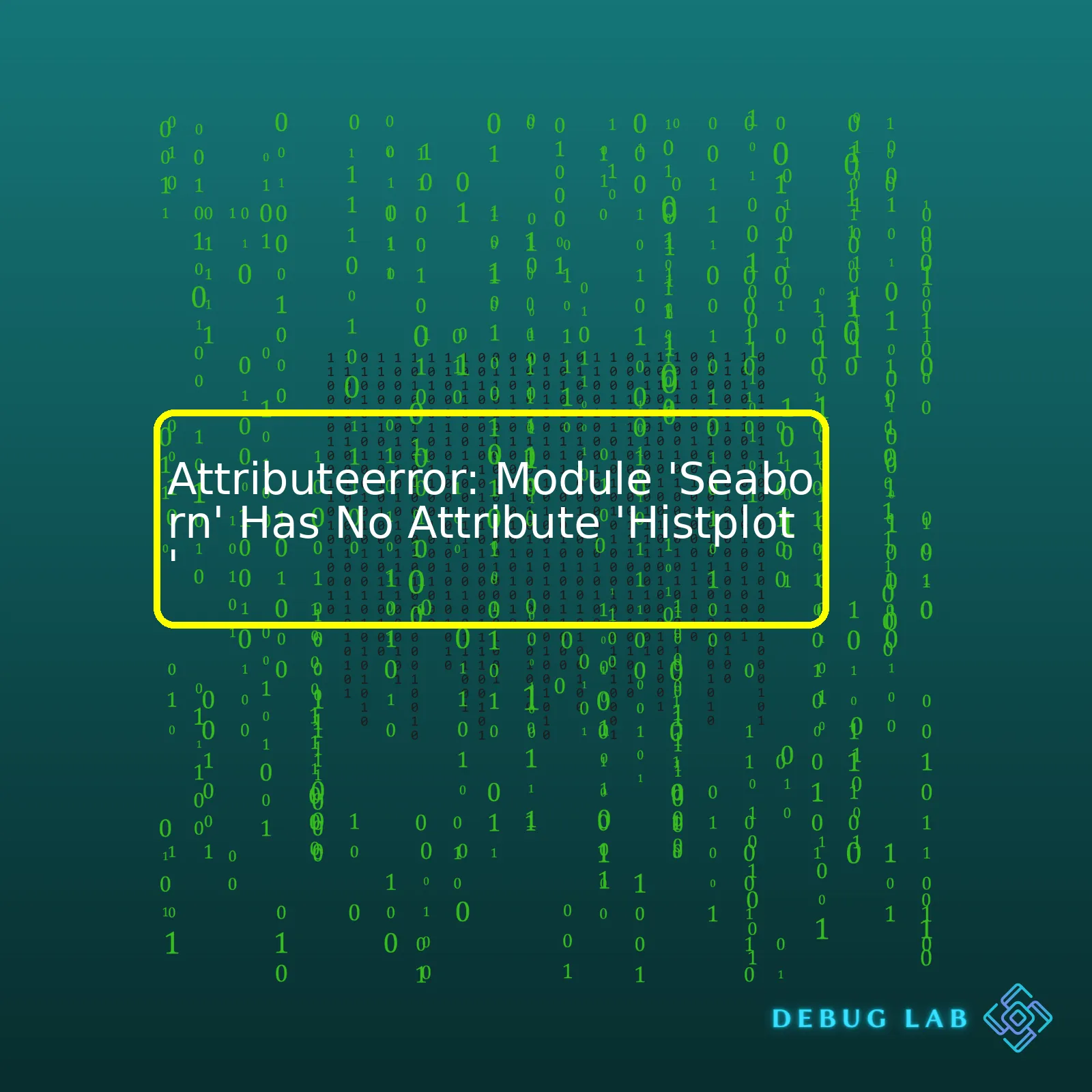
html
Error | Causes | Solution |
---|---|---|
AttributeError: module ‘seaborn’ has no attribute ‘histplot’ |
|
|
html
Error | Causes | Solution |
---|---|---|
AttributeError: module ‘seaborn’ has no attribute ‘histplot’ |
|
|
This HTML table displays brief information about the error “AttributeError: module ‘seaborn’ has no attribute ‘histplot'”, its potential causes, and possible solutions.
