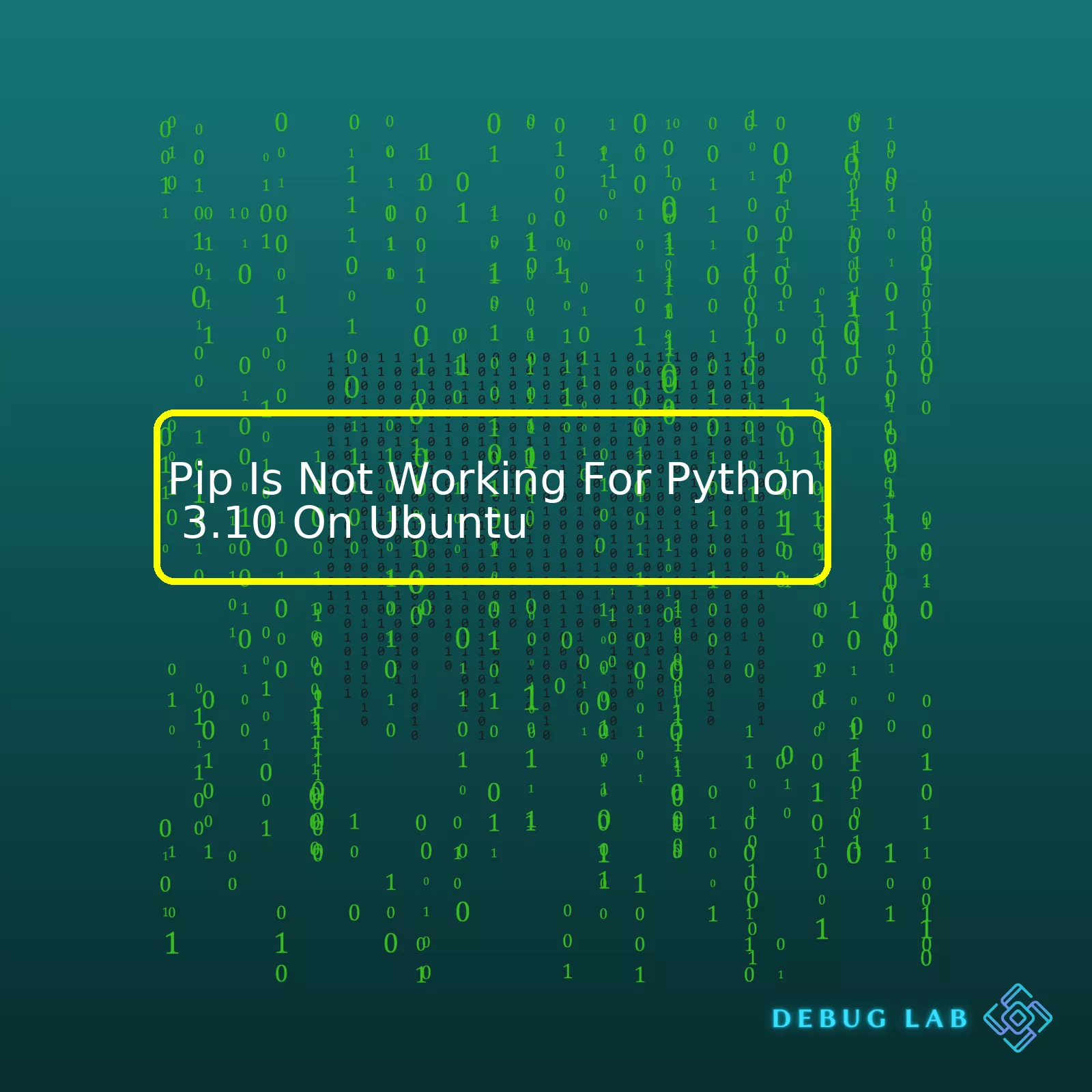
Problem | Solution |
---|---|
Pip not Working for Python 3.10 on Ubuntu | Install the right version of pip, uninstall old or incompatible versions, use python3 -m pip to ensure you’re using the correct version. |
The problem of Pip not working for Python 3.10 on an Ubuntu machine may be attributed to several factors. These could range from having installed an incompatible version of Pip for Python 3.10, to pip itself not being properly installed in your system. It’s also possible that the prior installed version of Python might be associating with Pip rather than the recently installed Python 3.10 creating a compatibility issue.
