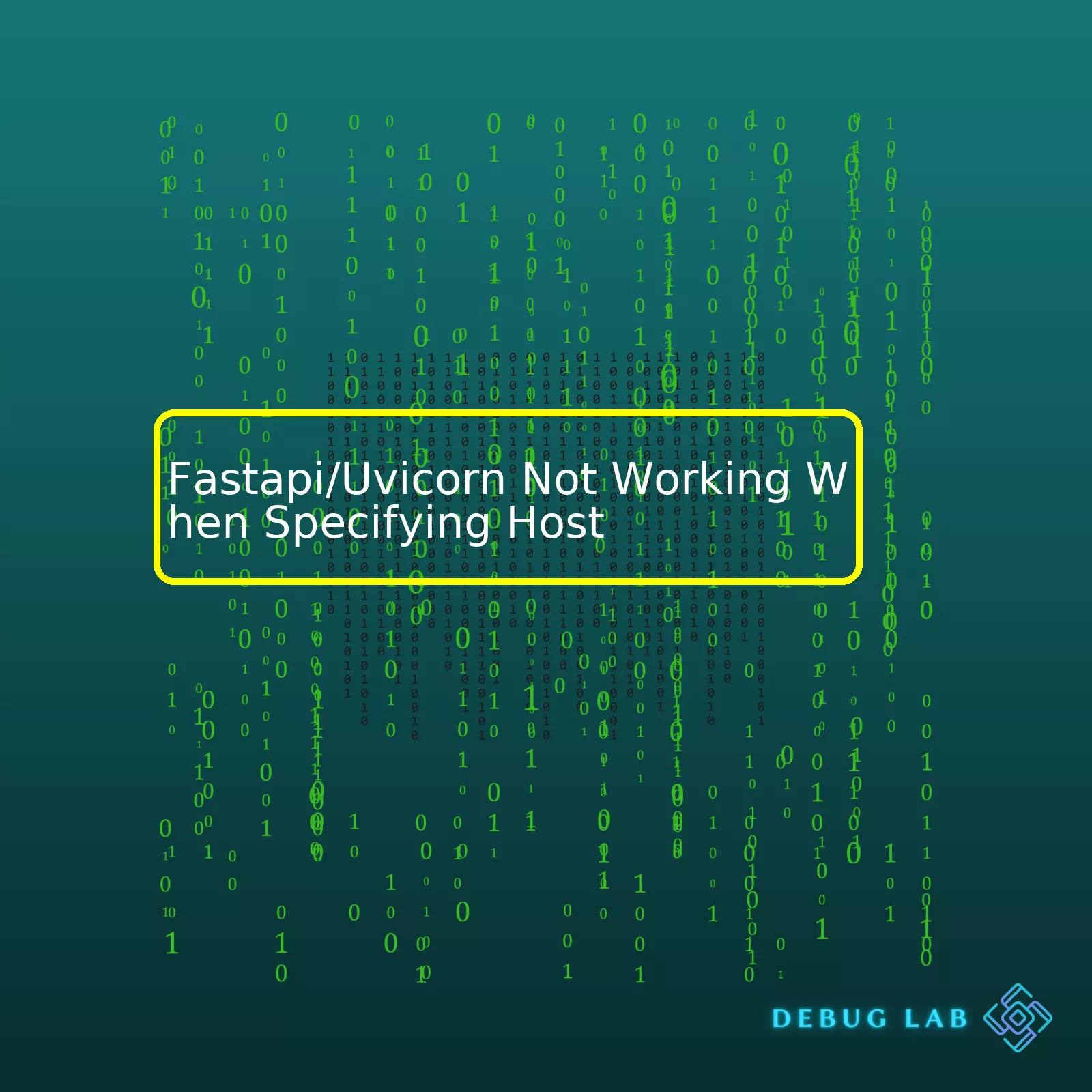
However, you may occasionally come across issues when specifying host in FastAPI or Uvicorn. You may have set up your FastAPI application, specified a host such as ‘localhost’ or ‘0.0.0.0’, but still, find that your site isn’t running as expected.
