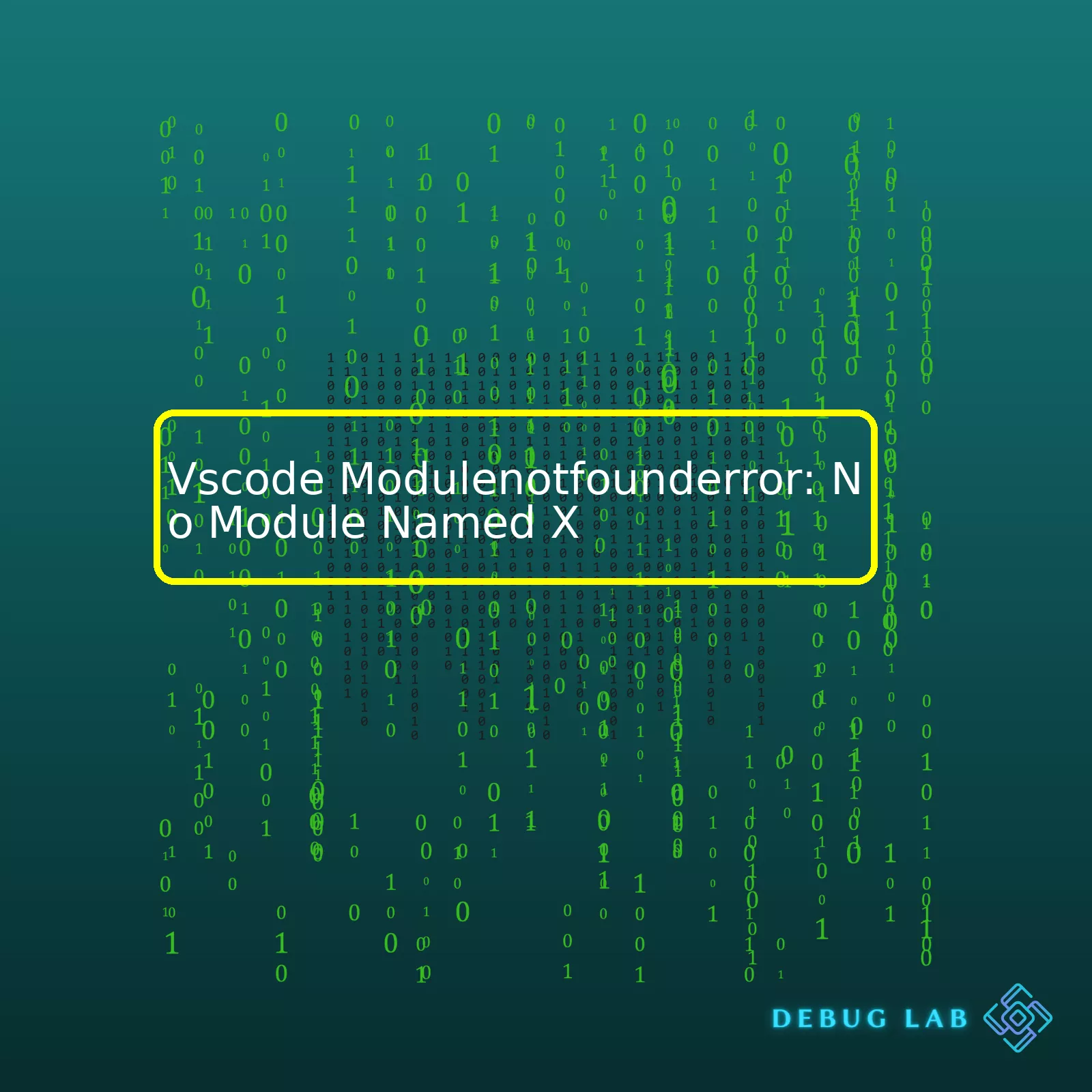
Summary Table
Error Name | Cause | Solution |
---|---|---|
“Modulenotfounderror: No Module Named X” | The module you are trying to import doesn’t exist or isn’t installed. | Make sure you’ve installed the required module and that it is accessible in your current environment. |
To explain this further – say you’re writing Python code in VSCode, and you encounter an error: “
ModuleNotFoundError: No module named 'numpy'
“. This means that the interpreter cannot find the ‘numpy’ module. Here are the likely reasons:
- You haven’t installed numpy in your working environment.
