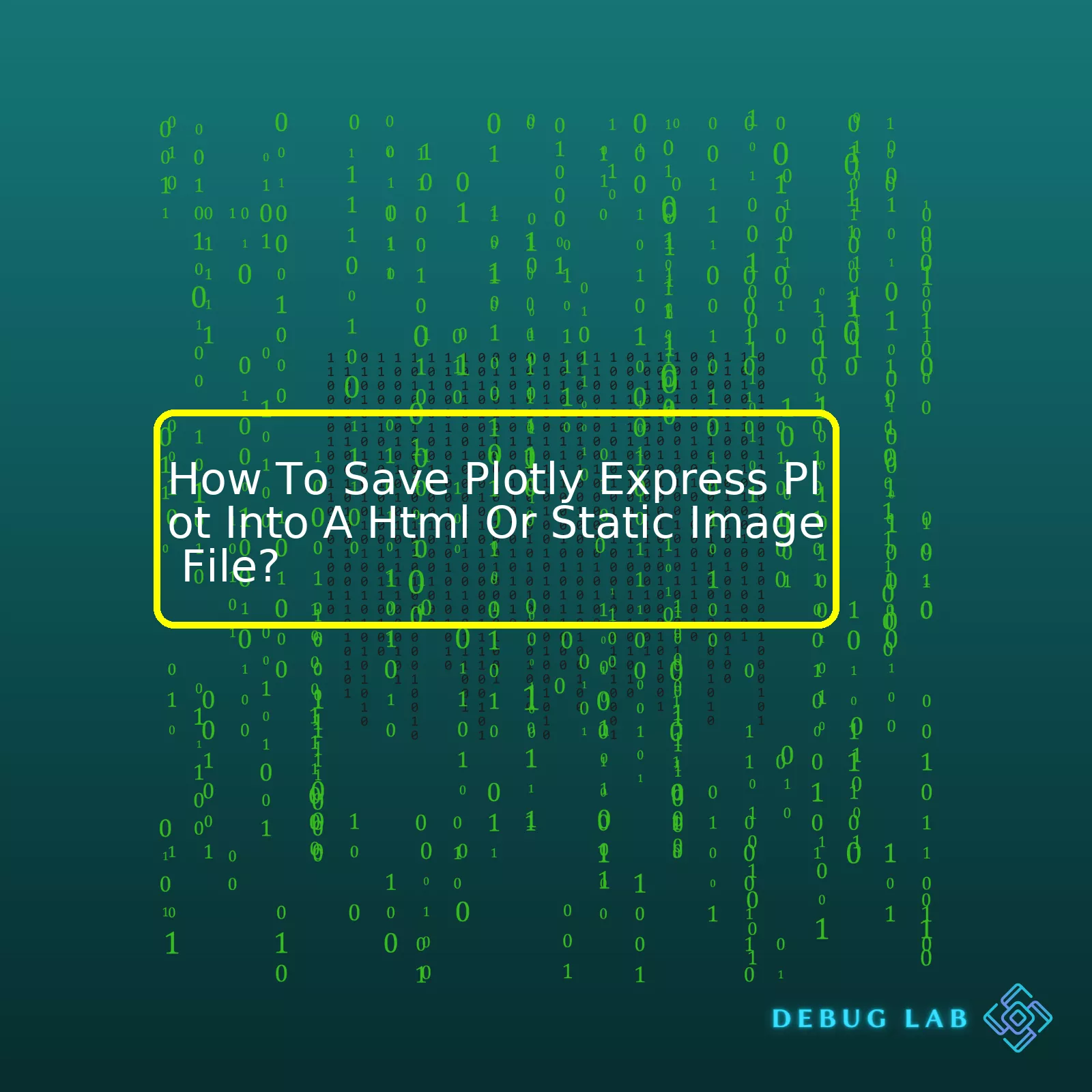
First, compare the different methods of saving Plotly Express plots to HTML and static images using a summary table:
Method | Detailed Steps |
---|---|
Saving as a standalone HTML file |
|
Saving as static image file |
|
For example, we might have a Scatter Plot saved as an “scatter_plot.html”:
import plotly.graph_objects as go fig = go.Figure(data=go.Scatter(x=[1, 2, 3, 4], y=[10, 15, 13, 17])) plotly.offline.plot(fig, filename='scatter_plot.html')
Or a line graph saved as a png image:
fig = go.Figure(data=go.Scatter(x=[1, 2, 3, 4], y=[10, 15, 13, 17], mode='lines+markers')) fig.write_image('line_graph.png')
The crux of preserving Plotly Express plots in HTML or Static Image files is discerning the essential functions and tools needed for the task. With focus on the aforementioned steps and illustrative examples, you too can easily store and share elaborate plots made with Plotly Express! Here are some online references that support these methods, Creating and Updating Figures in Python and Static Image Export in Python.