Here’s how you might be setting the host for your FastAPI app with Uvicorn:
import uvicorn
from fastapi import FastAPI
app = FastAPI()
if __name__ == "__main__":
uvicorn.run(app, host="0.0.0.0", port=8000)
And here’s a summary table highlighting some potential reasons why this could be happening and their solutions:
Potential Problem | Possible Solution |
---|---|
Host specification incorrect | Ensure that you are specifying the correct host. Typically, chose ‘0.0.0.0’ for global, ‘127.0.0.1’ for local, or ‘localhost’. |
Port might already be in use | If the port you’re trying to use is already in use by another service, you may face problems. Consider switching to a different port. |
DNS resolution failed | If you’re using a DNS name for the host and it is failing to resolve, check your DNS setup. You might need to switch to a numeric address or correct the DNS entry. |
Diving deeper into these potential issues, incorrectly specified hosts are one of the more common mistakes particularly for those new to web development or networking. Different systems can interpret hostnames differently – ensure that you’ve used a proper host format. For instance, ‘0.0.0.0’ represents an IP address that’s obtainable from any network interface on your system.
However, if a given port is already being occupied by another process, then also an error will occur. In this case, picking an unoccupied port would solve the problem.
Lastly, when a DNS name is adopted rather than a numeric address and doesn’t resolve, there can be connection difficulties. This might indicate a flawed DNS setup, and adopting a numeric address instead, or fixing the DNS configuration, could be beneficial.
Remember, effective debugging is key in development. Take a moment to understand what each part of your code does. If an issue arises, breaking down the code and understanding each component individually can help you pinpoint exactly where the problem lies. Whenever something doesn’t work, try to investigate the specific behavior further and seek out possible underlying causes.If you’re trying to get FastAPI and Uvicorn working together but are experiencing issues when specifying the host, there are several factors that could be causing the problem.
1. Wrong host address
The initial step in troubleshooting is to verify your host specification. The host command parameter should always be set to the right IP where your application runs on. If it is incorrect or incorrectly formatted, there might be connectivity issues.
For example, to specify the host as localhost, simply write the command:
xxxxxxxxxx
uvicorn main:app --host 127.0.0.1
Be sure that your server is set to listen to requests at this IP.
2. Firewall restrictions
Check if there might be firewall settings blocking incoming or outgoing connections to/from the specified host. Often, network protocols like TCP, HTTP, etc., can be blocked which prevents your server from receiving, responding to requests or even causes a total server failure.
Contact system administrators or consult with your hosting provider for possible solutions.
3. Port conflicts
By default, uvicorn will listen on port 8000. If another application on your server is also using this port, there could be a conflict which causes your FastAPI/Uvicorn setup not to work properly.
You might need to check for running processes that could be using the port 8000. In Unix-like systems, you can use the `lsof` command:
xxxxxxxxxx
lsof -i :8000
If an application is already using this port, consider stopping it temporarily or changing the port of the conflicting application.
Alternatively, you can specify a different port for Uvicorn:
xxxxxxxxxx
uvicorn main:app --host 127.0.0.1 --port 8080
4. Bug in application code
A bug in your FastAPI application code could lead to failing response handling or complete shutdown. This might make it seem like the FastAPI/Uvicorn setup is not working when specifying host even though it’s actually running fine.
In this case, inspect your logs closely for any unhandled exceptions or error messages. It may point to places in your code where a critical exception was throwed and not handled properly.
5. Issues with server configuration
Lastly, there could be an issue with your server configuration that is causing problems. Check all configurations related with your service. Inspect networking configurations specially, ensuring all requisitions and firewall rules are correctly placed.
Ensuring FastAPI/Uvicorn works smoothly with your specific host involves addressing potential misconfigurations, taking into account possible blockages within your network or firewall, returning expected errors from your service and dealing with any server instability. A good starting point for diagnosing FastAPI related issues is the official FastAPI debugging guide. For Uvicorn specific issues, the Uvicorn deployment guide could give you some hints.When coding with FastAPI, it is crucial to understand how the host specification works. If you’re encountering issues where your FastAPI/Uvicorn application isn’t working when specifying Host, there are several potential reasons worth considering.
Firstly, let’s analyze what the role of a host specification in FastAPI and Uvicorn is.