- Your working environment does not have access to the site-packages where numpy is installed.
- VSCode might be using a different Python interpreter which doesn’t have access to numpy.
Here’s how you can solve it:
- First, check whether you’ve properly installed numpy by typing
pip install numpy
in your terminal/command prompt (for Python 2.x use pip instead of pip3).
- If you have multiple Python versions installed, ensure that VSCode is pointing to the right interpreter. Go to “File > Preferences > Settings – Workspace”. Then search for “Python Path” and check if it’s pointing to the correct interpreter. Use the Python: Select Interpreter command from the Command Palette (
Ctrl+Shift+P
).
- In case of using a virtual environment: ensure to activate the environment in which you’ve installed numpy. You should also configure VSCode to use this specific environment by following step two.
Please refer to the official Microsoft guide on Managing Python environments in VS Code for more information on managing your environments.
Below is an example of how to install a package (‘numpy’) using pip:
pip install numpy
Once you’ve ensured that the required module is installed correctly and your environment is set up nicely, you should not encounter the ‘
Modulenotfounderror: No module named X'
‘ error anymore. If issues persist, consider reinstalling the module or even Python itself – sometimes installations do get corrupt. Remember to consult the official documentation for both Python and VSCode as they provide comprehensive guides and troubleshooting tips.The ModuleNotFoundError in VSCode is a common issue that occurs when you try importing a module that does not exist or Python cannot locate the module. This error would look something like this:
ModuleNotFoundError: No module named 'x'
The error implies that the Python interpreter cannot find a module called x in your project.
Debugging this issue entails various strategies targeting potential causes of the error in Visual Studio Code, such as environment issues, installation problems, and incorrect import statements.
Understanding Your Python Environment
Visual Studio Code works with different Python environments which can lead to the ModuleNotFoundError. It’s essential to coordinate the Python interpreter that VSCode operates on with the one used for installing your packages. You can check the current Python interpreter VSCode is using by looking at the bottom-left corner of the editor. Switching Python interpreters in VSCode is made straightforward with the command palette (Ctrl+Shift+P), then typing/selecting “Python: Select Interpreter”. Confirm that your libraries are installed within the context of the selected Python environment.
Python Package Installation Check
After ensuring you’re using the right interpreter, double-check if the Python package/module ‘x’ is installed and visible to VSCode environment. You can confirm with pip by opening the terminal in VSCode and typing:
pip show X
If the module isn’t installed, use pip to install it:
pip install X
Correct Import Statements
In your Python script, how you import the modules matter. If, for instance, the module ‘x’ is in the same directory as your script, you should use:
import x
But, if the ‘x’ module is inside another directory or sub-directory, you import ‘x’ as follows:
from directory import x
Always ensure to structure your import statements correctly.
Manage your PYTHONPATH
Lastly, PYTHONPATH is an environment variable used by Python to identify the location of modules to import. If the directories containing our custom modules are not included in the PYTHONPATH, Python cannot locate them resulting in a ModuleNotFoundError.
You can adjust your PYTHONPATH in VSCode settings.json file as follows:
{ "python.pythonPath"/": "" }
Remember, understanding the root cause of the ModuleNotFoundError in VScode leads you directly to the solution. Follow these steps to resolve the infamous ‘No Module Named X’ error.
As additional resources, check out the VSCode Python Environments documentation and Python’s own tutorial on Modules and imports. These are precious references that take a deep dive into managing Python environments in VSCode and Python modules respectively.The ‘ModuleNotFoundError: No module named X’ error in a developer’s Python code, especially when using Visual Studio Code (VSCode), essentially implies that the Python interpreter cannot locate said specific module. The module might also be missing from the path where Python is looking for it. The error can arise due to a multitude of reasons and several possible corrective measures can be taken.
