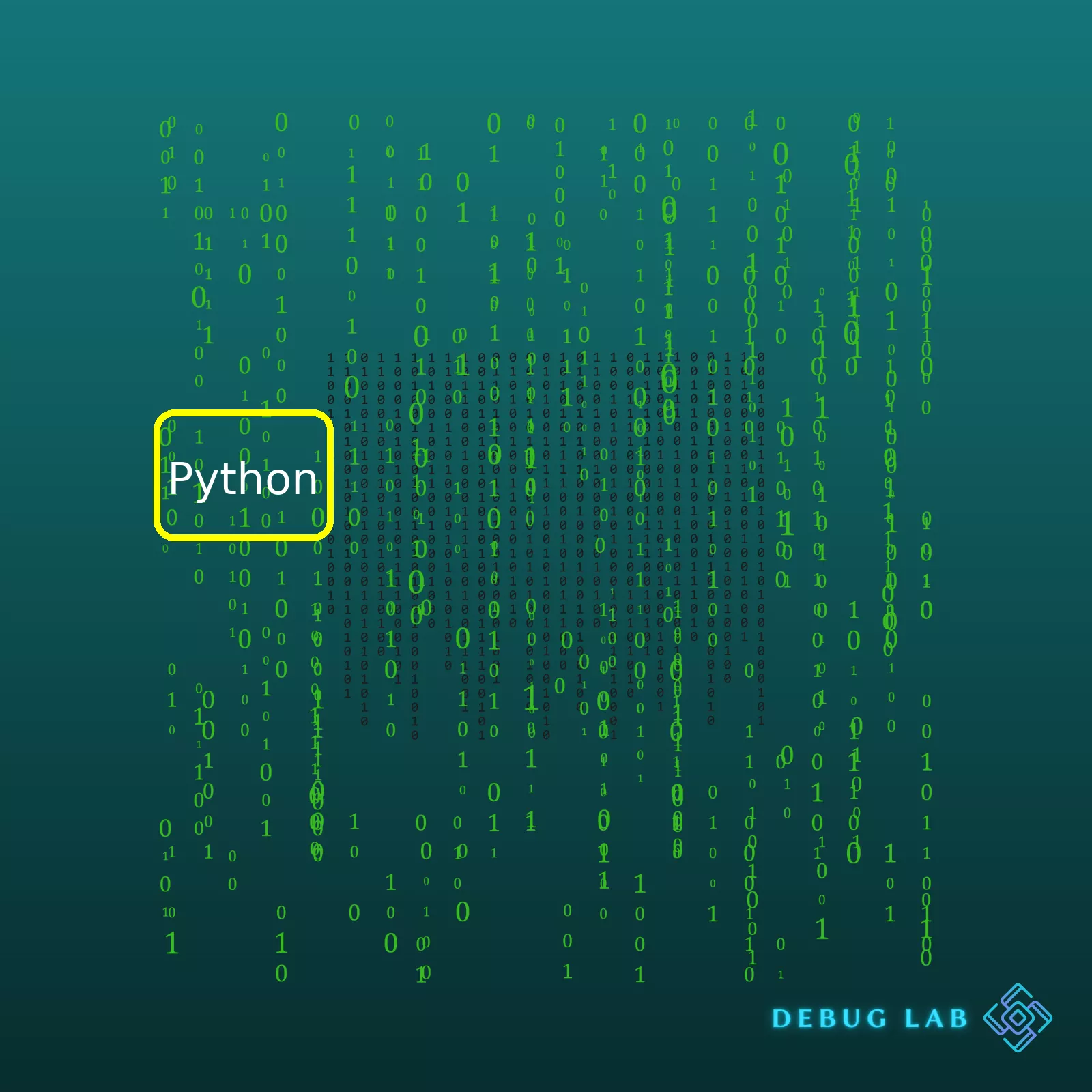
Firstly, we would need to install these libraries (if not installed) using pip:
pip install pandas tabulate
After successfully installing these libraries, I have written a simple Python script that generates a summary table in the form of HTML.