A
xxxxxxxxxx
–-host
parameter in Uvicorn defines the network interface that the server will listen on. By default, Uvicorn, alongside FastAPI, listens on localhost (127.0.0.1). This configuration means that it can only receive requests from the same machine where it is running. However, if you set the host as
xxxxxxxxxx
–-host 0.0.0.0
, then Uvicorn allows connections from anywhere on the internet. It essentially opens up your FastAPI application to accept all incoming communication requests. It’s relevant to note, if security concerns arise, it’d be prudent to configure an appropriate firewall.
Now, let’s delve into why FastAPI/Uvicorn might not work when specifying a host.
• Network Interface Restrictions: The error might occur due to restrictions on the specified network interface. There could be other processes using the designated port or system-level blocks preventing Uvicorn from binding to that address.
xxxxxxxxxx
# example command
$ uvicorn main:app --host 0.0.0.0 --port 80 # Port 80 might be used by another process
• Firewall Rules: Another factor could be firewall rules blocking incoming connections on the specified host. You need to ensure that the firewall allows incoming connections to the particular port Uvicorn is bound to.
• Incorrect Host Specification: Lastly, it could be an issue of incorrectly specifying the host in your Uvicorn command. For instance, incorrectly inputting the IP address or misspelling ‘localhost’.
xxxxxxxxxx
# incorrect host specification example
$ uvicorn main:app --host 127.0..1 # Incorrect IP
$ uvicorn main:app --host localost # Misspelled 'localhost'
To troubleshoot these issues:
Start by checking the network interfaces available and their respective permissions on your machine. Validate the host value against these details. Ensure you aren’t trying to bind Uvicorn to a network interface that does not exist or has particularly stringent access requirements.
Next, look into your machine’s firewall settings. Validating whether incoming connections to your chosen port are not being blocked by firewall rules, can help diagnosing the problem.
Lastly, revise the spelling and syntax of your Uvicorn command. Make sure that you’ve clearly spelled ‘localhost’, or you’ve entered a correct and appropriated IP address.
Please remember that while setting host as
xxxxxxxxxx
–-host 0.0.0.0
makes your FastAPI app accessible to any external network, it also opens your app up to potential malicious activity. It is advisable to consult information security guidelines before making such specifications in a production context. Thus take examining factors like firewalls rules seriously, not merely for troubleshooting purposes, but also an essential aspect of secure coding practice.
FastAPI is incredibly powerful and adaptable, yet with this versatility, comes a need to accurately understand its nuances, such as host specification. With complexity, often come challenges, as is the case here. But with careful examination and methodical troubleshooting, even perplexing issues like these can be resolved successfully.
For more detailed understanding refer this link: Uvicorn Deployment. Understanding the host specification thoroughly and resolving any related issues can help ensure smooth execution and effective performance optimization of your FastAPI applications.For running the FastAPI application with Uvicorn, one of the most common issues encountered by developers is related to setting up the host. You might have a situation where you try to specify your host and find that the FastAPI/Uvicorn combination does not work optimally.
Let’s take a closer look at several possible scenarios:
1. You’ve Entered an Invalid Host: Remember, the `–host` parameter requires a valid host address that the server can bind to. If you’re trying to specify a host that doesn’t exist or isn’t reachable such as by making spelling errors, it will obviously lead to failures.
Try this basic code using uvicorn:
xxxxxxxxxx
uvicorn main:app --host 192.168.1.100 --port 8000
In this example, ‘main’ is the name of the Python module (the .py file), ‘app’ is the object created inside the main.py file by the line `app = FastAPI()`, and ‘192.168.1.100’ is the host address you want to bind to. Ensure you adjust these terms as per your set-up.
2. You’re Trying to Use a Reserved IP Address: If you are erroneously setting the host as `0.0.0.0` or `127.0.0.1`, then uvicorn would not function as expected. These IP addresses have special meanings; specifically, `0.0.0.0` means all IPv4 addresses on the local machine while `127.0.0.1` is a loopback address.
For instance,
xxxxxxxxxx
uvicorn main:app --host 0.0.0.0 --port 8000
You’re essentially saying that you want the app to be accessible from any IP address that your machine may have.
3. Unauthorized Attempt: The problem could also arise when trying to bind to a network interface (i.e., specifying a host) without proper permissions. When attempting to run your service on a low-ported number (ports less than 1024), consider that you likely need superuser privileges.
4. Port Already in Use: Another possibility might be the port you are trying to use for hosting your application is already engaged by some other services.