When encountering such issues, installing the right version of Pip compatible with Python 3.10 is recommended. Uninstallation and reinstallation of Pip as well can aid when rectifying this problem. Removing older or incompatible versions of pip before reinstalling might present you with a functional Pip for your Python 3.10 environment.
Another method involves using
python3 -m pip install package-name
instead of
pip install package-name
. When you use python3 -m pip, it ensures you run the pip version for Python3. This syntax is structured in such a way so that Python runs the module as a script, hence resolving any conflict caused by multiple versions.
Remember, when dealing with software, especially ones involve compilation like Python and its packages, the principle should always be: “install what you need, keep your environment clean”. Multiple installations of different versions can lead to unwanted associations and invalid references, causing hiccups like these during execution time.
You can reference the official Python documentation to understand more about pip installation and troubleshooting guidelines here. Keep browsing Linux-related forums for community-driven solutions to such problems. They can provide excellent real-world scenarios with practical solutions for common problems like this.
python3 -m pip install --upgrade pip
This command can assist you in upgrading pip associated with Python 3. This ensures that Pip gets updated to the latest version, reducing chances of compatibility issues with newer Python versions such as Python 3.10.
Reinforcing your understanding of Python, Pip, and how Python manages modules will enable you to troubleshoot such situations quickly and efficiently. Furthermore, acquainting yourself with reliable coding practices will undoubtedly help prevent such roadblocks in the future.
Consider exploring Python virtual environments too. Using them can isolate your Python environments for different projects, avoiding version conflicts among packages. The venv module, available from Python 3.4, makes it easy to create lightweight virtual environments with their own site directories. You can learn how to set up virtual environments in Python here .
With these tips at your disposal, and an approach that prioritizes learning and adapting from problems, you’ll be armed to face even bigger challenges in your scripting endeavors.The issue at hand is understanding why pip, the de-facto package manager for Python, becomes unresponsive primarily in Python 3.10 on Ubuntu. This can be quite an inconvenience especially when you aim to install or manage Python packages.
First and foremost, we need to figure out whether it’s a version issue or not. Python 3.10 is relatively new compared to its other predecessors. Some modules and methods that worked efficiently in previous versions might cause issues in this iteration. It’s crucial to ensure pip is updated to support the new features and changes introduced in Python 3.10. You should upgrade pip, by opening your terminal and typing the following command:
python3.10 -m pip install –upgrade pip
In case this doesn’t rectify your problem, there are other possible causes that could result in pip becoming unresponsive. These include:
• Incorrect Installation: Pip is already included in Python 3.2 and newer versions. However, when manually installed or if the PATH environment variable hasn’t been set properly, it may interfere with pip’s functioning.
• Compatibility Issues: Certain packages may not yet be compatible with the latest Python version which may make pip freeze or even crash.
Functionality problems could also stem from not having the necessary rights to execute pip commands, or discrepancies with the Python environment.
Here is how you can troubleshoot these problems:
1. Install or Reinstall pip correctly: To check if pip is installed, use the following command in your terminal.
python3.10 -m pip --version
If pip isn’t properly installed or perhaps mistakenly removed, reinstall it using:
sudo apt-get install python3.10-venv python3.10-pip
2. Check Compatibility: In case pip is unresponsive while trying to install a specific package, check if that particular package supports Python 3.10. You can usually obtain this information on the package’s official website or its documentation.
3. Admin Rights: Ensure you have the necessary administration rights. If not, run pip alongside ‘sudo’ (granting admin privileges), like so:
sudo python3.10 -m pip install [package-name]
4. Virtual Environment: Consider using Python’s built-in venv module to create a virtual environment for your projects. This lets you install modules locally rather than system-wide, and can help evade any discrepancies between Python’s system-level settings and project-specific dependencies.
