upgrade to Django 4.0. The
url()
function, which was deprecated in Django 3.1, has been removed in Django 4.0.
Django Version | Status of
xxxxxxxxxx 1 1 1 url() |
---|---|
Django 3.1 and before |
xxxxxxxxxx 1 1 1 url() function available but deprecated |
Django 4.0 and above |
xxxxxxxxxx 1 1 1 url() function removed |
You could replace
xxxxxxxxxx
url()
with path() or re_path() according to your need.
Let’s take an example –
If your earlier code was:
xxxxxxxxxx
from django.conf.urls import url
...
url(r'^admin/', admin.site.urls),
You would now replace it with:
xxxxxxxxxx
from django.urls import path
...
path('admin/', admin.site.urls)
Remember, when replacing
xxxxxxxxxx
url()
with either
xxxxxxxxxx
path()
or
xxxxxxxxxx
re_path()
, carefully consider every instance as the replacement might require updating regular expressions present in your url patterns to new formatting style.
As always, the best practice to avoid such sudden errors is to frequently check Django deprecation timeline and update your code as necessary to ensure smooth transitions between different versions of Django.Oh, the joys of upgrading a dependency, finding everything broken, and scratching your head about what could have possibly gone wrong. Today, we’re taking a deep dive into the particular situation of trying to upgrade to Django 4.0 only to find ourselves facing
xxxxxxxxxx
ImportError: Cannot import name 'url' from 'django.conf.urls'
. Don’t worry if this is where you’ve found yourself. With my trusty coder cap on, I’ll guide you through the process of troubleshooting this pesky error and setting things right again.
The root cause of this error traces back to changes made in Django’s URL dispatcher. In versions prior to Django 2.0, the `url()` method was prevalent to define URL patterns. However, with evolution and enhancement, Django introduced the `path()` method starting from version 2.0 onward which simplifies URL pattern creation.
In Django 4.0, the `url()` method has been officially removed, causing the ImportError you’re encountering. But no need to fret, the solution is fairly simple — it involves replacing all instances of `url()` in your URL configuration files with the `path()` method or the `re_path()` if you have URL patterns using regular expressions.
Let’s say you had the following URL patterns in your Django application that utilise the now deprecated `url()` function:
xxxxxxxxxx
from django.conf.urls import url
from . import views
urlpatterns = [
url(r'^articles/2003/$', views.special_case_2003),
url(r'^articles/(\d{4})/$', views.year_archive),
url(r'^articles/(\d{4})/(\d{2})/$', views.month_archive),
url(r'^articles/(\d{4})/(\d{2})/(.+)/$', views.article_detail),
]
You’d simply rewrite them using the new `re_path()` function:
xxxxxxxxxx
from django.urls import re_path
from . import views
urlpatterns = [
re_path(r'^articles/2003/$', views.special_case_2003),
re_path(r'^articles/(\d{4})/$', views.year_archive),
re_path(r'^articles/(\d{4})/(\d{2})/$', views.month_archive),
re_path(r'^articles/(\d{4})/(\d{2})/(.+)/$', views.article_detail),
]
See? Not so scary after all! This change not only allows you to work with Django 4.0 without any ImportErrors but also prepare you for future Django updates where the `path()` method will be highly emphasized. You can refer to this [official documentation](https://docs.djangoproject.com/en/stable/releases/3.1/#features-deprecated-in-3-1) of Django to learn more about the deprecation timeline.
Bear in mind, this isn’t just about fixing an error; it’s about embracing the evolutionary changes occurring within Django. The `path()` method brings in more readability and simplicity to work with, ultimately enhancing coding efficiency. The same applies when comparing `re_path()` to `url()`, especially when regular expressions are involved.