A simple way to check whether a port is already in use is with the command `netstat -tuln`. This will list all the ports currently in use, so you can choose a different, free port.
5. Firewall Settings: Sometimes, even if everything is set correctly, the firewall settings on the computer or network might be blocking incoming connections to the specified host and port. In that case, you need to revisit your firewall configurations.
Remember, debugging involves considering all possibilities for the problem. Compare the error message given by uvicorn and FastAPI with the above points to pinpoint the issue. If you’re still encountering problems, consider seeking technical support with a detailed report of your setup state.
Do know that Uvicorn and FastAPI have rich communities ready to assist with specific situations once you detail out the scenario.
When deploying an application using FastAPI and Uvicorn, you may encounter problems specifying the host. This might lead to your service being unavailable or unresponsive. In order to ensure optimal performance in your FastAPI application, it’s crucial that you correctly specify a host in Uvicorn.
Typically, when running a Uvicorn server, you would use a command like this:
xxxxxxxxxx
uvicorn main:app --host 0.0.0.0 --port 8000
Here, “main” is the name of your Python file (i.e., main.py), “app” stands for the FastAPI application instance inside your Python file, “0.0.0.0” specifies that the app should be available on all available network interfaces, and “8000” defines the port on which the app listens.
If you want to restrict access only to the local machine (for development purposes, for example), you can change the host to “127.0.0.1”. This ensures your application only responds to requests from the same machine:
xxxxxxxxxx
uvicorn main:app --host 127.0.0.1 --port 8000
If specifying the host as above doesn’t work, then there are a few things you might want to consider:
Possible Issue | Solution |
---|---|
You’re trying to bind to a host address that is not available on your machine. | Ensure that you’re binding to an IP address that exists on your current machine and is active. |
The specified port is already in use. | Try changing the port number that you’re binding to. For example, instead of 8000 you might use 8001 or 8080. |
There’s a problem with ASGI lifespan events, causing the server to crash at startup. | Disable lifespan events by using the
xxxxxxxxxx 1 1 1 --lifespan off option. |
Firewall restrictions prevent binding to certain ports. | Check your firewall settings or contact your system administrator. |
Remember that before deploying your application to a production environment, it’s recommended to use a production-grade ASGI server such as Uvicorn, but behind a robust HTTP server like Gunicorn. To ensure an optimal interaction between Uvicorn and Gunicorn in a production setting, use the following command format:
xxxxxxxxxx
gunicorn -k uvicorn.workers.UvicornWorker main:app
This allows Uvicorn to handle the ASGI part while Gunicorn provides a sturdy, battle-tested HTTP server platform.
To sum up, make sure that you’re binding to the right host and port, taking into account the specifics of your network setting and any restrictions imposed by firewall rules. Disabling ASGI lifespan events might help resolve issues related to server startup. When moving to production, remember about encapsulating Uvicorn behind an HTTP server like Gunicorn for optimal performance.
When trying to connect to Fastapi using Uvicorn and specifying a host, but experiencing connection failures, there could be several potential reasons for this issue.
Firstly, it’s critical to mention that FastAPI is a modern, fast (high-performance), web framework for building APIs with Python 3.6+ based on standard Python type hints [source]. It is often run with Uvicorn to provide the ASGI server.
On the other hand, Uvicorn is a lightning-fast ASGI server implementation, using uvloop and httptools [source]. When we specify a host in Uvicorn or any web service, we are trying to bind the service to that particular IP address.
Let’s dive into some of the specific issues you might be encountering:
1. Specified Host is Incorrect
You might have incorrectly typed the host IP address when running Uvicorn. This means that Uvicorn tries binding to an IP address that doesn’t exist or isn’t accessible from its network interface.
FastAPI application is typically run as:
xxxxxxxxxx
uvicorn main:app --host 127.0.0.1 --port 5000
Ensure the host specified indeed exists and is reachable by Uvicorn.
2. Try not to Use `localhost`
Localhost (`127.0.0.1`) is IP address that’s used to establish a connection to the network that hosts your server, assuming both endpoint resides on the same device.
Sometimes, `localhost` might not work especially when trying to access the FastAPI service from a different machine. In such cases, using the actual local IP (e.g., `192.168.1.10`) or `0.0.0.0` will allow machines on the same network to connect.
