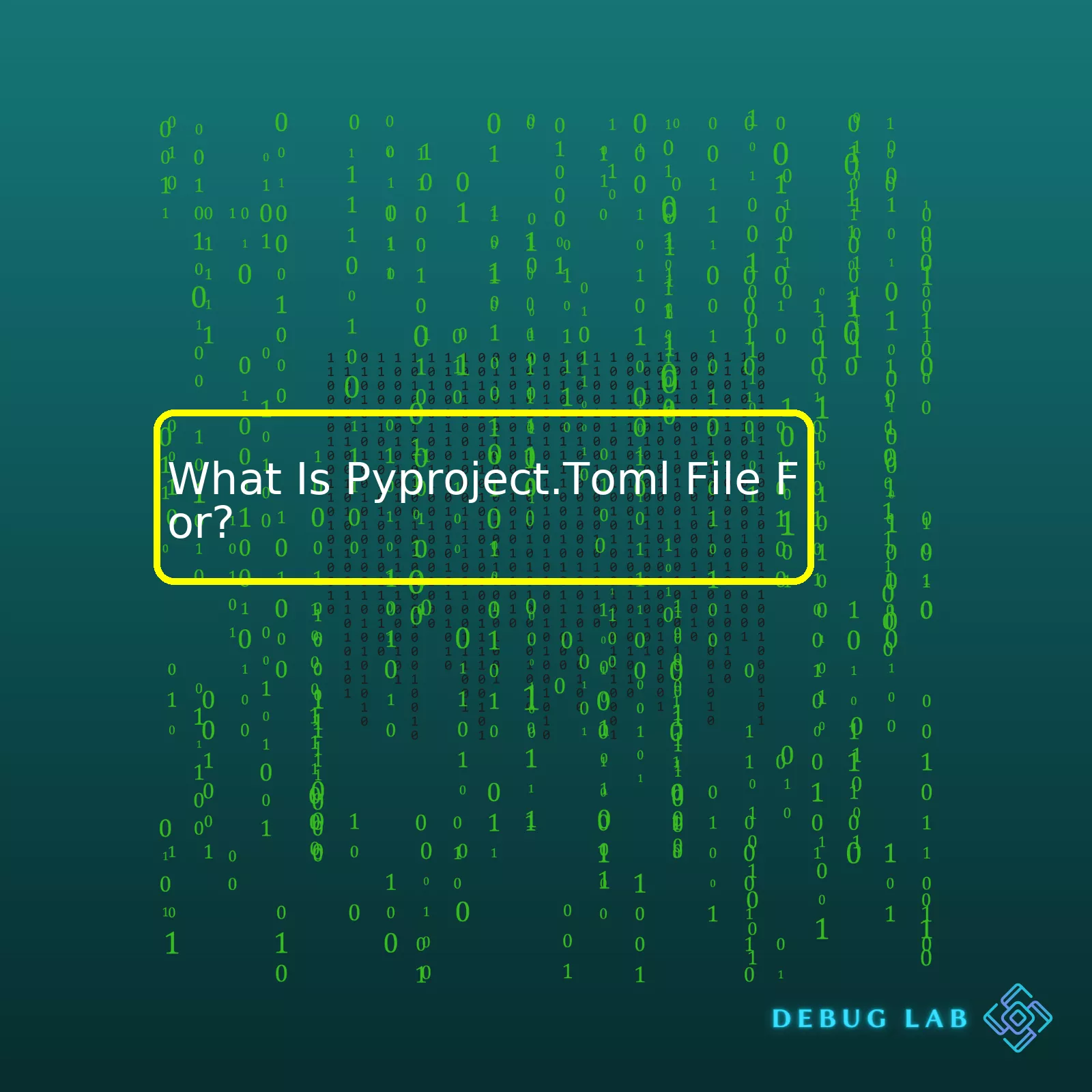
Here is a basic example of how a pyproject.toml may look like:
[build-system] requires = ["setuptools", "wheel"] [tool.black] line-length = 88 target-version = ['py36', 'py37', 'py38'] include = '\.(py|pex)$' [tool.flit.metadata] module = "example_pkg" author = "Example Author"
The above example informs us that this Python project requires setuptools and wheel to be built. Additionally, it’s configured to use black for auto-formatting with a specified line length and target Python versions. And it uses flit for packaging with some basic metadata about the module and author.
