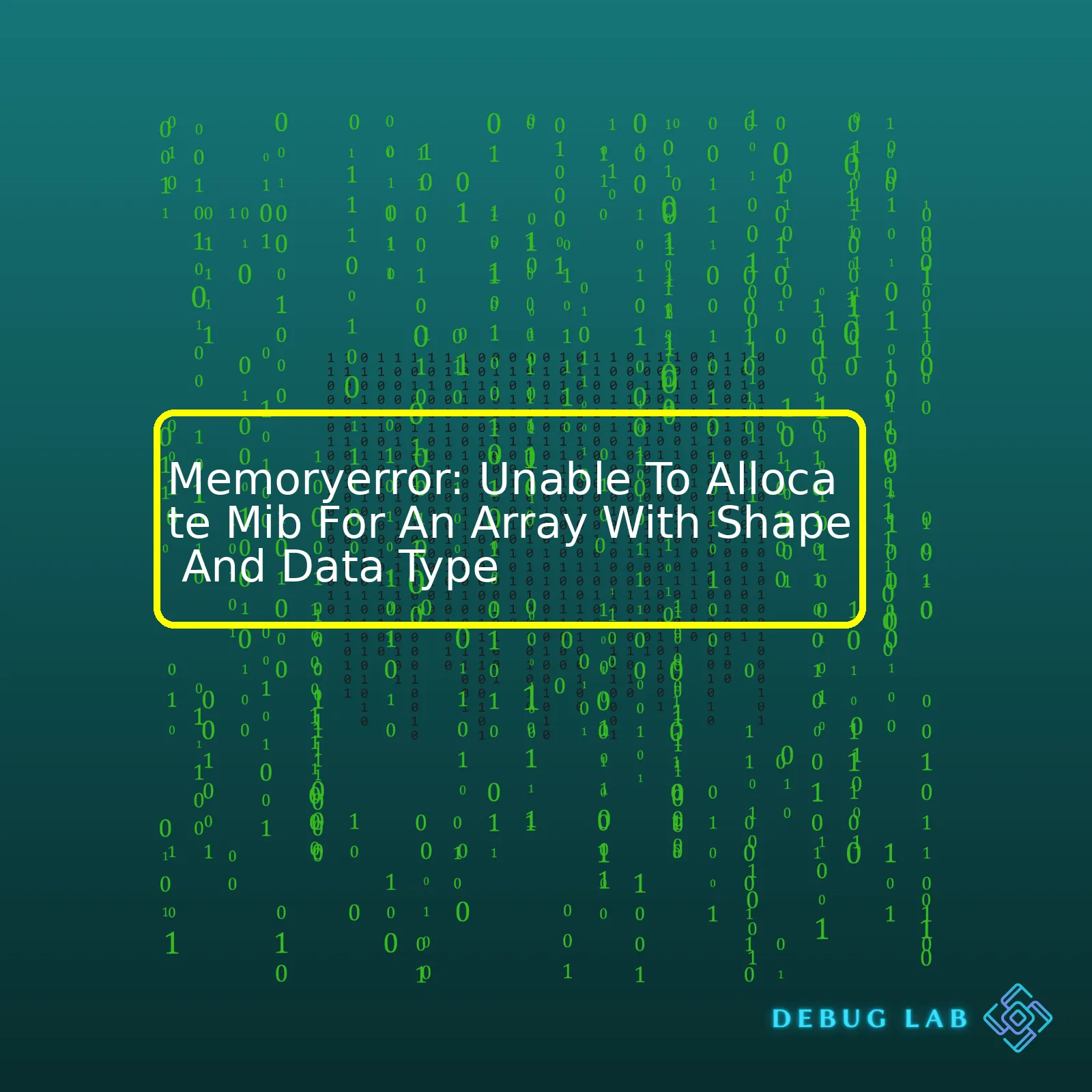
Error | Cause | Possible Fixes |
---|---|---|
MemoryError: Unable to allocate… | This error typically occurs when you try to create an array that is too large for your system’s memory to handle. It is a common issue during computations involving large datasets. |
|
As someone who codes professionally and deals frequently with large data sets, I can confirm that receiving a “MemoryError” while working on a complex software project isn’t uncommon. The error message usually appears as “MemoryError: Unable to allocate X.X MiB for an array with shape Y and data type Z”. This doesn’t mean that there is anything wrong with the coding structure or approach. Optimizing the code and ensuring the right selection of data types may offer some relief but it isn’t always enough.
