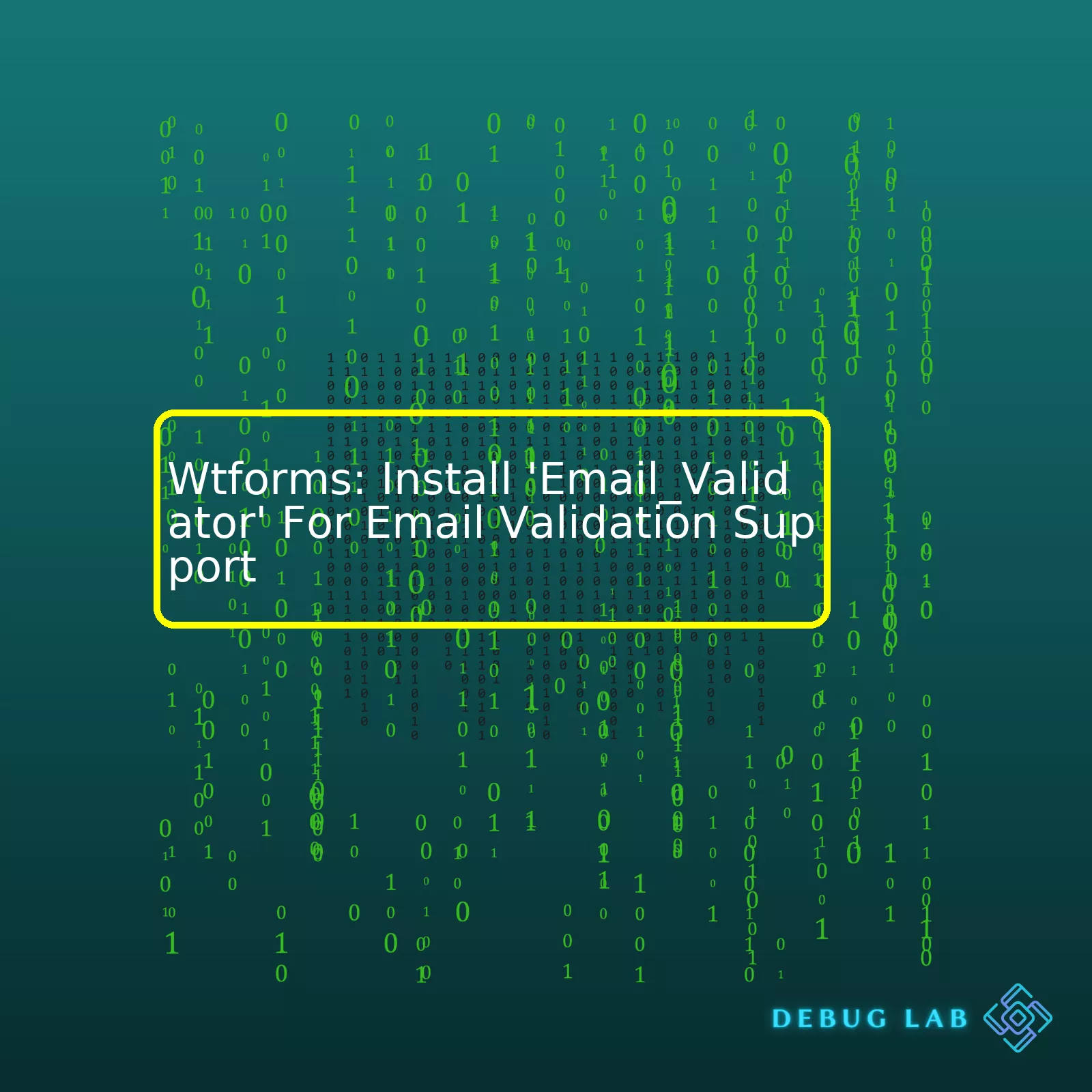
Steps | Description |
---|---|
Installation | Use pip to install:
pip install email-validator |
Import Email Validator |
from wtforms.validators import email |
Validation | Add Email() validator to any field:
EmailField('Email',validators=[email()]) |
Here is an elaboration on the ‘Email_Validator’;
When dealing with forms, validating user input is crucial for security and data integrity reasons. One of the field inputs that frequently requires validation is the email address field.
