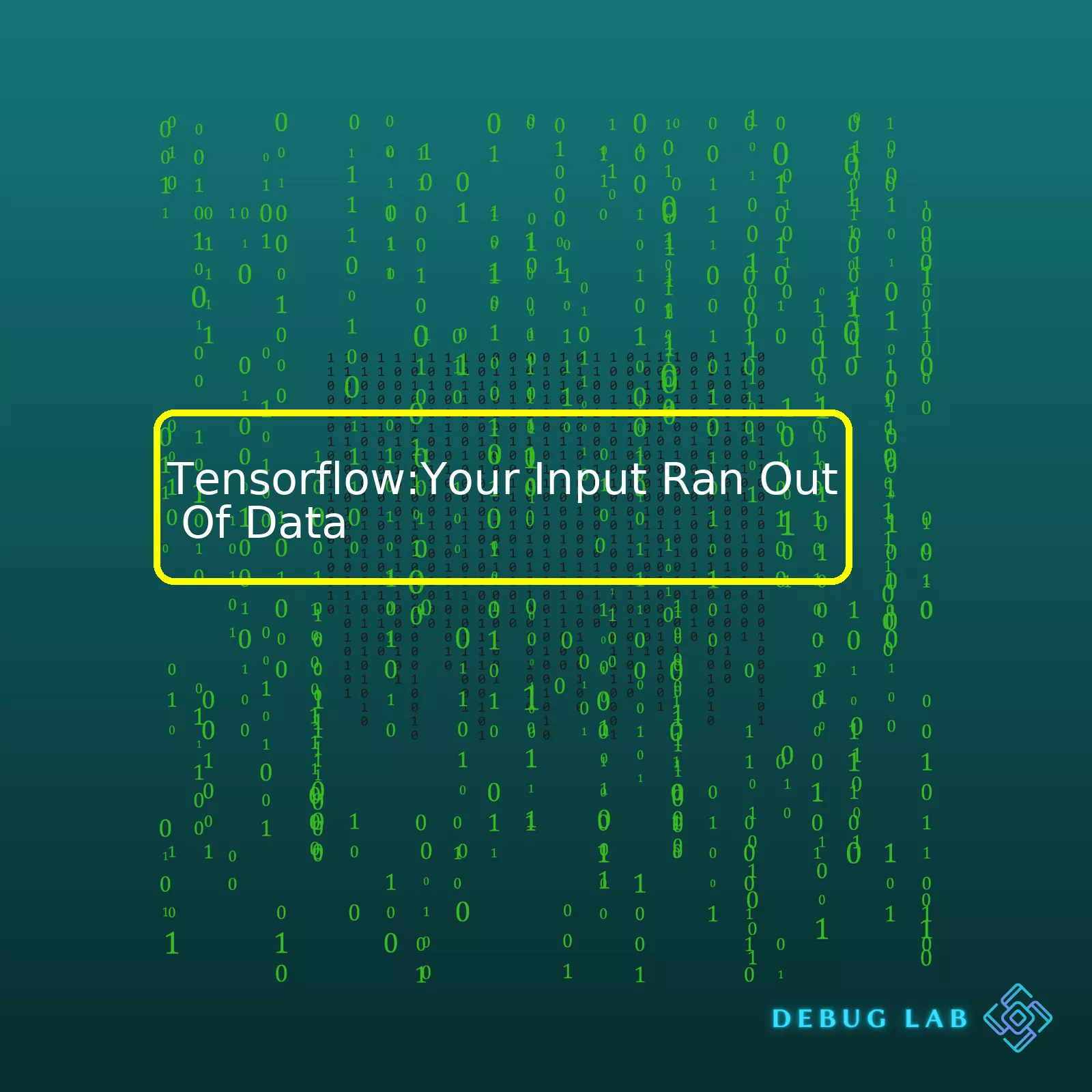
Let’s first start with a basic summary table. This table lists down and explains some of the key factors to consider when diagnosing and resolving the common TensorFlow error ‘Your input ran out of data’; which typically occurs when you’ve exhausted your entire dataset before completing an epoch in training.
Issue | Description | Possible Solution |
---|---|---|
Dataset Size | This pertains to the number of instances in your dataset. If there are not enough samples to fulfill the requirements of a batch or epoch during training, the error may occur. | Ensure your dataset size is adequately large for your model’s needs. Verify your dataset against any set parameters such as batch size and number of epochs.[Ref] |
Division of Dataset | The division of the dataset into training, validation, and test sets can impact whether all the data is utilized or not. | Double-check how you are dividing your data. Make sure it makes sense proportionally and no data subset is left underutilized.[Ref] |
Data Generator | If using custom data generators, coding errors can lead to unintentional draining of the dataset. | Debug your data generator carefully. Additionally, make use of Keras’ built-in data generators, which follow best practices and are less likely to introduce this error.[Ref] |
The “TensorFlow: Your Input Ran Out Of Data” warning comes from the TensorFlow framework and essentially means that your code has consumed all available data inputs; therefore, unable to proceed with subsequent operations like training, validation, or testing. This error isn’t specifically tied to a catastrophic issue but rather reflects an indication that the provided dataset does not align with the specific requirements of the computational model.