3. The Port is Already in Use
Each service on a host is identified by a unique port number. Two services can’t share a single port simultaneously. If you’re trying to run your Fastapi app on a port currently in use, you’ll encounter connection errors. Check if the port you chose is already in use and try picking another one.
4. Firewall/Security Groups
The host you’re trying to bind might have firewall settings preventing connections to the specified port. Firewalls monitor and control incoming and outgoing network traffic based on predetermined security rules, blocking unauthorized access while permitting outward communication.
Hence, review your network firewall settings or security group policies if you’re using cloud services like AWS.
5. Network Issues
Rarely, hardware issues or network misconfigurations can disrupt your ability to connect to your specified host. Ensure the host’s network interfaces are functioning correctly, and its networking configuration (DNS, default gateway, etc.) is appropriately set.
Here are examples of successful connections:
If you want your server to be accessible externally, you can do so by:
xxxxxxxxxx
uvicorn main:app --host 0.0.0.0 --port 8080
While if you want to make sure your server is only accessible in your localhost, use:
xxxxxxxxxx
uvicorn main:app --host 127.0.0.1 --port 8080
In conclusion, whenever you face issues connecting to your FastApi service using Uvicorn, firstly make sure you’ve entered the correct host address and port, and secondly, check for potential issues such as ports being in use, firewall restrictions, and network configurations and hardware malfunctions.FastAPI and Uvicorn are top-notch possibilities for developing high-speed web applications. FastAPI is an innovative, rapid API framework, while Uvicorn, a lightning-fast ASGI server, assists in executing Python code effectively.
Using uvloop in place of the traditional asyncio event loop can significantly enhance performance. However, you might come across a difficulty while hosting your application on different machines because specifying a host when launching your FastAPI application with Uvicorn may not work correctly. This could be due to a variety of reasons such as network configurations or incorrect use of command-line arguments.
What to Consider
By default, when you start Uvicorn, it binds only to localhost (127.0.0.1), so it won’t be accessible from other machines. You must set the host parameter explicitly for Uvicorn to make your application accessible from external networks:
xxxxxxxxxx
uvicorn main:app --host 0.0.0.0
However, if the issue continues:
- Check the firewall settings: Firewalls might block specific ports or all incoming connections. You should check your machine’s security settings to ensure that the server’s port (default 8000 for Uvicorn) isn’t blocked.
- Network configuration: Double-check the network settings and ensure your host machine is visible from the network you’re attempting to access your application from.
- Correct use of commandline arguments: Ensure there are no typo errors when passing host via command line arguments to Uvicorn.
While uvloop and other asyncio enhancements can provide significant speed gains, always consider the specific needs and constraints of the system you’re building before picking a solution. The beauty of asynchronous programming in Python, specifically with tools like FastAPI and Uvicorn, lies in the ability to pick and choose the components that best suit your project’s needs.
Therefore, when everything is properly set, you can successfully reap the benefits of using Generic Event Loop System of Asyncio (uvloop) in hosting on different machines in FastAPI using Uvicorn.
Remember to refer to the official Uvicorn deployment documentation to get more insight about how to serve your FastAPI application.
The below table provides the advantages and limitations of using uvloop:
Advantages | Limitations |
---|---|
UVLoop often speeds up asyncio-style networking code by 2x-4x | uvloop makes it hard to integrate I/O library into languages other than Python |
Can replace asyncio’s default event loop | Lightly documented |
Backwards-compatible with the asyncio API | Code execution inside uvloop is slightly slower |
xxxxxxxxxx
import uvicorn
from fastapi import FastAPI
app = FastAPI()
get("/") .
def read_root():
return {"Hello": "World"}
if __name__ == "__main__":
uvicorn.run("main:app", host="0.0.0.0", port=8000, loop="uvloop")
From this sample source code, uvloop has been specified as the event loop policy, which means it will drastically improve your server’s performance provided it is correctly hosted. Remember to always verify that all dependencies are installed.
Useful references:
– Check out the uvloop GitHub page to find out more about how uvloop can optimize your application.
– Don’t forget to go through Uvicorn’s official documents to understand how to correctly specify options such as the host.Coding a Python server with Websockets employing FastAPI and Uvicorn can occasionally lead to network socket related problems. When specifically having trouble launching Uvicorn upon specifying a host, there are several likely issues and corresponding solutions.