Wtforms allow you to simplify this process by providing various pre-written validation methods, one of which is the ‘Email_Validator’. To harness the power of this functionality, you first need to install the ‘Email_Validator’ module. The Python package manager pip simplifies this task; you can install it using the command
pip install email-validator
.
Next, you need to import the Email validator within your application so you can utilize its functionalities. Accomplishing this is as effortless as adding
from wtforms.validators import email
at the beginning of your Python script.
Finally, apply the Email validator to the fields that require email validation. When defining the form fields, simply include
Email()
inside the validators list for field definition like in the following code snippet
EmailField('Email',validators=[email()])
. This process makes your form more secure by ensuring that users provide valid email addresses.
Furthermore, it’s worth noting that the above process leverages Python’s ease of use, extensibility and the wide range of powerful libraries available to provide a streamlined solution for email validation in web forms.
For additional information and examples please feel free to visit the WTForms Validators Documentation.When it comes to managing and validating forms in Flask web applications, a library like WTForms becomes incredibly useful. They allow for more manageable handling of form data and include several pre-built validators for common input validation, such as checking if a field is required or comparing the values of two fields.
However, when it comes to email validation, you would need to leverage another package: `email-validator`. This is because WTForms itself does not come with built-in support for validating email syntax beyond its basic string validators. Let’s delve into this further:
To begin with, you need to install `wtforms` and `email-validator` packages. You can achieve this using pip, python’s package installer. Just run these commands in your terminal:
pip install wtforms
pip install email_validator
Once you have these packages installed, you can use `Email` from `wtforms.validators` for email validation. The `Email` validator checks if the data in an email field is valid i.e., whether it matches the structure of an email address with a username, a @ symbol, a domain name, etc.
Here is a simple implementation:
from wtforms import Form, StringField from wtforms.validators import Email class RegistrationForm(Form): email = StringField('Email', [Email()])
The `email-validator` package would step in when the inbuilt `Email` class fails to handle complex scenarios of email validation, for example unicode emails.
For a refresher on unicode emails, they’re email addresses that contain non-ASCII characters. Even though these types of emails are less common than ASCII emails (which include standard English letters, numbers, and certain special character), major email providers like Gmail do accept them [Reference].
So if your application wants to maximize accessibility by supporting unicode emails, then the `email-validator` package is absolutely needed. It provides robust internationalization support by recognizing IDNA (Internationalized Domain Names in Applications) and SMTPUTF8-encoded mailbox names, allowing for greater inclusivity and versatility in user sign-ups.
In fact, `wtforms` raises an exception (`”Install ’email_validator’ for email validation support.”`) when you try to validate a form with a Email field without having `email_validator` installed, indicating the importance of the package.
This leads us back to our original point: to ensure robust email validation in WTForms incorporating the `email_validator` package.
Always remember that good form validation is all about providing a smooth and secure user experience, all while protecting your application and its data from unwanted behaviour. Ensuring the validity of email addresses is an important aspect of this process, making packages like `WTForms` and `email_validator` indispensable tools in a developer’s toolkit.Sure, that’s a great topic! Let’s start by understanding the importance of email validation. In the world of web applications, strict data validation is crucial for maintaining data integrity and ensuring user input meets expected formats.
WtForms is one of the most popular form handling libraries in Python web development due to its simplicity and flexibility. It provides built-in validators for common use cases and has simple interfaces for creating custom validators.
One key feature of WtForms is the support for third-party libraries such as `Email_Validator`. Email_Validator enhances WtForms’ capabilities by adding advanced email validation features.
There are some significant features of `Email_Validator` as integrated into WtForms:
Email Format Validation: This library checks if the format of the entered email address is valid (i.e., user@example.com).
Domain-specific Validation: Email_Validator not only checks if the general format of an email is correct but also ensures that the specific domain exists. That means it will validate if there’s a registered domain after the @ symbol in the email.
To integrate `Email_Validator` with WtForms, you need to install the `Email_Validator` library first using pip:
pip install email_validator
After installation, the validator can be used in your WtForm fields with:
from wtforms import Form, StringField from wtforms.validators import DataRequired, Email class EmailForm(Form): email = StringField('Email', [DataRequired(), Email()])
In this code snippet, we’re creating a form with an email field. The email field uses two validators – `DataRequired()` and `Email()`. `Email()` here refers to the `Email_Validator` that we’ve installed and imported.