Let’s visualize the purpose and usefulness of Pyproject.toml in an HTML table summary:
Attributes of Pyproject.toml File | Description |
---|---|
Build system requirements | The build-system.requires is where you specify the tools needed to build your package; for instance, setuptools, flit, and wheel. |
Tool configuration | Optionally, you can include the configs of any tools you’re using in your project; for instance, tool.black in the example mentions configurations for the Black code formatter. |
Project metadata | This makes it easy for packaging tools to gather necessary info about the project without relying on executing setup.py. Metadata like version, author, license can be provided. |
So, having a pyproject.toml in Python-based projects allows smooth build operations through clearly stated requirements, makes way for additional configurations based on project need, and importantly, helps define essential metadata crucial for packaging and distributing the package or application. The PyPA (Python Packaging Authority) emphasizes pyproject.toml as the recommended standard for building and distributing Python software. More details about Pyproject.toml as per official Python docs could be found here .Fundamentally,
pyproject.toml
is a configuration file that specifies build system requirements for Python projects. As specified by PEP 518 and later enhanced by PEP 621, it contains the metadata about the project like dependencies, scripts, versioning control, and other build-related declarations.
Previously, Python’s packaging scene had disparate ways to declare these kinds of information — via
setup.py
,
setup.cfg
, or
requirements.txt
. The advent of
pyproject.toml
was an endeavor to reduce the clutter and bring standardization and coherence to Python’s package distribution and installation process across multiple platforms.
Following are some key points that express and highlight the importance and usability of
pyproject.toml
in Python Projects:
1. Stipulating Build System Requirements:
Initially proposed under PEP 518,
build-system.requires
table lists the tools and packages required to build the module from source. A typical example would look like this:
pyproject.toml |
---|
[build-system] |
Here, setuptools and wheel are prerequisites to build the project.
2. Project Metadata:
PEP 621 extended the scope of
pyproject.toml
to include project-level metadata under
[project]
table. This includes description, version, authors, classifiers and more. It provides a better structure for maintaining the project details. For example,
pyproject.toml |
---|
[project] name = "myproject" version = "0.0.1" authors = [ {name = "Author Name", email = "author@email.com"}, ] |
3. Declaring Dependencies:
With
pyproject.toml
, dependencies can be clearly defined under
[project.dependencies]
and
[project.dev-dependencies]
tables, almost similar to Pipenv’s approach. This makes tracking dependencies straightforward.
pyproject.toml |
---|
[project.dependencies] requests = "^2.25.1" [project.dev-dependencies] pytest = "^6.2.4" |
The documentation and tooling around Python’s
pyproject.toml
are steadily improving. Implementations like Flit and Poetry have already embraced this configuration format fully and it signals an optimistic future for
pyproject.toml
becoming standardized across the ecosystem.
Additional reading:
- PEP 518 — Specifying Minimum Build System Requirements for Python Projects
- PEP 621 — Storing project metadata in pyproject.toml
- Flit’s pyproject.toml file
- Poetry’s pyproject.toml file
When we delve into Python programming, one file that stands out due to its critical importance for Python projects is the
pyproject.toml
file. Essentially, it is a configuration file used by various tools that are utilized in the Python ecosystem.
Looking at the nomenclature,
.toml
is an acronym for Tom’s Obvious, Minimal Language, which is a data serialization standard that allows for straightforward yet comprehensive document structuring. Think along the lines of JSON, YAML and INI, but more powerful and user-friendly. The
Now, let’s understand the actual function of a
pyproject.toml
file:
-The philosophy behind its birth was to attend to the fragmentation issue among Python packaging tools (like setuptools, distutils, pip, etc). It provides a unified interface, enforcing all tools to refer to
pyproject.toml
for necessary project metadata.
