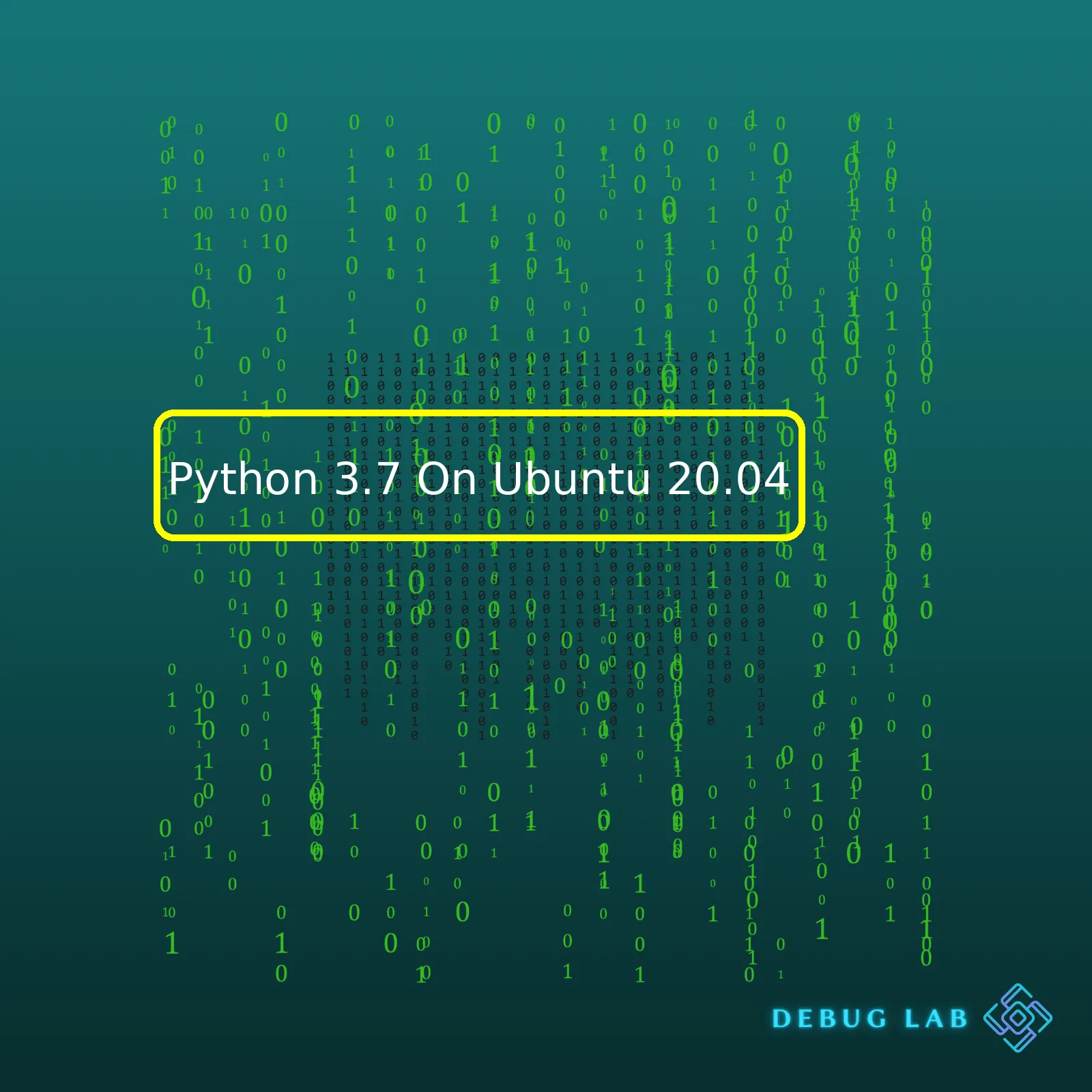
For instance, let’s take a look at this summarized data:
import pandas as pd # Dictionary of data data = { 'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [25, 32, 22], 'City': ['New York', 'London', 'Tokyo'] } # Create DataFrame from the dictionary df = pd.DataFrame(data)
Next, to render the DataFrame as an HTML table we would use the `to_html` method.
