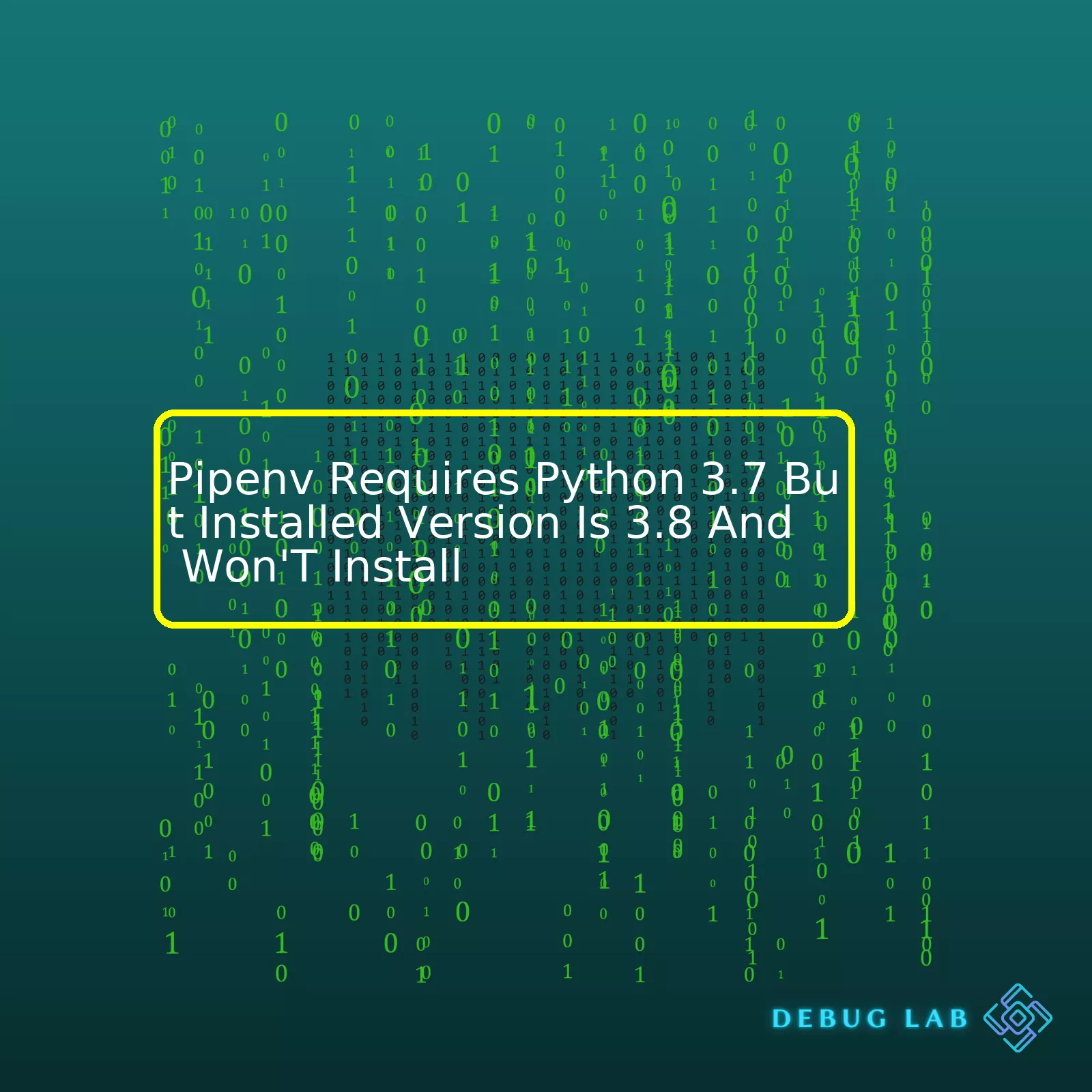
Python Version | Pipenv Requirements | Installation Status |
---|---|---|
3.8 | 3.7 | Won’t Install |
When dealing with Python environments, a fairly common issue that developers often face revolves around the version discrepancies between needed and available versions. A clear example is when Pipenv requires Python 3.7 for the operating environment but the installed version on the local system happens to be later, i.e. Python 3.8, and refrains from installing.