-At its core, this file helps define build system requirements—as originally proposed in PEP 518. Consequently, it guarantees that specified software packages have the correct version when building the distribution package.
-By utilizing PEP 621 (a more recent proposal),
pyproject.toml
can also store project metadata such as name, version, description, authors, and so forth.
-Additionally, it’s often used to store configurations for various other development tools like black, flake8, isort, mypy, etc.
Taking an example,
[build-system] requires = ["setuptools", "wheel"]
In the example above, specifying the build-system table and requires list indicates that to build this package, both setuptools and wheel should be installed.
Here’s an example of setting up project metadata with PEP 621:
[project] name = "my_project" version = "0.1.0" description = "This is an example project." authors = [ {email = "you@example.com"}, ]
With this structure, you’re informing about your project’s name, version, description, and author.
Let’s extend this to include tool configuration, say for black:
[tool.black] line-length = 88 exclude = 'src/extern/'
This snippet customizes black’s configuration, setting a specific line length constraint and excluding a directory from being checked.
It’s important to note that while most modern tools support
pyproject.toml
, some could still be incompatible—although the Python packaging authority continues working to make it the standard.
Given its extensive capabilities, you can see why a proper understanding and usage of the
pyproject.toml
file is essential in Python coding, particularly in larger projects requiring meticulous dependency and configuration management.The `Pyproject.toml` file serves as a critical and central configuration file in Python packaging. This file outlines how your project should be packaged, including steps like building the package and installing it. Not only does `Pyproject.toml` strengthen component management, but it also enhances build dependencies and supports standard configuration maintenance.
Historically, Python developers experienced challenges in managing different setup files. However, PEP 518 initiated the utilization of `Pyproject.toml` to prevent such challenges. But, what makes this so important?
The Role and Importance of Pyproject.toml
Ideally, the `Pyproject.toml` file gives Python developers control over:
- Project Metadata: This includes details about your project, such as its name, version, author, description, etc., using the
[tool.poetry]
table in the project setup. It’s a systematic way of listing crucial project details, making it easier for other programmers and users to identify what your software is all about.
- Dependencies Management: The file defines your project dependencies in the
[tool.poetry.dependencies]
section and development dependencies in the
[tool.poetry.dev-dependencies]
section. It covers the accurate versions of your software’s dependencies, reducing inconsistencies that may arise due to changes in external libraries.
- Building and Packaging: `Pyproject.toml` guides the build system on how to package your software including library compilation, code conversion, etc. using the
[build-system]
table. With this, you can configure the build systems without having to change your source code every time.
A basic example of a `Pyproject.toml` file looks like this:
[tool.poetry] name = "myproject" version = "0.1.0" description = "A sample Python project." authors = ["Your Name"] [tool.poetry.dependencies] python = "^3.8" [tool.poetry.dev-dependencies] [build-system] requires = ["poetry-core>=1.0.0"] build-backend = "poetry.core.masonry.api"
This file outlines a straightforward plan — setting up a new “myproject” project with Python 3.8 as its main dependency.
In relation to SEO, the `Pyproject.toml` file is not directly involved. However, by contributing to a flexible, efficient, and manageable coding environment, it indirectly benefits SEO. Better-structured projects facilitate better collaboration, fewer bugs, faster updates deployment, which positively reflect on the SEO integrity of web applications built with Python.
Resorting to reliable resources when working with `Pyproject.toml` is advisable. Check out the official Python PEP 518 guide for more insights. You can also expand your knowledge about specific elements of the file using the official Python Poetry documentation.
Overall, the `Pyproject.toml` file is on track to becoming a one-stop-shop solution for Python developers to manage their projects’ configuration. It cuts down multiple-file complexity, offering an organized approach to define metadata, manage dependencies, and handle build settings.
Certainly! We can venture into the realm of Python packaging by discussing two crucial components:
setup.py
file and
pyproject.toml
file.