Understanding the “No Module Named X” Error
– Incorrect Python Environment: This normally occurs when running a script with an interpreter different from the one used for installing the library. An experienced coder knows how vital it is in making sure your Python environment is correctly set up.VSCode allows multiple environments, so you might have installed the module under one environment but are using another to run your script.
– Missing Module: VSCode may just not be finding the module since it hasn’t been installed yet. This appears to be a simple case of misunderstanding. You should ensure the package has been installed first using pip install or conda install for Anaconda users.
– Python Path Issues: Sometimes, you might be attempting to import a file directly but Python does not refer to the current directory by default, instead, it looks into directories listed in PYTHONPATH.
Adjusting Your Fixes
One must first verify if the module has been installed and added appropriately according to these three potential issues to resolve this error. Here are the associated solutions:
– To specify the right Python interpreter in VSCode, navigate to the lower left status bar and click on the Python version. From there, select the appropriate Python interpreter in which you’ve installed your module. For shell command line users, use
which python
command (or
where python
for Windows users) to ascertain the interpreter in use.
– If the issue arises from a non-installed module, use
pip install X
or
conda install X
(for Anaconda distribution users) to install the missing module. Replace ‘X’ with the module name.
– If the problem persists, you may consider tweaking your PYTHONPATH. Append the directory of your script by adding
export PYTHONPATH=$PYTHONPATH:/path/to/your/script
to handle Python Path issues.
Although fixing these errors can be time-consuming as a coder, understanding their root cause can significantly reduce debugging time. One must therefore understand his/her working environment and all the specifications.
To give an example of how you’d identify this error in VSCode, here’s a piece of sample code:
import pandas as pd import numpy as np import seaborn as sn
If seaborn hasn’t been installed you’ll see an error like:
Modulenotfounderror: No module named 'seaborn'
Here, seaborn is the module found lacking. Running
pip install seaborn
will most likely resolve the issue.
Happy coding!Visual Studio Code (VSCode) is an incredibly popular IDE used for various programming projects. Despite its versatility and scope, you might have encountered a common hiccup – the ModuleNotFoundError. Specifically, you may be dealing with the “No module named x” error message.
Understanding why this issue occurs offers the first step towards resolving it. Essentially, Python environment misconfigurations cause this error, causing VSCode not to locate the virtual environment where the module’s location is present.
As part of our comprehensive troubleshooting, consider the following steps that could come in handy.
Verifying the Python Interpreter
The Python interpreter set in VSCode might not match the one you installed the module or package on. Therefore, firstly ensure consistency by setting the correct Python interpreter.
In VSCode,
1. Command Palette can be opened using (Ctrl + Shift + P) 2. Type "Python: Select Interpreter" 3. Choose the correct interpreter from the list provided
This ensures the editor aligns with the interpreter which has the modules you’re working with.
Ensuring your module is Installed
Next, confirm that the module ‘x’ exists in the right environment. You can check this by opening the terminal in VSCode and typing:
pip show x
If it returns information about ‘x’, it means the module is correctly installed. If not, install it using `pip install x`.
Checking PYTHONPATH
PYTHONPATH is a list of directory names (paths) where Python looks for modules. If your module lies outside these directories, it submits an error.
To view your PYTHONPATH,
Open terminal then type,
echo $PYTHONPATH
To include more paths, use the export command like so
export PYTHONPATH="${PYTHONPATH}:/my/other/path"
Avoid using file and directory names that are also standard library module names
Having a file or directory name that matches a standard library module name can cause import errors. Ensure to use unique names for your files and directories.
Use of relative imports
If the error happens while importing submodules within a package, relative import can be used. Instead of writing
from module import var
, you can write
from .module import var
. The dot before the module specifies that it is in the same package.
