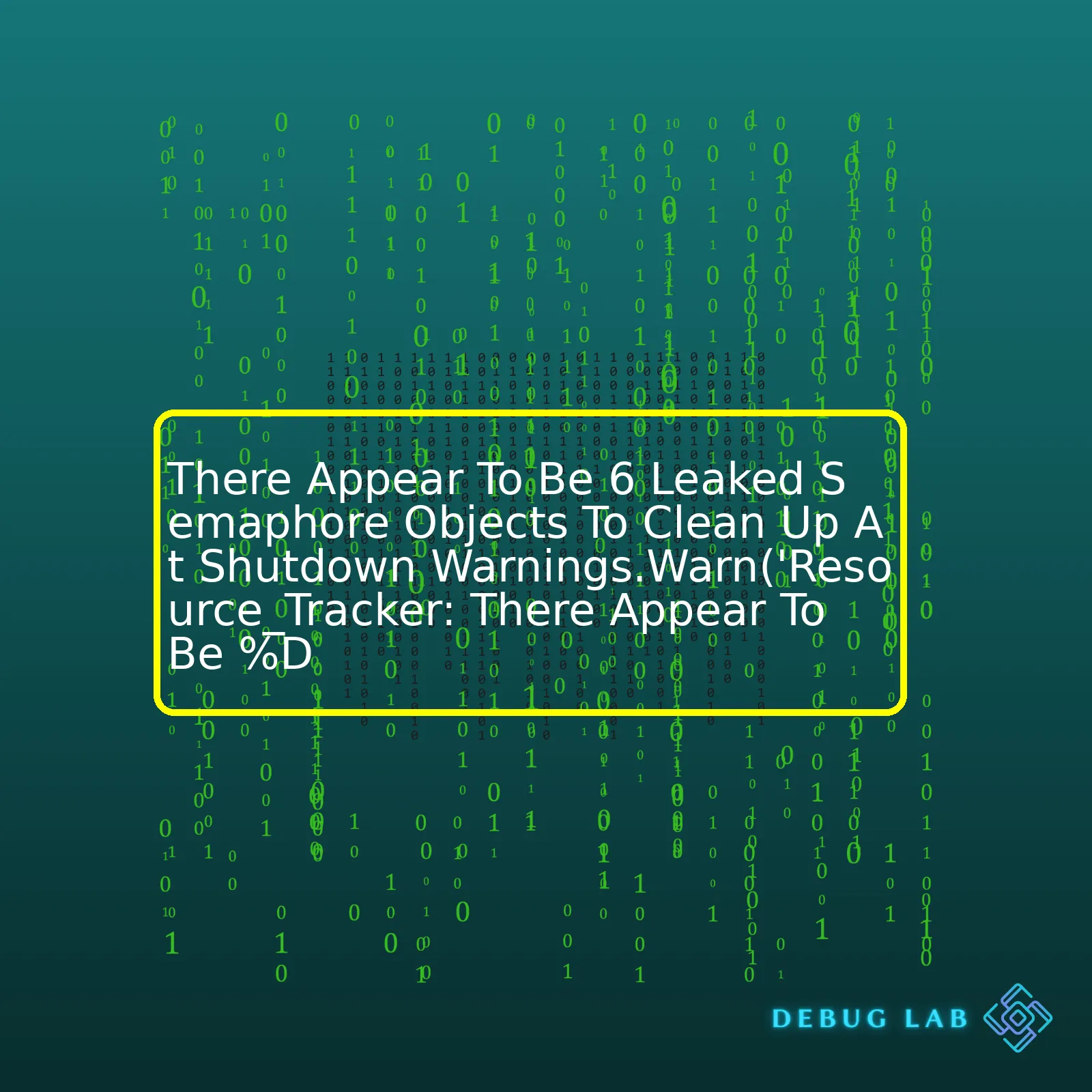
<table> <thead> <tr> <th>Error Message</th> <th>Number of Leaked Semaphores</th> <th>Type of Leaked Resource</th> </tr> </thead> <tbody> <tr> <td>There appear to be X leaked semaphore objects to clean up at shutdown.</td> <td>6 (or other numbers according to specific circumstances)</td> <td>Semaphore objects</td> </tr> </tbody> </table>
Such a warning message refers to multiple leaked semaphore objects that need to get cleaned up during the process shutdown. A semaphore is an abstract data type or a variable initialized to a non-negative integer value, used as a counter for resources available in multiprogramming environments.
