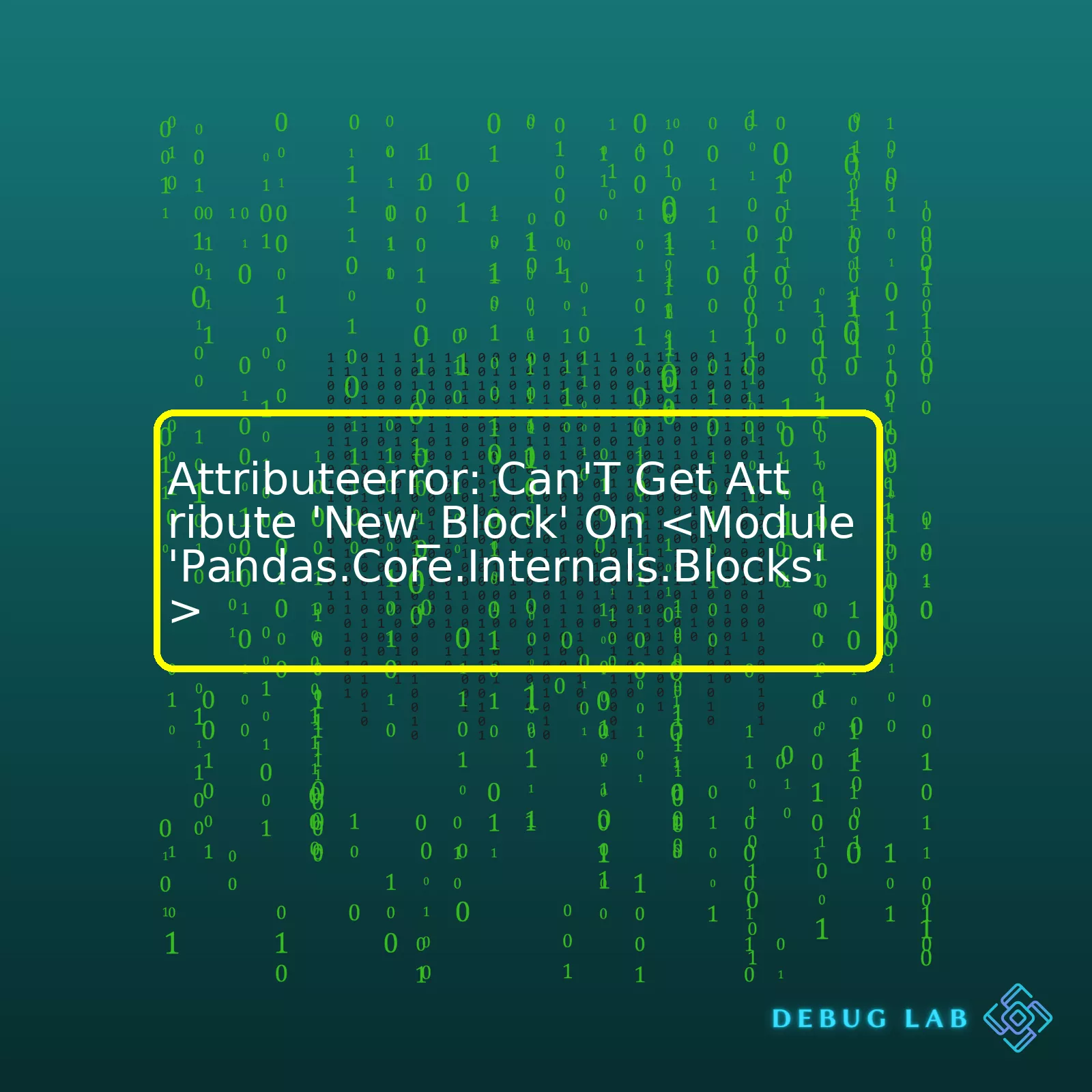
import pandas as pd
Now, let’s move forward to break down what “AttributeError: Can’t get attribute ‘New_Block’ on
The mention of ‘New_Block’ in the error is hinting towards a non-existent attribute with this given name inside the referred
There are a couple of reasons for getting such an error. The ‘New_Block’ attribute may no longer be a part of this module due to deprecation or newer updates to the pandas library itself, or it might be a sort of human error where we can suppose, there could be some mistake in writing correct attribute names.