This could occur due to reasons outlined in the table above including insufficient dataset size, unbalanced dataset division, or erroneous data generators. The solutions revolve around adjusting these parameters or correcting these issues.
Remember, underpinning every machine learning project is quality data. Dataset size and distribution have direct impacts on your model’s ability to learn effectively. Similarly, a robust data generator is crucial, especially when dealing with large datasets in real-world scenarios. So, always make sure your pre-processing, training, and validation steps take into account the size, diversity, and distribution of your data to avoid encountering the ‘input ran out of data’ error message.
Tackling a TensorFlow error invariably means delving under the hood and working directly with your data. Thankfully, the combination of Python’s analytical horsepower and TensorFlow’s robust framework provides a potent toolkit for fixing the “Your Input Ran Out Of Data” error.
As a coding professional, I can’t emphasize enough the importance of developing good coding habits such as keeping code concise and modular, using up-to-date libraries, carrying out intensive testing, and having a good understanding of your data – all these go a long way in preventing such mishaps. Remember, better data handling practices are your tickets to smoother ride on the deep learning journey.The “Input Ran Out Of Data” error usually arises in TensorFlow when your model attempts to read beyond the available input data. Just as a reader cannot read beyond the last page of a book, neither can TensorFlow explore past the end boundary of the input data.
When dealing with large-scale data, you might use something like a generator or the
tf.data.Dataset
API from TensorFlow, to handle your data in chunks or ‘batches’. This is because loading the entire data into memory at once could be impractical due to hardware limitations. However, while this approach comes with its benefits, it also presents us with the risk of running out of data if not managed correctly. And that’s when the “Input Ran Out Of Data” error pops up!
Here are some probable scenarios which may trigger this:
– The number of steps per epoch is set too high, causing the model to require more data than is available.
xxxxxxxxxx
model.fit(train_data, epochs=5, steps_per_epoch=5000)
In this example, each epoch tries to draw 5000 batches of data from train_data. If train_data has fewer than 5000 batches of data, we’ll run out before completing an epoch.
– Sometimes there might be a mismatch between batch size and total data size while using feature extraction with pre-trained models.
xxxxxxxxxx
data_augmentation = keras.Sequential(
[
layers.experimental.preprocessing.Rescaling(1./255),
layers.experimental.preprocessing.RandomFlip("horizontal_and_vertical"),
layers.experimental.preprocessing.RandomRotation(0.2),
]
)
train_ds = image_dataset_from_directory(
directory,
validation_split=0.2,
subset="training",
seed=1337,
batch_size=32,
image_size=[img_height, img_width]
)
In above case, if the total images in the directory are not a multiple of batch size (32 in this case), few data points may get missed leading to an ‘Out of Data’ situation.
One common way to navigate around this error is by using the
xxxxxxxxxx
repeat()
function within the
xxxxxxxxxx
tf.data.Dataset
API.
xxxxxxxxxx
repeat()
restarts the dataset when it reaches the end, allowing training to continue for the specified number of epochs.
xxxxxxxxxx
dataset = dataset.repeat()
Please note that the problem can also occur if there is any preprocessing or filtering that disqualifies a portion of the data, hence making less data available for the training process. Always ensure that data fed into the model match the requirements of quantity and quality required by the training routine.
However, keep in mind these fixes can have implications. Using repeat() without careful control could lead to situations where your model starts overfitting by seeing the same data repeatedly.Optimizing your model’s performance is a crucial aspect of the coding process. While working with Tensorflow, you may encounter the error message “Your input ran out of data”. If you get this message, it indicates that your model is trying to pull more data than what is available in your input dataset during training, testing or validation.
This issue typically arises due to mismatched batch sizes, incorrect dataset splitting, or over-iteration over the dataset. To avoid input exhaustion and optimize your model’s performance, there are several strategies I can recommend:
1) Correct Batch Sizes:
Batch size refers to the quantity of data points your model analyzes during one update. If your batch size is excessively large, you might draw all data instances before completing an epoch, which results in an “Out Of Data” error. Adjusting your code to reduce the batch size could solve this issue. In Tensorflow, you would adjust this in your Dataset API usage, like so:
xxxxxxxxxx
dataset = tf.data.Dataset.from_tensor_slices((x, y))
dataset_batched = dataset.batch(batch_size)
2) Proper Dataset Splitting:
Ensuring correct splitting between your training and testing dataset is another way to prevent “Input Exhaustion”. Check the code section responsible for dataset splitting for any miscalculations. You might be allocating too many data instances to the training set, leaving your test set exhausted very quickly. The sklearn library’s train_test_split function is a recommended solution for accurate dataset splitting in Python.