To create a virtual environment and activate it, use:
python3.10 -m venv my_env source my_env/bin/activate
Working with a fresh, new installation of Python 3.10 presents unique challenges due to incompatibilities and undiscovered glitches. However, by making sure every software piece interacts correctly, we can seek to create a development environment that fortifies efficiency and minimizes downtime. Always refer to the official Python documentation for accurate and reliable resources.Python’s Pip is nothing short of an amazing tool that bridges the gap between a Python developer and over 235,000 Python packages. However, complexities arise when trying to use it with Python 3.10 on Ubuntu.
Before diving into these specifics, it’s pertinent to understand what Pip is and how it functions particularly in an Ubuntu environment.
Pip stands for ‘Pip Installs Packages‘. It’s a package manager in Python used in installing and managing software packages. Think of Pip as the easy button for adding more functionality; besides installing, Pip also enables you to manage versions and dependencies amongst your Python projects.
Ubuntu, on the other hand, leans toward Python 2 until recent editions. Since Ubuntu 20.04 LTS, Python 3 has been the default installation. By default, the command python or pip will activate Python 2 (if installed).
Don’t be surprised if your ‘pip install’ lines don’t seem to work for Python 3.10 because they’re probably working for an older version without you realizing it.
Now let’s examine why Pip isn’t working correctly for Python 3.10 on Ubuntu:
To install Python packages for Python 3.10 specifically, your system needs to differentiate this command from others. This requires using the python3.10 -m pip install syntax. The ‘-m’ argument implies the subsequent module should be executed.
For instance:
python3.10 -m pip install numpy
However, there may sometimes arise a situation when even the above syntax won’t work. It generally happens either because pip for Python 3.10 wasn’t installed, or Ubuntu doesn’t recognize pip for the latest Python version. To solve this issue, there are various solutions:
• Running the apt-get command in terminal to automatically fetch, unpack, and build required packages:
sudo apt-get install python3.10-pip
After which, try running the previous command related to pip install.
• Downloading pip for Python 3.10 explicitly by relying on curl and python script:
curl https://bootstrap.pypa.io/get-pip.py | sudo python3.10
It fetches and secures the latest version of pip compatible with Python 3.10 and installs it your system.
Remember, sometimes even after following the above steps, Ubuntu might still refer to the old Python version. In such cases, update the default Python version to Python 3.10.
update-alternatives --install /usr/bin/python python /usr/bin/python3.10 1
Through high variability in setups and configurations, it’s not uncommon to encounter issues with Pip on Ubuntu, especially when dealing with newer Python versions like 3.10. But by understanding the backbone of how Pip functions in Python and utilizing the aforementioned solutions, you can streamline your coding experience and fully exploit Linux’s powerful, open-source magic
Despite the usefulness of Pip, Python’s package management system, in automating the installation and management of software packages written in the Python language, several hurdles are often encountered. When using Pip with Python 3.10 on the Ubuntu operating system, some of these common drawbacks could include:
- Incompatibility. At times, pip is not compatible with Python 3.10 because existing libraries may not be updated to support the newer version. Some may cause complications due to syntax changes, depreciation or updates in Python 3.10.
- Dependency errors. While attempting to install some packages, pip may encounter potential conflicts due to unsatisfied dependencies which can lead to an unsuccessful installation. Resolving such dependency issues can sometimes prove a challenge.
- Pip version mismatch. A prevalent problem is having different versions of Pip for different Python versions installed on the machine. This inconsistent environment can cause significant issues when trying to use Pip.