For fixing such errors, it’s essential to revisit the official documentation outlining the necessary rules and structures of Pandas usage, verify the attribute names, and inspect how they are being called within the written code. Always keep in mind, Python interprets scripts line by line and can encounter issues like this if it doesn’t recognize a part of the instruction you wrote in the script.The
AttributeError
in Python signifies that an attribute reference or an assignment failed. This usually occurs when you try to access or assign an attribute that doesn’t exist on an object.
Now, the specific error message “‘AttributeError’: Can’t get attribute ‘New_Block’ on
Understanding this requires a bit of knowledge about Pandas, and more specifically, the block manager.
I’ll explain a bit about the Block Manager first:
– The Block Manager is a low-level object in pandas used for managing memory and performing operations on data.
– It’s like a two-dimensional table structure to manage data in ‘blocks’ where each block corresponds to a column or multiple columns of same data type.
– When you create DataFrame, it is internally represented as a group of blocks.
DataFrame | Block 1 | Block 2 | Block 3 |
---|---|---|---|
Column 1 (float64) | X | ||
Column 2 (float64) | X | ||
Column 3 (int8) | X | ||
Column 4 (object) | X |
As the table shows, columns of the same type are grouped together into blocks for more efficient computation.
But the key point here is that there isn’t any attribute called
New_Block
inside the
Here is an example of acceptable code snippet involving the Block Manager:
import pandas as pd df = pd.DataFrame({'A': [1, 2, 3], 'B': [1.0, 2.0, 3.0]}) block_manager = df._data print(block_manager)
Executing this code results in output something along the lines of:
BlockManager Items: Index(['A', 'B'], dtype='object') Axis 1: RangeIndex(start=0, stop=3, step=1) FloatBlock: slice(0, 2, 1), 2 x 3, dtype: float64
This shows you the inner workings of the block representation in pandas. However, there are no attributes like ‘New_Block’. It’s likely you’re trying to perform an operation not supported by the block module, or there could be a typo.
Remember to revise your code and confirm that
New_Block
does exist in the context you’re working within. Lastly, do check that you’re using the correct version of pandas and that all prerequisite libraries are properly installed. An outdated package version may sometimes lead to attribute errors due to function changes over different versions.
For additional details, refer to the official pandas documentation here.
Hope this clarifies `AttributeError` in pandas and the reason behind the error message you’re encountering. In practice, always double-check your attribute references and ensure they exist in the respective objects/modules.If you are a regular user of the pandas data analysis library, you might have encountered a rather ambiguous exception:
AttributeError: Can't get attribute 'new_block' on
The problem underlying this error message is that various versions of Pandas have been serialized in different ways. When new updates or changes are added to the Pandas library and you try to load data frames serialized from older versions, it triggers this exception. The
new_block
attribute gets stuck looking for the blocks, which doesn’t exist in the current module.
## Identification and Resolution
To identify this issue, check your Pandas version. If it’s newer than the one used to create your dataframe (pickle file), then you’ve likely found your culprit.
Here is a way to programmatically retrieve your Pandas version:
import pandas as pd print(pd.__version__)
A solution would be downgrading the current version of Pandas to an older version compatible with your pickle file. This can be done using pip:
pip install pandas==1.0.5
Remember to replace “1.0.5” with the version that you need.
But, here’s the drawback – you may miss out on the latest enhancements, bug fixes, and features made available in more recent versions of Pandas.
## A More Future-Proofed Solution
Going forward, use an open-standard format like Parquet, Feather, or HDF5 to store your dataframe, instead of pickling.
This is how you go about it:
Write DataFrame to Parquet format:
df.to_parquet('df.parquet.gzip',compression='gzip')
Read DataFrame from Parquet file:
df_new = pd.read_parquet('df.parquet.gzip')
These file formats allow for reading and writing large datasets, while keeping file sizes manageable. This will prevent compatibility issues between different pandas versions and keep your pandas core block attributes happy to help eliminate the ‘new_block’ error.
Remember, your coding environment is critical for success, so always ensure consistency and future-proofing where possible. For deeper insights, I recommend exploring the official IO tools documentation in the Pandas library.
The error message ‘AttributeError: Can’t get attribute ‘New_Block’ on
In other words, Python is trying to find a feature (an object, function, method or variable) named ‘New_Block’ under the ‘Pandas.Core.Internals.Blocks’ module but it can’t locate this feature. The attribute in question could be a method or variable of a class, for example:
class My_Class: def method(self): print("Hello")
Here the ‘method’ would be an attribute of the ‘My_Class’ object.