Using Pandas DataFrame which allows us to create a table by initializing a 2D list and setting column names and indices with .DataFrame() and tabulate() functions.
import pandas as pd from tabulate import tabulate data = [['Tom', 10], ['Nick', 15], ['John', 14]] df = pd.DataFrame(data, columns = ['Name', 'Age']) print(tabulate(df, headers='keys', tablefmt='html'))
This code will output an HTML format similar to:
<table> <thead> <tr><th> </th><th>Name </th><th> Age</th></tr> </thead> <tbody> <tr><td>0 </td><td>Tom </td><td>10 </td></tr> <tr><td>1 </td><td>Nick </td><td>15 </td></tr> <tr><td>2 </td><td>John </td><td>14 </td></tr> </tbody> </table>
The code snippet above gives us an HTML-formatted summary table that showcases data about individuals and their respective ages. We created this table initially in Python, using the Pandas library and then used the ‘tabulate’ function from the Tabulate module to convert the dataframe into an HTML format.
It’s important to note here that:
– The ‘pandas’ library is a powerful tool for data manipulation in Python and ‘DataFrame’ is a two-dimensional table of data with columns that can be of different types.
– The ‘tabulate’ library provides a function ‘tabulate()’ that takes a list of lists (or another tabular data structure) and formats it into a nice textual table.
– While generating our HTML table, we chose the ‘headers’ parameter as ‘keys’ to display DataFrame’s column names, and the ‘tablefmt’ parameter as ‘html’ to get the table in HTML format.
The beauty of Python, in part, comes from its vast amount of libraries, allowing programmers to perform a huge variety of tasks without writing much code at all. In this context, it provides us with an ability to transform data structures into an HTML format quite effortlessly, making it immensely powerful when it comes down to handling and representing data efficiently. For more detailed implementation related to Pandas and tabulate, one can refer to official Pandas documentation here and also find Tabulate module information right here.It’s undeniable that Python has become one of the most popular programming languages in the world, and for good reason. Its clean syntax, vast library support, and ease of learning make it an ideal language for both beginners and experienced developers alike.
Let me take you through some key features and aspects of Python:
1. Python’s Syntax
Python’s syntactical structure is designed to be intuitive and clear, removing a lot of the ambiguity and complexities found in other programming languages. In Python, whitespace is part of the syntax. Every code block is defined by its indentation level, which allows the code to be both easy to write and read. For example:
def say_hello(): print("Hello, world!") say_hello()
2. Key Concepts of Python
Python, at its heart, embraces a few key principles:
- Readability: Code is meant to be as readable and unambiguous as possible.
- Simplicity: There should be one— and preferably only one —obvious way to do something.
- Explicit over implicit: Code should be straightforward and clear rather than relying on hidden functionality or guessing what the user wants.
These principles are outlined in The Zen of Python, a list of aphorisms that capture the guiding philosophies behind Python’s design.
3. Standard Libraries and Packages
Python provides a wide array of standard libraries and packages that come bundled with the language. This includes math and statistics modules, tools for handling dates and times, complex data structures, threading utilities, and much more.
For instance, with just a few lines of code, you can parse a CSV file using Python’s standard CSV library:
import csv with open('file.csv', 'r') as f: reader = csv.reader(f) for row in reader: print(row)
On top of this, there’s practically an endless supply of third-party packages available via Python’s package manager, pip, that can handle everything from web development to scientific computing.
4. Python Use Cases
Python finds application in several areas:
- Data Science: Python is a go-to language for Data Science and Machine Learning, largely because of libraries like Numpy, Pandas, and Matplotlib that provide powerful yet accessible tools for managing and analyzing data, and creating visual representations.
- Web Development: Python-based frameworks like Django and Flask enable robust and flexible web development.
- Automation & Scripting: Python’s simplicity and extensive library support make it great for writing scripts to automate various tasks.
- Education: Python is often the first language taught in many Computer Science programmes because of its simple syntax and readability.
While Python does have some disadvantages such as slower execution speed compared to languages like C++ or Java, its benefits far outweigh these limitations for most use-cases.
