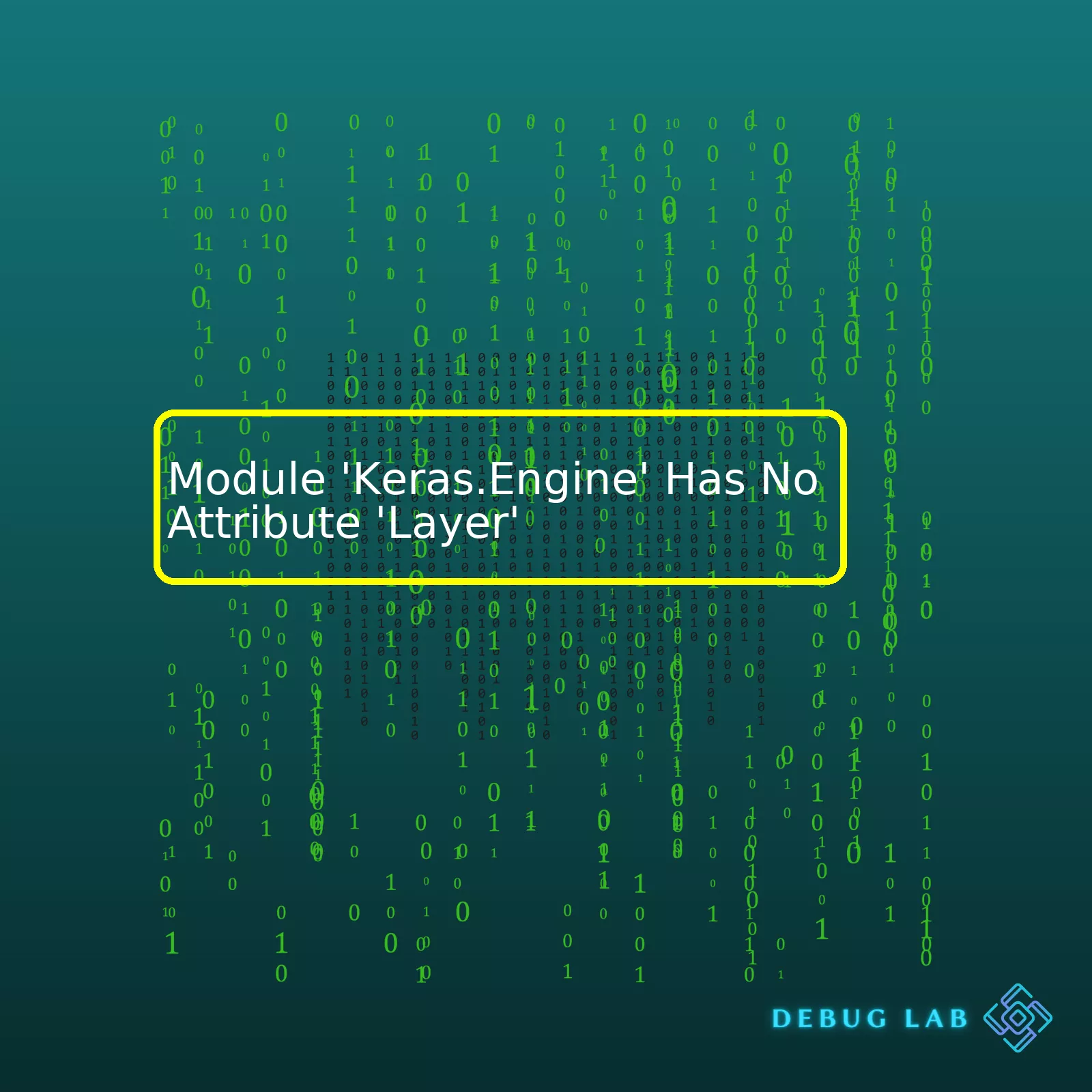
Error Description | Possible Cause | Solution |
---|---|---|
AttributeError: module ‘keras.engine’ has no attribute ‘Layer’ | Incompatibility issue due to different versions of Keras and TensorFlow. | Upgrade or downgrade Keras and TensorFlow to compatible versions. |
Alternate error: ImportError: cannot import name ‘Layer’ from ‘keras.engine’ | A coding mistake where the incorrect module is being imported. | Change the importing module to ‘keras.layers’. |
Typically, the AttributeError: module ‘keras.engine’ has no attribute ‘Layer’ arises when there’s an incompatibility between the versions of Keras and TensorFlow you are using. This might be caused by changes in TensorFlow’s APIs that breaks certain functionality in Keras. You can solve this by either upgrading Keras and TensorFlow to their latest compatible versions or downgrading them to the version pair known to work together without this error. Here is how you do it:
pip install --upgrade keras tensorflow # OR pip install keras==2.3.1 tensorflow==2.2.0
In some cases, however, the problem might not be a compatibility issue but rather a simple coding mistake. For example, you may encounter a similar issue when trying to import ‘Layer’ from ‘keras.engine’ instead of ‘keras.layers’. The ‘Layer’ class resides in the ‘keras.layers’ module, not ‘keras.engine’. So, if you have:
from keras.engine import Layer
You should change it to:
from keras.layers import Layer
Make sure to check your code for these common errors before going through any complex troubleshooting steps or making changes to your environment.