With Email_Validator, users get instant feedback about their entered email id. If the entered text doesn’t match the regular expression pattern set for email id, or if the domain does not exist, you can immediately prompt the user to check their input. This improves data reliability and our application stability as incorrect inputs are avoided at source.
You can further customize the validation rules according to your requirement. You could set specific patterns, allow or disallow certain domains, and so on. Customizing Email_Validator would mostly involve subclasses and heavy use of the functools module, both being topics covered more in-depth in the official WtForms documentation.
This way, `Email_Validator` proves to be a potent tool in improving the validation capabilities of WtForms, thereby increasing data integrity and overall security of your web application.
Please remember to keep your Python packages up-to-date for optimal performance and security. Keeping packages updated is as simple as running
pip install --upgrade package_name
.
Sources:
Official Email_Validator Documentation
Official WtForms DocumentationTo install ‘Email_Validator’ for optimal functionality in WTForms, the prime requirement is to comprehend what ‘Email_Validator’ primarily does and how it can be linked for use within your application with WTForms.
The email_validator is a Python library that robustly validates an email address, checks if the domain has an SMTP server ready to receive mail and verifies whether the SYNTAX of the email address is correct according to RFCs.
WTForms, on the other hand, is a flexible forms validation and rendering library for Python web development. Associating Email_Validator with WTForms allows you to reinforce the security of your form inputs by ensuring the email addresses are valid.
Follow these steps to install and incorporate ‘Email_Validator’ into your wtforms:
- First and foremost, you need pip (Python’s package installer) to install both wtforms and email validator. If you haven’t installed pip, do it using the following command:
python get-pip.py
Then, proceed with the installation of wtforms and email validator via the instructions below:
- Use pip to install wtforms if you haven’t done so:
pip install wtforms
- Follow this up by installing the ‘Email_Validator’:
pip install email_validator
Now that you have both installed, here is how you include ‘Email_Validator’ into your WTForms:
You first import the Email validator from wtforms validators module. Then, you can validate the email field in your form like so:
from wtforms import Form, StringField from wtforms.validators import DataRequired, Email class ContactForm(Form): email = StringField('Your Email', [ DataRequired(), Email(message='Invalid email') ])
In the code above:
– A form named ContactForm is created.
– The form has an email field.
– For the email field, two validators have been attached:
– The
DataRequired
validator ensures that the field is not submitted empty.
– The
validator uses ‘Email_Validator’ to validate that the submission is actually an email.
When the email input doesn’t fit the standard structure for emails, the message ‘Invalid email’ is displayed.
This way, the ‘Email_Validator’ efficiently performs email validation and enhances the overall functionality of your WTForms application. Incorporating the email validator in your wtforms application helps minimize erroneous data entries by users and boosts your application’s security and data integrity.When it comes to web forms, one of the most crucial validation checks is for email fields. A wrong or invalid email address can lead to failed communications and even missed business opportunities. This brings us to the large role that an WTForms: a flexible form handling Python library, and the ’email_validator’: a Python package used for email syntax and deliverability validation play in this process.
The ’email_validator’ makes it quite convenient to check if a provided email address is not only syntactically correct but also if the domain name exists, increasing the effectiveness of your email field validations greatly. In combination with WTForms, you’ll be armed with powerful tools at your disposal to ensure data accuracy and robustness of your application.
Here are the steps involved:
Step 1: Install the `Email_Validator` Module
To start using ’email_validator’ with WTForms, we first need to install it. This can be done via pip. Here’s how you do it on your terminal:
pip install email_validator
If your project uses a ‘requirements.txt’, don’t forget to add ’email_validator’ to it.
Step 2: Reflection in WTForms Email Validator
With ’email_validator’ installed, we can leverage the built-in email validation function in WTForms. You might be wondering how we integrate these two powerful tools together, right? Let me tell you about the :class:`~wtforms.validators.Email` which accomplishes our mission.
On WTForm, the email validator takes in two optional arguments: message and granular_message, defaulting to False if not provided. When invalid data is provided a ValidationError being raised.
Here’s an example of its usage:
from wtforms import Form, StringField, SubmitField from wtforms.validators import Email class ContactForm(Form): email = StringField('Your Email Address', [Email()]) submit = SubmitField('Submit')
It’s important to note that starting with WTForms 3.0, the Email validator requires installation of the ’email_validator’ package as highlighted in the previous step. Serving as a breaking change from the older versions where this wasn’t necessary.