Python is truly a versatile tool and understanding its potential applications and leveraging its strengths can significantly boost your productivity and creativity as a coder.Python syntax and key concepts are essential in understanding this powerful, open-source programming language. In this section, we are going to focus more on Python syntax, its unique characteristics, and program flow control concepts like loops and conditionals.
Python Syntax:
1. Indentation: Unlike other languages that use braces to define the scope of loops, functions or conditional blocks, Python uses indentation. Improper indentation can lead to a
SyntaxError
.
Here’s an example:
def greet(): print("Hello World") greet() # This will print: Hello World
2. Comments: Python uses
#
for single line comments and triple quotes (
'''...
or
"""..."""
) for multi-line comments.
Example:
# This is a comment ''' This is a multiline comment '''
3. Line Continuation: Python supports implicit line continuation inside parentheses
()
, brackets
[]
, and braces
{}
. For other cases where implicit continuation is not possible, we use a backslash (`\`).
# Implicit line continuation var = [ 'item_one', 'item_two' ] # Explicit line continuation var = 'item_one' \ + 'item_two'
Python Key Concepts:
1. Data Types: Python comes with built-in data types like integers, floating point numbers, strings, lists, tuples, sets, and dictionaries.
2. Functions: Functions are reusable pieces of code. They are defined using the
def
keyword. There’re also anonymous functions called lambda.
3. Modules: A module is a file containing Python definitions and statements intended for reuse. We can use any Python source file as a module by executing an import statement in some other Python source file.
4. Exception Handling: Python provides a way to handle the exception so that the code can be executed without interruption. It uses
try....except
block to catch and handle exceptions.
5. Classes/Objects: Python supports Object Oriented Programming. We can define classes and create objects of those classes.
In addition to these, topics like file operations, regular expressions, JSON, databases, and multithreading are also important points in Python which you must know.
Coding in Python becomes much simpler once we understand its unique syntax patterns and key concepts. Pythonic way of writing code encourages keeping it simple, readable, and explicit. You can find more details in the official Python Documentation.In the world of Python programming, understanding data types is crucial as they lay the foundation of every operation. Data types are essential as they determine what type of value a variable can hold and what kind of operations can be performed.
Python Built-In Data Types
Python standard data types include; integers, floating-point numbers, strings, lists, tuples, dictionaries, sets, Booleans, and complex numbers, among others.
Data Type | Description |
---|---|
Integers | Numerical values which can be positive or negative but without a decimal point, such as 10, -15, or 4000. |
Floating-Point Numbers | Numbers having one or more digits past the decimal point such as 1.0, -15.21. |
Strings | Sequence of characters within quotations. Python supports both single(‘) and double(“) quotes to encapsulate strings, like “Hello, World!” or ‘Python Programming.’ |
Lists | A list in Python is an ordered sequence of items (of any type), enclosed in square brackets([]). |
Tuples | A tuple is a collection of objects which are immutable and imply order amongst its elements. |
Dictionaries | Also known as associative arrays, keys mapped to values using key-value pair. It is mutable and does not preserve the order of declaration. |
Sets | An unordered collection data type that is iterable, mutable, and has no duplicate elements. |
Booleans | Used for truthy values. Can only contain True or False. |
Complex Numbers | A combination of real and imaginary numbers. For example, 2+3j is a complex number. |
Defining and Using Python Data Types Examples
For example, you can define an integer, a float, and a string in Python as shown below:
an_int = 3 a_float = 3.14 a_string = "Pi approximates to"
You can use these variables, print them out, or execute operations on them.
print(a_string, a_float) sum = an_int + a_float print(sum)
Python automatically identifies data types at runtime, so there’s no requirement to declare a variable’s data type beforehand.
Conversion Between Data Types
Maintaining versatility, Python allows conversion between data types. However, ensure to watch out for erroneous conversions.
num_string = "123" num_int = int(num_string)
Above code is converting the string “123” into an integer.
Apart from built-in data types, Python also supports custom data types. Often used in OOP paradigm where user-defined types like ‘Classes’ are commonly created.