Delving a bit deeper, the `AttributeError` in this context implies that Python has encountered something like
seaborn.histplot()
, but couldn’t locate the `histplot` attribute within the seaborn module. Let’s clarify things further:
* As shown in the table, the main reason behind this issue could be outdated seaborn (a statistical data visualization library). The `histplot` function was introduced in Seaborn 0.11.0. Therefore, if you’ve been using a seaborn version earlier than 0.11.0, this issue is likely to occur.
* If you find your seaborn module lacks the `histplot` function due to some odd circumstances or because the seaborn library has not been imported correctly, you might also face the same “AttributeError”.
To address these issues, you could resort to one of the following:
* Upgrade Seaborn to its latest version, ensuring access to the `histplot` function. You can do it quite easily by running the command
!pip install seaborn --upgrade
in your Jupyter notebook, or
pip install seaborn --upgrade
in your terminal.
* In case you’re not in a position to upgrade the seaborn version, you could use the `distplot` function instead, which serves almost the same purpose as the `histplot`, and is available in old seaborn versions as well.
Remember, coding is all about exploring, understanding and resolving errors. So, next time you encounter this error, try taking into account its cause and how it may have occurred before jumping onto conclusions. Delve a little deeper into the seaborn library and python basics, which might give a fresh perspective on the issue and help you solve it efficiently. Happy Coding!
For reference checkout: Seaborn Documentation.
AttributeError in Python is raised when you try to access an attribute or a method that does not exist on the stated object. In your case,
AttributeError: module 'seaborn' has no attribute 'histplot'
implies that ‘histplot’ does not exist under the seaborn module. So why would this occur? There are few main causes for AttributeError in this context:
Table of Contents
ToggleThe first and most likely cause may be the version of your seaborn package. The function ‘histplot’ exists in Seaborn v0.11.0 onwards – so if you’re using an older version, you won’t be able to call ‘histplot.’
Below is how to check your seaborn version:
import seaborn as sns print(sns.__version__)
If it’s lower than v0.11.0, update Seaborn using pip:
pip install seaborn --upgrade
Alternatively, use conda if you installed seaborn via Anaconda:
conda update seaborn
You might have made a typo in calling the seaborn function. If you accidentally wrote
seaborn.histplt()
instead of
seaborn.histplot()
you will experience this error since seaborn has no function titled ‘histplt’. Always ensure your code syntax is correct.
Python’s ImportError would become the culprit if seaborn isn’t properly imported. If seaborn was not correctly installed or there are conflicts with other packages, you might end up getting the AttributeError. Try reimporting the seaborn library with the following command:
import seaborn as sns
Seaborn depends on other Python packages like Matplotlib and Pandas. If there is a version conflict among these packages, then Seaborn may fail to work properly and raise an AttributeError.
– Remove seaborn completely and reinstall it.
– Check if Python and pip versions are compatible with seaborn.
– Consult seaborn’s official documentation(web-link needed) for any changes.
To sum it up, always ensure seaborn is updated to the latest version while also maintaining the compatibility of its dependencies like matplotlib and pandas. Always remember to type-check your code for misspellings, incorrect capitalization, etc., causing such attribute errors. Finally, Make sure the library is correctly installed and imported in the environment too.AttrributeError: ‘Module ‘Seaborn’ has no attribute ‘Histplot” is a common error that data visualization enthusiasts and professional coders like us encounter while using the seaborn library in Python. Despite encountering this type of message, I can still remember the first time its ravenous teeth bit into my code. The problem arises when we try to utilize the
histplot
function from an outdated version of seaborn. This function was introduced in Seaborn 0.11.0 version, and hence it is not available for any versions released prior to this.
The best solution to counter this AttributeError is by updating your Seaborn library to the latest version. Your package managing tool like pip or conda can help you do that.