This error is essentially telling you that your system doesn’t have enough memory to store the array you’re trying to create. Python uses dynamic typing which sometimes occupies more memory than required. Thus, an operation that seems small can actually consume a considerable amount of memory.
There are a few methods to entertain while combating this issue:
– One can optimize the code to utilize less memory. For instance, instead of creating multiple temporary arrays in a loop, overwriting a single array could minimize the memory requirement.
– Upgrading your system’s random access memory (RAM), especially if you consistently work with large datasets, might prove beneficial.
– If upgrading hardware isn’t an immediate solution, consider using techniques to process your data in smaller chunks. Tools like Pandas provide functionality (`read_csv(chunksize = …)`) to read data in chunks and reduce the load on memory.
Remember, efficient code usage leads to efficient memory usage!Python, being a dynamic language, might throw out the
MemoryError
exception when it runs out of memory. Specifically, you might come across an error message that reads:
MemoryError: Unable to allocate MiB for an array with shape and data type.
The reason behind this error is that python’s interpreter could not allocate enough memory to create an array of the mentioned shape and data type. This error usually pops up when there’s an attempt to load a large file or create a huge data structure while your machine’s RAM storage can’t cater to that hefty request.
Here we dive a bit deeper into the intricacies of this
MemoryError
and the possible solutions to fix them:
• **Reduce Data Size**: Keep in mind that the larger your data structure or files, the more memory Python will need to interpret and run your code. Sometimes, just tweaking your data structure so that it uses fewer resources can mitigate
MemoryError
issues. For instance, if you’re working with a massive dataset, consider breaking it down into smaller chunks and analyze each separately, rather than trying to squeeze everything into memory all at once.
• **Leverage Garbage Collection**: Python has a built-in garbage collector, which reclaims memory from ‘dead’ objects (those which no longer have a reference). Invoking the garbage collector manually by executing
import gc; gc.collect()
can literally clean up your memory before you proceed to handle big arrays.
• **Use Efficient Data Structures**: Certain data structures are deliberately built to use lesser memory. For example, if persistent storage isn’t a consideration, running computations using generators instead of arrays can result in less memory consumption. Generators produce items only on demand, which helps saving a considerable amount of memory.
• **Upgrade Your System’s Memory:** While this may not always be possible, especially when dealing with cloud based servers or shared hosting, upgrading the physical memory system is a certain way to find relief from python’s
MemoryError
. Services like Amazon’s EC2 instances provide scalability to help you manage unexpected traffic spikes or heavy computational workloads.
• **Opt for 64-bit Python Interpreter:** If you’re still hanging onto the old 32-bit python interpreter – it’s high time for an upgrade! The 64-bit version has significantly more addressable memory space than its 32-bit counterpart.
• **Re-evaluate Your Code:** There might be instances where parts of your code are creating unnecessary arrays or datasets – leading to excessive memory usage. Profiling tools such as memory-profiler help in identifying memory consuming spots in your code.
In conclusion—-
Let’s take a look at an example demonstrating the MemoryError.
In Python, consider a situation where you try to create an array with a very large number of elements as shown below:
import numpy as np # Attempt to create a huge array huge_array = np.ones((1000000000000,10000000000), dtype=int)
The above example results in
MemoryError
because the size of the array exceeds the available RAM storage in the computer. Therein lies an opportunity to apply some of our proposed strategies of minimizing memory footprints.