Plotly Express is a terse, consistent, high-level wrapper around Plotly.py for rapid data exploration and figure generation. It’s a handy tool we can use to create stunning visualizations in Python. To delve in, I’ll explain ways to generate plots using Plotly Express and then save these plots into HTML or static image files.
Saving Plots as HTML
In order to save any plot created using Plotly Express as an HTML file, you’ll integrate the function
plotly.offline.plot()
. This function can be used to render your plots in HTML and automatically open them up in your default web browser. Here’s a simple line of code that creates a scatter plot and saves it as an ‘output.html’ file:
import plotly.express as px import plotly.io as pio df = px.data.iris() # sample data scatter_plot = px.scatter(df, x="sepal_width", y="sepal_length") # Save the plot in html format pio.write_html(scatter_plot, 'output.html')
The created ‘output.html’ file will contain the scatter plot that you have generated with Plotly Express.
Saving Plots as Static Images
If you’d need to save your plots as a static images like .jpeg, .png, etc. Plotly Express has a built-in functionality for exporting the plot as static images. You just call the
write_image()
function from plotly.io (or your alias for it), like so:
import plotly.express as px import plotly.io as pio df = px.data.iris() # sample data scatter_plot = px.scatter(df, x="sepal_width", y="sepal_length") # Save the plot in png format pio.write_image(scatter_plot, 'output.png')
This will save your scatter plot as a ‘output.png’.
Please note, if you’re planning on saving figures as static images, you must install “plotly-orca” command-line utility and the psutil and requests Python libraries. To extend support for more formats pdf and eps, also install the poppler-utils command-line utility.
Extension | Description |
---|---|
HTML | Interactable and can be easily shared |
PNG | Static image, widely accepted, lossless compression |
JPEG | Static image, wide browser compatibility |
Static image, vector format | |
SVG | Static image, vector format, editable in drawing software |
Hence, Plotly express, being a versatile tool, not only provides us a way to generate numerous types of plots but also gives us the ability to store these plots in various formats. Remember the function write_html will save the figure as an html while the function write_image, which utilizes orca utility behind scenes, shall export the graphs as static images in mentioned formats.
Remember, your choice on whether to export plots as HTML or static image would depend largely on your need – whether you want interactivity or whether you require to share or include it across different platforms.
Plotly Express provides numerous plot types that can be effectively utilized for visualizing data in a range of contexts. Some common plot types include line charts, bar charts, histograms, scatter plots, and pie charts.
Moving on to addressing the part about saving the Plotly Express plot into either an HTML or static image file, let’s take, for instance, a bar chart representation:
To begin with, you’ll want to import the two necessary libraries, pandas and plotly express:
import pandas as pd import plotly.express as px
Next, create some dummy data that can be used to plot a bar chart:
df = pd.DataFrame({'Category': ['A', 'B', 'C', 'D', 'E'], 'Value': [33, 85, 88, 90, 76]}) fig = px.bar(df, x='Category', y='Value')
Here, we’ve created a simple DataFrame with two columns Category and Value. We then generate a bar chart using Plotly Express.
From here, you have two options for exporting your plots – either as an HTML file or as a static image file:
1. Save the Plotly Express plot to an HTML file:
fig.write_html("output.html")
You can open up the HTML file created above (in this case, “output.html”) to view the plot you’ve created. This is a great way to share plots as anyone will be able to see the interactive version of the plot just by opening the HTML file.
2. Save the Plotly Express plot to a static image file:
fig.write_image("output.png")
You may need to install the ‘kaleido’ package if it isn’t already installed in order to use the write_image function. You can do so by running:
pip install -U kaleido
After successfully running your code, an image titled ‘output.png’ will be created in your current directory. This option is particularly handy if you’re looking to share the plot as an image; keep in mind, however, that the interactivity of the plot doesn’t carry over into the image file.