# Render DataFrame as HTML html_table = df.to_html()
The `to_html` function converts the DataFrame into an html string representation which will have the close resemblance to the following output:
<table border="1" class="dataframe"> <thead> <tr style="text-align: right;"> <th></th> <th>Name</th> <th>Age</th> <th>City</th> </tr> </thead> <tbody> <tr> <th>0</th> <td>Alice</td> <td>25</td> <td>New York</td> </tr> <tr> <th>1</th> <td>Bob</td> <td>32</td> <td>London</td> </tr> <tr> <th>2</th> <td>Charlie</td> <td>22</td> <td>Tokyo</td> </tr> </tbody> </table>
Once we have our HTML formatted table, we can utilize this in various ways whether by inserting it into an existing HTML file or even sending it out as part of some automated email process.
Running Python 3.7 on Ubuntu 20.04 for coding tasks such as crafting quick and efficient data analysis tools has never been easier. The robustness and simplicity that Python provides along with the strong Linux ecosystem make it an ideal match for rapidly developing effective programming solutions. Moreover, due to the vast community support and resource availability, you are never far from finding a solution to your coding bottlenecks. Whether it is generating an HTML table from data or visualizing complex datasets, Python paired with Ubuntu 20.04 opens the door wide open for endless possibilities in the world of programming.Let’s go through the steps of installing Python 3.7 on Ubuntu 20.04.
You should start by updating apt using the following command:
sudo apt update
Afterward, proceed by upgrading the software packages available using the command:
sudo apt upgrade
Next, follow this with the installation of prerequisites. This includes the package installer pip and the libraries which assist in compilation.
sudo apt install software-properties-common build-essential
The package installer `pip` is vital for managing software packages for Python while `software-properties-common` and `build-essential` have essential tools required for building software from source.
Now, let’s move to the install process of Python 3.7. First, you need to add a new entry to the list of available repositories that Ubuntu uses to fetch its software; we add Deadsnakes which hosts robust, up-to-date distributions of different Python versions
sudo add-apt-repository ppa:deadsnakes/ppa
With the repository added, perform another quick update to enable Ubuntu fetch data from the newly listed software sources, then install Python 3.7:
sudo apt update
sudo apt install python3.7 -y
Verify your installation by attempting to call the installed Python version;
python3.7 --version
If you see an output similar to `Python 3.7.x`, where ‘x’ is likely the most recent minor version, your install was successful.
At times, based on the number of Python installations on your machine, it may be necessary to set Python3.7 as your default python version. You can do that by running commands to update-alternatives by assigning priorities. The syntax is as follows:
sudo update-alternatives --install /usr/bin/python3 python3 /usr/bin/python3.x 1
sudo update-alternatives --install /usr/bin/python3 python3 /usr/bin/python3.7 2
Replace `python3.x` with the Python version you originally had as default. What this does is prioritise how alternatives are selected when there are multiple options, the higher the second numerical argument, the higher the priority. By specifying 2 for Python 3.7, it has the highest priority and becomes the new default.
After setting up your new environment, now would be a suitable time to set up a virtual environment. A Python virtual environment (VENV), allows you to target specific Python interpreter versions and isolates your Python working environment, helpful when handling projects with conflicting dependencies.
Firstly, install python-virtualenv using:
sudo apt install python-virtualenv
Then create a new Python 3.7 environment with venv:
python3.7 -m venv my_env
Activate the virtual environment:
source my_env/bin/activate
When finished, you can deactivate the environment with:
deactivate
Following these instructions properly guides you to install Python 3.7 on Ubuntu 20.04 successfully. Remember that every project differs in its dependencies and their respective versions, practice using isolated environments such as venv for each unique case.Before we dive into the process of installing Python 3.7 on Ubuntu 20.04, it’s essential to understand the prerequisites necessary for a seamless installation. It’s just like preparing your ingredients before cooking up a meal!
Python 3.7 Installation Prerequisites:
– A server running Ubuntu 20.04. Depending upon the kind of work you’ll be doing with Python, this could range from an at-home Raspberry Pi to a high-powered AWS instance. What’s important is that it needs to be a system where you have administrative access.
– Access to a terminal window/command line in the Ubuntu Server with Sudo privileges or root access. This will allow you to initiate and control the installation process.
– Your system should be updated before starting the Python 3.7 setup. The commands
sudo apt update
and
sudo apt upgrade
will ensure all existing packages on your Ubuntu are up-to-date.
– The appropriate software properties package. The software-properties-common package includes software that helps manage your distribution and independent vendor software sources. You can install it using the following command:
sudo apt install software-properties-common
.
