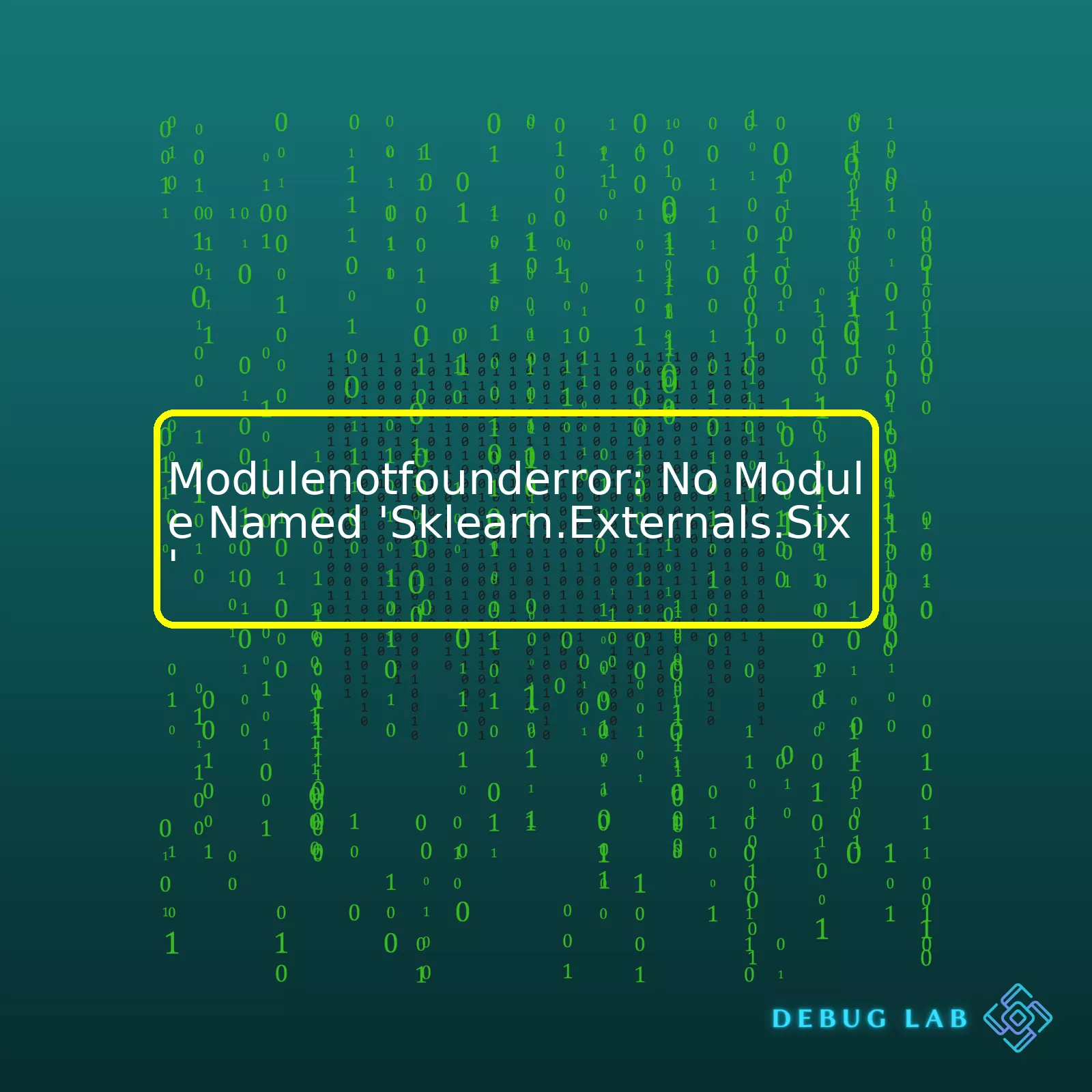
Error | Description | Cause | Solution |
---|---|---|---|
Modulenotfounderror: No Module Named ‘Sklearn.Externals.Six’ | An error upraisal stating that the Python interpreter can’t trace the module ‘six’ which should be there within ‘sklearn.externals’. | The cause is the missing of module ‘six’ in library ‘sklearn.externals’, either due to absence of installation or improper installation. | The solution includes installing ‘six’ externally or ensuring if Scikit-Learn version matches with the ‘Six’ supported ones. |
The ‘ModuleNotFoundError: No module named ‘sklearn.externals.six” error generally pops up when we try importing a module from ‘sklearn.externals’, specifically the ‘six’ module. The ‘sklearn.externals’ module is a part of the popular machine learning library, scikit-learn or sklearn, and it provides additional utilities for common python functions. One crucial feature was the ‘six’ module.