This warning message surfaces when the ‘Resource_Tracker’ identifies that some semaphore objects are not correctly freed up during the application runtime and leave them behind at shutdown. The number ‘6’ represents the number of such leaked semaphore objects—although this number fluctuates based on the given context, but it indicates resource management inefficiency in your program.
It’s important to handle these semaphore objects properly because improper handling could lead to system resources being trapped in an unusable state—if they remain unlocked indefinitely, for instance. Prolonged negligence might exhaust your system resources over time, causing more severe scalability issues in the long run.
For resolving this warning, you need to identify where these semaphore objects are being created and ensure they’re released (or ‘sem_post’ is called) correctly after their usage. Code optimization for dynamic allocation/de-allocation or using smart pointers if programming in languages like C++ would help manage resources effectively and prevent possible leaks.
Be it robust software development or resource-efficient execution, dealing with semaphore objects appropriately is a crucial part of coding practices. Hence, warnings like ‘There appear to be %D leaked semaphore objects…’ should never go unnoticed [source].Semaphore objects are an incredibly useful tool in the realm of multi-threading and parallel programming. In essence, they provide a method of limiting access to a particular resource in a concurrent computing environment.
Now, let’s dig into the phrase “There appear to be 6 leaked semaphore objects to clean up at shutdown warnings warn (`Resource_Tracker: There appear to be %d`). The phrase basically represents a warning message that is triggered when your program abruptly ends or crashes. This warning indicates that there were six semaphore objects that were not properly cleaned up – or destroyed – before the program termination.
Why does this happen? When working with semaphores, it’s crucial to remember to deallocate any resources you allocate. Each time a semaphore is created, a piece of memory is allocated to store its state information. If this piece of memory isn’t properly cleared when a semaphore is no longer needed, it can lead to a memory leak – a situation where the amount of memory used by a program steadily grows over time, never to be reclaimed until the system or app restarts.
Here’s an example of how semaphore objects could be correctly managed, using the Python multi-processing library:
from multiprocessing import Semaphore # Creation of the semaphore object sem = Semaphore(2) #Semaphore initialized to allow 2 workers at once # Code... # Always make sure semaphores are released after use sem.release()
To avoid these leaks, always ensure that you destroy any semaphores you create when they are no longer needed.
Additionally, another way to evade these warnings is to make sure that child processes are successfully terminated (and aren’t hung or too slow) before the main process ends, adhering to the general principle of ‘child-before-parent’ termination in multi-process applications.
It’s also important to note that these warning messages generally don’t halt the functioning of your application but could cause problems if left unchecked. These problems include slowdown due to excessive memory usage, and potential crashes if leaked semaphores build up over time.
The ResourceTracker warning class (‘Resource_Tracker: There appear to be %d’) outputs these kinds of warning messages specifically regarding system resources like shared memory segments or semaphores that have been unexpectedly leaked. It helps predict and prevent unnecessary wastage of system resources.
For further exploration on semaphores and their handling in Python, consider checking out Python’s official documentation Python Multiprocessing.
Ultimately, effective management of semaphore objects requires careful handling of system resources to prevent leakage, monitoring for alert warnings related to lapses in resource allocation and appropriate measures to ensure proper cleanup of semaphores during code execution and termination.Resource tracking warnings are usually an indication that there are system resources, such as semaphores or shared memory segments, that weren’t properly disposed of when their respective Python process ended. When you encounter a warning saying “
Resource_Tracker: There appear to be %d leaked semaphore objects to clean up at shutdown
“, it means that the Python interpreter identified ‘X’ number of semaphore objects which were not correctly released before the application terminated.