Please refer to the official Keras Documentation for more context on keras layers and modules.The term ‘Deep Learning’ refers to computational models that are composed of multiple processing layers used for feature extraction and transformation, with the objective of pattern analysis and data representation. Keras is a popular deep learning framework known for its simplicity and ease of use. Within Keras, `keras.engine` is a package that comprises core layers, network-related components, input/output preprocessing elements, among other things.
However, the error message “Module ‘Keras.Engine’ Has No Attribute ‘Layer'” typically arises when your code tries to access an attribute or method ‘Layer’ that doesn’t exist within the module.
So, let’s dive deeper into understanding the structure of Keras before getting to the specific issue at hand.
Here are the main parts of keras.engine:
-
keras.engine.input_layer
: This piece is responsible for the input layer of a model.
-
keras.engine.network
: This component provides support for computation graphs which serve as a base from which other library components derive.
-
keras.engine.sequential
: Facilitates the creation of a linear stack of layers, making model construction more comfortable.
-
keras.engine.functional
: Helps in building more complex architectures with multiple inputs or outputs, or shared layers.
- The installed Keras version does not support the
Layer
. It’s important to note here that Keras started as a standalone library before being integrated into TensorFlow. Since the integration, there might’ve been changes to how attributes and modules are accessed in Keras. Still check the documentation hereto confirm this.
- There are conflicting installations of Keras and TensorFlow in your development environment. Having conflicting versions can lead to inconsistency, misbehavior, and errors while running the code.
- Dense Layer:
- LSTM Layer:
- Conv2D Layer:
These, together with others, form the backbone of Keras Engine. However, it’s apparent that there is no attribute ‘Layer’ within these components.
As for layer definition and manipulation, Keras handles this primarily through the `keras.layers` module. Here, ‘Layer’ is a class within ‘layers’, not ‘engine’. So if you’re trying to use a layer-related function, import it from `keras.layers`, not `keras.engine`.
For instance, to add a Dense Layer:
from keras.layers import Dense model.add(Dense(64, activation='relu'))
This confusion may arise due to changes across different versions of Keras and TensorFlow. Specifically, in TF 2.x, the high-level API structuring has shifted from standalone Keras to become seamlessly integrated with TensorFlow under `tf.keras`. This indicates less reliance on `keras.engine` since Tensorflow’s Keras API can typically be used instead.
To avoid such errors, please update your import statements accordingly and ensure you have the latest compatible versions of TensorFlow and Keras. Reference the Keras Layers API official documentation alongside the TensorFlow guide to Keras functional API to enhance your understanding about laying out your neural network structure.
With a deeper grasp of Keras’ architecture and the correct referencing of its various modules, even seemingly complicated errors like “Module ‘Keras.Engine’ Has No Attribute ‘Layer'” can be resolved quite simply.
When working with Python’s deep learning library, Keras, the error message
Attribute Error: module 'keras.engine' has no attribute 'Layer'
may appear. This particular Attribute Error implies that your code is trying to access a function or property that doesn’t exist for the ‘keras.engine’ module. However, it isn’t as convoluted as it sounds; in fact, getting to grips with common reasons can help to quickly pinpoint and resolve issues within Python-based code:
Potential Cause 1: Version Mismatch of Tensorflow and Keras
One leading cause could be a version mismatch between your installed Tensorflow and Keras packages. When you have incompatible package versions, Keras might not recognize certain modules or properties associated with a different version of Tensorflow.