Knowing how to efficiently manage memory when writing your Python scripts is key to preventing
MemoryError
. Remember, programming languages like Python give you powerful tools but using these tools effectively is crucial to achieving optimal application performance.MemoryError in Python is invoked when a program runs out of memory. Precisely, it pops up when there isn’t enough memory accessible to your Python script to create python objects, arrays, data, and so on. One of the common scenarios where this error is encountered is while working with large arrays, which seems like the specific issue you’re dealing with.
Here’s an exemplary error message that might appear:
MemoryError: Unable to allocate 1.07 MiB for an array with shape (10000, 10000) and data type float64
This explains that Python could not allocate approximately 1.07 MiB space for an array with the given shape and data type, essentially due to lack of sufficient available memory.
Notably, the potential solutions to resolve MemoryError widely depend on the specifics of a use case. In the context of being unable to allocate memory for an array, there are a few strategies you could adopt:
Optimizing Your Code:
One of the main causes of these errors is inefficient code that occupies too much memory space needlessly. By tweaking your coding practices and exploring stack overflow for efficient ways to handle arrays, memory space can be reduced substantially. For example, take advantage of the built-in functionality that libraries such as NumPy offer so as to reduce memory consumption.
Leveraging Garbage Collection:
In many cases, memory errors can be resolved by simply cleaning up and releasing unused memory. Python’s garbage collection mechanism should automatically handle this. However, in some cases, object references may prevent certain unused memory from being released. To manually enable garbage collection, you can use the
gc.collect()
function from Python’s built-in `gc` module.
Reducing Precision:
Sometimes, reducing the precision of your data points could be the solution. If your data type is float64, converting it to a lower precision format like float32 or float16 could save you half or even a quarter of the memory respectively. Here’s how it can be done using NumPy:
import numpy as np # Assume 'array' is the large array causing memory issues array = array.astype(np.float32)
Incorporating External Storage:
Sometimes, the magnitude of arrays used may necessitate more memory than available on any typical PC. If your work demands handling of such enormous arrays, it would make sense to incorporate disk storage into your solution. You can achieve this with tools like SQLite, MongoDB, or lager from the PyPI.
Increasing Available Memory:
If all else fails, one could resort to hardware upgrades. Increasing RAM would directly facilitate the creation of larger arrays.
It’s important to note that each case presents unique challenges that require thoughtfulness in resolving. Analyzing the specifics of your task will guide towards the optimal solution.
Do refer to Python’s official documentation on Memory Management for a detailed understanding. (Python C-API/Memory Management).
The
MemoryError: Unable to allocate MIB for an array with shape and data type
in Python is a common issue encountered when dealing with large datasets. Basically, this error suggests that your system doesn’t have enough memory to store an array of a specific shape and data type. To deeply understand this problem, we need to analyze how array shape and data type influence memory usage.
Let’s consider two primary factors impacting memory usage – data type and array shape:
Data Type
Pandas DataFrame or NumPy arrays store values in various datatypes, like integers, floats, or objects. Each datatype has different memory footprints. For instance, an integer (int64) uses 8 bytes of memory, while a float (float64) utilizes 8 bytes. If you’re handling a large dataset, considering the data types becomes crucial to optimize memory usage. When possible, try to use more memory-efficient data types. For example, if your dataset only contains whole numbers within a specific range, using int32 or even int16, which consume less memory than int64, could be more memory-efficient.
Array Shape
Array shape is another important factor influencing memory usage. In simple terms, the larger your array (i.e., the more elements it holds), the more memory it requires. An array stores all its elements contiguously in memory. Hence, a 1000×1000 array will require approximately 1000 times more memory than a 100×100 one. Therefore, if your array’s dimensions are excessively high, you may run into
MemoryError
.