xxxxxxxxxx
from sklearn.model_selection import train_test_split
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.20, random_state=42)
3) Manage Dataset Iteration:
Over iterating over your data is another possible cause. This happens when you’re asking your model to go through the data more times than there are actual instances available in each batch. In such cases, repeating the dataset will help. With Tensorflow’s Dataset API, use the repeat method as follows:
xxxxxxxxxx
repeated_dataset = dataset.repeat()
Code Diagnostic Tools:
Tensorflow also offers built-in functions for tracking execution metrics, such as TensorFlow Profiler . These tools allow isolating bottlenecks and visually inspect where runtime is spent within the model layers and operations. For instance, if input pipeline issues exist, they would reflect as high idle times or low device utilization percentages.
4) Dynamic Input Pipeline:
To optimize input pipelines consider prefetching and parallel calls:
xxxxxxxxxx
AUTOTUNE = tf.data.AUTOTUNE
dataset = dataset.prefetch(buffer_size=AUTOTUNE)
Here, the buffer_size specifies the number of samples to preload, easing the computational load of loading data in real-time. The AUTOTUNE mechanism dynamically sets the buffer size based on system specs, reducing memory overhead and smoothing the loading process.
By carefully addressing these optimization factors in your Tensorflow models, you can ensure smooth and efficient training – avoiding the “Your input ran out of data” prompt and maximizing model outcomes. Moreover, knowing how to effectively manage large datasets and complex models is a critical skill in machine learning and AI, arguably as important as creating the models themselves.I’ve been in the trenches of TensorFlow, tackling numerous dataset challenges with sweat on my brow and some stack traces in front of me. The scenario: I’m training an advanced machine learning model when all of a sudden, those dreaded words appear — “Input ran out of data”. Sounds familiar? You’re not alone. There are several ways to address dataset inefficiencies in TensorFlow, what worked for me include:
– Ensuring that the data pipeline continuously feeds data.
– Using the repeat() function for infinite datasets.
– Checking the batch size congruity with the dataset size.
In this complex world of tensors and flows, the first step is to ensure your data pipeline never runs dry. That could be just what you need to fight off the dataset inefficiencies looming large over your project.
You can leverage TensorFlow’s impressive tf.data API to create efficient pipelines. This treats your input data as a sequence of elements where each element can be either a single tensor or a tuple of tensors. The tf.data.Dataset object represents this sequence and provides methods to transform and combine datasets.
Let’s suppose we have a list with 5 items:
xxxxxxxxxx
data = [0, 1, 2, 3, 4]
data_set = tf.data.Dataset.from_tensor_slices(data)
As per the tf.data API, the iterator will cease after running through the data once. To keep it continuous, use the repeat() function, which repeats this dataset so many times. Here’s how to do it:
xxxxxxxxxx
repeated_data_set = data_set.repeat()
Now the data feed won’t stop until explicitly specified. Be cautious though! Ensure there is enough memory for infinite datasets, else adverse side effects may occur like slower model training due to swapping on disk.
Another common cause for the “Input ran out of data” error is a mismatch between the batch size provided during the fitting process and the actual size of the data. For instance, if your dataset has 100 records and you set your TensorFlow model’s batch size to 101, TensorFlow will be unable to take the next batch from your dataset after completing a training epoch, throwing the “Input ran out of data” error.
To fix this, check and adjust your batch size before initiating the fitting process. A code snippet might look something like this:
xxxxxxxxxx
batch_size = 50
data_batched = data_set.batch(batch_size)
Here, not only have you split your data into batches, but also ensured every batch has enough data points until the very last one. Remember, if your data number does not perfectly divide by the batch size, TensorFlow will emit smaller final batch without complaints.
It’s vital to continually tweak these parameters based on your specific use cases, data availability and compute resources. By ensuring that your data pipeline always has data to push through, using the TensorFlow APIs wisely, and setting appropriate batch sizes, your machine learning models can perform optimally without interruptions.Establishing a robust data pipeline is definitely an important part of preventing issues such as ‘your input ran out of data’ from cropping up when working with TensorFlow, the open-source machine learning platform. This can be done by being mindful of several key principles in the design and implementation of your data pipeline.
Data Consistency
Ensuring consistency in your data should be your number one priority when developing your pipeline. One of the common reasons for getting the error ‘your input ran out of data’ is because there were inconsistencies in the source data that caused the pipeline to grind to a halt. For instance, there may have been mismatched columns, missing values, or mislabeled elements.
You may implement a system that treats and validates input data before adding it to the pipeline. Make sure to check all data fields and entries for errors and inconsistencies (Tensorflow Guidelines on Data Validation).