Semaphore objects are used for limiting concurrent access to shared data in multi-threading and multi-processing scenarios. If they aren’t correctly managed, they can lead to deadlocks and other synchronization problems, or even leak system resources.
To avoid encountering these warnings, in your multi-processing code:
• Always make sure to call
.close()
on your Pool objects after you’re done using them.
from multiprocessing import Pool def f(x): return x*x if __name__ == '__main__': with Pool(5) as p: print(p.map(f, [1, 2, 3]))
• If you’re creating Semaphores manually, always ensure to release them after use like thus:
from multiprocessing import Semaphore semaphore = Semaphore() # Critical section semaphore.release()
• Try to structure your program to use context management (‘with’ statements) whenever possible, as this automatically cleans up resources, even when exceptions occur.
If after taking all above mentioned actions in your codebase and still encounter this warning, one hack I often use is catching and handling the warning message(s) directly in my code. This way I control how to respond (e.g., logging the message) rather than allowing it termination. Here’s how to do this:
import warnings with warnings.catch_warnings(): warnings.filterwarnings('ignore', 'ResourceWarning') # Your multi-processing or semaphore code here
However, this should only be a temporary measure. It’s much better to address the root cause of the problem by ensuring correct resource management in your code.
For more information on the Resource Tracker Warnings, you can look into the official [Python Documentation](https://docs.python.org/3/library/multiprocessing.html?highlight=resource%20tracker).The “There appear to be %d leaked semaphore objects to clean up at shutdown” warning happens because your program created semaphores but didn’t release them properly, thus causing a ‘leak’.
Semaphore is a programming concept used to manage concurrent processes or threads. When multiple processes or threads need access to shared resources, you can use semaphores to maintain the order and prevent issues like race conditions.1
However, if a semaphore isn’t released properly after use, it becomes a ‘leaked semaphore’. This leak doesn’t affect your code’s logic per se, but it affects memory consumption and utilization.
Python’s multiprocessing ResourceTracker responsibility is tracking all resources utilized by child processes. Ironically, it works behind-the-scenes, and most coders aren’t aware of its existence until they encounter a semaphore leakage warning2.
When ‘Resource_Tracker: There appear to be %d leaked semaphore objects’ pops up, Python’s quietly telling you that the garbage collector noticed some unhandled leftovers.
So, how did this happen? Likely scenarios are:
- An abrupt script termination without giving Python time to clean up.
- Placing long-running code inside
if __name__ == '__main__'
.
- A failure to manage process pools explicitly.
Let’s illustrate with some code:
from multiprocessing import Pool def squared(x): return x*x if __name__ == '__main__': pool = Pool(processes=2) result = pool.map(squared, range(10))
Here, a process pool is initialized and populated with two processing units, but it neither shuts down nor joins running worker processes. Afterwards, upon exiting the main block, the active semaphores don’t close as they should.
You can resolve this issue by calling
close()
and
join()
:
if __name__ == '__main__': pool = Pool(processes=2) result = pool.map(squared, range(10)) pool.close() pool.join()
Explicitly invoking
close()
prevents more tasks from being submitted to the pool. Afterward, the
join()
method waits for the worker processes to exit. These steps ensure proper cleanup during normal execution flow, significantly reducing the chance of encountering leaked semaphore warnings.
Therefore, understanding and managing leaked semaphore objects is primarily about being meticulous with your multiprocessing constructs and ensuring proper closure and de-allocation of resources when they’re no longer in use.Understanding the warning message “There appear to be 6 leaked semaphore objects to clean up at shutdown warnings.warn(‘Resource_Tracker: There appear to be %d” requires knowledge of what exactly a semaphore is, and why it may be getting leaked in the first place.