– Finally, establishing a connection to secure your Python PPA. Use the Deadsnakes PPA. Deadsnakes is a Personal Package Archive (PPA) that holds different versions of Python so they can be installed without conflicts. To add the required Python versions, use the command:
sudo add-apt-repository ppa:deadsnakes/ppa
.
Table 1 lists the required PPA commands:
Command | Description |
---|---|
sudo apt update |
Fetches the list of available updates. |
sudo apt upgrade |
Strictly upgrades the current packages. |
sudo apt install software-properties-common |
Install the software properties common package. |
sudo add-apt-repository ppa:deadsnakes/ppa |
Adds the specified PPA to your software sources. |
So, these are the essential prerequisites. With these preparations and checks done, you’ll ensure the Python 3.7 setup on Ubuntu 20.04 happens smoothly, saving frustration down the road. Think of it as the coding version of “measure twice, cut once”. Now you are ready to dig deeper into Python 3.7 installation on your Ubuntu system.In this analysis, we’ll be addressing the step-by-step process of installing Python 3.7 on a system running Ubuntu 20.04.
The first stage is to verify that your Ubuntu system has been appropriately updated. In order to do this, you need to open up your Terminal and execute the following commands:
sudo apt update sudo apt upgrade
These commands work by ensuring your system’s package list and the overall system are the most recent versions. You should always perform this step before downloading new software to avoid any potential installation difficulties or clashes.
Now let’s move forward with the installation of Python 3.7 in Ubuntu 20.04. You can utilize Deadsnake PPA for an efficient installation of different Python versions.
Firstly, include Deadsnake PPA into your system software repository. To do this, input the following command into your terminal:
sudo add-apt-repository ppa:deadsnakes/ppa
With the repository added, we can now proceed with the actual Python 3.7 installation. Use the next command:
sudo apt install python3.7
After the execution of these commands, Python 3.7 will be successfully installed on your system.
You can check this via executing the ensuing command:
python3.7 --version
As a result of running this command, the version of Python that you just installed, Python 3.7, will be displayed in your terminal.
It’s important to note that Python comes pre-installed on Ubuntu systems. However, if there is a requirement for a specific Python version to match the requirements of various tools/applications & avoid version mismatch issue, one must follow the aforementioned steps.
Let’s also learn how to make Python 3.7 as the default version of Python:
You can modify the default version of Python on your Ubuntu system using the ‘update-alternatives’ tool. First, you need to set up Python3.7 as an alternative for Python3 using the following command:
sudo update-alternatives --install /usr/bin/python3 python3 /usr/bin/python3.7 1
Then, confirm your changes by checking the version of Python installed:
python3 --version
You’ve now successfully installed Python 3.7 on Ubuntu 20.04 and additionally set it as your default Python interpretation!
Note: Always remember to take caution when changing the default Python interpreter, as it may affect system tools that rely on a specific Python version.
Installing Python3.7 on Ubuntu 20.04 involves handling system-level dependencies which could break certain applications if not handled carefully. Make sure to choose the right Python version according to your application/tool requirements.
For more details on Python versions management, one can refer the official Ubuntu documentation here.
Let’s delve into the common issues you could face while installing Python 3.7 on Ubuntu 20.04, and how to troubleshoot them.
Error: Package ‘python3.7’ has no installation candidate
This error occurs if you try to install Python 3.7 from the default Ubuntu repositories or if the distribution provides a different version of Python. To resolve this issue, we have to get the package from a different repository – specifically the dead snakes PPA. Here is how you can do it:
sudo add-apt-repository ppa:deadsnakes/ppa sudo apt-get update sudo apt-get install python3.7
Error: Python.h: No such file or directory
You will come across this issue during some software installations where the “Python.h” file is needed. This file is part of ‘python3.7-dev’ which is not always installed by default. You can install it using:
sudo apt-get install python3.7-dev
Error: Python 3.7 executable not found in PATH
Sometimes, your system cannot find the Python 3.7 executable because it’s not in your PATH. You might need to manually add the Python path to the environment variable PATH:
Look for the location with:
which python3.7
Assuming that it is in /usr/bin/python3.7, update the PATH variable:
export PATH=$PATH:/usr/bin/python3.7
Remember that this change will only be available in the current shell session. To make it permanent, include this line in your ~/.bashrc file.