Remember to consider the trade-offs and uses of each format when deciding on which one to use. Exporting your plots in different formats allows you to utilize them as per your requirements while leveraging the versatility of Plotly Express at the same time.Following the thirst for knowledge to understand Plotly Express, a Python graphics library, we will dive into unraveling the step-by-step process of creating Plotly graphs and saving them as HTML files or static images. In the same vein, let me assure you that upon mastering this prototypical library’s capabilities, your data visualization tasks will be effortless and fascinating.
Save Plotly Express Plot Into A HTML File
The library’s adoption in numerous applications has been hastened by its interactive nature. The first step involves installing the required libraries with the following command:
!pip install plotly !
After successfully making sure these libraries are present in your Python environment, generating graphs have never been easier. The step involves creating a bar chart. Here’s how it’s done:
import plotly.express as px df = px.data.tips() #Fetches the sample dataset fig = px.bar(df, x='day', y='total_bill')
By running these codes, one can create a bar chart. Now, the final stage of saving the created chart as an HTML file needs executing the codes below:
fig.write_html('file.html')
Here,
write_html()
is a built-in function in the Plotly library used for saving visualizations in HTML format. You could replace ‘file.html’ with your preferred filename. View the saved HTML using any web browser by opening the HTML file.
Save Plotly Express Plot As Static Image
At times, there is a pressing need to save plots as static images majorly for quick sharing, especially in locations with shy internet connectivity. To do this, you will need to install the kaleido package, integrated tightly with Plotly for rendering static images (SVG, JPEG or PDF). This package could be installed using the command:
!pip install -U kaleido
To save as a static image:
fig.write_image('file.png')
Once again, ‘file.png’ can be swapped with your choice filename. This feature is appealing as images can be shared effortlessly across diverse platforms. You can open the image using any program capable of reading PNG files.
Remember, each time you change your chart and find it satisfactory, use the appropriate saving method to retain your work. Establishing new routes within our plotting journey using Plotly Express will add depth to your exploring toolbox; consider going through the official documentation to gain in-depth insight.
In all humility, my task of guiding you on using this tool effectively is worthwhile considering the results you will yield from these answers and further harnessing their universality purely out of practising invariably. Get coding and bridge superb expressions of your colorful datasets into reality.Saving a Plotly Express Plot as a Static Image
Saving a Plotly Express plot into a static image file or HTML isn’t a daunting process. The key advantage of Plotly’s interactive plots is that they can also be exported to standalone HTML or static images such as PNG, JPEG, and SVG.
Here is how you can do it.
Export to Static Images:
To export Plotly Express plots as static PNG, JPEG or SVG files, the process is pretty straightforward. You’ll first want to import the ‘plotly.io’ module in addition to ‘plotly.express’. Consider an example using the following Python code:
import plotly.express as px import plotly.io as pio df = px.data.iris() fig = px.scatter(df, x="sepal_width", y="sepal_length") pio.write_image(fig, 'fig1.png')
In this example, we’re simply:
– Importing the necessary modules (‘plotly.express’ and ‘plotly.io’)
– Creating a scatter plot with ‘px.scatter’
– Saving our created plot as a PNG file with ‘pio.write_image’. You could change the file extension to .jpeg or .svg for other formats.
Export to HTML:
If you prefer to have your Plotly Express plot as a standalone HTML, the procedure is similar to creating static images. Here is a quick Python script:
import plotly.express as px df = px.data.iris() fig = px.scatter(df, x="sepal_width", y="sepal_length") fig.write_html("scatter_plot.html")
This script is doing the following:
– Importing ‘plotly.express’
– Creating a scatter plot with ‘px.scatter’
– Saving the plot as an HTML file using the ‘write_html’ function.
Note: This option is particularly useful if you wish to share the interactive version of your graph without requiring the viewer to run your Python script. The resulting HTML includes all necessary dependencies and will work offline.
For reference, ` write_html` and `write_image` methods are part of Plotly’s figure object methods which allows direct export from Plotly figures to various popular static file formats and HTML. Also remember that to use the `write_image` method specifically, the plotly orca utility must be installed. This is because Orca is the technology Plotly uses server-side to make generating static images fast and robust.