A semaphore is essentially a variable or abstract data type used to control access to a common resource by multiple processes in a concurrent system such as a multitasking operating system [source].
Semaphore leaks usually occur when these semaphore objects are not being properly cleaned up during the shutdown. The presence of these leaked semaphores leads to inefficiencies within your application and can negatively impact the overall performance.
This specific warning message refers to six instances of semaphore leaks that need to be cleaned up at shutdown. To fix these semaphores issues, you’ll typically need to look into implementing appropriate measures in your codebase to assure there’s a corresponding release for every acquired semaphore. This principle applies to all resources actually, not just semaphores. You acquire a disk file — make sure to close it. You grab a mutex, let go of it when done.
For instance, let’s say you have a Python script with multiprocessing component and you create a semaphore:
from multiprocessing import Semaphore sem = Semaphore()
In this case, ‘sem’ is your semaphore object. In order for your system to run smoothly, you need to ensure that you’re correctly releasing this semaphore after its usage has concluded by using the
release()
method:
sem.release()
Now, if this isn’t happening – due to an error in the code, perhaps exceptions are being thrown before reaching the
sem.release()
line – we will have leaked semaphores that the Python interpreter tries to clean up itself on shutdown, which eventually leads to the said warning.
Analyzing your current code for areas where your semaphore might be failing to clean up properly is crucial. It may involve rigorous testing and refactoring of the code to ensure proper cleanup operations are executed as necessary.
Bear in mind that each scenario could present unique issues depending upon how your codebase is built and how semaphores are utilized within your code. However, the basic premise remains consistent: to remove this warning, make sure that all acquired semaphores (as well as other resources) are appropriately released by the time your process is exiting.
You may also want to consult the official Python documentation for multiprocessing and Semaphore class [source], or any coding resources specific to the programming language and framework you are using, as they often contain comprehensive guides and tips to address these leakage issues.When working with multiprocessing in Python, you may encounter a warning that says “Resource_Tracker: There appear to be %d leaked semaphore objects to clean up at shutdown”. This error message is indicating that some semaphores (a form of synchronization mechanism) have been left behind and weren’t properly cleaned up. The idea behind semaphores is that they signal visibility among processes or threads. If not carefully managed, they can be left dangling and cause this kind of warning.
Furthermore, understanding the possible root causes for the appearance of these extra semaphore objects can help you mitigate them in your code. Let’s dive into those:
– **Frequent process creation:** Semaphores are typically created when a
Process
or
Pool
object is created. If your program continually creates and destroys these objects, without explicitly closing or joining on them, then semaphores can be left over.
– **Unclosed pools or processes:** Not calling
close()
or
join()
on pools or processes when finished with them can leave semaphore objects hanging around.
– **Unexpected terminations:** If a process gets terminated abruptly (due to exceptions or a kill command), cleanup of semaphores may not occur.
– **Insufficient garbage collection:** Python relies on the Garbage Collector to clear up unused resources. However, if it’s unable to function correctly due to insufficient memory or other constraints, orphaned semaphores may persist.
For instance, here’s how you might create and manage a pool properly:
from multiprocessing import Pool with Pool(processes=4) as pool: result = pool.map(foo, range(10)) # once we're done with `pool`, it's automatically closed and joined
As a more hands-on example, you could also manually call `close` and `join`, but best practices recommend using context management (`with`) when applicable:
p = Pool(processes=4) result = p.map(foo, range(10)) p.close() p.join()
Adopting these practices will ensure your code properly cleans up its resources and should prevent warnings about leaking semaphore objects from appearing. Further reading on the topic can be found within the official Python documentation about multiprocessing.