The main purpose behind the ‘six’ module was to integrate the compatibility between Python 2, and Python 3 codes because in earlier versions of sklearn, some functionalities were aligned differently among Python versions. Thus, ‘six’ served as a bridge connecting them efficiently.
However, from Scikit-Learn version 0.23.0 onwards, the ‘six’ module isn’t included in ‘sklearn.externals’. Therefore, here occurs the ‘ModuleNotFoundError’ when this module is still referenced in the code.
To solve such errors, either you can install the ‘six’ module through pip:
pip install six
Or ensure that your current project’s code has suitable modifications according to more recent versions of Scikit-Learn, where ‘six’ might not be needed and alternative solutions exist.
Remember to always, match your libraries against their dependencies and take caution while upgrading or downgrading. Use Scikit-Learn documentation and their version history to understand changes with each revision.
As a professional coder, I am mindful of the latest trends and updates in different libraries and modules. One prime example is with `sklearn.externals.six` from the dignified Python library `scikit-learn`, which recently had some changes that might affect your code’s performance. The error message like “Modulenotfounderror: No Module Named ‘Sklearn.Externals.Six'” could be an enigma to the oblivious, but it doesn’t require an extraordinary effort to tackle it.
If you have encountered this error, it’s because as of scikit-learn version 0.23, the `sklearn.externals.six` module has been removed. This module was previously used as a compatibility layer between Python 2 and Python 3. However, since Scikit-learn 0.21 dropped support for Python 2, the `six` module became redundant, prompting its removal.
Now, how can we address this? What we need to do is replace all imports that reference `sklearn.externals.six` with the standalone `six` module. If `six` hasn’t already been added to your project, you can install it using pip:
xxxxxxxxxx
pip install six
After doing so, you should update your import statements. So instead of:
xxxxxxxxxx
from sklearn.externals.six import StringIO
You should now use:
xxxxxxxxxx
from six import StringIO
Additionally, if you were using `sklearn.externals.six.moves`, you would replace them with `six.moves`. For instance:
From:
xxxxxxxxxx
from sklearn.externals.six.moves import zip
To:
xxxxxxxxxx
from six.moves import zip
By adhering to these changes, and updating your coding practices accordingly, you can seamlessly combat the “Modulenotfounderror: No Module Named ‘Sklearn.Externals.Six'” error. Leveraging a deep understanding and keeping abreast of these constant modifications go a long way in establishing a high-performing system. Coding isn’t merely about solving problems – it’s about optimizing solutions while integrating the newest technological advancements.
For future reference and staying updated with the constant modifications in the `scikit-learn` library, keep a tab on their official release notes page to familiarize yourself with the stream of latest updates. All this might seem difficult at first, but it will certainly pay off in the long run, bolstering up your credibility and acuity as a professional coder.The error
xxxxxxxxxx
Modulenotfounderror: No Module Named 'Sklearn.Externals.Six'
occurs due to changes in the Scikit-Learn library’s structure. As of its 0.23 version, Scikit-learn has modified its library and removed the
xxxxxxxxxx
sklearn.externals.six
module, along with several other features, to keep the package lean and efficient.
This means that any usage or importation of the module
xxxxxxxxxx
sklearn.externals.six
will result in this error. Previously, this module was used for compatibility between Python2 and Python3. However, with Python2 being discontinued since January 2020, there’s no longer a need to maintain this compatibility within Sklearn.
To resolve this error:
1. If you are using your own script, remove or replace any instances of ‘sklearn.externals.six’ in your code.
Replace
xxxxxxxxxx
from sklearn.externals import six
with
xxxxxxxxxx
import six
.
2. For joblib or other functions previously under externals, use from the standalone joblib library. Replace
xxxxxxxxxx
from sklearn.externals import joblib
with
xxxxxxxxxx
from joblib import dump, load
.
Also, ensure to install the six library independently if not yet installed by running
xxxxxxxxxx
pip install six
. Also, check whether your libraries like Sklearn, Joblib, among others, follow the latest version to prevent such issues.