Issues and Solutions
Potential issue: The host specified for Uvicorn is incorrect or unavailable.
Solution: Make sure to specify the right host- typically
xxxxxxxxxx
"0.0.0.0"
for allowing connections from any IP. If you are trying to connect remotely, check if the target machine’s public IP is correctly entered.
Potential issue: Application configuration may not be correct.
Solution: Verify the configuration of your FastAPI application. Incorrectly specified parameters may impede the server from running correctly. For example, in the command:
xxxxxxxxxx
uvicorn main:app --host 0.0.0.0 --port 8000
,
the `main:app` segment represents the name and location of your specific FastAPI Python file (`main.py`) along with the variable holding the FastAPI instance (`app`). Ensure this is properly defined in your case.
Potential issue: The port address is currently in use.
Solution: Free the port address or utilize another port. This can happen if another application or service is already using the port you’re attempting to assign to the Uvicorn server. Linux-based systems, can use command-line tools like
xxxxxxxxxx
lsof
or
xxxxxxxxxx
netstat
to check ports in use and kill processes if required.
Potential issue: Inadequate user permissions.
Solution: Run the command as an administrator or root user. Sometimes, certain actions cannot be executed without the necessary permissions.
Potential issue: Firewall or security settings blocking the connections.
Solution: Adjust or disable firewall rules temporarily to ascertain whether it’s causing the problem. Be careful when tweaking your firewall since this could expose your system to potential threats.
Debugging Process
I would recommend following the steps below :
– Firstly, confirm that the host, application, and port configurations match with how they’re declared in your setting.
– If the problem persists, check if any other applications are using the port intended for Uvicorn.
– Inspect if the application has all the necessary permissions to run.
– Finally, examine your firewall and network settings to make sure that they aren’t inhibiting the Uvicorn server.
Remember, performing these actions in-order helps to systematically triage the issue and find out the root cause. Ensuring you have a structured approach can very efficiently deal with network socket-related issues when implementing servers.
For further understanding, I’d recommend going through official Uvicorn documentation and FastAPI documentation. These resources provide comprehensive guides for advanced options and potential scenarios you may encounter while deploying your application.
I’ve had my fair share of experiences when FastAPI/Uvicorn decided to give up on me just because I specified a host. It may seem like an inexplicable dilemma but the solution usually rests in the understanding of the fundamental principles of networking and the inherent structure of Uvicorn/FastAPI.
The good news is, you’re not alone in this. The issue can often arise due to multiple reasons:
- Net address translation or firewall hindrance: If your application is running behind a NAT or a Firewall, it might have restrictions in place limiting traffic from certain specified hosts.
- Inappropriate network adapter settings: If you’re specifying 0.0.0.0 as your host, technically it should listen to requests on all network interfaces. However, configurations with your local machine or network adapters can sometimes interfere.
- Misconfigured DNS entries: Sometimes if DNS entries are configured incorrectly, they may redirect or block traffic essentially yielding similar behaviour.
When you come across this problem, start diagnosing systematically. Consider these steps:
xxxxxxxxxx
# Step 1. Test Locally
uvicorn main:app --host 0.0.0.0 --port 8000
This will facilitate in determining whether the issue lies within the app’s configuration or with external factors such as Firewalls/NAT configuration.
Additionally:
xxxxxxxxxx
# Step 2: Bind to Default Network Interface With Default Port
uvicorn main:app
If you fail to connect while binding the server to the default network interface, additional debuggery becomes imperative. You’ll need to ensure the proper accessibility of your application.
Moreover:
xxxxxxxxxx
# Step 3. Attempt Direct Connection.
curl http://localhost:8000
A direct connection attempt will help verify if there’s any intermediary causing obstructions.
Approaches toward solving problems surrounding Uvicorn/FastAPI include comprehensive comprehension of its inherent structure, configuration settings, and provisioned functionalities. An extensive probe into network configurations and potential roadblocks must be carried out whenever FastAPI/Uvicorn isn’t working as expected after specifying a host. Remember, the devil is most often found lurking in intricate details. By dealing with one roadblock at a time, we can resolve this issue effectively. Focusing on potential solutions rather than bundling it all together results in an efficient problem-resolution methodology. Plus, it also adds to our competency as professional coders and developers in handling networking puzzles.