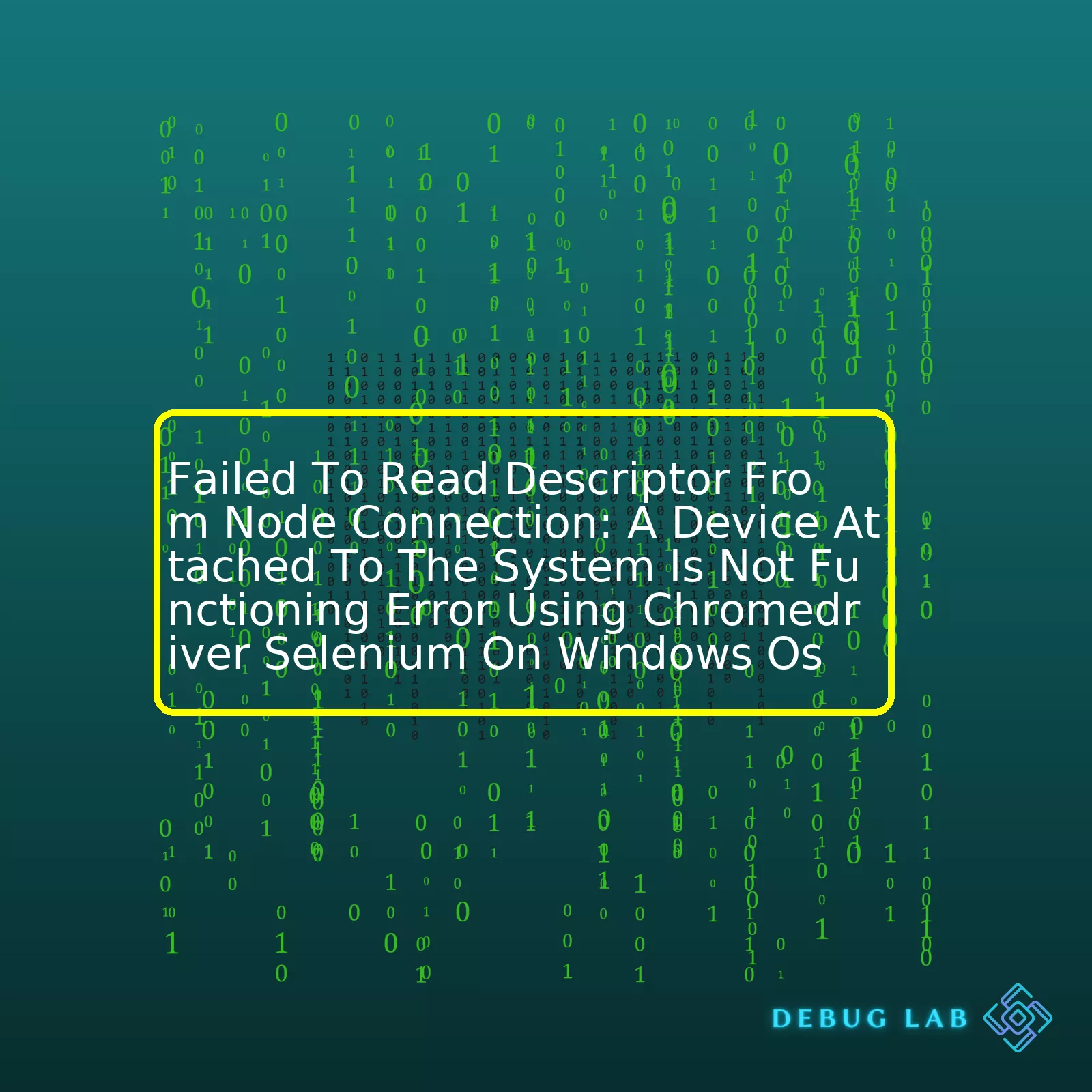
HTML table for your reference:
Error | Cause | Solution |
---|---|---|
Failed To Read Descriptor From Node Connection | The common cause for this issue is typically a conflict between different versions of Chrome browser and Chromedriver. | One should either upgrade Chromedriver to match with the installed Chrome version or downgrade Chrome to match with the Chromedriver. Alternatively, using WebDriverManager can automatically manage the driver versions. |
A Device Attached To The System Is Not Functioning | This issue usually comes up when there are problems with hardware devices attached to your system or their drivers not properly functioning. | Ensure all system drivers are updated. Check if any external device connected to the system is causing the problem. Also, check if the Selenium tests are trying to interact with any unavailable system resources. |
“Failed To Read Descriptor From Node Connection: A Device Attached To The System Is Not Functioning Error Using Chromedriver Selenium On Windows Os” is an error typically experienced by developers employing Selenium automation testing with Chromium-based web browsers – notably Google Chrome. It arises chiefly due to compatibility issues between the Chrome Browser’s version and the Chromedriver being used. This discrepancy makes communication glitchy, thereby obfuscating the descriptor from the node connection and interrupting interactive sequences with inoperable outcomes.