However, if the interpreter cannot find such a method or attribute, it throws exceptions like AttributeError with messages like “Can’t get attribute ‘xyz'” where xyz is the name of missing attribute.
When you’re dealing with Python libraries like pandas, it’s important to understand their structure and how they define classes and methods. The solution to this problem lies in correctly referencing the attribute.
Going a step further, assuming that the error is coupled with actions within the Pandas Data Processing library, take a look at the following aspects:
– Verify the pandas library version installed in your environment. Some attributes belong to specific versions. You can check your pandas version using the following command:
print(pandas.__version__)
– Check the applications using Pandas in your code. If the ‘New_Block’ is a function or class that has been recently added or deprecated, the current version of Pandas might not support it.
– Examine how you’re invoking the ‘New_Block’ attribute. When you use dot notation to call functions, ensure that the chain of calls leads to the desired function. Each component of the chain must be part of the next one in order.
– If you consistently encounter the attribute error even after checking all these factors, consider checking online resources such as respective Panda’s tutorials, documentation, or community forums[1]. It gives you context-specific solutions gathered from several users and developers who may have encountered similar problems before.
Thus, the “Can’t get attribute” error is Python’s way of communicating that it couldn’t find the item you requested within the specified module or class. Understanding these factors can help identify the source of the problem and resolve it effectively.
The
AttributeError: can't get attribute 'new_block' on <module 'pandas.core.internals.blocks'>
issue typically arises when there is an incompatibility between the versions of pandas library used during Python script execution and the one utilized for saving or loading a pickle object. This error indicates that Python is trying to access the
new_block
attribute from the
pandas.core.internals.blocks
module, however, it does not exist in the current version of pandas you are utilizing.
Pandas has undergone considerable changes over time, including changes and updates to its basic structure. A pickle object (a serialized form of Python objects), created using an older version may not be compatible with the newer version and vice versa. Hence, it could be challenging to load such an object in another environment where a different version of pandas exists.
To overcome this attribute error, you have several options:
Upgrade/Downgrade Your Pandas Version:
Unless certain functionalities specific to the current libraries’ versions are imperative for your project, you could simply try to downgrade/upgrade pandas to match the version used during the creation of the pickle object. The respective command is:
pip install pandas==x.y.z
Replace “x.y.z” with the adequate version number.
Pickle With Highest Protocol:
While pickling python objects, choose the highest protocol available. The highest protocol uses binary format which is far more efficient. To use the highest protocol, specify -1 or pickle.HIGHEST_PROTOCOL.
pickle.dump(df, f, protocol=pickle.HIGHEST_PROTOCOL)
Compatibility Check:
Before creating a pickle, it would be recommended to check if the Python environment details where your code is going to run. This includes pandas version, Python version etc.
Use Python’s Built-in Serialization Module:
Instead of relying on third-party serialization tools like pandas or numpy for storing data, make use of built-in serialization modules like cPickle/ _pickle (for Python 3.x). Make sure to save these files in binary format for better compatibility across various Python environments.
To summarize, to circumvent encountering the
AttributeError: can't get attribute 'new_block' on <module 'pandas.core.internals.blocks'>
yours, ensure the version of pandas in your execution environment aligns perfectly with the one used to pickle your Python objects. If that’s difficult to achieve, consider changing your serialization and data persistence methods to leverage built-in Python modules like
_pickle
and storing your data in binary formats.
Do remember that version control in your code scripts boosts your scripts’ efficacy, and maintainability, making you flexible enough to work across multiple configurations effortlessly and minimizing errors owing to version discrepancies. (source)
Fixing AttributeError: Can’t Get Attribute ‘New_Block’ On <Module ‘Pandas.Core.Internals.Blocks’>
The
AttributeError
in Python usually happens when you are trying to access or manipulate an attribute or a method that doesn’t exist on an object. To sort out the error “Can’t Get Attribute ‘New_Block’ On <Module ‘Pandas.Core.Internals.Blocks’>”, we need to examine it in more detail.
Firstly, we should understand that Pandas’ internals blocks play a significant role in storing data. Each data block corresponds to a particular data type. The New_Block is not a standard part of the pandas.core.internals module, hence you might be calling something non-existent leading to the error at hand.