In order to fully understand the relevance and importance of the
pyproject.toml
file, we first need to cover the significance of the
setup.py
file. In the early days of Python packaging,
setup.py
was the standard workhorse — a build script for setuptools, which automatically builds, packages and installs your Python project. This script is run when you install a package using
pip install
or do a local installation using python
setup.py install
. Its main function involves providing the metadata (like name, version, description) and specifying dependencies to setuptools.
Here’s an example of what setup.py may look like with a fictional ‘example_pkg’ package:
from setuptools import setup, find_packages setup( name="example_pkg", version="0.0.1", description="A useful module", author="John Doe", author_email="john@doe.com", packages=find_packages(), )
However, setups using the
setup.py
file have bred some issues due to its inability to declare build system dependencies leading to occasional problems in the package building processes.
This is where
pyproject.toml
comes into play. Python Enhancement Proposal 518 (PEP 518)[source] introduced this new configuration file. The objective is to enable the declaration of all build dependencies in one place – namely, the
pyproject.toml
file.
What makes
pyproject.toml
particularly advantageous is that it aims to provide a static, declarative configuration method. Unlike
setup.py
, which is a dynamic execution script,
pyproject.toml
is a structured file that contains all the necessary information about the project that does not need parsing.
For instance, if our hypothetical package needed a specific version of setuptools, here’s how we could use pyproject.toml to ensure this:
[build-system] requires = ["setuptools>=40.1.1"] build-backend = "setuptools.build_meta"
Thus, while both files serve a purpose related to the configuration of Python projects,
pyproject.toml
has been championed as a powerful, standardized solution working towards streamlining the all too important task of error-free packaging and distribution for Python developers. Moreover, it is considered the future of Python packaging standards, demonstrating its clear relevance in the Python ecosystem.
With an understanding of these differences in mind, now let’s answer your question about the relevance of the
pyproject.toml
file.
The
pyproject.toml
is tremendously significant for Python development. It is intended to store the build system requirements, thus replacing the functionality previously handled by the
setup.py
file. This ensures that all common tools used in Python can be configured from just this one file — making it hugely convenient for developers.
From specifying versions for pip to declaring project metadata, build dependencies, or configuring tools like Black and pytest, the
pyproject.toml
facilitates diverse operations. It offers a potential for significant simplification across different tools, something that’s highly anticipated and recognized as part of PEP 518[source].
In essence, the aim of the Python community with
pyproject.toml
is to eventually make it the base of all project-related configuration, retaining the role of
setup.py
in limited use cases. The progression of making
pyproject.toml
the dominant player greatly enhances the predictability and robustness of Python packaging and deployment, especially for large scale projects with complex dependencies.Understanding the pyproject.toml file is essential for every Python developer as it serves vital roles in software management. These include dependency management and specifying build system requirements, among others. To understand this better, let’s dive deeper and explore the significance of this file.
Defining pyproject.toml: The Manifest for Modern Python Projects
In essence, the pyproject.toml file can be likened to other manifest files in different programming languages; similar to package.json in JavaScript or Gemfile in Ruby. This file, specifically in the .toml format (Tom’s Obvious, Minimal Language), keeps metadata about your project and delineates aspects such as using Poetry for dependency management.
[build-system] requires = ["poetry-core>=1.0.0"] build-backend = "poetry.core.masonry.api" [tool.poetry] name = "my-package" version = "0.1.0" description = "A detailed examination of the pyproject.toml file." authors = ["Your Name"] [tool.poetry.dependencies] python = "^3.7" requests = "^2.25.1" [tool.poetry.dev-dependencies] pytest = "^6.2.2"
The above sample code illustrates a pyproject.toml file managed by Poetry. From dependency management to providing necessary metadata, all areas are covered here.
Parsing the pyproject.toml File
Now that you have an understanding of the importance of pyproject.toml, let’s shift our attention to parsing this file. Python’s built-in configparser module does not support TOML syntax, hence we have to fall back on third-party libraries such as toml or uiri/toml.