On occasions, it might also be provoked by an inadequate response from system-attached devices, especially during their interaction phase in automated tests. If such devices or perhaps their drivers malfunction or fail to respond seamlessly, they may cascade into the Selenium script, thereby causing the failure in test execution.
To troubleshoot these issues, it’s key to establish conformity between your Chrome Browser and the Chromedriver versions. Additionally, ensuring system drivers are up-to-date can potentially mitigate problems related to attached devices. WebDriverManager too can serve as a resolution strategy as it consummately manages these mismatches by matching and synchronizing browser-driver versions, consequently fostering seamless testing experience on the go!There’s a fair possibility that you’ve found yourself knotted amidst cryptic console errors while using Chromedriver Selenium on Windows OS. One pesky error in particular is “Failed to read descriptor from node connection: A device attached to the system is not functioning.” Although it might appear inscrutable, it invariably relates to issues with the Chrome Browser or the Chromedriver instance. Moreover, this problem can pop up due to intermittent issues in communication between the node and Selenium WebDriver.
Let’s delve into the debugging journey and walk you through various troubleshooting steps:
1. Update Your Chromedriver
A mismatch between Google Chrome browser version and Chromedriver version often results in anomalies. Always ensure that your Chromedriver corresponds with the installed Google Chrome version.
webdriver-manager update
2. Reinstall Google Chrome Browser
Sometimes, reinstalling Google Chrome can help since an unclean or incomplete previous installation could be causing the issue. Uninstall the following components:
- Google Update Helper
- Google Software Updater
- Chrome Remote Desktop Viewer
- Google Chrome browser
Once done, download Google Chrome afresh from its official website and reinstall.
3. Try Running The Script Administration Mode
Selenium scripts may require administrative rights during execution depending on file access permissions. Run your Integrated Development Environment as ‘administrator’ and execute your WebDriver script again.
4. Adjust User Account Control Settings On Windows
Windows UAC (User Account Control) prevents users from making unauthorized system modifications. However, it can interfere with Selenium’s script execution at times, affecting the interaction between Selenium WebDriver and ChromeDriver.
# Access UAC settings via command line: C:\Windows\System32\UserAccountControlSettings.exe # Set it to 'Never notify'
5. Tweak Experimental Capabilities Options
You can initialize chrome options keeping in mind specific capabilities. Check if altering these helps:
from selenium import webdriver options = webdriver.ChromeOptions() options.add_experimental_option('excludeSwitches', ['enable-logging']) my_driver = webdriver.Chrome(options=options)
6. If nothing works, use Headless Browser
If the above resolutions seem uneffective for some reason, you may use Google Chrome in headless mode. This configuration enables everything that the browser comprises of, sans the Graphical User Interface. This mitigates the chances of encountering GUI related issues like our candidate error here.
from selenium import webdriver from selenium.webdriver.chrome.options import Options chrome_options = Options() chrome_options.add_argument("--headless") driver = webdriver.Chrome(chrome_options=chrome_options) # Add here your selenium code
Please note: Influencing tools involve myriad uncontrollable factors like system state, network conditions, browser behaviour, or even other applications running alongside. Thus, debugging requires a good understanding of these dependencies besides knowing just programming.
Given the many reasons behind our error on the table, each solution neither ensures a universal fix nor promises elimination of the root cause. Nonetheless, adopting a systematic, step-wise debugging approach goes a long way in ensuring efficient troubleshooting.