To surpass these issues, frequent troubleshooting methods include:
Updating Pip
Ensure that you have the most recent version of pip installed. The older versions may have compatibility issues with Python 3.10. To update pip on Ubuntu, execute this command from your terminal:
python3 -m pip install --upgrade pip
Specifically Telling Pip The Python Version
Specify the Python version while calling pip such that it knows exactly what it should integrate with. For Python 3.10, modify your pip install commands like so:
pip3.10 install <package name>
Using Virtual Environments
Make use of Python’s built-in venv module or third-party packages like pyenv to create isolated environments for project dependencies, ensuring pip usage will be tied to the appropriate python version.
There is also the combination of Pipenv, it seamlessly integrates Pip and Venv into one entity providing much-needed streamlining for package management with Python.
Remember, tools like pip are constantly updated, so it’s crucial to stay informed about new releases and how they improve or change the tool’s functionality. This will mitigate many of the challenges encountered while using Pip with Python.
In case you face any other issue with pip, don’t hesitate to refer to its official documentation.Assuming you’re working in a development environment of Ubuntu and have hit an obstacle while trying to use pip for Python 3.10, let’s dive deep into how to troubleshoot this issue. When it comes to resolving errors or conflicts, the first area we need to key into is understanding the error message. Usually, the crux of the issue lies there.
So why is pip not working for Python 3.10 on Ubuntu? One possible reason could be that the necessary version of pip isn’t installed yet for Python 3.10. Python packaging tools are evolving, and some older versions of pip may not support Python 3.10 at all.
Another common problem arises when multiple Python versions are installed on your system. The default Python version might not be set to Python 3.10. As a result, when you use the
pip
command, it probably points to another Python version.
How do You Solve these Errors?
First thing you should check is if pip has been installed for Python 3.10. If not, you can fetch and install using the get-pip.py script provided by pypa.io. You would use the following command:
wget https://bootstrap.pypa.io/get-pip.py
python3.10 get-pip.py
This script will fetch the latest version of pip and install it for your Python 3.10 interpreter.
The easiest way to ensure pip is pointing to Python 3.10 is to use pip as a module within Python like so:
python3.10 -m pip install YourPackage
Here, replace “YourPackage” with whatever package you’re trying to install. This way, we’re enforcing the use of Python 3.10 interpreter along with its pip tool.
Alternatively, if you want Python 3.10 to become the default on your system, you can update the alternatives system providing the right path of your Python 3.10 executable:
sudo update-alternatives --install /usr/bin/python3 python3 /usr/bin/python3.10 1
Here we’ve assumed: ‘/usr/bin/python3.10’ as the installation path for Python 3.10, which is quite standard for Ubuntu. But ensure to replace ‘/usr/bin/python3.10’ with actual Python 3.10 installation path in your machine.
Pitfalls to Avoid
While troubleshooting, don’t forget that it’s advantageous to keep different Python versions isolated, especially when managing project dependencies. It avoids clashes between Python versions while installing/upgrading packages.
For such cases, consider using virtual environments. Tools like venv in Python or third-party tools like virtualenv helps you create isolated Python environments. Here’s how to create one for Python 3.10:
python3.10 -m venv my_env
And that’s how you utilize venv to create a new Virtual Environment for Python 3.10 named ‘my_env’. To activate this environment, execute:
source my_env/bin/activate
While working with pip always make sure that you’re operating within an appropriate Python environment. Consistently observing this practice reduces chances of running into ‘pip is not working’ errors.
As you can see from our deep dive, solving pip issues for Python 3.10 on Ubuntu involves checking whether pip is installed correctly for Python 3.10, making sure the correct Python version is being used with pip, and considering the use of virtual environments to avoid conflicts and manage dependencies better.
References:
Python Packaging User Guide – Installing Packages
Python Docs – Python Virtual EnvironmentsLet’s dive right into unveiling the best practices for effective use of Pip with Python 3.10, keeping in mind an Ubuntu environment where you might encounter issues such as “Pip Is Not Working For Python 3.10”.
Make sure the pip is compatible with Python 3.10
The compatibility of Pip with Python versions could be quite a headache. Before anything else, you need to verify if the installed pip version supports Python 3.10.