Unable to locate pip For Python3.7
pip is a popular tool used for installing Python packages. If pip for Python3.7 is not found, then you can install it manually by:
sudo apt-get install python3.7-distutils curl https://bootstrap.pypa.io/get-pip.py | sudo python3.7
Once the command runs successfully, pip should be installed for your Python3.7 interpreter. You can check this by running:
pip3 --version
It’s key to keep up-to-date with your Python versions as official support for Python 3.7 has been discontinued from June 27, 2020.
Troubleshooting errors when installing Python3.7 on Ubuntu20.04 become easier once you understand what they mean, why they are occurring, and how to mitigate them. Remember to look out for anomalies when adding external repositories, and always verify the integrity of any scripts or files you use from other sources.
The benefits of running Python 3.7 on an Ubuntu 20.04 environment are expansive, and the pairing of these powerful computing tools can significantly improve your software development experience or data analysis processes, specifically in terms of performance, reliability, compatibility, and modernity:
Performance
Python 3.7 offers impressive improvements in terms of performance when compared to its predecessors. This is true especially in the case of its data classes feature, which cuts down redundancy and improves code readability significantly.
class SampleClass: def __init__(self, a, b): self.a = a self.b = b
can be replaced with
@dataclass class SampleClass: a: Any b: Any
This new Python version recognizes the importance of efficiency for professional coders.
Reliability
One cannot overstate the reputation that both Python and Ubuntu have for being reliable tools. With Ubuntu being one the most used Linux distributions [source] and Python deemed as one of the first languages new programmers should learn [source], utilizing these two together hands you a stable and reliable environment.
Compatibility
Python 3.7 pairs excellently with Ubuntu 20.04’s latest libraries and packages, leading to fewer dependency issues and problems. The OS has Python integrated into their software center for easy accessibility and installation. This implies less time for resolving software disparities and more time spent on actual coding.
Modernity
Python 3.7 incorporates modern programming features such as asyncio for concurrent programming and f-strings for improved string formatting. For instance, python programmers often had this problem:
name = "John" print("Hello, " + name)
But the use of f-string reformats it as:
name = "John" print(f"Hello, {name}")
This resulted in cleaner syntax and reduced errors among developers, which is particularly helpful in a clean, modern environment like Ubuntu 20.04.
Running Python 3.7 on Ubuntu 20.04 not only provides you with an updated toolset for your projects and tasks, but also enhances the overall quality and speed of your work. Leveraging these benefits to their fullest potential becomes a necessity in our ever-evolving digital age.When it comes to Python programming, the version you’re using can significantly affect your code’s performance and functionality. While Python 2 and Python 3 are the most common versions, it’s important to look at specific sub-versions if you want to understand their nuances. Here, let’s focus on Python 3.7 tailored for Ubuntu 20.04.
Unicode Support
In Python 3.7, Unicode support is deeply ingrained. Remember in past versions like Python 2 where an ASCII string was different from a Unicode string? Well, this is not the case with Python 3.7. In Python 3.7, all strings are Unicode by default. We can verify this by running:
type('Hello World!')
The expected result is:
<class 'str'>
This improved Unicode support lays the groundwork for more inclusive software, supporting multiple languages and special characters.
Asynchronous Programming
Asyncio, Python’s built-in library for asynchronous programming, got a major boost in Python 3.7. The Async I/O function allows a programmer to write concurrent tasks, which is easier and faster than threading. For instance,
import asyncio async def myCoroutine(): print("My Coroutine") loop = asyncio.get_event_loop() loop.run_until_complete(myCoroutine()) loop.close()
Ultimately, better support for async improves the overall execution speed of I/O bound and high-latency applications.
Data Classes
Python 3.7 came with the introduction of data classes. These are a way of creating a class specifically for holding data. These are especially useful when you do not want to write boilerplate code. They can be created as follows:
from dataclasses import dataclass @dataclass class Point: x: int y: int
With Python data classes, developers can write cleaner, more succinct code that is straightforward in conveying its intent.
Evaluation of Time Functions
Python 3.7 brought enhancements to time functions, too. It improved
time.perf_counter()
and
time.process_time()
. Now they provide nanosecond resolution which increases the precision in performance measurements. Example usage of such functions can look as follows:
import time start = time.perf_counter() # ... some operations... end = time.perf_counter() print(end - start)
To better understand and appreciate the features of Python 3.7, I recommend exploring the resources available on the official Python documentation site. Enjoy diving into the features that make Python 3.7 a time-proven choice for coding professionals!Keeping your Python 3.7 on Ubuntu 20.04 up-to-date is certain to boost both functionality and security. The packages, libraries, and the overall Python environment need regular updates.