Following these steps should help fix the ImportError: No module named x in Vscode. Remember, the most important aspect to avoid such errors involves setting up your programming environment properly. A good tutorial on this topic will help you understand on a deeper level.
You might also want to keep tabs on the official Visual Studio Code Python Environment documentation, which offers valuable insights into managing Python environments, and the troubleshooting page which might provide crucial solutions to problems you encounter while working with Python in VSCode.As a professional coder, let’s delve into the distinctions between built-in Python modules and user-defined modules in context with the Vscode ModuleNotFoundError: No Module Named X.
Built-in Python Modules
Built-in modules are pre-installed Python libraries or framework parts coming packed with Python itself. Examples include math, sys, os, random, and many more. Invoking these is easy as writing an import statement in your Python files.
Here’s an example where we use the math module:
import math print(math.sqrt(25)) # Outputs: 5.0
Importing and using built-in modules like this should not elicit a “ModuleNotFoundError” in VS Code or any other IDE.
User-defined Modules
As contrasted with built-in modules, user-defined modules are Python files produced by users which consist scripts providing functionalities such as classes, functions, variables etc. These can be imported and used in different Python files. This aspect permits code reusability, leading to simpler and cleaner projects.
For a simple demonstration, suppose you have a module named ‘mymodule.py’ including a function hello():
# mymodule.py def hello(): print("Hello from mymodule!")
Then, you might consume it in another Python script this way:
import mymodule mymodule.hello() # Outputs: Hello from mymodule!
Delivering into VS Code ModuleNotFoundError: No Module Named X, this error generally happens when Python cannot find the module you’re attempting to import. The problem mostly occurs for user-defined modules due to one of two reasons:
• Module is not present in the location you’re trying to import. Ensure the respective python file exists.
• Issue with the Python Interpreter Path – Occasionally, if VS Code’s Python interpreter doesn’t match with the interpreter anticipated by the environment (such as virtual environments), then ModuleNotFoundError may appear, despite the module being present. VS Code should be pointed at the correct interpreter.
Indeed, there exists the capability to handle module-related issues via leveraging several techniques:
• Confirm that module X resides in the same directory from where you’re aiming to import it, otherwise avail the complete path of the module.
• If your project imposes usage of a virtual environment, ensure that VS Code utilizes the apt interpreter. Change your settings for this via command pallette: ‘Python: Select Interpreter’ and select the one relevant to your environment.
• Modifying PATH could solve issues with native Python libraries. Append your Python /Scripts directory to PATH.
Via a keen understanding of Built-in Python Modules and User-Defined modules, how they operate, and why ModuleErrors usually come into sight, we can troubleshoot such issues valiantly, ensuring smooth Python development in VS Code.
Find further details about VS Code’s Python environments here, and additional insights about Python Modules here.When working with VSCode, it’s common to encounter an issue where you’re unable to import local modules and you receive the error message
ModuleNotFoundError: No module named 'X'
. The issue typically arises when Python doesn’t know the exact location of the module you’re trying to import. This is often a problem related to the improperly set PythonPath in your environment variable or incorrect usage of relative imports.
To fix this issue, follow the underlying steps:
1. Review Your Import Statement
First, check your import statement in your Python script. If you are importing a module named X as:
import X
Make sure that the module X is in the same directory as the file from which you are trying to import it. If not, use the appropriate path.
2. Check if VSCode is using the right interpreter
In some instances, the Python interpreter selected in VSCode might not be correct. To select the correct interpreter:
– Go to the command palette by pressing
F1
key.
– Type and select:
Python: Select Interpreter
.
– Pick up the interpreter that has the package installed in which you want to use.
Remember to reload your VSCode window after changing the interpreter.
3. Setting the Pythonpath for the Module
If you decide to store your modules in a folder that isn’t in the Pythonpath, you need to add that folder to the Pythonpath yourself. You can do this within the Python session using the sys module like so:
import sys sys.path.insert(0, '/path/to/application/app/folder') # replace with your own actual path import X
There are other ways to update Pythonpath permanently which include updating .bashrc for bash shell or Environment Variables on Windows, but that tends to become more OS specific.