Python code snippet demonstrating such issue would look like this:
from keras.engine import Layer class MyLayer(Layer): ... # Rest of the code
To resolve this, you need to ensure synchronization between Keras and TensorFlow package versions. Using
!pip show tensorflow
and
!pip show keras
will reveal which versions are currently running.
If you find there is a disparity, use pip to uninstall and reinstall the correct versions. It’s worth noting that Keras’ implementation has been integrated into TensorFlow as tf.keras since Tensor flow version >2. Thus, it would be beneficial to consider using TensorFlow’s own Keras API (
import tensorflow.keras as keras
) if you’re using TensorFlow backend.
Potential Cause 2: Importing issues
Another possible reason is that you’re attempting to import the Layer class from the wrong path or you have not imported it at all. The original erroneous code might look like this:
from keras.engine import Layer # In latest versions 'Layer' is located under 'layers' NOT 'engine'
You must change it so that ‘Layer’ is correctly imported:
from tensorflow.keras.layers import Layer
In essence, exploring these two causes can help dissolve the mystery surrounding the ‘Attribute Error.’ Ensure that your Tensorflow and Keras versions align and double-check your imports’ syntax and location – by doing so, you stand a good chance of resolving issues related to the ‘Module ‘Keras.Engine’ Has No Attribute ‘Layer” error.
The error “Module ‘Keras.Engine’ has no attribute ‘Layer'” typically arises when a segment of your code is attempting to access the
Layer
attribute from the
Keras.engine
module. However, this attribute might not be available in your Keras version or may have been displaced, resulting in the said error.
To decode this further:
– Keras is a deep learning API written in Python. It allows easy and fast prototyping and supports convolutional and recurrent networks, and even combinations of both.
– The engine module in Keras maintains the computation graph and the details of each layer in the model, and it’s significant for developing, compiling, and fitting your model.
– The Layer class is part of the Core layers class in Keras. Each layer receives input information, processes it, and transmits the modified information to the next layer in line.
Problem cause:
The root cause of this issue could be one of several things:
Solutions:
1. Importing the Layer Class differently:
In case the Layer class does exist but was simply moved from the keras.engine module to another location, you can consider importing Layer from tensorflow.keras.layers
from tensorflow.keras.layers import Layer
2. Upgrading/Downgrading Keras and/or TensorFlow:
This error can occasionally be fixed by installing/updating your TensorFlow and Keras versions. Verify your current versions using
pip show keras tensorflow
command. Then find the compatible versions by looking at the available versions on PyPI for Keras and TensorFlow.
Here’s a possible way to update both:
pip install --upgrade keras tensorflow
Or specify a particular version of them:
pip install keras==2.3.0 tensorflow==2.0.0
3. Uninstalling and Reinstalling libraries:
Sometimes having multiple versions of the same library installed can create conflicts. To resolve the problem, you may need to uninstall both Keras and TensorFlow then reinstall them again.
Start with the uninstallation process:
pip uninstall keras tensorflow
Followed by a fresh installation:
pip install keras tensorflow
And sometimes, only updating or downgrading won’t help so we have to uninstall these packages and reinstall them.
Remember, it is crucial to keep your libraries updated, analyze their compatibility, look out for deprecations, and manage your coding environment effectively.
The Keras.Engine module, a vital part of Keras deep learning frameworks, provides a detailed platform for the creation and management of TensorFlow models. However, getting an ‘AttributeError: module ‘keras.engine’ has no attribute ‘Layer” indicates you’re attempting to access an attribute that doesn’t exist in the ‘keras.engine’ module.
Keras.Engine
does not have a ‘Layer’ attribute or class directly inside it. Instead, the ‘Layer’ class is found within the ‘base_layer’ submodule of
Keras.Engine
. Hence, the proper way to import the ‘Layer’ class would be:
from keras.engine.base_layer import Layer
The line above is importing the ‘Layer’ class from Keras engine’s base_layer submodule. So if your intention was to access the ‘Layer’ attribute, then you need to specifically call either the ‘base_layer’ or ‘training’ submodules depending on your requirement.