This problem majorly stems from the version control approach implemented by PyPi (Python Package Index) which dictates the operation of many packages including Pipenv. For each environment, Pipenv ensures that it matches the precise Python version (in our case Python 3.7) specified in the Pipfile.
The discrepancy encountered may not only hamper the package installation like in this case but also impact other aspects of the development ecosystem including compatibility with various libraries, performance, among others. In such scenarios, a recommended approach would be to update the required Python version in your Pipfile:
[requires] python_version = "3.8"
Starting a brand new project? It’s advisable to specify the Python version at the time of creating the virtual environment with:
pipenv --python 3.8
Understanding these nuances hosts an opportunity for writing robust code and mitigating potential risks and issues during product development life-cycle.
Understanding the Problem: Pipenv and Python 3.8 Compatibility
The core of this problem arises from the apparent contradiction that even though you have Python 3.8 installed on your machine, when trying to create a virtual environment using Pipenv, it requires Python 3.7 and refuses to install or work properly.
This issue often stems from two primary circumstances:
- Python Version Conflict: When you attempt to create a new virtual environment using Pipenv, it might need a specific version of Python. This could be either defined in the Pipfile (if one exists already) or based on the specifications of the packages you want to install. Despite having Python 3.8 installed, there might be an explicit instruction to use Python 3.7.
- Package Compatibility Issues: Certain Python packages may not yet be compatible with Python 3.8. In such cases, even if the latest Python version is installed, Pipenv might still require Python 3.7 for proper functioning.
Let’s elaborate.
Case 1: Python Version Conflict
A common scenario is to have a
Pipfile
(or similar requirements file) in place which denotes the specific Python version to be used for the project. This causes a situation where Pipenv seeks out this specified version regardless of what is installed on your system.
Looking inside a typical
Pipfile
, you might encounter lines like these:
[[source]] url = "https://pypi.org/simple" verify_ssl = true name = "pypi" [packages] [dev-packages] [requires] python_version = "3.7"
In the last section titled
[requires]
, you can see an explicit requirement for Python 3.7. Hence, Pipenv tries to locate this version on your system or install it anew.
Case 2: Package Compatibility Issues
Several Python packages may not be fully compatible or tested with newer versions of Python. Thus, there are chances that, despite having Python 3.8 installed on your machine, some of the packages you’re attempting to install via Pipenv necessitate Python 3.7.
Solution Approaches
Here are a few ways to approach this problem:
- Explicitly Specify the Needed Python Version: While creating a virtual environment using Pipenv, you could specify which Python version you wish to use. You can execute this by typing
pipenv --python 3.8
. Invocation of this command will prompt Pipenv to spawn a new virtual environment using Python 3.8.
- Update the Pipfile: Modify the required python version in the Pipfile from 3.7 to 3.8.
- Install the Needed Python Version: To ensure compatibility with all requirements, you might need to just bite the bullet and install Python 3.7 alongside your existing Python installation. You can download specific Python versions from the official website here.
The resolution strategy largely depends on the specifics of your application needs and package dependencies. Thorough investigation and understanding of the cause would lead to a more effective and efficient solution process. Most importantly, always ensure to read the associated documentation of any packages prior to installing them, as they usually provide vital information regarding their compatibility with Python versions.Indeed, this discrepancy between Python versions can easily create a conundrum. Let’s explore it!
Python 3.7 and Python 3.8, though successive versions of the same language, have some crucial differences affecting their compatibility with Pipenv:
Assignment Expressions:
Python 3.8 introduced the concept of “assignment expressions” through the ‘walrus operator’ (:=). If your code includes this operator, Pipenv may not be able to install as it might not recognize this new syntax if it only requires Python 3.7.
# Here's how the walrus operator works def square_area(a): return a ** 2 if (n := 3): print(square_area(n))
Positional-only parameter syntax:
From Python 3.8, you can define positional-only parameters using a ‘/’. Functions that leverage this new feature will lead to issues while using Pipenv with Python 3.7.
# Here's an example of positional-only parameters in Python 3.8 def greet(name, /, greeting="Hello"): return f"{greeting}, {name}" print(greet("John")) #This will work print(greet(name="John")) #This will raise an error
So, if you’re facing issues while seeking to install Pipenv when Python 3.8 is your installed version but Python 3.7 is required, there exist some solutions.