I hope these tips will assist you on your path to becoming a more proficient automated tester.
Facing a ‘Failed To Read Descriptor From Node Connection: A Device Attached To The System Is Not Functioning’ error while using Chromedriver Selenium on Windows OS could be quite frustrating. The good news is that there are several ways to eliminate this error and have your system running smoothly once again.
One possible reason for this issue might arise due to an outdated or incompatible version of ChromeDriver you might be using. ChromeDriver needs to compatible with your installed Chrome browser version. To solve this, here’s what you’ll need to do:
- First, ascertain the version of your Chrome browser (Chrome Menu > Help > About Google Chrome).
- Then download the matching version of ChromeDriver from the ChromeDriver download page.
- Replace your existing ChromeDriver with the newly downloaded file.
[code]
from selenium import webdriver
driver_location = “C:\\path\\to\\chromedriver.exe” # Replace this with your path
driver = webdriver.Chrome(executable_path=driver_location)
[/code]
In case after aligning both versions, you still face the same issue, another possible reason could be your Antivirus software or Firewall blocking the communication between Selenium WebDriver and Chrome Browser.
To check if it’s indeed your security software causing the problem:
- Temporarily disable your antivirus or firewall.
- Run your Selenium Script to see if the issue persists.
If this resolves your problem, it means you will have to whitelist certain processes in your security software. Remember to re-enable your antivirus or firewall as soon as you finished testing.
Last, but not least, your system environment variables may also conflict with Selenium WebDriver. Updating PATH variable with the absolute location of your WebDriver binary may solve the issue:
setx path "%path%;C:\path\to\WebDriver\binary"
Please remember to replace ‘C:\path\to\WebDriver\binary’ with the actual path where your WebDriver binary is located.
By following these strategies, it is highly likely to eliminate the ‘Failed To Read Descriptor From Node Connection: A Device Attached To The System Is Not Functioning’ error while operating Chromedriver Selenium on a Windows OS. If you’re still experiencing issues afterward, it’s worth seeking professional troubleshooting advice – this can help diagnose any underlying problems which may be resulting in the failed connection.
Sure, when working with
Chromedriver Selenium
on Windows OS, one of the common issues many users encounter is: “Failed To Read Descriptor From Node Connection: A Device Attached To The System Is Not Functioning Error.” This is a device malfunction error that occurs on the user’s computer while using Chromedriver Selenium for automated testing.
Let’s break down this issue and understand the potential causes behind it, and also discuss some solutions:
Potential Causes:
– Incompatibility: One major cause can be incompatibility between the Selenium Chromedriver and the Chrome browser versions. The WebDriver communicates with the browser at the endpoint specified by W3C standards, but if there’s an inconsistency between versions, it may fail to communicate properly leading to errors.
– Corrupted Files: If the Chromedriver or any related file has been corrupted, this might lead to operational issues. Sometimes, downloading these files from unverified sources may lead to contamination by malicious software which may interfere with proper functioning.
– Operating System Issues: This error could also stem from Windows system-related problems, like incorrect configuration, outdated system, or defective hardware.
Possible Solutions:
Firstly, you need to ensure that your
Chromedriver version
matches with the
Chrome browser version
. This is a crucial step as mismatches could lead to such issues. For example, if you’re using Chrome v85, the Chromedriver also needs to be v85.
WebDriver driver = new ChromeDriver();
Secondly, confirm the source of your files. It’s strongly recommended that you download your Chromedriver directly from the official site (https://chromedriver.chromium.org/home) to avoid the risk of corrupt or infected files.
Lastly, check your Windows OS version and update it if necessary. Go through your system updates and drivers; make sure everything is up-to-date and compatible with each other.
By keeping these checkpoints in mind, it could significantly reduce the occurrences of such error. An efficient code not only relies on strong programming skills but also deep understanding of its running environment including operating system and driver supports.
For further understanding, please visit the official Selenium documentation page Selenium Documentation.
Sources:
– Selenium HQ – https://www.selenium.dev/
– W3C WebDriver – https://www.w3.org/TR/webdriver/For many developers like me, automating browser tasks with Selenium is a very important part of their workflow. However, as you’ve pointed out, there sometimes may be compatibility issues when using ChromeDriver and Selenium on Windows OS. One such incompatibility issue manifests as the “Failed to read descriptor from node connection: A device attached to the system is not functioning” error message.