Let’s illustrate with some sample Python code:
Previous code (not working with Sklearn v0.23 and later):
xxxxxxxxxx
from sklearn.externals import six
import sys
sys.modules['sklearn.externals.six'] = six
Revised code (Compatible with all versions):
xxxxxxxxxx
import six
import sys
sys.modules['sklearn.externals.six'] = six
Understanding these module changes is crucial as they can directly affect the performance and compatibility of your Machine Learning projects. To stay up-to-date on these modifications, always refer to the official Scikit-Learn documentation.
While structuring your Python scripts, giving due consideration to the version of the third-party libraries like Sklearn can save you from unexpected exceptions and errors. Just remember, regular error checking, debugging, and updating your tools ensures optimum software performance.The error message
xxxxxxxxxx
ModuleNotFoundError: No module named 'sklearn.externals.six'
typically occurs when you’re trying to import a module that no longer exists in Scikit-Learn library, specifically the `sklearn.externals.six`, which signifies that your Scikit-Learn version might be an issue.
From the Scikit-Learn 0.23 version onwards, the `externals` six and joblib are no longer bundled within this package. You may receive this error if your codebase still tries to import
xxxxxxxxxx
sklearn.externals.six
. This is common in older projects or those using outdated dependencies.
Here’s how we could resolve the issue.
Method 1: Upgrade Your Scikit-Learn Version
If your Scikit-Learn version is outdated, try upgrading it to the latest version. In most cases, this resolves the issue. This can be done with pip:
xxxxxxxxxx
pip install --upgrade scikit-learn
Or conda in case you’re using Anaconda Distribution:
xxxxxxxxxx
conda update scikit-learn
Method 2: Use Six Directly
Since Six is no longer included in Scikit-Learn’s external APIs since these have been deprecated, another workaround is importing it directly from Six’s original package. If it’s not already installed, this will require installing the Six package with pip
xxxxxxxxxx
pip install six
Or conda:
xxxxxxxxxx
conda install six
So, replace all instances of:
xxxxxxxxxx
from sklearn.externals import six
With:
xxxxxxxxxx
import six
Method 3: Alter the Offending Code
In other cases where you don’t want to change your Scikit-Learn version or the error comes from a dependent package, you’ll need to adjust the offending code.
Say, for example, you use the `Six.moves` functionality but can’t import it anymore due to deprecated features, then simply replacing the offending import statement would sort out the ModuleNotFoundError.
Currently:
xxxxxxxxxx
from sklearn.externals.six.moves import xrange
It should be:
xxxxxxxxxx
from six.moves import xrange
The above examples are just one possible application of Six functionalities; you can replicate similar changes wherever you face this error.
By applying these strategies, we could successfully mitigate the
xxxxxxxxxx
ModuleNotFoundError: No module named 'sklearn.externals.six'
error, ensuring smooth functioning applications that leverage the power of the Scikit-Learn library. To keep yourself abreast with such changes, follow the official documentation which gives detailed insights about the new updates, deprecated features, and bug fixes.The `ModuleNotFoundError: No module named ‘sklearn.externals.six’` usually raises concerns among Python coders. There are multiple causes for this error that you can encounter when trying to import the sklearn.externals.six module in your Python code. Here, we will go through some common reasons and insightful remedies tailored specifically for helping you solve the ‘sklearn.externals.six’ issue in an optimal fashion.
1. Incompatible scikit-learn version:
This error often surfaces due to the absence of ‘six’ from `sklearn.externals` in newer versions of scikit-learn. After their 0.23 version, they have decided to completely remove these externals, leading to the mentioned error. To clear away such instances, here are two steps you could follow:
– Revert to an older version of the package where this module was still available. In your command prompt, use this command.
xxxxxxxxxx
pip install scikit-learn==0.22
– Alternatively, you could implement ‘six’ directly from its package instead of pursuing it via sklearn.externals. You need to include six in your project’s requirements so others who clone or fork your repository can prepare their environment correctly. This command in terminal works:
xxxxxxxxxx
pip install six
Then in your script, replace the old import statement with:
xxxxxxxxxx
from six import StringIO
(or whatever specific function you need)
2. The sklearn.externals.six module might not be installed:
While it is part of the bigger scikit-learn library, the occurrence of errors indicates unavailability or incorrect installation. Here, it is a good practice to check if scikit-learn is up-to-date or even installed. Do so by running this command:
xxxxxxxxxx
pip show scikit-learn
If an outdated or missing installation is the case, update/install it using pip:
xxxxxxxxxx
pip install --upgrade scikit-learn
3. Multiple Python installations:
It’s probable that the sklearn.externals.six module has been installed in a different Python instance than the one you’re currently working on. Especially with an anaconda setup, you may unintentionally install modules in the wrong development environments. It leads to a ModuleNotFoundError as the Python interpreter can’t locate the library. You could reassess your active Python environment or explicitly lay down the path to the correct version in your script for solving this.