Overall, it is integral to understand how different Python data types interact with each other and their various methods to master Python coding.
To delve deeper into Python data types, consult Python’s official documentation.When discussing Python programming, a couple of crucial components are functions and decorators. Functions and decorators play an instrumental role in helping developers write cleaner, more efficient, and reusable code.
Functions in Python
A function in Python can be interpreted as a collective block of re-usable code aimed at performing specific tasks. Functions essentially enhance code reusability and help to structure the program in a sizable manner. They also allow high levels of code modularity as developers can create blocks for repeated actions.
To declare a function in Python we use the
def
keyword. Here’s the basic syntax for a Python function:
def function_name(arguments): # function_body return output
In our example,
function_name
is the name of our function,
arguments
is optional and it represents the inputs that the function works with, and
# function_body
refers to the block of code to perform a set of specific tasks. It’s not obligatory to include a
return
statement in your function; if omitted, a Python function will still work but won’t send any value back when called.
Let’s take a look at a simple function that multiplies two numbers and returns the result:
def multiply_numbers(num1, num2): result = num1 * num2 return result
This function, named “multiply_numbers”, takes two arguments: num1 and num2. It then multiplies these numbers and the product is returned by the function.
Decorators in Python
Python decorators act as wrappers, modifying the behavior of the code before and after a target function execution, without altering the function itself, augmenting the original functionality and thus enriching its behavior[source]. They are a syntactically elegant way to alter functions or methods, or even to extend their behavior.
A decorator in Python is implemented as a callable object (like a function) that returns a wrapper function. We denote a decorator by the
@
symbol followed by the decorator name.
@decorator_name def function_name(arguments): # function_body
Here’s how you can define a simple decorator to calculate and display the execution time for a function:
import time def execution_time_decorator(func): def wrapper(*args, **kwargs): start_time = time.perf_counter() result = func(*args, **kwargs) end_time = time.perf_counter() print(f"{func.__name__} executed in {end_time - start_time:.4f} seconds") return result return wrapper @execution_time_decorator def multiply_numbers(num1, num2): result = num1 * num2 return result
The function “multiply_numbers” is decorated by “execution_time_decorator”. The wrapper function inside the decorator calculates the execution time of the function call and then returns the result of the function call.
In conclusion, Python promotes clean and efficient programming by using functions and decorators. While functions allow developers to organize and reuse codes, decorators grant us the capacity to modify or expand the behavior of functions without permanently modifying them.In the world of Python programming, there can be numerous ways to solve a single problem. However, the efficiency with which we address these problems is what sets apart good coding practices from great ones. It’s about code that runs faster, uses fewer resources, and performs tasks more effectively. One way to improve efficiency in your Python code is by optimizing it with advanced libraries.
One such advanced library for Python is NumPy. NumPy stands for “Numerical Python,” and it’s a fundamental package for high performance scientific computing and data analysis.
Why use NumPy? Well, consider the case where you have to perform operations on an array of numerical data. With basic Python arrays, you’d naturally resort to loops. While this might get the job done, it’s certainly not the most efficient approach as the data size increases.
Imagine you are burdened with the task of adding two large lists element-wise. Here’s how you would generally do it:
list1 = [1, 2, 3,..., n] list2 = [4, 5, 6,..., n] sum_list = [] for (item1, item2) in zip(list1, list2): sum_list.append(item1 + item2)
Now, suppose you were to solve the same problem using NumPy:
import numpy as np list1 = np.array([1, 2, 3,..., n]) list2 = np.array([4, 5, 6,..., n]) sum_array = np.add(list1, list2)
For larger lists, the second approach will provide a significant boost in terms of both memory usage and computation speed. The reason being NumPy arrays are densely packed arrays of a homogeneous type, while Python lists are arrays of pointers to objects, adding a layer of overhead.
Another optimization-focused python library is Pandas. Pandas is one of the most widely used python libraries in data science. It provides high-performance, easy-to-use structures and data analysis tools.
Working with Pandas can significantly enhance productivity due to its user-friendly data structures like series, dataframe and functions for data manipulation like merging, reshaping, slicing etc.