Let’s delve into this issue.
Causes of the Issue
This error generally arises when an installed version of ChromeDriver isn’t fully compatible with the version of Google Chrome installed on your Windows machine. Few further reasons might be:
- The downloaded version of ChromeDriver isn’t compatible with the one used by Windows.
- A third-party software manipulation or firewall could cause interference between ChromeDriver and Selenium WebDriver.
- There could be a wrong configuration setting that is causing this issue.
Each different version of ChromeDriver supports a specific range of Chrome browser versions. Therefore, if your installed Chrome version and ChromeDriver versions don’t align, you will probably encounter compatibility problems.
Solution Strategies
The solution to this problem usually involves ensuring that both Chrome and ChromeDriver are mutually compatible. You have several options:
1. Update Both Chrome and ChromeDriver: Ensure that both the Chrome browser versions and the ChromeDriver versions are up-to-date. This solution works because updates often come with bug fixes designed to resolve existing compatibility issues.
You should use the following commands to upgrade ChromeDriver:
# Locate and enter your webdriver-manager directory: cd [directory] # Clear out downloaded drivers: webdriver-manager clear # Update drivers: webdriver-manager update # Verify successful update: webdriver-manager status
2. Match The ChromeDriver Version To Your Chrome Version: If updating doesn’t work, check the Chrome browser version and manually download that same version of ChromeDriver from the official ChromeDriver downloads page [here](https://sites.google.com/chromium.org/driver/).
To locate your chrome version:
1. Open Google Chrome.
2. Click the vertical ellipsis (⋮) icon in the upper-right corner of Chrome.
3. Hover over ‘Help’ and click ‘About Google Chrome’.
Afterwards, while installing the correct version of ChromeDriver, follow these steps –
# Remove the current chromedriver rm /usr/local/bin/chromedriver # Install the correct version of chromedriver wget https://chromedriver.storage.googleapis.com/VERSION_NUMBER_HERE/chromedriver_linux64.zip unzip chromedriver_linux64.zip sudo mv chromedriver /usr/local/bin/ sudo chown root:root /usr/local/bin/chromedriver sudo chmod +x /usr/local/bin/chromedriver
ChromeDriver Version | Supported Chrome Version |
---|---|
92.0.4515.43 | 92.0.4515 |
91.0.4472.101 | 91.0.4472 |
90.0.4430.24 | 90.0.4430 |
After applying these tips, you should no longer receive the “Failed to read descriptor from node connection: A device attached to the system is not functioning” error message and your Selenium functions should operate correctly!
References:
When you’re working with Chromedriver and Selenium on Windows OS and you receive the error “Failed to read descriptor from node connection: A device attached to the system is not functioning,” there could be many potential causes. However, let’s dissect this problem step by step:
Understanding the Error
The error “Failed to read descriptor…is not functioning” usually manifests when your test script attempts to interact with an element that has either been removed or is not working in the expected way. This could be due to reasons such as the device being disconnected, driver malfunctions, or issues/connection probed with USB devices.
Steps To Fix The Error
Let’s go through some steps that can potentially help mitigate this issue:
1. Update or Reinstall Your Web Driver (Chromedriver)
One primary cause of issues when working with Selenium is having an outdated Chromedriver. Ensuring that you have the most recent version of Chromedriver can often resolve many headaches.
Here, the best first course of action is to update your version of Chromedriver.
WebDriverManager.chromedriver().setup();
If this doesn’t resolve your problem, another option is to reinstall Chromedriver entirely.
2. Check and Update Your Google Chrome version
Make sure that the installed Chrome browser and the Chromedriver are compatible. Every version of Chromedriver supports certain versions of Chrome only. Visit Chromedriver download page to find out the compatible versions. If they don’t match, then updating to the appropriate versions can help.
3. Verify Connection and Status of Attached Devices
Another important area to investigate when encountering this error message is the status of the devices connected to your computer. Make sure all connected devices are working correctly. Disconnect, then reconnect the device, if possible, to reset the connection.