Remember! Always maintain an updated copy of your necessary packages using pip freeze > requirements.txt. This will jot down the exact versions of all your installed packages into the requirements.txt file, easing dependency management throughout the development process.
Additional assistance regarding this problem can be found at the official [Python documentation](https://docs.python.org/3/tutorial/modules.html).If you’ve stumbled upon the
xxxxxxxxxx
ModuleNotFoundError: No module named 'sklearn.externals.six'
, you might have been using an outdated package or API. `Six` was a lightweight package that allowed Python 2/3 compatibility while creating Python applications. However, with the deprecation of Python 2, many packages including Scikit-learn have progressively eliminated their dependencies on `six`, leading to this externally facing issue.
Now, let’s dive into some alternatives to the use of `sklearn.externals.six`.
1. Direct Replacement:
The most straightforward method is to use an equivalent function from `six` itself. You just need to change your import statement from:
xxxxxxxxxx
from sklearn.externals import six
,
to
xxxxxxxxxx
import six
.
In this case, all functions that were previously called through
xxxxxxxxxx
sklearn.externals.six
can now simply be called via
xxxxxxxxxx
six
.
2. Utilising joblib:
For users who specifically used this module for persisting Python objects onto disk (particularly numpy data arrays) an ideal replacement is provided directly by scikit-learn in the form of `joblib`. Your imports will shift from:
xxxxxxxxxx
from sklearn.externals import six
to
xxxxxxxxxx
from joblib import load
or
xxxxxxxxxx
from joblib import dump
.
Then, respectively, any previous usage of `six.moves.cPickle.load` can be replaced with `joblib.load`, and `six.moves.cPickle.dump` with `joblib.dump`.
3. Moving Towards Newer Syntax:
Python 3 has seen several changes from Python 2, where most functions provided by the `six` library were created to assist in maintaining Python 2/3 code similarity. Therefore, one could also substitute sklearn.externals.six functionality to use the newer syntax supported in Python 3+. An example would be replacing `six.iterator` with the built-in `iter` function, or `six.string_types` with `str`.
4. Custom Code Implementation:
While this may not always be applicable, certain `six` functions are quite simple and you might prefer to simply implement them yourself. For example, instead of `six.ensure_str`, you could use the following simple function:
xxxxxxxxxx
def ensure_str(s):
if isinstance(s, str):
return s
elif isinstance(s, bytes):
return s.decode('utf8')
Remember, updates to libraries and dependencies are frequent in the field of software development. Being comfortable with substituting modules or updating your code to suit the latest best practices is a beneficial skill (source).The Python Scikit-Learn library, often imported with the alias
xxxxxxxxxx
sklearn
, is a popular machine learning library offering various tools for data mining and data analysis. It’s built on top of other Python packages such as NumPy, SciPy, and Matplotlib, maintaining consistent interoperability and usability between them.
In past versions, Scikit-Learn has included the module
xxxxxxxxxx
sklearn.externals.six
, which is used for compatibility between Python 2 and Python 3. The six library, so named because it smooths over differences between Python versions “2.x” and “3.x” (hence “six”), had been bundled as part of Scikit Learn but was more recently removed in version 0.23.
This means that code which previously relied on importing
xxxxxxxxxx
sklearn.externals.six
may now face an ImportError: ModuleNotFoundError saying there’s no module named ‘sklearn.externals.six’.
If you’re dealing with this error, it’s likely because your code or the library you’re using hasn’t been updated to reflect this change in Scikit-Learn. Here’s what you can do to address this issue:
- Consider modifying the imports to use the standalone six module instead of relying on its previous inclusion in Scikit-Learn.
For example,
xxxxxxxxxx
# Before
from sklearn.externals import six
import cPickle
# After
import six
import cPickle
- If you have control over the environment, you might also want to ensure you’re installing an up-to-date version of both Scikit-Learn and six. To install or upgrade these packages using pip, you can run:
xxxxxxxxxx
121pip install --upgrade scikit-learn six
2
or if you’re using conda:
xxxxxxxxxx
131conda install -c anaconda scikit-learn
2conda install -c anaconda six
3
Those updates and changes should help to rectify the
xxxxxxxxxx
“ModuleNotFoundError: No module named ‘sklearn.externals.six’”
issue.