As you venture further on your coding journey, remember that encountering bugs and working to resolve them is integral to making you a better programmer. Embrace the challenge – happy coding!Before discussing the
xxxxxxxxxx
ImportError: Cannot import name 'url' from 'django.conf.urls'
, it’s crucial to get a comprehensive understanding of what django.conf.urls is all about. This module performs an essential task in Django web application development by mapping routes to respective views. However, if you’re familiar with Django 3 and recently upgraded to Django 4, you might deal with ImportError as
xxxxxxxxxx
url()
from django.conf.urls isn’t available in Django 4.
In Django 3.x and earlier versions, you would structure your URLs like this:
xxxxxxxxxx
from django.conf.urls import url
urlpatterns = [
url(r'^admin/', admin.site.urls),
]
However, starting with Django 4.0, the url() syntax has been deprecated in favor of re_path() and path(). The new syntax for urlpatterns in Django 4.0 looks like this:
xxxxxxxxxx
from django.urls import re_path
urlpatterns = [
re_path(r'^admin/', admin.site.urls),
]
In this case,
xxxxxxxxxx
re_path()
uses regex for routing urls, just like
xxxxxxxxxx
url()
. This change resulted from the decision to simplify and streamline URL routing in later Django versions. Developers should embrace the new way of doing things since they provide more straightforward and concise URL pattern representations.
Let’s use an example:
xxxxxxxxxx
from django.urls import path
# Django 3
from django.conf.urls import url
urlpatterns = [
url('articles/2003/$', views.special_case_2003),
url('articles/(?P<year>[0-9]{4})/$', views.year_archive),
]
# Django 4
from django.urls import re_path
urlpatterns = [
re_path('articles/2003/$', views.special_case_2003),
re_path('articles/(?P<year>[0-9]{4})/$', views.year_archive),
]
Firstly, the table below shows the changes in Django.conf.urls from Django 3.x to Django 4.x:
Django 3.x | Django 4.x |
---|---|
xxxxxxxxxx 1 1 1 from django.conf.urls import url |
xxxxxxxxxx 1 1 1 from django.urls import re_path |
For developers looking to upgrade their applications from Django 3 to Django 4, one of the first necessary alterations is replacing
xxxxxxxxxx
url()
with
xxxxxxxxxx
re_path()
or
xxxxxxxxxx
path()
.
If you want more specific information about this update and its implementation, check out the Django official documentation. It provides the detailed technical documentation on the enhancements and optimizations in Django’s newer versions. By refining your knowledge, you can better manage migrations from older Django versions (like Django 3.x) to the newer ones such as Django 4.x.
Dedicate some time into reading this documentation to understand how this transition may affect your project’s codebase.
The upgrade doesn’t have to be a daunting process, and with a little bit of thoughtful planning and attentive execution, you can bring your Django project up to speed with the most recent developments in this robust and flexible framework.
The upgrade to Django 4.0 removed a key component utilized widely in older versions of the framework – the ‘url’. This removal could create significant challenges if you’ve been relying heavily on this feature for managing your project’s URL structures.
Why did Your Project Break?
The 'url' function has been discarded beginning with Django 4.0. It used to provide an easy way to define URLs for your application based on regular expressions. However, from Django 2.0 onwards, the Django team began pushing developers towards using ‘path’ and ‘re_path’ for URL routing to make things more intuitive and easier to manage.
If you encounter the ImportError: Cannot import name ‘url’ from ‘django.conf.urls’, it is because your code, or parts of it still rely on the 'url' function. This problem arises when you’ve upgraded to Django 4.0 since that function no longer exists within the new version of the framework.
Solving the Issue
The most direct solution is to replace instances of 'url' usage with either 'path' or 're_path'. Below is a comparison to help illustrate the changes required:
Before Django 4.0 (using 'url'): | After Django 4.0 (using 'path'): |
---|---|
xxxxxxxxxx 1 1 1 url('^about/$', views.about) |
xxxxxxxxxx 1 2 1 from django.urls import path 2 path('about/', views.about) |
The first column demonstrates how URLs were mapped before Django 4.0 while the second column shows the recommended approach. This switch means performing an audit of your current URLs, identifying their usage of 'url', and replacing those instances where applicable with the ‘path’ function.