To parse the file, you’d have to encode and decode the text in the .toml file. Let’s use the toml python library to illustrate this:
import toml # Read the file with open('pyproject.toml', 'r') as file: data = toml.load(file) # Accessing values print(data['tool']['poetry']['dependencies'])
The snippet of code above demonstrates how you would handle loading and parsing the contents of the .toml file using the
load()
function in our preferred toml library.
Situation-Specific Uses of pyproject.toml
Different setups may require specific fields in the pyproject.toml file. Here’s a glance at some:
1. Define Build System Requirements:
[build-system] requires = ["setuptools", "wheel"] build-backend = "setuptools.build_meta"
2. Black Code Formatter:
[tool.black] line-length = 79 target-version = ['py36']
3. Flit for Packaging:
[build-system] requires = ["flit_core >=2,<4"] build-backend = "flit_core.buildapi" [tool.flit.module] py_module="sample_module"
Remember, these variations do not discount the essence of pyproject.toml but rather fortify its significance by offering diverse options tailored to specific project needs.
This answer gives a quick overview of pyproject.toml, its importance in declaring metadata and managing dependencies, and parsing the data from this project descriptor. It's beyond doubt that pyproject.toml is a game-changer in python projects configuration, offering flexibility and convenience in project setup.Creating an efficient Python project using .toml files, most especially the "pyproject.toml" file, is a practice that caters to modern conventions in Python software development. It incorporates all configuration data for your Python project and also standardizes setup procedures – a major upgrade from earlier practices of having disorganized, multiple configuration files scattered across the repository.
[build-system] requires = ["setuptools", "wheel"] # These become build requirements. build-backend = "setuptools.build_meta" # Here we specify the build backend.
So, what exactly is pyproject.toml file for?
The pyproject.toml is pivotal for PEP 518 and PEP 621 which represent improvements in Python packaging. PEP 518 introduces a method of declaring dependencies needed to build a source distribution, while PEP 621 provides a way to specify project metadata.
Pyproject.toml file can be used to specify:
• Dependencies required to build your project
• The build system you are using
• Metadata about your project like version, description and url (PEP 621)
This is how you can use .toml files, including "pyproject.toml," to lay out an organized, scalable, and efficient Python project:
1. **Specify Build System**: Within "pyproject.toml," designate your build system and its prerequisites under the [build-system] section.
[build-system] requires = ["poetry>=0.12"] build-backend = "poetry.masonry.api"
2. **Declare Project Metadata**: Project information, such as name, version or author can be declared. This allows for transparency regarding the project's identity and current version status.
[tool.poetry] name = "helloworld" version = "0.1.0" description = "A simple Hello World program." authors = ["Your Name"]
3. **List Dependencies**: For a project to run effectively, it may require certain packages. The "pyproject.toml" file creates an organized environment where these can be listed.
[tool.poetry.dependencies] python = "^3.7" requests = "^2.22"
4. **Dev Dependencies**: Similar to dependencies, but are only used during development. They are not installed with the main application when deploying.
[tool.poetry.dev-dependencies] pytest = "^5.2"
5. **Create script entry points** : If you want your application callable similar to command-line tools. Currently supported by poetry build system.
[tool.poetry.scripts] yourcommand = 'yourpackage.yourmodule:main'
Following this structure will help maintain efficiency throughout your Python project development phases. Most importantly, it ensures that your project setup gains compatibility with current Python packaging standards, increasing the maintainability and accessibility of your projects.source.As your Python project’s complexity starts to grow, it becomes increasingly challenging to manage dependencies and configure settings. The PyProject.Toml file seeks to address these challenges by providing a standardized configuration file for Python projects. This is part of the PEP 518 initiativePEP 598 which aims to increase the predictability and reproducibility of Python projects.
The pyproject.toml file is basically notation language tomato, hence the ".toml" in its name. Like JSON or yaml, it's a way to represent data as text that's easy for humans to understand and write, but also simple for computers to generate and parse.
Structure of PyProject.Toml File
The basic structure of a PyProject.Toml file consists of key-value pairs grouped together under different section headers, which are enclosed in square brackets like so:
[tool.poetry] name = "my_library" version = "0.1.0" [build-system] requires = ["poetry-core>=1.0.0"] build-backend = "poetry.core.masonry.api"
In the above example, we have defined two sections: "tool.poetry", which specifies metadata about our project, and "build-system", which configures our build dependencies.