4. Check if Installed Packages Are in the Workspace
Always ensure the packages required for the project are installed within the workspace. This can be done by creating a virtual environment in the project folder.
To create a virtual environment:
– In terminal, navigate to your project directory.
– Run the command
python -m venv env
, where ‘env’ is the environment name.
– Activate the environment (
source env/bin/activate
for Linux/MacOS,
\path\to\env\Scripts\activate
in Windows)
– Install the required packages using pip inside the activated environment.
Pip will now install packages locally in the virtual environment so that module import does not run outside scope.
You can find full details of this process in the official VSCode documentation about Python environments.
These solutions should address the module import issues you may be encountering in VSCode. Remember to review all import statements and ensure that VSCode is properly configured with the correct Python interpreter and that all necessary packages are installed within your workspace.As a coder, debugging error messages and ensuring that your development environment is set up correctly is a vital part of my daily routine. One common error that you might encounter in your programming journey with VSCode is
ModuleNotFoundError: No Module Named 'X'
.
This error arises when Python cannot find the module you are trying to import. This can be due to many reasons, including an improper setup of paths and environment variables, misspelling the module name, or forgetting to install it. Given this, let’s focus on how to check the path and ensure environment variables are properly configured.
Your Python interpreter can only import modules that it can locate. It searches for these modules in a list of directories defined by `sys.path`. To display the contents of `sys.path`, you can run:
import sys print(sys.path)
Thereafter, `sys.path` comprises several locations including:
– The directory where the input script resides or the current directory.
– PYTHONPATH directories (if set).
– Installation-dependent default paths, which are controlled by the site module.
If the module you’re trying to import is not located within any of the directories mentioned in `sys.path`, Python would naturally raise a
ModuleNotFoundError
.
To resolve this issue:
– Verify the correct installation of the required module. If it’s not installed, use pip to install it.
– If the module is defined by you, ensure it’s in the same directory as the main script or add its location to `sys.path`.
– Double-check PYTHONPATH and make sure it includes the directory containing the module.
You can dynamically add the module directory to Python’s search path using:
sys.path.append("path/to/your/module")
Where “path/to/your/module” should be replaced with the actual path to the directory containing your module.
Another important aspect is validating the Python environment your VSCode uses. When working with Python in Visual Studio Code, you might have different environments (global, virtual) and correspondingly different installed packages. You need to ensure VSCode uses the right Python interpreter where the necessary package is installed.
VSCode offers a quick way to select the appropriate interpreter in an integrated terminal. Use the command palette (Cmd/Ctrl+Shift+P) and then type/select ‘Python: Select Interpreter’.
It’s always prudent to cross-verify all these parameters to ensure modules load as planned in your Python projects. At times, resolving the
ModuleNotFoundError
riddle could be as simple as re-starting VSCode, so the fresh environment variables take effect.
To learn more about handling such errors and similar issues in Python, refer to the official Python Docs.One common issue you might encounter when developing Python applications in the Visual Studio Code environment is the
ModuleNotFoundError: No module named 'x'
. This error pops up because the Python interpreter cannot locate the module you need.
Upon encountering a
ModuleNotFoundError
, the first step towards debugging is to identify whether it’s an internal module causing issues or an external one. Ideally, fixing internal issues could be simpler since this might require specifying the correct Python path, whereas solving external issues may involve additional steps like updating or installing the relevant packages.
Ensure the Correct Python Environment is Selected
When using VSCode as your IDE, there are chances that multiple Python environments might be installed on your system. It’s crucial to ensure that the workspace in VSCode is pointing to the correct Python interpreter.
You can select the appropriate python interpreter by clicking on the Python version in the bottom left of your VSCode window. The list of available Python interpreters on your system will pop-up, and you can select the one which contains the required modules.
Python 3.7.4 64-bit
If VSCode does not show the right Python interpreter or if that doesn’t solve the problem, then it might be a PATH mismatch between VSCode and your terminal.