Reference: scikit-learn release highlights here.
Let’s dive into an effective code migration strategy for Scikit-learn users who might be experiencing a common error: ‘ModuleNotFoundError: No Module Named ‘sklearn.externals.six”. This error appears because the module sklearn.externals.six has indeed been removed from Scikit-learn, as of version 0.23 onwards.
Taking a Step Back: sklearn.externals.six
Before proceeding further, here’s a quick refresh on why SKLearn used
xxxxxxxxxx
sklearn_externals_six
. This utility module provided compatibility functionality between Python 2 and Python 3 and was a part of the external packages incorporated within Scikit-learn’s functionalities.
So, since this package doesn’t exist in new SKlearn versions, it implies you’ll need to update legacy codebases using this component to ensure they’re compatible with current and future Sklearn versions. One way to do this is via effective Code Migration Strategies.
Code Migration Strategy
You need to replace the failed imports from
xxxxxxxxxx
sklearn.externals.six
with another module that provides the same functionalities. Here are some methods:
1. Using six module:
The most direct method for replacing
xxxxxxxxxx
sklearn.externals.six
is with
xxxxxxxxxx
six
, available on PyPI.
xxxxxxxxxx
# Replace this
from sklearn.externals import six
# With this
import six
xxxxxxxxxx
Six
is a Python 2 and 3 compatibility library developed by Benjamin Peterson. It helps makes code compatible with both Python 2.x and 3.x. If your codebase uses any of the methods, functions or class definitions provided by
xxxxxxxxxx
six
, this switch will work unproblematically.
2. Using joblib:
If your code extensively uses functionality like creating pickles of Sci-kit learn models (which six supported), then switching to
xxxxxxxxxx
joblib
would be a good strategy. Functions like
xxxxxxxxxx
dump
and
xxxxxxxxxx
load
can handle large numpy arrays efficiently.
xxxxxxxxxx
# Replace this:
from sklearn.externals import joblib
# With this:
import joblib
Update Your Development Environment
To facilitate these changes, you also need to ensure that you have the necessary modules installed in your environment. If not already installed, you can add them to your project using pip:
xxxxxxxxxx
pip install six
pip install joblib
In conclusion, the key to an effective code migration strategy is understanding your existing dependencies on deprecated libraries, identifying alternative libraries that can serve the same functions, and rigorously testing your code post-migration. By implementing these robust solutions, you can seamlessly solve the
xxxxxxxxxx
No Module named sklearn.externals.six
issue.
Note: The removal of the externals.six module indicates a bigger shift towards Python 3 and withdrawing support for Python 2. Thus, consider switching to Python 3 if your project still relies on Python 2.
p>
Your error message ‘ModuleNotFoundError: No module named ‘sklearn.externals.six” could often appear due to the unavailability of the required package in your workspace.
If you are working with a Python environment, it’s crucial to verify that your modules are installed correctly. You can inspect this by using the command
xxxxxxxxxx
pip show sklearn
. If you don’t see any results, simply install Scikit-learn by typing
xxxxxxxxxx
pip install -U scikit-learn
.
Remember, the Scikit-learn version distributed on PyPI is bundled with required modules, including six, but sometimes there may be issues related to the path of these libraries that cause such errors. Furthermore, the removal of the six module in recent versions of Scikit-learn (0.23 and onwards) may also cause this error if your code refers to this now deleted module.
To circumvent this, consider directly utilizing ‘six’ rather than ‘sklearn.externals.six’. By installing or updating the ‘six’ module via
xxxxxxxxxx
pip install -U six
, you can keep your application robust against changes in modules provided by Scikit-learn.
In situations where your code is dependent on an old version of Scikit-learn, it’s advisable to create a virtual environment and install the required version within it. Python’s built-in venv module or external tools like conda can be used to manage different environments with their own dependencies.
In conclusion, ModuleNotFoundError issues mainly involve managing your Python dependencies properly, and if things break after a version update, scrutinizing those changes for potential breaking updates. Staying informed about those updates would turn your programming journey into a less bumpy ride. Remember, StackOverflow and official documentation sites are great resources whenever you encounter such issues.