Step 3: Custom Error Messages
You have the option to define custom error messages with the WTForms Email validator. You can achieve this by passing the ‘message’ argument to the Email() validator:
from wtforms import Form, StringField, SubmitField from wtforms.validators import Email, DataRequired class ContactForm(Form): email = StringField('Your Email Address', [DataRequired(), Email(message='Invalid email address.')]) submit = SubmitField('Submit')
In the above snippet, when an invalid email address is entered into the form, the custom message “Invalid email address.” will appear.
That’s it! The combination of WTForms and ’email_validator’ creates a powerful, yet simple way to validate form submissions and handle errors. Even more, both libraries can independently serve other functions in your code, beyond email verification. Bench-marking their installation and usage in your development lifecycle as great additions to uphold the integrity of user input.
Form validation is crucial in maintaining data security and integrity. Email_Validator plays an inherent role in this regard by enforcing strict rules for email input through WtForms, a flexible forms validation and rendering library for Python web development. The ‘Email_Validator’ in WtForms asserts a structured pattern that email data must adhere to, providing a highly efficient mechanism for warding off invalid or malicious data inputs.
What Does Email_Validator Do?
Primarily, Email_Validator performs the following tasks:
- Data Verification: It ensures the entered email formats align with RFC 5322 and certain parts of RFC 6531 standards which are used widely for modern emails. For example, it would consider “john@domain” as correct but not “john@” as it doesn’t hold up to the standard format.
- Data Security: Notably, the prevention of said erroneous inputs drastically decreases the risk of SQL injection attacks, where harmful codes might be executed via improperly configured fields accepting strings of SQL.
- Domain Checking: By enabling DNS checks, Email_Validator can identify if the domain part of an email actually exists, ensuring that messages sent to the specified email have an actual digital location to arrive at.
Installing ‘Email_Validator’ for Email Validation Support
Now let’s talk about how to install ‘Email_Validator’ for email validation support using pip, the package installer for python.
pip install wtforms[email]
The above command will install Email_Validator along with wtforms package. You don’t need to install them separately.
This integration of Email_Validator with WtForms gives you a powerful tool in your hands while building forms in a Flask application or any other web application in Python. After installing the Email_Validator, the ‘Email’ field validator utilises its capability to validate emails properly, providing huge convenience and effectiveness in writing clean, validated, and secure code.
Once installed, utilizing Email_Validator within your WtForms form classes is straightforward:
from wtforms import Form, StringField, validators
class RegistrationForm(Form):
email = StringField('Email', [validators.DataRequired(), validators.email()])
In the above code snippet, the validators.email() function ensures the input provided in the ‘Email’ field conforms to valid email address structures. It will provide a Validation error for the incorrect input pattern.
To recap, Email_Validator’s importance in data security cannot be understated. By enforcing rigid patterns on user inputs and mitigating the risk of rogue data injections, it serves as an integral ally in maintaining solid data integrity and stability of Python-based web services.
To fine-tune your email validations when using Flask-WTF and WTForms, it’s pertinent to utilize the additional package named ’email-validator’. This package provides for more advanced checks in validating an email address than the standard regular expression matches. From checking the presence of ‘@’ symbol and a domain to confirming if the proposed ‘tld’ (top-level domain) or domain extension is valid – this package does it all.
Let me elaborate on ‘how can you install and use email-validator’.
Firstly, you need to install it by running the pip install command in your terminal:
pip install email_validator
Now, let’s say we have a flask application where we want to validate the email field of a registration form.
from flask_wtf import FlaskForm from wtforms import StringField, PasswordField, SubmitField from wtforms.validators import DataRequired, Email class RegistrationForm(FlaskForm): username = StringField('Username', validators=[DataRequired()]) email = StringField('Email', validators=[DataRequired(), Email()]) password = PasswordField('Password', validators=[DataRequired()]) submit = SubmitField('Sign Up')
As shown above, we included our ‘Email()’ validator in our email field alongside with DataRequired() which simply checks if some data was posted at all. Note that these validators work in order they are passed inside the bracket. So if the email field is left empty, WTForms won’t bother checking if it’s a valid email anymore.