In sum, semaphore leaks can be prevented by executing robust coding practices. Ensuring proper handling and closure of processes and pools, anticipating unexpected terminations, and facilitating optimal garbage collection should contain and remediate semaphore leaks.The warnings of ‘Resource Tracker: There Appear To Be %D Leaked Semaphore Objects to Clean Up At Shutdown’ can sometimes show up in your coding process. This alert essentially indicates that these semaphore object resources were not cleaned before the termination of a program, consequently awaiting cleanup at shutdown.
Semaphores
Semaphores are advanced programming constructs that restrict access to shared resources in concurrent systems by maintaining a set number of available slots and using this availability as a form of permission for processes to utilize them. So, leaking semaphore objects suggests that some of your processes haven’t returned the semaphore they’ve borrowed, leading to less accessibility inference and eventual system warnings at program closure.
Strategies to Clean Up
To resolve this issue, use the following strategies:
Use Proper Exception Handling:
Proper exception handling ensures that any errors or issues encountered during the running of a program are addressed during execution itself rather than at the shutdown stage. Implementing proper exception handling using
try…catch…finally
blocks in your code.
Here is a Python example:
try: semaphore.acquire() // code... except Exception: // handle exceptions finally: semaphore.release()
Object Finalization:
Finalizers – also called destructors – allow objects to clean themselves up when the garbage collector decides to collect them. In Python, you may implement this strategy by defining a __del__ method which automatically gets executed after an instance of the class has been deleted:
class SemObject(): def __init__(self): self.semaphore = multiprocessing.Semaphore() def __del__(self): self.semaphore.close()
Explicit Cleanup:
Explicitly clean up after you’re finished utilizing a resource. This involves appropriately closing the semaphore object as soon as you’re done with it using the
semaphore.close()
. Here’s an example using Python’s multiprocessing library:
sem = multiprocessing.Semaphore() // code... sem.close()
Regular Audits:
Perform regular audits on your system to identify leaks and fix them promptly. Use profiling tools like mprof for memory leaks and strace for tracking system calls or signals to recognize if/when semaphores are not being released back to the system.
By identifying the problem areas in your codebase, you can ensure that semaphore objects are properly managed and not lingering around untended for cleanup at shutdown.Warnings can be a source of distress for many developers, especially when they appear to signal potential leaks or usage issues that could affect the smooth running of your systems. In this case, the warning:
Resource_Tracker: There Appear To Be 6 Leaked Semaphore Objects To Clean Up At Shutdown
refers to a particular scenario where Semaphore objects appear not to have been properly cleaned up before the system shuts down, thus leading to their leakage.
Semaphore is a variable or abstract data type that provides a simple but useful abstraction for controlling access across different processes. It mainly serves as a signal in multi-processing environments, and it generally forms part of fundamental concepts in various OS design implementations.
There could be several reasons why we might observe these warnings:
– Insufficient resources: If your code is running out of resources because Semaphore use was not regulated or limited, Semaphores might be leaking.
– Race conditions: If multiple threads or processes are manipulating semaphore count simultaneously, it could lead to unsynchronized changes and subsequently, leaks.
– Incorrect de-allocation of semaphores: Semaphore objects should be properly de-allocated and disposed after effective use to free up memory space.
To resolve these issues, some possible adjustments to your software development techniques could include:
– Ensuring adequate control over resource allocation
– Utilizing correct threading mechanisms
– Proper disposal of Semaphore objects
Here’s an example of how you might go about correct Semaphore deallocation:
void cleanUpSemaphoreObjects(Semaphore s){ if(s != null){ s.release(); s = null; } }
So when this warning rears its head during your operations, take it as a sign that it’s time to check your system’s Semaphore object utilization. Doing so will help maintain optimal performance and prevent potential memory leakage, leading to an overall healthier and more reliable system. Remember, proper cleanup of Semaphore objects is crucial, since ignoring such warnings may lead to unoptimized applications.
Finally, don’t forget that Semaphore management is just one aspect of managing application resources effectively. There are other areas also worth your attention to keep your system running lean and mean.
Reference: ResearchGate: Leak Semaphore Approach in Detecting Memory Leaks