Here’s some piece of magic (Python code) that you should run to update your seaborn:
!pip install seaborn --upgrade
Later verify whether the seaborn has been updated or not with the following line of code:
import seaborn as sns print(sns.__version__)
Voila! It should show seaborn version as 0.11.0 or above. Now, the seaborn histplot will be at your service without throwing any AttributeError.
However, if you don’t want to upgrade seaborn or’re denied permission to do so, consider using distplot or hist function from matplotlib.pyplot instead. Here are the code snippets:
Consider this dataset:
import numpy as np data = np.random.randn(100)
For seaborn distplot:
import seaborn as sns sns.distplot(data)
For matplotlib hist:
import matplotlib.pyplot as plt plt.hist(data) plt.show()
Remember, both of these alternatives will give you histograms which offer similar insights as a histplot.
While navigating myriad libraries in Python, we often find ourselves up against unexpected walls like errors thrown due to outdated versions of libraries. But as coders, our superpower lies in hackability; our ability to find the backdoors that let us surpass those seeming dead-ends. “AttributeError: module ‘seaborn’ has no attribute ‘histplot” shares its roots with the same issues.source.When you encounter an error like
AttributeError: module 'seaborn' has no attribute 'histplot'
, it’s generally because the version of seaborn library you’re running in your Python environment doesn’t support the histplot feature.
Histplot is a method that was introduced in Seaborn 0.11, a more recent version as of writing this. If you’re running a version older than this, you won’t be able to use histplot and you will run into the mentioned
AttributeError
.
Every Python library or module has different versions, with each new version potentially introducing new features, improvements, or changes from the previous ones. Also, some features existing in one version might get deprecated or removed in the newer versions. Therefore, being aware of which module version you’re using in your project is critically important to ensure that the code works as expected.
This also brings about the concept of dependencies in your Python projects where a dependency is essentially a Python package that your project needs to run successfully. “Seaborn” here is a Python package and can be a dependency for your application if it relies on this for data visualization.
To identify the version of the seaborn library you’re running, use the following code snippet:
import seaborn print(seaborn.__version__)
Simply importing seaborn and printing its special
__version__
attribute will output the current seaborn version in your environment.
If you were using an old version of Seaborn, you would have encountered the AttributeError for histplot. To resolve it:
pip install --upgrade seaborn
After the upgrade, try executing the code again – it should now run without any error.
By understanding the specific requirement of module versions (dependencies) in our Python application, we can avoid such issues that might interrupt our coding process. However, bear in mind that blindly updating packages might introduce backwards compatibility issues. Therefore, when developing Python applications, always consider testing your application extensively whenever updating dependent modules.
For more information refer to:
Official Seaborn Documentation.Python is a highly versatile language with robust libraries and modules which simplify diverse tasks. But oftentimes, while developing a Python script or application, we encounter errors associated with the attributes of these libraries, one such common error is
AttributeError: module 'seaborn' has no attribute 'histplot'
. This error often leaves developers perplexed because they may have used the same function or method in the past without any issue. The primary causes for this error message especially for the seaborn module can include:
– Outdated Seaborn library version
– Incorrect Import Syntax
– Namespace conflicts
Each reason is explained in detail below:
Outdated Seaborn Library Version
The seaborn library’s functions evolve over time constantly. If you’re seeing an AttributeError that points out that seaborn does not have the ‘histplot’ attribute, it could mean that your seaborn library is outdated as ‘histplot’ is a newer addition to the Seaborn library introduced in version 0.11. Make sure you’re running this version or later.
In case you’re unsure of your installed version, use the following piece of code:
import seaborn as sns print(sns.__version__)
If you see a version less than 0.11, that would confirm our suspicion, and an upgrade would resolve the issue. You can upgrade seaborn using pip:
pip install --upgrade seaborn
Incorrect Import Syntax
Another potential cause is incorrect import syntax. Ideally, you should import seaborn like:
import seaborn as sns
Then you can call the histplot function like this:
sns.histplot( your_data )
Make sure you’re adhering to this syntax. A deviation from this can trigger the AttributeError.