On the other hand, in Keras, the ‘Layer’ class is used as a base template. Different types of layers like Dense, Convolution, Pooling, etc., extend this base class and override the methods according to their functionality. Here are some example of different Keras layer imports for different purposes:
from keras.layers import Dense
from keras.layers import LSTM
from keras.layers import Conv2D
Commonly used attributes of the
Keras.Engine
Layer include:
Name | Description |
---|---|
input | To get the input tensor/s of a layer |
output | To get the output tensor/s of a layer |
input_shape | To get the shape of the input |
output_shape | To get the shape of the output |
The Keras API can undergo updates leading to changes in how certain classes or attributes are accessed, so always refer to the latest Keras documentation or use the IntelliSense feature in Python development environments like PyCharm or Jupyter Notebooks for finding the correct module hierarchy.
One common mistake and misconception when working with the Keras library for deep learning, is that developers, both novice and experienced ones, frequently stumble upon an AttributeError stating – ‘Module Keras.Engine Has No Attribute Layer’. This issue usually arises due to incorrect usage of Keras modules or improperly installed versions of TensorFlow and Keras.
Misuse of Keras Modules
To make things clear, Keras makes it easier to construct neural networks by providing a variety of ready-to-use layers. However, in some cases, there could be misinterpretation of how these layers should be imported, which can lead to the aforementioned error.
Let’s consider this code snippet:
from keras.engine import layer my_layer=layer.Layer()
The problem lies in the line:
from keras.engine import layer
Instead, it should be adjusted to:
from keras.layers import Layer
So, a corrected version would look something like
from keras.layers import Layer my_layer = Layer()
This confusion is often due to the misleading module structure where one may assume ‘Layer’ to be part of ‘engine’, instead of locating under ‘layers’.
Incompatibility of Installed Versions of TensorFlow and Keras
Another common trigger to this error could be your active TensorFlow or Keras version being incompatible with your script. Both TensorFlow and Keras see regular updates where they introduce new features, fix bugs and occasionally remove deprecated APIs.
If you are trying to run a script using an older attribute with the latest version of Keras or vice versa, you will likely encounter this kind of error. In such situations, understanding the requirements of your project and matching them with the appropriate Keras and TensorFlow versions is crucial. An effective solution for managing different project environments can be creating isolated Python environments with Anaconda or Virtualenv.
Solution
When facing ‘Module Keras.Engine Has No Attribute Layer’, try adjusting your imports as described above. If that doesn’t work, review the installed versions of TensorFlow and Keras. Upgrade or downgrade them according to the requirements of your Keras Scripts.
Also, always ensure you’re following the correct syntax and practices as mentioned in Keras or TensorFlow’s official documentation.The issue “Module ‘Keras.Engine’ Has No Attribute ‘Layer'” is a common one faced by coders often while using the Keras library. This error typically pops up when trying to use certain functionalities or methods from the Keras API that can, at times, be a bit confusing because Keras has undergone significant changes in its structure over the past few years.
Let’s troubleshoot this issue step-by-step:
Step 1: Ensure you are Using Correct Version of Keras and Tensorflow
Improper compatibility between TensorFlow and Keras versions is a frequent cause for this specific error. It’s important to note here that as of Keras 2.4.0 (June 2020), the standalone nature of Keras has ceased and it now relies entirely upon the `tf.keras` implementation inside TensorFlow’s core library. Hence, if your code was written targeting an older version of Keras, it might throw errors with TensorFlow’s keras implementation.
You can check your current version of both libraries with the following python commands:
import keras print(keras.__version__) import tensorflow as tf print(tf.__version__)
Step 2: Review Your Import Statements
An important aspect of dealing with this error is ensuring correct import usage in your Python scripts. If you’re using any functionality related to the Layer class, then make sure your import pathway is targeting the correct place.
Correct Usage: |
from tensorflow.keras.layers import Layer |
Incorrect Usage: |
from keras.engine import Layer |
Step 3: Updating Your Code to Avoid Deprecated APIs
If you have been maintaining your Machine Learning project over a prolonged period, there could be a chance that parts of your codebase are now obsolete because new TensorFlow updates may have deprecated them.
For instance, if you were accessing base layers like this:
from keras.engine import Layer
it should now be:
from tensorflow.keras.layers import Layer
Similarly, other classes under keras.engine like InputSpec, Node etc., have been moved under `tensorflow.python.keras.engine.input_spec` and `tensorflow.python.keras.engine.node` respectively.