You can check this using:
pip --version
Given Python’s notorious forward-compatibility issues, it is crucial to monitor all versions. If your pip isn’t compatible with Python 3.10, you can update it by running:
python -m pip install --upgrade pip
You can see more about compatibility problems here.
Use Virtual Environments
When developing various Python projects, a single global installation could lead to a mess in managing different dependencies and library versions. Here comes the practice of using Virtual environments.
Let me explain how this works by making use of the term ‘venv’. It essentially allows creating an isolated Python environment for each project, letting you have multiple versions of a package on the same system without any conflicts.
In order to create a virtual environment, navigate to the project directory and run:
python -m venv my_env
You then activate the environment using:
source my_env/bin/activate
Now, any packages you install using ‘pip’ will be placed in ‘my_env’, isolated from the global Python installation.
Reference for this practice available here.
Always use requirements.txt
Another good practice to ensure smooth functioning involves using a ‘requirements.txt’ file. This file store info about what module versions your project requires. You or anyone else can recreate the same development environment later using this file.
To generate a requirements.txt file:
pip freeze > requirements.txt
To install dependencies:
pip install -r requirements.txt
For more information on this exercise, you can refer here.
While adopting these best practices should advise Pip’s efficacious use, remember that every codebase and operating environment is unique and might require specific considerations. Happy coding!While DIYing your way through tech issues can be a rewarding journey, sometimes the dust of confusion can be hard to shake off when you’re deep into a complicated matter like making sure Pip is functioning well with Python 3.10 on your Ubuntu system. Keeping your computing environment up-to-date plays a huge role in solving such issues, and I’m here to break things down for you.
Python 3.10
is one of the latest versions of Python available in the market. Its sophisticated features and efficient programming characteristics have drawn many developers’ attention. However, some users have encountered trouble while using Pip with Python 3.10, particularly on their Ubuntu systems.
The common issue here is that Ubuntu may not have updated
Pip
to work with
Python 3.10
. Therefore, the most straightforward solution is to update your system regularly. Consistent system upgrades benefit users because they allow smooth operation, compatibility, security, and the ability to leverage the latest technologies. When you upgrade your system:
- It receives the latest feature updates and improvements.
- Your Ubuntu operating system becomes bug-free as these are usually fixed during every release.
- The security vulnerabilities found in the older version become patched.
- The new OS version potentially enhances system performance and efficiency.
The same principle applies to Pip and Python. With each release, potential bugs are being fixed and new features are added. So when Pip is not working for Python 3.10 on Ubuntu, one of the recommended solutions would be to upgrade both Python and Pip to their more recent versions.
Here’s how you can install the latest version of Pip for Python 3.10:
$ sudo apt update $ sudo apt install python3.10-venv python3.10-dev $ python3.10 -m venv myenv $ source myenv/bin/activate (myenv) $ python -V -- output should be Python 3.10.x (myenv) $ python -m ensurepip (myenv) $ python -m pip install -U pip
Here, we’ve used Ubuntu’s package manager apt to perform an update, installed
python3.10-venv
and
python3.10-dev
, set up a virtual environment named
myenv
, activated it,and finally installed Pip. By executing this series of commands, we assure that Pip installed is compatible with Python 3.10.
As coding professionals, we always need to keep our systems primed for the challenges our coding adventures throw at us. Keeping software and system components up-to-date, including our beloved dev tools like Python and Pip, is one crucial part of ensuring a smooth experience. By regularly upgrading your systems, you’ll always have the needed gears ready for your projects.Understanding how to deal with the issue “pip is not working for Python 3.10 on Ubuntu” involves utilizing some advanced debugging techniques and resolution methods. In this scenario, there could be various reasons causing the problems, such as installation issues, PATH environment variables configuration mishaps, or version conflicts. Here are a few ways that professional coders can tackle this kind of problem effectively:
Troubleshooting Pip installation
First, ensure pip is properly installed. Pip is bundled with Python installations starting from Python 3.4 for Python 3 and Python 2.7.9 for Python 2. To check if pip is installed, run the following command in your terminal:
python3.10 -m pip --version
The output should show the pip version if it’s properly installed. If not, you might have to manually install pip for Python 3.10.