Below is an example where I demonstrate a simple CSV file read operation using pandas:
import pandas as pd # Read the CSV into a pandas data frame (df) df = pd.read_csv('myfile.csv') # View the top data in df print(df.head())
Lastly, never under-estimate the capabilities of the builtin Python library – collections, itertools, functools and more. They come bundled with Python standard-library, and have major contributions towards improving the code readability and execution speed.
The real crux lies in choosing the correct tool for the given task at hand. Often using advanced libraries help in writing cleaner, faster and efficient codes. They come optimized out-of-the-box, suitable for tackling issues related to numerical computations, machine learning, algorithm complexities et al. All one needs is getting familiar with their functionalities and implementing them correctly.Deep diving into machine learning with Python is truly a rewarding journey, riddled with curiosity tweaks and satisfying ah-ha moments. Python stands out among programming languages due to its simplicity, vast community support, and abundant libraries essential for machine learning.
Why Use Python for Machine Learning?
Python continues to rise in popularity in the realm of data science and machine learning for several reasons:
- Readability: Python’s clean syntax makes it easy to read, write, and maintain – perfect for large-scale projects.
- Libraries: Python has expansive libraries (numpy, pandas, matplotlib) that make manipulating data and building models easier than ever before.
- Community: An extensive community sharing tips, tricks, and troubleshooting hacks means you’ll never be stuck for long!
Key Python Libraries for Machine Learning
The key playing libraries when discussing machine learning with Python include:
-
Pandas
: Used for high-level data structures and analysis. It’s perfect for cleaning, transforming, manipulating, and analyzing data.
-
Numpy
: A library for Python, adding support for large, multi-dimensional arrays and matrices, along with an assortment of mathematical functions to operate on these arrays.
-
Matplotlib
: This plotting library creates static, animated, and interactive visualizations in Python.
-
Scikit-learn
: This is the golden library for machine learning in Python! It features various classification, regression, clustering algorithms, and efficient tools for data mining and data analysis.
Walkthrough of Basic Machine Learning Program using Python
Let’s explore a practical example of how these libraries can work together in a simple linear regression problem with Python:
# Import necessary libraries import pandas as pd from sklearn.model_selection import train_test_split from sklearn.linear_model import LinearRegression from sklearn import metrics # Load dataset dataset = pd.read_csv('dataset.csv') # Feature selection feature_cols = ['column1', 'column2', 'column3'] X = dataset[feature_cols] y = dataset['target_column'] # Splitting the Data X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.2, random_state=0) # Model Training model = LinearRegression() model.fit(X_train, y_train) # Predicting the Test set results y_pred = model.predict(X_test)
The code snippet above highlights a simplified process of loading a dataset using
pandas
, pre-processing (featuring splitting), training a linear regression model with
scikit-learn
, and predicting on a test set.
Intermediate and Advanced Machine Learning Techniques
At a more advanced level, Python supports a variety of machine learning strategies:
- XGBoost: An optimized distributed gradient boosting system designed to be highly efficient, flexible, and portable.
- TensorFlow: An end-to-end open source platform for machine learning with a comprehensive, flexible ecosystem of tools.
- Keras: A high-level neural networks API for seamless deep learning with TensorFlow.
In a nutshell, Python’s easy-to-read style, vast collection of libraries, and scalability make it ideal for every stage of your machine learning journey. From simple regression models to complex neural networks, Python provides all the versatility you need to create, experiment with, and deploy machine learning models.If you’re a Python programmer aiming to delve into web development, Django is an optimal choice for your toolkit. Django is a high-level Python framework that encourages clean and efficient design. It follows the DRY (Don’t Repeat Yourself) principle and comes with a vast collection of modules built-in, which can be used to handle regular web development tasks.
One key feature of Django is its Object Relational Mapper (ORM), which simplifies database operations. Instead of writing raw SQL queries, we can use the ORM to interact with the database in a more intuitive way as it supports all the major database systems like MySQL, SQLite, PostgreSQL, etc.