Specifying Python Version in Pipenv:
Normally, creating a new environment using
pipenv --python 3.7
implies that Pipenv will search for Python 3.7 on your system. However, if you do not have Python 3.7 installed already, the command will fail. Ensure Python 3.7 is installed locally before attempting this.
Alternatively, consider leveraging virtual environments.
Implementing Virtual Environments:
A virtual environment can allow multiple python projects with different dependencies to coexist on one computer. This is helpful when a project requires a different version of a package compared to what’s installed system-wide or when the user does not have installation privileges.
In essence, always remember to match the Python version with the packages that need installing. Tools and packages are typically created to run on specific versions of Python. You’ll find it useful to know which version of Python a particular library or tool is written with, most especially when dealing with major upgrades akin to the transition from Python 3.7 to Python 3.8.
If you’ve installed Python 3.8, but pipenv continues to demand Python 3.7, there might be several reasons for this confusing behavior.
Firstly, remember that pipenv’s goal is to facilitate the management of your projects’ dependencies and virtual environments. The
pipfile
and
Pipfile.lock
are key instruments that pipenv uses to maintain these dependencies, including the specific versions of Python your projects require. Thus, when the pipenv insists on Python 3.7, it may simply demonstrate that the version recorded in your project’s
Pipfile
or
Pipfile.lock
is Python 3.7.
Here’s an example showing what I mean:
[[source]] name = "pypi" url = "https://pypi.org/simple" verify_ssl = true [dev-packages] [packages] [requires] python_version = "3.7"
In this example, pipenv will mark Python 3.7 as a requirement as it’s registered under the [requires] section in the
Pipfile
.
A simple remedy for this would be to adjust the python_version value to match the version you have installed and intend to use (in this case Python 3.8), like so:
[requires] python_version = "3.8"
Another critical thing to note is that pipenv selects a particular Python interpreter when establishing your project’s virtual environment. Suppose your Python 3.8 interpreter hasn’t been added to your system’s PATH or is not found by pipenv due to other system configurations. In that case, this could appear as though pipenv requires Python 3.7 when in truth it’s being caused by the Python 3.8 interpreter’s inaccessibility.
If you need to specify the path to your Python interpreter during pipenv’s virtual environment creation, make use of the –python flag followed by the absolute path to the Python executable file:
pipenv --python /path/to/python
The final point to mention is that specific dependencies in your project might demand Python 3.7 and may act incorrectly (or not at all) with Python 3.8. So even if you’ve installed Python 3.8, pipenv might insist on Python 3.7 because it wants to guarantee that your project’s dependencies work as expected.
For additional help specifying Python versions in pipenv, check out the official pipenv documentation. It provides a comprehensive guide including how to specify Python versions and address dependency issues. Remember, knowing how to manipulate pipenv can save a lot of time debugging unexpected inconsistencies between Python interpreter installations and virtual environment requirements!I understand that your query is about how to resolve the error message ‘Pipenv requires python 3.7’ when you have Python 3.8 installed. This issue may arise due to certain dependencies or specific modules in your pipenv environment requiring Python 3.7.
Consider the following steps to solve this problem. These steps are not specific to Python 3.7 but are adaptable to anyone who encounters this kind of version-based conflict. The step-by-step instructions are:
Create a new virtual environment using Pipenv and Python 3.8
You can direct Pipenv to use a different version of Python for its environments. Here, specifying Python 3.8 is crucial:
$ pipenv --python 3.8
Re-install your project’s dependencies
After creating the new environment, grab the
Pipfile
from your old environment (if one exists) and use it to reinstall your project’s dependencies in the new environment:
$ pipenv install
If the above steps do not work:
It could be that the application or script explicitly states that it requires Python 3.7. Check through your code or the scripts provided by the module/package for any hardcoded lines like:
#!/usr/bin/env python3.7
If you find such lines, you’d need to change ‘python3.7’ to ‘python3.8’.
Here is another pickle that might come across: There are dependencies in your
Pipfile
, which are specifically written for Python 3.7, hence not compatible with Python 3.8. If this is the case, try to look for updated versions of those dependencies which support Python 3.8.