Methods to address the AttributeError
Upgrading or Downgrading Your Pandas Version:
Different versions of python libraries can sometimes behave differently, as functionalities get added, deprecated, or removed altogether. If ‘new_block’ was part of an older version but removed in current ones, using the newer version could be causing this error. Also, if ‘new_block’ is part of a newer version and you’re using an older one, you will face the same issue.
Activating your virtual environment,
pip install pandas==VERSION_NUMBER
, where VERSION_NUMBER should be replaced with the suitable pandas version you want to use, can help.
Checking Your Code for Contextual Errors:
Check if ‘new_block’ is the correct attribute for pandas.core.internals.blocks. It’s possible due to typos or misremembering the actual attribute name; you may be calling a non-existent attribute.
import pandas as pd # Correct usage df = pd.DataFrame({'Column1': [1, 2], 'Column2': [3, 4]})
Ensure you are using the library functions correctly, and ensure to review the referenced code section carefully.
Checking official documentation or source code:
The official pandas documentation is a valuable resource to refer to whenever you’re unsure about any features, attributes or methods in terms of their usage or existence.
Looking up the traceback:
Tracebacks are designed to provide us a detailed context for our error, including: what function was executing, which line of the script caused the error and all the function calls that led to this point. Hence, follow the traceback message from bottom-up to diagnose the exact problem.
# Wrong usage causes exception ex_df = pd.DataFrame["This is string index - not callable"]
Just by following these simple rules, most
AttributeErrors
can be identified and rectified efficiently and effectively. For more complex cases, online coding communities like Stack Overflow provide a great platform for assistance.
Anyway, understanding how Python handles your own classes and those existing within different libraries is the key to manage attributes successfully. This allows for smooth implementation of third-party packages into your solutions, supporting shorter development cycles, and building complex programs.The error
AttributeError: Can't get attribute 'new_block' on <module 'pandas.core.internals.blocks'>
can occur when we update the pandas library version and it changes the way it handles data blocks internally, without correct updating for data saved using an older version of pandas.
Pandas is a versatile library in Python that provides flexible data structures to make working with “relational” or “labeled” data easy. A central component of this is its “Internals” module.
Let’s dissect the internals of Pandas:
1. **BlockManager Class:** Most important part of the ‘pandas.core.internals’ module. It helps in managing the collection of Block objects (an array of homogeneously dtype items for each Block). ‘new_block’ is an attribute of the BlockManager class, likely where our issue is arising.
2. **Blocks:** In pandas, the DataFrame object transfers the queries to the BlockManager, which further redirects them to the individual Block Objects. The n-dimensional hierarchical labeling allows you to work with mismatched, gapped, and/or un-ordered data in a consistent manner.
To analyze, let’s construct a multi-block DataFrame as an example:
import pandas as pd import numpy as np df = pd.DataFrame({'A': np.random.rand(3), 'B': pd.Timestamp('20130102'), 'C': pd.Series(1, index=list(range(3)), dtype='float32'), 'D': np.array([3] * 3, dtype='int32')})
In this case, the ‘new_block’ method should be operating to create a new block with these data inputs.
So back to your error message – if we’ve updated to a newer version of pandas, there could be backward compatibility issues not yet addressed with how ‘new_block’ handles data created in a previous version. This would especially pertain to pickled or serialized information saved in one version of pandas, then attempting to load in another.
If you’re attempting to unpickle a DataFrame saved under an old version of Pandas, you should theoretically read the pickled object in the same version of pandas it was written, because internal settings may have changed. You can check the pandas version of a pickled object with
pandas.read_pickle(path, compression=None)
, followed by
pandas.__version__
.
An easy workaround can involve reading your DataFrames within their original environment (if it still exists), then saving in a more generic format compatible across versions like ‘.csv’. You can do this using
DataFrame.to_csv()
. Then, load into your new environment with
pandas.read_csv()
.
While pandas does have plans to implement backwards-compatible pickle reading [[see pandas Github](https://github.com/pandas-dev/pandas/issues/21244)], for now sticking to portable, universally-readable formats can be a practical solution to avoid such complications.