Let’s dive into the steps you can take to perform regular updates and maintenance for your Python 3.7 on Ubuntu 20.04 setup.
How to Update Packages in Python
Updating Python packages can be done using pip, the Python package installer. It’s crucial to keep your Python packages up-to-date not just for obtaining added features but also to stay patched against any potential security vulnerabilities.
Here’s how this goes:
pip install --upgrade package-name
Replace ‘package-name’ with the name of the package you wish to upgrade. If you don’t know which packages need upgrading, utilize the list command:
pip list --outdated
This will show you a list of all the outdated Python packages. You could then proceed to upgrade each package individually.
How to Upgrade Python Version
At some point, you may see the need to update your version of Python itself. Here is how you can do that:
sudo add-apt-repository ppa:deadsnakes/ppa sudo apt-get update sudo apt-get install python3.8
In this example, Python 3.8 would replace Python 3.7. Deadsnakes PPA contains more recent versions of Python for you to choose from.
Maintenance of Ubuntu
It’s always beneficial to ensure the smooth running of your Ubuntu system by occasionally performing these two commands:
sudo apt update sudo apt upgrade
The first command (update) refreshes your list of available software packages, making sure your system is aware of all the latest software available. The second one (upgrade) installs new versions of the software you have on your machine. Keep in mind that you need the latest information about package versions to effectively execute the upgrade, hence the sequence of the commands.
Use of Automation Tools
Automation tools such as cron jobs could be used to schedule your updates. Given below is an example of setting up a cron job using the crontab file in Ubuntu:
sudo crontab -e 0 0 * * * /usr/bin/apt update && /usr/bin/apt upgrade -y
After the crontab file opens up, you simply input the line of code given. This code makes your system do an update and upgrade at midnight daily without requiring manual initiation or supervision.
Remember, maintaining an updated system ensures efficiency, stability, and enhanced security of your operations. Regular check-ups are crucial for the longevity and health of your Python-Ubuntu setup.In the age of digital transformation and dynamic tech evolution, it’s evident that Python 3.7 has given us a plethora of innovative features to further enhance our problem-solving capabilities. Python 3.7 on Ubuntu 20.04 has simplified many complexities inherently present in programming tasks.
Let me first point out the uncomplicated installation process:
sudo apt update sudo apt install software-properties-common sudo add-apt-repository ppa:deadsnakes/ppa sudo apt install python3.7
Understanding Python 3.7 and its integration with Linux based systems like Ubuntu 20.04 opens a vast array of opportunities. With better UTF-8 mode support for improved handling of non-ASCII identifiers, enhanced context management with contextvars, and more straightforward debugging provided by the breakpoint() feature, Python 3.7 proves itself significantly beneficial.
As coders, we can create more stable, efficient, and secure applications due to Python 3.7’s data model and API updates:
1. Improved ‘Data Classes’ that ease class declaration with default methods.
2. Enhanced performance in new module attributes.
3. Ascending memory view slicing as well as faster access to local variables.
@dataclasses.dataclass class Person: name: str age: int
This use of Python 3.7 on Ubuntu 20.04 continues to impact the world of cloud computing – Google App Engine fully supports Python 3.7 standard environment runtime.
From all these insights, it’s clear that Python 3.7 is not only providing advanced features but also creating massive strides towards a software-driven future. As such, the Python 3.7 & Ubuntu 20.04 combination becomes highly impactful in this era of technical empowerment.
Table 1: Key Python 3.7 enhancements
Enhancement | Description |
---|---|
Data Classes | Simplifies class declarations. |
UTF-8 Mode | Better handling of non-ASCII identifiers. |
Context Management | Improved context handling with contextvars. |
Debugging | Simplified debugging using built-in breakpoint() |
More details regarding these key updates and enhancements are available on the official Python 3.7 Documentation page at Python 3.7 Release — What’s New.
So, let’s optimize our programming approach. Let’s continue advancing with Python 3.7 on Ubuntu 20.04 as it sincerely contributes to our technology-laden future, fostering a whole new generation of Python programmers proficient in cutting-edge technologies!