Here’s how to install pip for specific python version:
sudo apt-get install python3.10-venv python3.10-pipInspecting PATH Environment Variables
Second, investigate your PATH environment variable. Sometimes, these issues arise because Python or pip isn't configured properly in your system's PATH. The PATH is a list of directories where the system looks for executable files. Use the echo command to check your PATH variables:echo $PATHEnsure that the path to your Python 3.10 and its corresponding pip are included.
If not, add Python and pip to your PATH by modifying your .bashrc or .bash_profile file depending on your shell:
export PATH=/path/to/your/python3.10:$PATHexport PATH=/path/to/your/pip:$PATHSave the changes and reload .bashrc or .bash_profile:
source ~/.bashrcor
source ~/.bash_profileChecking Version Conflicts
Thirdly, version conflicts could also lead to pip problems. If different versions of Python are installed on your system, the pip corresponding to Python 3.10 might conflict with other versions.
Resolve this by using pip associated explicitly with Python 3.10 using the command:
python3.10 -m pip install package-nameRemember, when you use 'pip' alone, it installs packages for the default system Python version, which may not be 3.10. Explicitly tying the Python version ensures you're installing pip packages on Python 3.10 specifically.
Reinstalling Pip
Lastly, if pip still doesn't work, consider reinstalling it. Uninstall the current pip then reinstall it via the Python Package Index (PyPI):
Uninstall pip:
python3.10 -m pip uninstall pip setuptoolsReinstall via curl and PyPi:
curl https://bootstrap.pypa.io/get-pip.py -o get-pip.pypython3.10 get-pip.pyI hope these solutions assist you in troubleshooting the issue. Remember, the order in which the routes are attempted is crucial in finding the cause of the pip malfunction quickly and efficiently. For more insights, the official Python Guide for Installing in Linux(Ubuntu) and the Pip Installation Documentation offer invaluable information.With a firm understanding of the complexities around Pip not working for Python 3.10 on Ubuntu, it's essential to investigate four main solutions.
The first step revolves around checking the currently installed version of Python with the command
python --versionand Pip, using
pip --version.
Understanding the root cause begins by verifying that both dependencies -Python and Pip- are properly installed and updated.A possible issue might be that Pip isn't installed, in which case it can be easily fixed running
sudo apt install python3-pip. This will enable you to download and install Pip, making it functional with Python 3.10 on Ubuntu. You might also have Pip installed but not specifically for Python3. You can verify this by typing
pip3 --version.
Commands Description python --versionCheck installed Python version pip --versionCheck installed Pip version sudo apt install python3-pipInstall Pip for Python3 pip3 --versionCheck installed Pip version for Python3 The second step involves updating Pip -this is a common solution to problems related to Pip functionalities. Running
pip install --upgrade pipon your terminal should do the trick. With an upgraded version of Pip, compatibility issues with Python 3.10 on Ubuntu could be minimized.
The third strategy is looking into virtual environments such as venv or pyenv. Some Python libraries may not be compatible with the latest Python version (3.10) and may still need an older version which could lead to Pip malfunctioning. Creating a virtual environment and installing the needed version of Python there might just solve the problem.
Last but not least, one might consider getting packages from source (like GitHub), instead of fetching them from PyPI, the official third-party software repository for Python, especially if these packages are particularly complex ones.
This deep-dive exploration into why Pip might not work with Python 3.10 on Ubuntu, equips any coder confronting similar scenarios to quickly traverse through the landscape of possible solutions like checking and updating installations, leveraging virtual environments, and considering alternative package sources. For more comprehensive details, consider perusing the official Python documentation. Stay tuned for updates on managing Python dependencies effectively! Remember, asking questions and exploring alternatives is what makes coding an exciting journey, even when initially things don't seem to work out as expected. Keep coding!
For further reading:
1. Pip and Virtual Environments.
2. Installing pip.
3. Best Practices for Python Dependencies