For instance, if you have a Django model representing blog posts:
from django.db import models class BlogPost(models.Model): title = models.CharField(max_length=200) content = models.TextField() pub_date = models.DateTimeField('date published')
With ORM, it’s easy to do CRUD operations on this data:
from django.utils import timezone # creating an object post = BlogPost(title='Hello World', content='My first post', pub_date=timezone.now()) post.save() #picking an object post = BlogPost.objects.get(id=1) #amending an object post.title = 'New Title' post.save() # eliminating an object post.delete()
Also, Django helps handle user authentication right out of the box. This includes user registration, login/logout, password reset, and many others.
Another highly beneficial feature of Django is its automatic admin interface. This allows end-users to manage content on their sites without needing any understanding of how databases work.
As per SEO optimized code, Django uses templates to structure HTML code, avoiding code repetition, making your application lightweight thus boosting loading speed and SEO ranking. Here is an example of a simple Django template:
<!DOCTYPE html> <html> <head> <title>Django Post</title> </head> <body> <h1>{{ post.title }}</h1> <p>{{ post.content }}</p> </body> </html>
You can see usage of curly braces { { } } for variable declarations which makes them dynamic from backend view.
Additionally, Django also supports middleware classes which provide hooks for request/response processing. These classes hold methods executed during every request/response cycle. It’s handy for a variety of actions such as session management, authentication, cross-site request forgery protection, and more.
Above are only some of the sophisticated features that Django offers making it a go-to Python framework for web developers. Coupled with Python’s simplicity, Django greatly reduces the complexity of web development, making the process more enjoyable while maintaining professional standards. Going deeper into Django will make you appreciate its efficiency and what it brings to the table for both novice and pro web developers.If we take a journey through the landscape of Python, it becomes apparent why this language has secured such a dominant position in the world of coding. Coming to the end of our discussion, we don’t actually want to finish without delving into some of the reasons that set Python apart and makes it truly unique in its field.
One of the key attributes of Python that makes it so attractive for coders around the globe is fundamentally its simplicity. Clarity and brevity are at the core of Python’s development philosophy, making it extremely readable and henceforth user-friendly. When coding in Python, the syntax feels natural with an easy to comprehend structure that lowers the entry barrier for newbie coders.
def hello(): print("Hello, World!") hello()
In the code snippet above, even a non-programmer can guess that this script prints “Hello, World!” on the screen. This is a testament to Python’s readability and how inherently intuitive it is.
Another charm of Python lies in its versatility. This bears out as Python finds use across a multitude of fields – be it web development, machine learning algorithms, or data analysis – the list is endless. However, one common feature in these fields is data handling, be it In Pandas DataFrame in Data Analysis or Numpy arrays in Machine Learning. Each of these libraries make Python an all-rounder coding language.
A critical factor lending itself to widespread adoption to Python can be attributed to vast standard library and supplementary module availability. This allows developers to avoid rewriting many common tasks and instead rely on tested, reliable Python libraries that can increase efficiency and reduce time to market. Following is a simple Python script using requests from Python standard library to fetch HTML content from a URL:
import requests response = requests.get('https://www.python.org') print(response.text)
This script showcases just how straightforward it is to pull webpage contents with just a few lines of code. All due to the power-packed modules present in Python’s robust ecosystem.
Moreover, Python’s active community provides essential support to any Python developer facing issues or bugs in their code. They provide resources like documentation, tutorials, and forums that assist in resolving problems more conveniently.
However, no programming language is perfect, so does Python. It might not be ideally suited for applications requiring high speed or dealing with complex calculations because Python is relatively slower than languages like C++ or Java.
Given these points, Python still carries the day in most scenarios due to its strengths significantly outweighing its weaknesses. Python’s blend of simplicity, versatility, and a vibrant ecosystem makes it an attractive choice for many developers.
To further explore Python’s capabilities and delve deeper into mastering Python, consult the official Python Documentation, where extensive tutorials, guides, and references are available.
Python, as a language, continues to evolve while maintaining its roots firmly planted in simplicity, making it a powerful tool that will undoubtedly continue to shape and influence the world of programming.