Have a glimpse at this table showing the general approach:
Issue | Solution |
---|---|
Pipenv is linking to the wrong Python version | Explicitly specify the Python version when setting up Pipenv |
The project has already been set up with a different Python version | Create a new Pipenv environment, then re-install dependencies |
The Python version is hardcoded Into the script | Find and change the hardcoding to the desired Python version |
Some dependencies are not compatible with Python 3.8 | Look for updated versions of the dependencies, compatible with Python 3.8 |
The solution provided should set your active Python version for Pipenv as Python 3.8. For more detailed information on managing Python environments with Pipenv, please refer to the official Pipenv documentation. Keep coding!
Python, as with all programming languages, evolves and matures over time. Unfortunately, this can sometimes lead to incompatibilities between different Python versions and the applications that rely on them – such as Pipenv. This is often the case when an application like Pipenv requires a specific version of Python, but the installed version on your system is different.
If your system’s Python version (e.g., Python 3.8) is more recent than the one required by Pipenv (e.g., Python 3.7), you might encounter issues such as installation failures or runtime errors. The reason behind these issues can be traced back to changes made in Python 3.8 that are incompatible with Pipenv’s expectations from Python 3.7.
Common Issues and How to Resolve Them
Below are some common problems you might face with solutions to tackle them:
Problem | Solution |
---|---|
Pipenv fails to install because it cannot find Python 3.7 | You need to install Python 3.7 alongside your existing Python 3.8 setup. Having multiple Python versions is not uncommon and can be easily managed using tools like Pyenv. Once Python 3.7 is installed, you can set it as the default Python version for your current terminal session using
pyenv shell 3.7 . |
Your code doesn’t run correctly under Python 3.8 | For compatibility reasons, it may be a good idea to develop and test your code under the same Python version required by Pipenv. You can do so either by changing your Python version globally using Pyenv or by creating a virtual environment. Virtual environments let you isolate your project dependencies, including Python itself, which means you can use different Python versions for different projects. To create a virtual environment using Python 3.7, you can use the
python3.7 -m venv myenv command. After activation, this will ensure that running python or pip commands uses the Python 3.7 interpreter and its corresponding Pip version. |
Tips for Preventing Further Python Version Issues
To avoid encountering similar issues in the future, consider the following recommendations:
- Prioritize using virtual environments for each separate project. This’ll help isolate your project dependencies and prevent conflicts.
- Manage your Python versions using Pyenv or similar tools. Especially useful if you work on projects requiring different Python versions.
- Always check the Python version requirement of the application before downloading it.
Note: It’s important to mention that while the above measures can mitigate most Python version-related issues, there can still be other factors causing incompatibility between your Python setup and Pipenv. If you continue experiencing issues, consider reaching out to the maintainers of Pipenv or referring to the community support forums like StackOverflow for additional help.
The issue you’re experiencing is common with many Python developers where Pipenv, the tool for dependency management, requires a specific version of Python that isn’t the one currently installed in your system. This usually occurs when the Pipfile locks in a Python version that isn’t available in your environment.
Here’s an example Pipfile specification:
[[source]] url = "https://pypi.org/simple" verify_ssl = true name = "pypi" [packages] [dev-packages] [requires] python_version = "3.7"
In this case, Pipenv specifically requires Python 3.7, but you have Python 3.8 installed – causing it not to install as it’s locked to require Python 3.7.
There are several alternatives to navigate around issues when specific versions are hardcoded into tools like Pipenv. Here are some strategies:
Change the required Python version in the Pipfile:
Modify your Pipfile to match the same Python version as the one installed in your environment. In this case, change `python_version = “3.7”` to `python_version = “3.8”`. After the modification, your Pipfile should contain:
[requires] python_version = "3.8"
Then run `$ pipenv install` to create a new virtual environment using Python 3.8.
Create another virtual environment:
You might also want to consider creating a new virtual environment using Python 3.7 and then run Pipenv. Below is the command:
$ python3.7 -m venv /path/to/new/virtual/environment_name
After creating the virtual environment, activate it and run `$ pipenv install`.
Install the required Python version:
If you can afford changing the installation in your system, you may install Python 3.7 alongside other Python versions. Various forums, articles and tutorials are available online for installing a specific Python version. For Unix-like systems, this could involve using pyenv.