4. Adjust Your Test Script
Sometimes the issue is not with your system environment, but rather with the test script itself. For example, if your script is trying to interact with an element that isn’t loaded yet, it will fail. You may need to adjust your script to wait for this element to load. The simplest way to achieve this in Selenium is to use explicit waits and conditional waits.
5. Scan for Hardware Changes in Device Manager
Rescan the Device Manager for hardware changes as sometimes it can help force-launching the system detection routine and thereby fixing the “Device Descriptor Request Failed” error.
Use this code snippet to rescan for hardware changes:
devmgmt.msc
Your job is more like a detective’s work. Keep checking these various areas until you find the source of your problem. Remember, patience is the fellow developer’s weapon when debugging! If these suggested solutions don’t seem to help, seeking assistance from online communities like Stack Overflow can be helpful – someone else may have encountered the same specific issue before.
Source Codes:
WebDriverManager Github Repository
Selenium Documentation
When you encounter the “Failed To Read Descriptor From Node Connection: A Device Attached To The System Is Not Functioning” error message, this is usually indicative of a problem with ChromeDriver when trying to automate tasks in Selenium on a Windows Operating System environment.
The first step toward addressing this issue within Selenium involves understanding its root cause. The error message signifies that the problem lies not within your code or arrangement of scripts, but instead it is likely caused by a malfunction with the USB driver, Chromedriver’s interaction with it, or a node connection communication issue. Here is an in-depth examination of potential causes:
- Incidental Glitch: In some instances, the error will just be an incidental glitch beyond our control as developers. This can arise from such things as intermittent connectivity issues or minor temporary compatibility bugs between different systems and chrome’s binaries.
- USB Device Compatibility & Drivers: If a malfunctioning or incompatible device is connected via USB during chromedriver execution Browser, this particular error can be triggered. The problem may also lie on the USB driver itself. These drivers could either be outdated or they have compatibility issues.
- Chromedriver Issue: On occasions, you might find that a specific version of chromedriver has a bug which doesn’t play well with certain system configurations or versions of selenium and throws out this error.
Now let’s explore various troubleshooting steps for this issue:
- Reboot Your System: Given that this error can sometimes arise due to a simple system glitch, the most straightforward solution is to reboot your system before anything else. Although it is simple, ever so often, this tactic fixes many problems that appear to manifest in rather complex ways
- Disconnect Unnecessary USB Devices: Disconnect unnecessary USB devices that are not relevant to the running of your selenium scripts. Then re-run your automated tests to ascertain if the fault still exists.
- Update Drivers: Update USB drivers if the error persists. Drivers should always be kept up-to-date to help mitigate any device compatibility issues.
- Update Chromedriver: Lastly, an upgrade to the latest Chromedriver release could resolve any feature-bug conflicts inherent in the current Chromedriver setup, leading to a successful resolution of the error.
from selenium import webdriver driver = webdriver.Chrome('/path/to/updated/chromedriver') driver.get('https://www.yourTestWebsite.com') # Proceed with your automated actions here. driver.quit()
Note: Replace /path/to/updated/chromedriver with the path to the updated ChromeDriver executable and replace ‘https://www.yourTestWebsite.com’ with your test webpage URL.
This information should provide you with a clear path towards alleviating the `”Failed To Read Descriptor From Node Connection: A Device Attached To The System Is Not Functioning”` error message. Such errors can without doubt be frustrating, but through approach of systematic troubleshooting, these challenges can mostly be overcome. Keep this guide handy for reference to swiftly navigate this type of predicament in your future interactions with chromedriver and selenium on Windows OS.
I can’t help but empathize, as I’ve previously found myself in a similar predicament, trying to automate my work using Selenium WebDriver with the Chromedriver setup on my Windows system. This was until I encountered an incessant error popping up – “Failed to read descriptor from node connection: A device attached to the system is not functioning.” Initially, I thought it must be due to some peripheral device issues or OS glitches, while in reality, the solution was not out of the box; instead, it lay within the myriad of settings, library versions, and drivers that I needed to tweak and adjust.
Error Description
The error message “Failed to read descriptor from node connection: A device attached to the system is not functioning” indicates that there’s an issue with Chromedriver and Google Chrome Browser compatibility. Typically, you might experience this problem when your Chromedriver version doesn’t match your Google Chrome Browser’s version.