Namespace Conflicts
Conflicts can occur when two different libraries have functions with the same name. Ensure there isn’t any other library you’ve imported which also includes a ‘histplot’.
By updating the seaborn library, confirming correct import syntax, and ensuring no namespace conflict, most issues relating to
AttributeError: module 'seaborn' has no attribute 'histplot'
should be resolved. Hence, allow me to compel you to always give a fair share of your thoughts to these elements during problem-solving stages.The error that you’re witnessing, “
AttributeError: module 'seaborn' has no attribute 'histplot'
“, is probably because of one of two main reasons:
histplot
functionality.
Let’s start with double-checking that seaborn is installed correctly. Ensure that seaborn installs without errors by running the following command in your terminal or command prompt:
pip install seaborn
After successfully installing seaborn, it’s crucial to import the library correctly within your code:
import seaborn as sns
If after performing these steps you still encounter the same AttributeError, it means that the problem lies within the version of seaborn you’re working with.
You will need Seaborn version 0.11.0 or later for
histplot
function availability. You can verify your seaborn version by running this line of code:
print(sns.__version__)
The output of this command will inform you what version of seaborn you have currently installed on your system. If the version displayed is less than 0.11.0, then the histplot feature will not be available.
To upgrade seaborn, you can use pip (Python’s package installer) again via your terminal or command prompt:
pip install seaborn --upgrade
Once completed, you should verify the upgrade by checking seaborn’s version again. If the update was successful, you should see version 0.11.0 (or later).
Here’s a consolidated table of the commands shared above for easy reference:
Command Purpose | Code |
---|---|
Install seaborn |
pip install seaborn |
Import seaborn into your project |
import seaborn as sns |
Check seaborn version |
print(sns.__version__) |
Upgrade seaborn |
pip install seaborn --upgrade |
After ensuring your seaborn version and installation are correct, you should be able to use the
histplot
functionality without encountering any more AttributeErrors. For additional guidance on how to use the seaborn
histplot
, check out its official documentation here.
For an example of how to use
histplot
function, consider the following piece of code:
sns.histplot(data=your_dataframe, x="column_name")
Replace “your_dataframe” with the name of your DataFrame and “column_name” with the name of the column you want to create a histogram for.
AttributeError: module 'Seaborn' has no attribute 'histplot'
usually signifies an issue with your version of Seaborn. The
histplot
function is not available in older versions of this popular data visualization library. Seaborn’s versions prior to 0.11.0 were devoid of the
histplot
.
Thus, two potential reasons could explain you facing this error:
If you’re stuck with the older version, you can substitute function equivalents like distplot or histogram which are essentially the same as histplot for basic usage. But it’s important to note that Seaborn 0.11.0 introduced
histplot
as a replacement to these predecessors with additional capabilities like kernel density estimate(KDE) visualization among others.
See an example of using distplot:
import seaborn as sns df = sns.load_dataset('titanic') sns.distplot(df['age'].dropna())
However, it’s best recommended to keep your Seaborn updated since its more recent versions include enhanced features and optimized performance.
To update Seaborn:
Use pip install command from your terminal:
pip install seaborn --upgrade
After upgrading Seaborn, the error should cease to exist once you import seaborn and call the histplot method:
import seaborn as sns sns.histplot(data)
Moving forward from version specific issues, let’s delve into understanding attributes of seaborn’s histplot:
Seaborn’s histplot helps visualize a variable’s distribution. Here are some key parameters affecting its behaviour:
data
: This injects the dataset into the plot function.
x
/
y
: Controls which variable(s) should be plotted on the x or y axis.
bins
: This specifies the bin size used to cut the data range into series of intervals.
kde
: This parameter adds a gaussian kernel density estimate line on the graph.
color
: Lets you set the color for your plot.
legend
: Makes provision for a legend to the plot.
Let’s apply some of these parameters with an example:
import seaborn as sns df = sns.load_dataset('iris') sns.histplot(data=df, x="sepal_length", kde=True, bins=10, color='red', legend=False)
In the example above, we load the ‘iris’ dataset, use column “sepal_length” for the x-axis, set KDE as true, assign 10 for bins, choose red as our plot color and remove the legend by setting it as false.