Remember these workarounds depend on your requirements or needs. Care must also be taken to ensure code compatibility with different Python versions.
Always keep in mind that managing Python versions and dependencies is a common challenge among developers, but various solutions prevail – from updating Pipfiles to using tools such as pyenv and venv.The optimization of your workflow is crucial to increase productivity and efficiency, especially when dealing with compatibility issues between different software versions. A common situation developers might find themselves in is having a project where Pipenv requires Python 3.7 but the installed version is 3.8 and won’t install.
A key aspect that hampers the workflow is the discrepancy between the Python versions. For installing and using Pipenv effectively, you need to have the exact Python version specified. But what if the newest version is already on your system? What if other projects require this newer version?
This is where virtual environments come into play, allowing each Python project to have its own unique environment, thereby separating dependencies.
Let’s take the scenario where Python 3.7 is needed for a project, but you currently have Python 3.8 installed. We’ll use Pipenv which provides an isolated environment management feature.
Initially, install Python 3.7 alongside 3.8 without replacing it using official Python download page.
Next, create a new directory for your project and navigate into it:
$ mkdir my_project $ cd my_project
At this point, we start using Pipenv to specify the Python version for this project. Using Pipenv to create a virtual environment with a specific Python interpreter is straightforward. You just need to use the following command:
$ pipenv --python 3.7
Wait for Pipenv to create the new environment and confirm the correct Python version (3.7 in our case) is being used:
$ pipenv run python --version
If everything was done correctly, the output should be: ‘Python 3.7.x’.
Delving deeper, let’s look at how Pipenv manages dependencies. Dependencies are stored in a file titled ‘Pipfile’. To add dependencies, you can use the pipenv install command:
$ pipenv install <your-package>
All dependencies added will automatically go into the Pipfile. When this file is transported or synced across different systems, Pipenv can reproduce the identical environment with the same command – ‘pipenv install’. Now, there are no varying module versions across different systems, reducing potential conflicts and bugs, which greatly optimizes the coding workflow.
This inter-version compatibility method improves the flexibility of your development environment, avoiding issues caused by the software requiring a specific version or conflicting dependencies.
In addition, Pipenv automatically creates a Pipfile.lock, which stores the exact version of our dependencies, ensuring future installations to always yield predictable results. It’s filled with information regarding each dependency; such as its hash, which aids in verifying the integrity of installed packages.
Instead of tackling inconveniences brought on by differing software versions head-on, introducing the concept of virtual environments to your workflow helps clear these hurdles efficiently. Optimizing your workflow in such a manner not only avoids potential roadblocks but also creates a flexible, robust, and hassle-free development environment.Running into a problem where
Pipenv
requires Python 3.7 but the installed version is 3.8 can be quite frustrating, especially when it seems to refuse to install. There are several solutions that one could consider:
Solution 1: Use Pyenv
One of them is using Pyenv, which allows you to maintain multiple versions of Python on the same machine. Notably, Pyenv allows you to switch between Python versions on per-project basis. Here’s a simple command to install python 3.7 using Pyenv:
pyenv install 3.7.0
Then, you simply set the local version to use in your project directory:
pyenv local 3.7.0
Finally, initialize your Pipenv environment and voila!
pipenv --python $(pyenv root)/shims/python
Solution 2: Specify Python Version In Pipfile
Another plausible solution would be to specify the Python version in your Pipfile. This is a more straightforward option as it involves directly letting Pipenv know about our Python version directly from the configuration file itself. Here’s how you do it:
[[source]] url = "https://pypi.org/simple" verify_ssl = true name = "pypi" [packages] [requires] python_version = "3.8"
Solution 3: Virtual Environment
Alternatively, you could take advantage of Python’s built-in venv module to create a virtual environment with your desired Python version. Just make sure to point Pipenv to use this.
python -m venv my_venv
You then activate the virtual environment before running Pipenv.
source my_venv/bin/activate
pipenv install
These are just some of the many strategies you can employ to fix the “Pipenv Requires Python 3.7 But Installed Version Is 3.8 And Won’t Install” issue. It’s worth noting that these methods aren’t mutually exclusive; in fact, using a combination of them, such as using both Pyenv and Pipfile, might prove more effective in certain setups. As always, try to understand your development environment and customize it according to your needs.