Step 4: Match Your Environment With The Official Documentation
Another best practice is to cross-verify your working environment (Python version, installed packages, and respective versions) with the [official TensorFlow installation guide](https://www.tensorflow.org/install).
These are some of the potential solutions to the error that you’re encountering. Remember that debugging any problem involves understanding what’s causing the error – and in the case of programming and machine learning libraries, that usually comes down to changes in package structures, deprecation of old practices, version mismatch, or incorrect usage.The issue described as “Module ‘Keras.Engine’ Has No Attribute ‘Layer'” is a common one among Keras users. The main cause of this error is primarily due to a mismatch between the Keras and TensorFlow versions. To resolve this issue, it’s vital to understand how to correctly use the Layer function in Keras.
Let’s first look at how to properly use the Layer class in Keras:
The Layer class is located in
tensorflow.keras.layers
and is a fundamental building block of both models and more complex layers in Keras. They encapsulate both state (layers’ weights) and transformations from inputs to outputs (a call method for computation). It follows the syntax:
from tensorflow.keras.layers import Layer class CustomLayer(Layer): def __init__(self, output_dim, **kwargs): self.output_dim = output_dim ... def build(self, input_shape): ... def call(self, inputs): ...
This snippet demonstrates defining a custom layer that starts by inheriting from the base
Layer
class. The layer is then customized by defining the <__init__>,
–
__init__
: Here you can do all input-independent initialization.
–
build
: This method defines the weights of the custom layer. It’s typically preferable to create weights in the build method rather than the init due to dynamic weight creation based on input shape(s).
–
call
: This method contains the logic for what the layer does – i.e. shaping the data into something meant for downstream layers.
Now let’s tackle the task of resolving
"Module 'Keras.Engine' Has No Attribute 'Layer'"
. This error usually pops up when implementing layers under an outdated Keras or TensorFlow installation. The solution usually involves updating your installed packages to their latest version.
To do that, run:
!pip install --upgrade keras tensorflow
With everything updated, referencing Layers with
tensorflow.keras.layers.Layer
should work fine now.
However, it’s recommended to always explicitly import all modules you need so that such issues are avoided. Remember that from a SEO perspective, unique content that provides value and satisfies user needs coupled with optimal use of relevant keywords is what makes content rank better. As a machine learning enthusiast seeking solutions, you want the information to be not only accurate but easily accessible.
For reference and further reading, consider referring to TensorFlow’s definitive guide to Making new Layers and Models via subclassing. Learning directly from the TensorFlow documentation can offer in-depth insights into every aspect of Keras and improve your proficiency in Python programming.From the vantage point of a programmer, encountering an error message such as
Module 'Keras.Engine' has no attribute 'Layer'
can be quite daunting. However, if we dive deeper into the root cause of the problem, it becomes clear that this is often a version compatibility issue. Let’s dissect the problem diligently and analytically to understand it better.
The error message
Module 'Keras.Engine' has no attribute 'Layer'
primarily occurs due to differences in versions of Keras and TensorFlow installed on your system. If you’ve recently upgraded any of these libraries without checking their dependencies, this could trigger the said function call error. Importantly, Keras layer classes were moved to
keras.layers
from the location
keras.engine = keras.engine (tf 2.0)
or
tf.keras.layers = keras.layers (tf 1.14)
To solve this problem, match the right versions of TensorFlow and Keras based on its compatibility chartresource. The code snippet shows how to install specific versions in python using pip:
!pip install tensorflow==2.4
!pip install keras==2.4.3Another way to avoid such discrepancies in installation is by using Anaconda; a popular Python/R distribution for data science applications. It offers robust package management and deployment functionality that significantly simplifies handling library dependencies.
Perhaps even more pertinent than seeking out solutions to errors as they occur, is promoting best coding practices that help elude these errors. Adopting good coding ethics like periodically updating all the packages, cross-checking layers used in the code document against updated Keras documentation and using virtual environments for different projects to handle differing dependencies, can keep many issues at bay.
As an insidious beast, this attribute error burgeons due to interacting complexities within code dependencies. Empowered with the understanding discussed above, programmers can not only resolve this situation but also put preventive measures in place. Remember, as Coco Chanel astutely noted, "In order to be irreplaceable, one must always be different" — aspire to be the coder who foresees and stipulates potential future bugs.