Possible Solutions
To rectify this issue with Selenium WebDriver set up on your windows OS, I suggest you consider the following solutions:
- Update Your Chromedriver:
The most probable reason for this error is because the Chromedriver version is incompatible with your existing Google Chrome browser. Updating the Chromedriver using WebDriverManager can usually solve the problem.
Inside your Python environment, you could use pip command to install the webdriver_manager module and then update the respective driver.
pip install webdriver_manager from selenium import webdriver from webdriver_manager.chrome import ChromeDriverManager driver = webdriver.Chrome(ChromeDriverManager().install())
- Update Your Google Chrome Browser:
If updating your Chromedriver didn’t resolve the issue, I suggest considering upgrading your Google Chrome Browser to the most recent version.
- Downgrade Your Chrome Version:
In rare cases, downgrading your Google Chrome Browser version to match the compatible Chromedriver version also works when the latest updates or features rolled out have bugs or technical glitches.
- Setting Binary Location:
Another method to solve the error would be to set the binary location explicitly in the webdriver code. Here is a sample of how that might look like:
from selenium import webdriver from selenium.webdriver.chrome.service import Service from selenium.webdriver.common.by import By from webdriver_manager.chrome import ChromeDriverManager from selenium.webdriver.chrome.options import Options options = Options() options.binary_location='C:/Users/username/AppData/Local/Google/Chrome/Application/chrome.exe' service=Service(ChromeDriverManager().install()) driver = webdriver.Chrome(service=service, options=options)
Note: Make sure to set binary_location to the correct Google Chrome path on your system in options.binary_location.
Reference Documentation and Further Reading
Mull over the official documentation of Selenium WebDriver and Chromedriver for additional information. You can also refer to articles and stack overflow questions where experienced programmers share their experiences dealing with such issues pertaining to Selenium WebDriver and Chromedriver.
These proposed solutions are based on my coding expertise and hands-on experiences with Selenium WebDriver and Chromedriver. One should realize that what worked for one might not work for others. It’s all about finding what fits best in your situation after understanding the root cause of the error.
The “Failed to read descriptor from node connection: A device attached to the system is not functioning” error when using Chromedriver Selenium on a Windows OS is typically an indicator of some mismatch between the versions of Selenium, Chrome, and Chromedriver you are using.
To resolve this issue, it’s paramount to ensure that you’re working with compatible versions of these software. Especially, the Chromedriver should match the version of your installed Chrome browser.
Let’s consider in detail how to address each component:
– Inspecting the Chrome Browser Version: Navigate to the ‘About Google Chrome’ section under help in the Chrome browser menu to confirm its version.
– Matching Chromedriver with Chrome: Download the version of Chromedriver which corresponds with the installed Chrome browser. The version information can be confirmed from the Chromedriver homepage.
Regarding Selenium:
– Selenium Web Driver: Download the most recent stable version of selenium WebDriver. This can be done via Python pip installer
pip install -U selenium
At times, this problem could also arise if your webpage contains embedded elements sourced from the http protocol, while your main site is running on https. If this is the case, altering the webpage or using specific webdriver settings(like ignoring certificate errors) may nip the problem in the bud.
Another approach to resolve this lies in tinkering with the start-up preferences of your chromedriver. You could configure it to start maximized, thus dispensing with the need for explicit window size setting commands that sometimes precipitates this error.
For example,
from selenium import webdriver from selenium.webdriver.chrome.service import Service option = webdriver.ChromeOptions() option.add_argument('--start-maximized') driver = webdriver.Chrome(service=Service('path_to_chromedriver'), options=option)
There may be other possibilities as well depending on your configuration or the website you’re trying to test. By following the guidance aforementioned, your solution path presumptively becomes more discernible.
Given the versatility of Selenium, its breadth of capabilities means that troubleshooting requires a bucketful of patience. But remember, the best error is the one you never make. Invest time in understanding the nuances of your tools and their interoperability constraints, and you’ll be able to avoid many common pitfalls that often trip up developers. Remember to always keep your tools updated and versions synchronized, and you’re well on your way to harnessing the full power of Selenium on Windows OS.