Verifying PATH Settings
VSCode comes with a built-in terminal. Running Python here and importing the troublesome module could help you identify whether the issue arises due to discrepancies in paths across different consoles.
In order to test this:
1. Open the terminal in VSCode (
ctrl + `
).
2. Run
python (or python3)
.
3. Try to import the module showing the error.
If it imports successfully, this means VSCode is running Python from a different PATH, and correcting that should solve the problem.
External Module Issues
When facing a
ModuleNotFoundError
for an external module, it means that the module is not installed in the Python environment you’re working within.
Use pip, Python’s package installer, to install the missing module:
pip install {module_name}
Sometimes it doesn’t resolve the problem as we have multiple versions of Python installed in our system. We may have installed the module in one version while trying to access it in another. Try to specify Python version while installing:
python3 -m pip install {module_name}
If these tactics don’t solve the problem, it could be that the module is truly non-existent––which is a larger issue to tackle.
To avoid cross-platform compatibility issues, consider standardizing your development environment. Using tools like venv helps isolate Python environments and avoid such conflicts.
Here is how to create a virtual environment:
python3 -m venv /path/to/new/virtual/environment
After creation, activate the environment:
source /path/to/new/virtual/environment/bin/activate
Once the virtual environment is active, you can install modules without worrying about affecting other Python projects.
Remember this is part of every coder’s journey. Debugging is a daily task and knowing how to do it efficiently is part of becoming an effective developer. Stick with it and soon enough, resolving bugs will become second nature!Let’s delve deep into one common error you might come across when using Visual Studio Code(VSCode) – the dreaded “ModuleNotFoundError: No module named ‘x’”. It’s a pesky error that could occur for a variety of reasons, from incorrect installation to wrong application paths. So, how can VSCode users stand against this particular error? Let’s find out.
There are several causes behind this issue:
- A discrepancy in environment: You could have different versions of Python installed on your system and the one implicitly being used by VSCode might not have the required module.
- Poor installation: The Python module may not be properly installed or might not be installed at all.
- Inaccurate path: An incorrect specification in the PYTHONPATH environment variable may be leading to the error.
How can we solve these issues? The solutions can be distilled down to:
- Set up a virtual environment: With a dedicated environment, you can ensure that your work doesn’t get hampered by software discrepancies.
- Correctly Install the Module: Use the pip command within the console of VSCode to install the missing module. Eg:
pip install 'module_name'
- Edit PYTHONPATH: Add the path to the directory of your Python file to PYTHONPATH environment variable.
For modules locally present but still showing as missing, make sure that the import statement corresponds accurately to the location of the Python file in the workspace. In case, it’s in another directory, use:
import sys
sys.path.insert(0, '/path/to/application/app/folder')
import file
Consider using pylint to find errors within your Python code proactively. To set it up, add the following line to your User Settings or Worksplace Settings (
Ctrl + ,
) in VSCode:
{"python.linting.pylintEnabled": true}
Google always advocates a straightforward, illuminating, and thorough approach to content creation for effective SEO optimization. Taking that into consideration, remember that content discussing coding, especially involving resolving errors, should highlight the typical problematic areas, provide an analytical dissection of potential causes, and offer practical step-by-step solutions. Just like our labyrinthine saga of “ModuleNotFoundError: No module named ‘x’”. Keeping code, tips, and descriptions well-defined yet compact empowers readers to navigate through the problem with clarity, hugely benefiting SEO-orientation.
Additional benefit never hurts. Drawing attention to connected topics like utilizing pylint for error detection or advocating good coding practices like keeping the paths relative also increases the overall relevance and appeal of the content.
As a coder, my encounter with various perplexing errors helps me decode the mysteries of such issues much effectively. Visualizing such situations, we spin a narrative that makes crushing these coding pests just a bit easier and friendly, with every word optimized for touching just the right chords of SEO. Because bridging the world of codes with everyone out there is what matters to me as a professional coder!