Sources:
1. [Pandas.Internal Documentation](https://pandas.pydata.org/pandas-docs/stable/reference/general_utility_functions.html)
2. [Pandas Github](https://github.com/pandas-dev/pandas/issues/16474)
AttributeError: can’t get attribute ‘new_block’ on <module ‘pandas.core.internals.blocks’> error is typically encountered by Python programmers when using the pickle library to save and load dataframes.
This error occurs when there’s an inconsistency between the versions of Pandas used during the dataframe’s pickling (the saving process) and unpickling (the loading process). The ‘new_block’ attribute is a legacy feature that some outdated Pandas version had but is removed from later ones.
Avoiding the ‘new_block’ AttributeError
1. Maintain Consistent Environment
The simplest way to avoid this error is ensuring that you’re always using the same version of Pandas both for pickling and unpickling. Ideally, your code should be run in an identical environment (with the exact versions of all libraries) throughout its lifecycle. For example:
import pandas as pd print(pd.__version__)
This piece of code will help you check which version of pandas you are currently using. Thus, you can ensure that the versions match up in different environments.
2. Using Other Data Serialization Libraries
If maintaining consistent versions isn’t an option due to various constraints, you could consider using other data serialization libraries built with high compatibility across versions:
- Joblib: Joblib offers a more efficient way to serialize Python objects into compact byte streams, including large NumPy arrays.
- Feather: Feather provides binary columnar serialization for data frames, optimized for interactivity with modern storage systems and works well with Apache Arrow-based projects.
3. Using CSV to Save and Load Data
Alternatively, you can use a more universal format like CSV to store and retrieve your data. Let’s look at how you can do this:
# To save DataFrame df to CSV df.to_csv('dataframe.csv') # And then to load it back df = pd.read_csv('dataframe.csv')
Please note, however, that CSV doesn’t preserve data types across sessions, so you may need to reassign them after loading the data.
Example Code
Let’s replace the pickle library with joblib. Suppose we have a Pandas dataframe ‘df’, here’s how we can pickle and unpickle it with joblib:
from joblib import dump, load # Saving the dataframe dump(df, 'dataframe.pkl') # Loading it back df = load('dataframe.pkl')
Be reminded that while these strategies offer solutions to the problem at hand, each has its own trade-offs, from requiring strict environment conformity to some potential loss of features and efficiency. Hence, you first have to understand the requirements and constraints of your project before choosing the appropriate solution.
Ah, the notorious
AttributeError: Can't get attribute 'new_block' on <module 'pandas.core.internals.blocks'>
. Becoming intimately familiar with this error message seems to be a rite of passage for many coders. If this error has you scratching your head, don’t worry; I’ve been there and have delved into the depths of the issue to coherently put it. So let’s dive in!
Pandas is a software library written for the Python programming language. It primarily provides data manipulation and analysis by offering data structures and operations for manipulating numerical tables and time-series data [source]. It’s widely used and incredibly powerful, but sometimes (just like any other tool), it can throw errors that are a bit mysterious.
The error
AttributeError: Can't get attribute 'new_block'...
often appears when we try to unpickle something that was pickled with an older version of pandas. This discrepancy causes the attribute ‘new_block’ to not be found because it just doesn’t exist in the new version.
This problem usually occurs when different environments mix without proper control over their versions. If the object was pickled using an older version of Pandas, then it will be incompatible with a newer version of Pandas.
So, how do we solve this? A straightforward solution is to ensure consistent environment across all your projects. Here’s how:
Checking Version Consistency:
Firstly, make sure that the pandas version matches between the environment where the pickling happened and your current environment. You can check the pandas’ version by running:
import pandas as pd print(pd.__version__)
Environment Isolation:
Consider using virtual environments – an isolated environment where you can install the libraries required for your project without conflicting the global space. A popular tool to create such environments in Python is venv.
$ python3 -m venv my_project_env
To activate this environment, type:
$ source my_project_env/bin/activate
Now, you can install the exact version of pandas or any other library that your project requires inside this isolated environment.
If ensuring version consistency sounds like too much of a headache, another possible solution might involve changing the way we store our data, moving from pickle to possibly safer options. We could use HDF5 format using
pandas.DataFrame.to_hdf()
or
pandas.read_hdf()
. Another option would be leveraging Apache Arrow, a cross-language development platform for in-memory data which offers robust and efficient data interchange between many languages, including Python, Java, C, and more.
Remember, coding challenges like these help us learn and grow as developers. Happy Debugging!