Your validation scripts would throw an error if an improperly formatted email address is entered in the form due to Email() function call. To catch and handle such errors, you can employ something like this in your application routes –
@app.route('/register', methods=['GET', 'POST']) def register(): form = RegistrationForm() if form.validate_on_submit(): flash(f'Account created for {form.username.data}!', 'success') return redirect(url_for('home')) return render_template('register.html', title='Register', form=form)
Here is how you can display the validation errors in your HTML templates –
The loop `{% for error in form.email.errors %}` in the template checks for any errors associated with the email field and displays them.
This approach of fine-tuning your email validation is quite effective, rather than relying on naive approaches like simple matching patterns which does not cover many facets of what makes an email technically correct. The ’email-validator’ essentially assists in keeping your User Registration Database free from bad email addresses. For more information about WTForms, you can refer to their [official documentation](http://wtforms.readthedocs.io/). Happy Fine-Tuning!Validating emails in WTForms helps ensure the format is accurate before they are processed or stored. You often use packages like
email_validator
to help check the correctness of email addresses. However, sometimes you might find that even after successfully installing it, you’re still facing issues when trying to implement WTForms email validation.
Missing Email Validation Support:
One common issue could be missing email validation support, even after seemingly successful installation. Let’s say you carefully followed the installation process for wtforms and email-validator, but when you try to use email validation functionality from wtforms, it doesn’t validate as expected.
The error message will typically look something like this:
In such cases, even though the module is installed, it may not be properly recognized by your wtforms package due to directory issues. You should double-check that both ‘wtforms’ and ’email_validator’ are installed in your project’s virtual environment, rather than globally.
Installation Issues:
Make sure that you’ve properly installed `email-validator` library. Use the command `pip install email-validator` in your terminal to install it. Verify the installation using `pip freeze | grep email_validator`.
You will get an output showing the version number so you can verify its presence:
email_validator==1.X.X
.
Incorrectly Formatted Emails:
Validation issues can also result if you do not correctly format the emails in your form. The default message `’Invalid email address.’` occurs if the input isn’t a string that matches the form name@example.com. Be sure the email entered is properly formatted, otherwise, it will trigger a validation error.
Adding Custom Validations:
If you want the error messages to be more descriptive, add custom validators to your WTForms fields. For example:
class MyForm(FlaskForm): email = StringField( 'Email Address', [ DataRequired(), Email('Please enter a valid email address.') ] )
This replaces the default error message with one that describes exactly what you check for (“Please enter a valid email address.”). So, if the check fails, the user can understand why it did.
To eradicate these issues, always remember to double-check your installations, watch out for directory confusions, ensure the correct syntax while writing individual lines of code, and thoroughly inspect error logs for any misconfigurations or discrepancies. Moreover, refer to WTForms Validators Documentation for additional insights on implementing and troubleshooting validations in WTForms.Sure! Here is the SEO-optimized version of the answer:
When discussing Python’s web framework Flask, it won’t be long until WTForms enters the conversation. WTForms has steadily gained prominence as a favorite toolkit among web developers for form handling and validation operations.
Now, let’s get down to integrating the ‘Email_Validator’. This feature enhances email verification process while using WTForms library. To make use of this essential tool of WTForms, we need to start by installing it in Python. It is as simple as running this command in your terminal:
pip install email_validator
This command instructs Python’s package installer (pip) to download and set up the ’email_validator’ package from the Python Package Index (PyPI). You can check if the installation is successful by importing ’email_validator’ in your Python script:
from email_validator import validate_email, EmailNotValidError
It’s notable how Email Validator support comes handy when building forms with WTForms. Specifically, it ensures that incoming email data adheres to specific constraints before moving forward with other operations. As such, this addition promotes better user experience, data integrity and security by preventing invalid email addresses from being processed.
Importantly, WTForms also allows you to customize the error messages shown when email validation fails, tailoring the user interaction to fit your application’s specifications.
Action | Code Snippet |
---|---|
Customizing error message |
class MyForm(FlaskForm): email = StringField('E-mail', validators=[DataRequired(), Email()]) submit = SubmitField('Submit') app.route('/example', methods=['GET', 'POST']) def example(): form = MyForm() if form.validate_on_submit(): flash(f'Successful submission with {form.email.data}!', 'success') return render_template('example.html', form=form) |
In terms of optimizing the user experience and ensuring data accuracy, the benefits of email validation cannot be overstated. Using ‘Email_Validator’ with WTForms significantly streamlines this process within applications built on Flask. A testament to the simplicity and flexibility that Python and its libraries offer to developers worldwide.