Overall, saving your Plotly Express Plot into a static image file or an HTML file is a very simple task. It allows you to preserve your visualizations and share them effectively with others.When working with Plotly Express, an efficient Python graphing library, you might have been faced with situations where saving plots into HTML or a static image file format could be problematic. While creating interactive visualizations are pretty straightforward, saving those created plots perhaps pose more than one snag. Let’s dive deep and look at some common issues that you might face when trying to save Plotly Express Plots to various file formats as well as how to effectively troubleshoot them.
Trouble Saving to HTML Format:
Plotly offers native support for generating static images, but there are times when you might encounter issues creating/saving HTML files. This is often due to the fact that the user has not properly used the
plotly.offline.plot
method.
For instance, if your goal is to generate an html file of a plot named ‘fig’, you should use:
import plotly.graph_objects as go from plotly.offline import plot fig = go.Figure(data=[go.Scatter(x=[1, 2, 3], y=[3, 1, 6])]) plot(fig, filename='my_plot.html')
This will create an ‘my_plot.html’ file in your working directory, showcasing your scatter plot.
Issues Saving as Static Images:
There could be instances where you might experience problems when trying to save plots as static images. The likely cause of this issue originates from either an outdated version of Plotly, an incomplete installation, or unavailability of necessary dependencies.
In such scenarios, you need to ensure two things:
– You have the latest version of Plotly installed. You can do so by running:
pip install plotly --upgrade
– Make sure you have the plotly-orca package installed alongside psutil and requests. They are required for image export. You can install these via pip:
pip install plotly-orca==1.2.1 psutil requests
This would normally resolve any difficulties related to exporting images. For additional information regarding exporting to static images using plotly, visit Plotly’s official documentation on static image exportation.
Non-display of Saved Figures on the Viewer:
In certain cases, the plot doesn’t display on the viewer after being saved to an HTML file. A possible solution is to instantiate the browser while using Plotly offline to display the figure, as seen in the following code snippet:
import plotly.offline as pyo fig = go.Figure(data=[go.Scatter(x=[1, 2, 3], y=[3, 1, 6])]) pyo.iplot(fig, filename='my_plot.html')
The
pyo.iplot()
function immediately opens the plot within an iframe in your default web browser when executed.
Hence, finding yourself stuck while trying to save Plotly Express plots isn’t an isolated challenge; it’s something faced by numerous data scientists and developers. With the correct steps taken and tools applied, it can be quickly rectified to produce excellent visualization results.When it comes to web development, the tradeoff between HTML and static images often boils down to a question of efficiency. However, since we are specifically discussing how to save Plotly Express plots into an HTML or a static image file, let’s turn our focus in that direction.
Plotly Express is a high-level interface for data visualization. It is built on top of Plotly.py which makes it easier to create complex plots from data in a data frame. In simple terms, Plotly Express will transform your data and produce an effective visualization.
Usually, Plotly charts are interactive and rendered using JavaScript, but they can also be exported as static files. These can either be an HTML file or a static image file. Each option offers some advantages:
– HTML: It’s interactive, lightweight, and can be easily shared via URLs. The plot can also include hover actions, zooming capabilities and more, because it is rendered in JavaScript.
Here’s an example code snippet on how to save a Plotly Express plot as an HTML file.
import plotly.express as px df = px.data.iris() fig = px.scatter(df, x="sepal_width", y="sepal_length") fig.write_html("filename.html")
– Static Images: These files are shareable too and they maintain their visual integrity across devices and platforms. It provides an accessible way for visuals to be easily included in reports, presentations and articles without the need for an internet connection.
To save a Plotly Express plot as a static image file, here is the example code you would use:
import plotly.express as px df = px.data.iris() fig = px.scatter(df, x="sepal_width", y="sepal_length") fig.write_image("filename.png")
Choosing between these two depends largely on your requirements. If you want interactive features and the ability to explore different layers of data, HTML is the ideal choice. On the other hand, if you require a snapshot of the plot for offline usage, then a static image is preferable.
Efficiency is not only about performance. It also includes maintaining the quality and learning curve along with easy integration. So when comparing the efficiency of HTML plots versus static image plots – it isn’t just about file size or rendering speed, but also about how effectively it can communicate data and interactivity based on your specific usage needs.