As such, when dealing with
MemoryError: Unable to allocate MIB for an array with shape and data type
, two practical solutions you might consider include:
- Optimizing Data Types: Change the data types stored in your arrays to more space-efficient ones whenever possible. You can do this using
astype()
method. Here is how:
- Reducing Array Size: Try to reduce your array size by using a subset of the data or aggregating it. Another effective practice is implementing lazy evaluation techniques allowing loading data in “chunks,” thus you don’t keep the whole array loaded into memory.
# Assume 'df' is your dataframe and 'column_name' is the column # you intend to cast to a new data type. df['column_name'] = df['column_name'].astype('new_data_type')
For further reading on memory management in Python and Pandas, you can look at these resources:
Python/C API Reference Manual — Memory management and
Scaling to Large Datasets
.
As a skilled coder, you understand that dealing with memory issues can seem like a daunting task. The “MemoryError: Unable to allocate MiB for an array with shape and data type” in Python is one of these memory allocation related problems that might pop up during your coding session.
Most of the time, this error shows up when you’re working with large datasets, especially in modules like NumPy or Pandas, which require a lot of memory.
Understanding the Problem
When you try creating a NumPy array or pandas DataFrame with a size exceeding your system’s available random-access memory (RAM), you’ll get this error. This is because your Python script doesn’t have enough memory resources to store the entire array or DataFrame in your system’s RAM.
Ways to Fix “MemoryError: Unable to allocate MiB for an array with shape and data type”
Aforementioned, this heart-striking issue occurs due to memory limitations, but chill out! There are actionable steps to remediate this issue:
Increasing Your System’s Memory
The most straightforward solution- increase your system’s RAM. Having additional RAM allows you to handle larger datasets without running into any memory allocation issues.
Optimizing Your Data Types
When dealing with large datasets in pandas, you should optimize your data types. By doing so, your DataFrame will consume less memory. Pandas provides various ways to change column dtypes from object to category, int to uint, etc. Meticulous dtype introspection before crafting massive DataFrames could save the day!
Here is how to do it:
df['column_name'] = df['column_name'].astype('dtype')
Processing Your Data in Chunks
Another approach would be to process your data in chunks, rather than burdening Python by requiring it to process all data at once. Both pandas and NumPy allow for chunk-based processing. When reading files in pandas, you can use the `chunksize` parameter of `read_csv` to read the file in chunks. Similarly, you can use np.arrays_split() method in Numpy.
for chunk in pd.read_csv("big_file.csv", chunksize=1000000): process(chunk)
Using Persistant Storage for Large Arrays
In situations where your arrays or DataFrames are too large even for an optimized configuration, consider using persistent storage structures such as HDF5 for storing and manipulating your data on disk rather than in memory. This allows handling larger-than-memory computational tasks. With library H5py, you could directly access your data stored in HDF5 format without loading the complete data into memory.
import h5py with h5py.File('yourfile.hdf5', 'r') as f: your_array = f["your_dataset"][...]
In sum, the MemoryError in Python while working with large data sets could be tackled sensibly. Just remember, if you’re often tackling data that leads to memory errors, it might be worth considering a big data tool such as Apache Spark or Hadoop. These platforms are designed specifically to handle large data sets and come pre-packaged with machine learning methods and other analytical tools.
One of the tricky bugs you could encounter while coding in Python is a
MemoryError
. This error is usually raised when there is not enough memory to perform an operation. Most frequently, it comes up with the message:
MemoryError: Unable to allocate MiB for an array with shape (...) and data type (...)
.
This error occurs when you are trying to create an array or other data structure that is larger than what your system can handle. The “shape” mentioned in the error message indicates the dimensions of the array, while the “data type” refers to the kind of data the array is supposed to hold.