Advanced Features
Beyond this basic use-case, the PyProject.Toml file offers much more advanced features:
Custom Scripts: You can define your own scripts in the [tool.poetry.scripts] section like this:
[tool.poetry.scripts] start = "python main.py"
These scripts can then be run using the poetry command-line interface.
Package Configuration: Here you can specify the metadata and configuration options required for packaging and distributing your Python package. This could include details like package name, version, author, description and various other fields.
[tool.poetry] name="myproject" version="0.0.1" description="This is my first Python project." authors=["Your Name <youremail@example.com>"]
Specifying Dependencies:
You can use the PyProject.Toml file to specify both your project's system requirements and its library dependencies. This may include specifics such as the version of Python your project needs to run, along with any other Python libraries it relies on. For instance:
[tool.poetry.dependencies] python = "^3.7" numpy = "^1.18.1" scikit-learn = "^0.22.1"
Intricacies
The power of PyProject.Toml comes with some intricacies that need to be understood and managed effectively:
Order of Dependencies: It's worth noting that the order in which you list your dependencies in the PyProject.Toml file matters. Python will install the dependencies in the order they are listed.
Error check: Errors may occur in your filename or path due to unsupported characters. Though it's rare, being mindful of this can save you from future headaches.
Data types: TOML supports standard data types like integers, floats, booleans, strings, datetime objects, arrays, and tables. However, nested data structures have their limitations. For instance, you cannot directly define a list of dictionaries in TOML. Understanding these quirks will help you make the most out of this powerful tool.
To put it briefly, the PyProject.Toml file provides a standardized way to manage Python project configurations. By understanding how this file works, we can greatly simplify the process of setting up, maintaining, and collaborating on Python projects. Care should however be taken to use it efficiently and effectively for the best results, taking note of the order of dependencies and the limitations of nested data structures.
pyproject.toml
plays an instrumental part in the world of Python coding. It is a core file used to keep track of established project metadata and dependencies, granting you tremendous control over your project's structure.
Now, let's examine the key functionality benefits that
pyproject.toml
brings to the table:
- Predictability: Controlling the project layout better means no unpredictable default configurations will be placed. This tremendously aids your maintainability efforts.
- Better Dependency Management: Dependencies are packaged inside the
pyproject.toml
file, steering clear of multiple different configuration files, like
setup.py
,
requirements.txt
, etc. This proper packaging can also tighten security since unauthorized manipulations could be held at bay.
- Enhanced Production Quality: Better handling of dependencies takes place using
pyproject.toml
which ensures one thing; minimal discrepancies during production releases.
Taking advantage of the
pyproject.toml
file has allowed me to streamline my Python projects in more ways than one. Infrastructure becomes orderly with its usage. It eliminates redundancies by replacing multiple configuration files with just one. Dependencies are easier to manage, thanks to the robust schema it follows.
To provide a quick example, let's consider setting up a simple dependency. Here is what the
pyprogram.toml
might look like:
[build-system]
requires = ["setuptools", "wheel"]
[tool.poetry]
name = "my-awesome-python-project"
version = "0.1.0"
description = "Demonstration of pyproject.toml."
authors = ["Author
[tool.poetry.dependencies]
python = "^3.7"
[tool.poetry.dev-dependencies]
pytest = "^5.2"
This succinctly packages all the project dependencies within the normative framework of
pyproject.toml
.
For anyone who loves to see their Python projects neatly structured and organized, harnessing the power of the
pyproject.toml
file will prove greatly beneficial. With a sharp focus on total project control, it's indeed a Python coder's dream come true for a smoother programming ride.
To explore more about how to use
pyproject.toml
file effectively, I highly recommend visiting this [Python Packaging User Guide](https://packaging.python.org/tutorials/managing-dependencies/#managing-dependencies) published by Python.org. Happy Coding!