Hence, whether you save your Plotly Express plot into an HTML or Static Image file, do ensure you take into account all the above factors.
For more insights on Plotly Express and its methods, you can visit the official documentation here.
While working on Plotly for creating interactive and visually appealing data visualizations, it is crucial to seize the control of code optimization techniques. Let’s dive deep to understand the best practices for code optimization while using Plotly. By the end of this discussion, we will also cover how to save a Plotly Express plot as an HTML file or a static image.
When optimizing your Plotly code, scale is key. Writing efficient code not only makes your plots load faster, it also helps in managing resources when dealing with large datasets. Here are the vital code optimization practices:
Use Cuff links: This is an advantageous library built on top of Plotly specifically designed for data frames. You can ideally convert Pandas data frames into interactive Plotly charts with a single line of code.import pandas as pd import cufflinks as cf df = pd.DataFrame(np.random.rand(10, 5), columns=['A', 'B', 'C', 'D', 'E']) df.iplot(kind='scatter')
– Reduce Redundant Code: Streamline repetitive processes into functions or loops instead of writing them over and over again. This minimizes the amount of unnecessary code floating around, which could potentially slow down your script.
– Optimize Data Structures: Using optimized data structures like numpy arrays instead of lists can drastically speed things up while dealing with numerical data.
– Leverage Itertools: Python’s itertools module has made several operations more efficient by reducing the memory overhead.
– Profiling: It’s hard to know where the problem spots in your code are without looking. That’s why Python offers tools such as the cProfile module to locate routines that require optimization.
Now let’s shift rewards and focus our attention on “How to save Plotly Express plot into an HTML or static image file”. The main advantage of using Plotly is its support for interactive plots. However, there are times when you might need to export your plots as static images or standalone HTML files. Here is how to do so:
To save a Plotly Express plot to an HTML file:
import plotly.express as px fig = px.scatter(df, x="sepal_width", y="sepal_length") fig.write_html("path_to_file.html")
Where `path_to_file.html` should be replaced with your desired file path and name.
To save a Plotly Express plot to a static image file, you will need to install the kaleido package because plotly doesn’t support static image export for SVG directly. Once the kaleido package is installed, use write_image function:
fig.write_image("path_to_file.png")
Replace `path_to_file.png` with your actual file path and the name you want for your image.
Essentially, both saving to HTML and static image involves ‘write_html’ and ‘write_image’ functions respectively after the figure object is defined. For further information, visit the official Plotly documentation on exporting plot as a static image.
By capitalizing on these practices, you enhance your code readability, efficiency and overall performance in visualization tasks with Plotly. Remember, code optimization is not one size fits all. You need to reflect on what works best with your specific scenario and dataset.Saving plots as a HTML or static image file using Plotly Express is a skill that will enhance the visualization of your data; whether you’re a data scientist, business analyst, or software developer. Plotly Express has made this process easy with its in-built functions.
Assuming we have already created an interesting plot from our data by invoking various Plotly Express charting functions, let’s examine the steps to save the resulting plot into a file:
To save the plot into a .html file:
To achieve this, use the
write_html
function from the plotly.io (or py) module:
import plotly.io as py fig = px.line(data_frame=data, x='time', y='value', title='Time series plot') py.write_html(fig, 'output.html')
This script will create an interactive .html file named “output.html” of your plot;
To save the plot into a static image file:
Saving as a static image is also quite straightforward using the
write_image
function from the plotly.io (or py) module. It’s worth noting that for this functionality, you need to install the kaleido package.
pip install kaleido
Then, use the
write_image
function:
import plotly.io as py fig = px.line(data_frame=data, x='time', y='value', title='Time series plot') py.write_image(fig, 'output.png')
The above script creates a .png version of your plot named “output.png”.
Remember, the ability to save your visualizations into a HTML or static image file effectively with Plotly Express not only serves you with the convenience of sharing your eye-catching graphics across different digital media and platforms with ease but also ensures that your trending insights can stand alone outside your original code base presenting just the insights and stripping away all the “geeky” parts which are indeed essential but less interesting to a non-technical audience.
Plotly Documentation: Creating and Updating Figures With Plotly’s Python Graphing Library provides more examples and detailed information about creating and saving graphs using Plotly Express.