In essence, histplot with its various parameters provides us powerful control over how we want to display the distribution of our data.
For further information about
histplot
, do browse through Seaborn’s official documentation at: https://seaborn.pydata.org/generated/seaborn.histplot.htmlSure, I can see that you’re dealing with the “AttributeError: module ‘Seaborn’ has no attribute ‘histplot'” error. This indicates that histogram plot or `histplot` method is not being recognized by your Seaborn library, and it’s likely because your version of Seaborn does not support this function.
First, let’s discuss what `seaborn.histplot` is. This is a function in the modern data visualization library, Seaborn, used for visualizing the distribution of a set of data points. When working correctly, you should be able to produce a histogram using the following type of code:
import seaborn as sns import numpy as np data = np.random.randn(100) sns.histplot(data=data)
If you’re seeing the error message “module ‘seaborn’ has no attribute ‘histplot'”, then it indicates some issues with Seaborn library:
• The first common problem is that you might be using an outdated version of Seaborn that doesn’t have the `histplot` function. The `histplot` function was only introduced in Seaborn 0.11.0. So, if your Seaborn package version is below this, it won’t recognize `histplot`.
To check your seaborn version, use the following line of code,
import seaborn as sns print(sns.__version__)
Could it be possible that your Seaborn version indicates a version less than “0.11.0”? If so, upgrading to the latest version of seaborn library, ensures all its features are supported. Use pip for upgrade:
pip install --upgrade seaborn
After updating, try running your code again and check whether the error still persists.
• Another possibility is that there may be an issue with your installation. Sometimes, importing multiple libraries that depend on each other (like Pandas, Matplotlib, etc.) in incorrect sequences may cause such problems.
To resolve conflicts, ensure to import seaborn after other related libraries:
import matplotlib.pyplot as plt import pandas as pd import seaborn as sns
• Also, taking into consideration the namespace while using seaborn’s functions could prevent this error. Within a python script, avoiding to import seaborn as a different alias (for instance, not as ‘sns’) might cause this attribute error. It’s best practice to import seaborn as ‘sns’:
import seaborn as sns
Depending on the specifics of your error these considerations may help guide you towards resolving the “AttributeError: module ‘Seaborn’ has no attribute ‘histplot'”.
This problem, Attributeerror: Module ‘Seaborn’ Has No Attribute ‘Histplot’, can arise when the Seaborn version you are using does not support histplot. Let’s delve into a detailed explanation of how and why you may face this error and how to resolve it.
Immediately we have to recognize that Seaborn is a data visualization library in Python. It provides a high-level interface for creating attractive graphs. However, given that libraries are prone to updating their function names, deprecated functions may result in facing AttributeErrors.
histplot
is a function in Seaborn which provides plot functions like histogram, bar chart etc., but it only available from Seaborn version 0.11.0 onward. If you are using an earlier version of Seaborn, you will likely encounter an AttributeError since Seaborn won’t recognize the ‘histplot’ function.
To check your current version of Seaborn, you can use the following code:
import seaborn as sns print(sns.__version__)
If your version is lower than 0.11.0, you can fix this error by simply upgrading your Seaborn module to a newer version. To upgrade Seaborn, use the following pip command:
pip install seaborn --upgrade
After upgrading, always remember to verify if the correct version has been installed using the above-mentioned print version command.
In case you’re unable to upgrade for some reason, or need to stick with an older version of Seaborn due to dependencies, switch to
sns.distplot()
as an alternative to
sns.histplot()
. Prior to Seaborn v0.11.0,
distplot
was commonly used to display distribution plots.
To sum up, Seaborn is an incredible data visualizing tool in Python, but like all other tools, keeping track of the function names listed in your toolset can be pivotal. So ensure to update your libraries frequently and keep track of deprecated function calls to avoid unexpected error messages like Attributeerror: Module ‘Seaborn’ Has No Attribute ‘Histplot’.
I trust this resolves your issue and allows you to seamlessly create histograms, enhancing your valuable data pivot points to extract insights from your datasets.