You might also consider employing Django’s support for third-party packages for routing incoming requests. Libraries such as django-hosts further extend Django’s built-in routing functionality. An elaborate guide can be found on the official documentation page.
Ongoing Maintenance
It’s essential to note that this change is a signal of Django’s shift towards simpler and more intuitive URL mapping. Keep up-to-date with these changes by regularly checking Django release notes. Following this practice allows you to proactively adjust your codebase to accommodate upcoming changes and avoid sudden breakages upon upgrading to newer releases of Django.
In summary, the ImportError faced after upgrading your Django to 4.0 is due to the discontinuation of the ‘url’ component in Django’s libraries. You can resolve this issue by refactoring your code to use ‘path’ or ‘re_path’ instead, thus not only solving your error but also aligning your project with the latest practices in Django URL management.
In Django 4.0, the
xxxxxxxxxx
url()
function was removed from the main package. This change likely led to the ‘ImportError: cannot import name ‘Url’ from ‘django.conf.urls” error you’re experiencing after upgrading to Django 4.0.
In lieu of using
xxxxxxxxxx
url()
, Django now recommends using the
xxxxxxxxxx
path()
and
xxxxxxxxxx
re_path()
functions instead. Below is an outline of why these functions are favored and examples of how to implement them.
Understanding:path() and re_path()
xxxxxxxxxx
path()
The
xxxxxxxxxx
path()
function simplifies URL pattern creation by eliminating regular expressions. With
xxxxxxxxxx
path()
, we use angle brackets to define variables in our URL pattern. Here’s a simple example:
xxxxxxxxxx
path('home/<int:id>/', views.my_view)
In this situation, any integer number passed in the URL as ‘id’ will be captured and handed over to the view. You can even specify string type or slug type parameters.
xxxxxxxxxx
re_path()
Although
xxxxxxxxxx
path()
can handle most URL patterns effortlessly, sometimes regular expressions are needed. For such situations, Django provides the
xxxxxxxxxx
re_path()
function. This works similarly to the old
xxxxxxxxxx
url()
function as shown in the following example:
xxxxxxxxxx
re_path(r'^home/(?P<id>\d+)/$', views.my_view)
This is how you might typically use regular expressions in Django URLs.
Updating Your Code
Subsequent to understanding the functions
xxxxxxxxxx
path()
and
xxxxxxxxxx
re_path()
, the next step would be to update your existing codebase – replacing all instances of the outdated
xxxxxxxxxx
url()
function with either
xxxxxxxxxx
path()
or
xxxxxxxxxx
re_path()
, depending on the use case. After doing so, it should resolve the ImportError you got after upgrading to Django 4.0.
Here’s how you can potentially change the import statement at the top of your
xxxxxxxxxx
urls.py
file:
Before:
xxxxxxxxxx
from django.conf.urls import url
After (using
xxxxxxxxxx
path()
):
xxxxxxxxxx
from django.urls import path
or (using
xxxxxxxxxx
re_path()
):
xxxxxxxxxx
from django.urls import re_path
With these changes implemented correctly, you should no longer be encountering the ImportError after the Django 4.0 upgrade. If you’d like to read more about URL dispatching and the various functions available, Django’s documentation provides a comprehensive guide.The ImportError that you’re encountering in Django 4.0, specifically ‘ImportError: Cannot import name ‘url’ from ‘django.conf.urls’, is often due to a change made after upgrading from an earlier version of Django to version 4.0.
In versions before Django 2.0, the
xxxxxxxxxx
url()
function was used to route URLs. However, this function has been deprecated in Django 2.0 and removed in Django 4.0. The
xxxxxxxxxx
path()
function is now recommended for URL routing. Therefore, if your Django project still uses the
xxxxxxxxxx
url()
function, you’ll get an ImportError after upgrading to Django 4.0.
Here is a step-by-step guide on how you can address this issue:
– Step 1: Open your urls.py file. This file is typically located within each app directory in your Django project structure.
html
/projects/myproject/myapp/urls.py