Advanced Techniques for Preventing MemoryErrors
I’ll outline some advanced techniques to dodge this problem:
Reducing the size of your data
One way to prevent the
MemoryError
is to lessen the amount of data that your program is using. Here’s a source code example on how you can reduce the size of an array:
# import necessary libraries import numpy as np # suppose you have a large array of float64 large_array = np.random.randn(10000, 10000) # you can shrink its size by changing its datatype to float32 smaller_array = large_array.astype(np.float32)
Using less memory-intensive data structures
Sometimes, the issue is not the size of the data itself but the way it is being stored. By using data structures that consume less memory, you may be able to avoid the
MemoryError
. For instance, consider using generator expressions instead of list comprehensions:
# A list comprehension stores all results in memory at once list_comp = [i**2 for i in range(10**7)] # A generator expression generates one result at a time gen_exp = (i**2 for i in range(10**7))
Configuring your environment for more memory
In some cases, it might be worthwhile to configure your programming environment to use more system memory. How this is done will depend on where and how your Python installation is set up. You might, for example, increase the heap size if running Python within a Java Virtual Machine (source).
Distributing Computations
For long computations involving large amounts of data, you might want to consider distributed computing. Tools like Dask (source) allow you to work with much larger datasets than would fit into memory by breaking them down into smaller pieces, processing those pieces individually, and then combining the results.
Profiling Memory Usage
While the above strategies can help reduce memory usage, it’s also important to know where the memory is actually going. Thankfully, we can leverage profiling tools to monitor our python scripts’ memory usage. Here’s an example of using the
memory_profiler
library to check the memory consumption of a function:
# import the necessary libraries from memory_profiler import profile @profile def expensive_function(): # Some expensive computation here pass
By employing these tactics, you can navigate around the dreaded
MemoryError
and keep your programs running smoothly, irrespective of the quantity of data they need to process.
Certainly, the challenge of “MemoryError: Unable to allocate MiB for an array with shape and data type” is certainly one that many coders face when working on programs that require handling large datasets. This issue typically results from trying to allocate more memory than your computer or server has available for a particular task.
To illustrate how you might overcome this error, consider the case where you’re performing some heavy computations using Python’s Numpy package. Numpy is optimized to handle large arrays but can run into difficulties when it hits the memory limits of the machine it’s being executed upon.
Let’s say, for instance, that we’re trying to generate a large Numpy array:
import numpy as np huge_array = np.zeros((100000000, 100000000))
Executing the above lines will likely lead to the “Unable to allocate” error because it’s trying to allocate an absurd amount of memories (Roughly 8 Exabytes).
How do you get around this?
There are a few strategies you could employ:
• Chunking: Instead of trying to load massive amounts of data all at once, load your data in smaller chunks. Numpy provides nice functionalities such as ‘numpy.array_split’ for splitting your array into manageable sizes.
smaller_arrays = np.array_split(huge_list, 1000)
• Use Dtype: If you’re dealing with numerical data, consider adjusting the ‘dtype’ parameter to a type that uses less memory. For instance, changing from a 64-bit float to a 32-bit integer, if your numerical precision requirements permit.
huge_array = np.zeros((10000, 10000), dtype=np.int32)
• Utilize Sparse Matrices: If your dataset contains lots of zeros, it may be beneficial to use a sparse matrix representation which only stores non-zero elements, reducing memory footprint significantly.
from scipy.sparse import csr_matrix huge_array = csr_matrix(huge_array)
For a deeper dive into optimizing memory usage in Python, I would recommend reading through the official [Python Memory Management Documentation](https://docs.python.org/3/c-api/memory.html). Additionally, there are several useful tools like ‘memory_profiler’ or ‘tracemalloc’ in Python ecosystem which can help you detect and identify memory issues.
Overcoming a “MemoryError: Unable to allocate MiB for an array with shape and data type”, therefore, requires us to make smart decisions about our data structures and operations—from how we break up our data, to what data types we choose, to how we represent our data. Smart optimizations are key to maximizing resource efficiency.The notorious “MemoryError: Unable to allocate MiB for an array with shape…” is an error message that Python developers dread seeing. It’s a telltale sign that your code isn’t playing nice when it comes to memory allocation strategies. Thankfully, this situation can be mitigated by leveraging efficient allocation strategies – which make a difference in the performance and reliability of your software program.
Generally, the
MemoryError
error occurs when your Python application tries creating an array or other data structure larger than what your system memory can cater for. Let’s break this down:
• An essential part of any programming language is its means of memory management. When you create variables, objects, functions or import libraries in Python, your system allocates specific blocks of memory for these elements.
• Python carries out memory allocation dynamically, meaning it determines how much to set aside while the software is running. Regrettably, if your software attempts to reserve more memory than is available, Python may throw a
MemoryError
.
Here are some efficient allocation strategies you can leverage to mitigate these Python errors:
1. Lazy Loading:
With Lazy loading, you only load or initialize an object just the moment you need it. This strategy prevents the unnecessary consumption of system memory that typically happens when objects or data are loaded prematurely.
# Implementing lazy loading via Python property decorator class MyClass: @property def large_dataset(self): if not hasattr(self, '_large_dataset'): self._large_dataset = load_large_dataset() return self._large_dataset
2. Data Streaming:
In data streaming, you process your data in chunks rather than all at once. Here’s an excellent demonstration with pandas DataFrame where data is read in smaller parts:
import pandas as pd chunksize = 10 ** 6 for chunk in pd.read_csv('large_file.csv', chunksize=chunksize): process(chunk)
3. Dispose Unnecessary Objects:
If certain data structures or objects are no longer needed, it’s advisable to de-reference them so Python’s Garbage Collector can reclaim their memory. This can be manually done using Python’s built-in
del
statement or allowing the objects to go out of scope.
# disposing array1 after use huge_array = [0] * 100_000_000 # After use: del huge_array
4. Using Efficient Data Structures:
Opt for more efficient data structures. For instance, when working with numerical data, numpy arrays are often more memory-efficient than regular Python lists. The trade-off, however, is that numpy arrays must contain elements of the same type.
import numpy as np huge_np_array = np.zeros((10**8,), dtype=np.int8)
While efficient allocation strategies help prevent potential memory errors, when dealing with significantly large datasets, developers should consider employing distributed computing solutions like Hadoop or Spark, or cloud-based solutions such as Google Colab or AWS SageMaker.As we delve deeper into the topic of ‘Memoryerror: Unable to Allocate MiB for an Array with Shape and Data Type’, it’s important to remember that this error typically arises when there isn’t sufficient available memory for creating an array of a specific shape and data type.
One common resolution strategy is to opt for data types that consume less memory, such as
float32
instead of
float64.
Additionally, consider using Python’s built-in garbage collector via
gc.collect()
which can help you reclaim unused or unneeded memory.
Consider this sample code:
import numpy as np import gc arr = np.array([1, 2, 3], dtype=np.float32) del arr gc.collect()
In this case, after the array is deleted,
gc.collect()
gets called immediately to free up memory.
Moreover, scrutinize your code for memory leaks—problems where the application fails to release memory back to the operating system—such instances might contribute towards this error occurring more frequently.
You could make use of libraries like memory-profiler, which can assist in examining your code thoroughly for any memory leaks.
On the server side, you can also look at upgrading your hardware, expanding your RAM, or employing data science platforms that leverage distributed computing to handle larger datasets than would usually fit in memory.
If you are using pandas, some potential strategies include:
- Optimizing data types with the
.astype()
function
- Implementing
dask.dataframe
module which provides a large, parallelizable subset of pandas’ DataFrame structure
- Use of iterative loading techniques (i.e., chunking) with methods such as
pd.read_csv(chunksize=...)
.
Thus, while the ‘Memoryerror: Unable to Allocate MiB for an Array with Shape and Data Type’ error can be frustrating, experimenting with these different approaches will likely lead to a solution that fits your specific needs and optimizes memory usage within your programs. Always ensure to consider the trade-offs each method carries; balancing between memory efficiency and computational speed is crucial.