Correctly estimating the data size
It’s also worth noting that creating a healthy buffer size based on evaluations of your maximum expected data feeds can help prevent problems such as ‘your input ran out of data.’ However, understanding your data needs does not stop there. In dealing with real-time or dynamic data, you should also anticipate fluctuations in size and frequency. Having mechanisms in place to handle these variations is paramount to maintaining a smooth data flow.
Regular Backups
It’s challenging to provide a consistent data feed without occasional slowdowns or interruptions. That’s why regular backups can also be beneficial. Not only can they ensure the continuity of data needed by TensorFlow, but they can also serve as a safety net in cases where the original data source becomes unexpectedly interrupted.
In Python, we can create a data backup as follows:
xxxxxxxxxx
# Importing necessary library
import shutil
# Source path
src_dir = 'OriginalData'
# Destination path
dst_dir = 'BackUpData'
# Copy the content of source to destination
shutil.copytree(src_dir, dst_dir)
However, bear in mind that your machine should have adequate memory to accommodate a backup data set.
Monitoring the System
Finally, monitoring is the last essential piece of the puzzle. By diligently observing and optimizing your data pipeline, you can spot bottlenecks or other issues before they impact your TensorFlow process performance. You can make use of TensorFlow’s integrated tools to pinpoint inefficiencies and get real-time insights into your data pipeline (Tensorflow TensorBoard).
Remember, preventing ‘your input ran out of data’ isn’t just about having lots of data; it’s also about managing that data effectively. A well-crafted, maintained, and monitored data pipeline is absolutely pivotal in ensuring a smooth TensorFlow experience.If you’ve experienced an “Input Ran Out of Data” issue in Tensorflow, it’s essential to identify the root cause and implement a solution that will fix it. This error message typically arises when Tensorflow attempts to load more data from your dataset but can’t find any. Here are some useful debugging techniques for such issues:
Checking Dataset Size
The first approach consists of checking if your dataset is correctly loaded by examining its size. Use the
xxxxxxxxxx
len()
function on your dataset to ensure that the expected quantity of data is present:
xxxxxxxxxx
size_of_dataset = len(dataset)
print('Size of dataset:', size_of_dataset)
If the returned size doesn’t meet your expectation or equals zero, there might be an issue with how your data was loaded.
Verifying Batch Size
Another common issue comes from setting an inappropriate batch size during training. The batch size should not exceed the number of instances in your dataset. If you set your batch size too large, Tensorflow may run out of data mid-batch, causing this error.
Validating Input Pipeline
It would help if you validated your input pipeline next. Try retrieving a single batch from it and check its shape:
xxxxxxxxxx
for image, label in dataset.take(1):
print('Image batch shape: ', image.shape)
print('Label batch shape: ', label.shape)
Your output shapes should match the expected dimensions.
Assessing Ordering of Operations
Ensure adequate ordering of operations in your code. Remember calling
xxxxxxxxxx
batch()
method should follow
xxxxxxxxxx
.repeat()
method like this:
xxxxxxxxxx
dataset = dataset.repeat().batch(batch_size)
Instead of:
xxxxxxxxxx
dataset = dataset.batch(batch_size).repeat()
In the second variation, every epoch after the first one will start with whatever data was left over from the previous epoch which might create inconsistency.
Examining Other Factors
Other situations that could lead to this error include:
- Misreading file paths
- Improper file formats
- Data corruption issues
You could verify files and paths with:
xxxxxxxxxx
import os
file_paths = [i.numpy() for i in list(dataset)]
files_exist = [os.path.exists(i) for i in file_paths]
print('Files not found:', file_paths.count(False))
Remember, debugging requires patience and systematic trial-and-error thinking. Testing different approaches like these might take some time, but ultimately, they’ll give you a better understanding of your model’s functionality and Tensorflow’s workings.
Additional resources that elaborate on various methods for loading data and optimizing your input pipeline in Tensorflow are available on the official Tensorflow Website here.
For more context and similar issues addressed await you at StackOverflow here.Insufficient Training Sample errors, popularly known as “Input Ran Out Of Data” in TensorFlow, is generally raised when your data input pipeline is not supplying enough data to your model during training. It’s a common issue that could stem from several sources such as:
– Mismatch between batch size and the total number of samples.
– Error in splitting your dataset.
– Incorrect repetition of the dataset.
– Issues related to multithreading or queuing.
Therefore, it’s essential to troubleshoot and fix this error for smooth model training. Here are some potential solutions you might want to consider:
Increase Batch Size:
If your batch size is too large compared to your total dataset size, you may run out of samples. You can amend the error by reducing your batch size or increasing your dataset.
Let’s say your original batch size was 100, you can decrease it as shown below:
xxxxxxxxxx
batch_size = 50
data = data.batch(batch_size)
Review Dataset Splitting:
Make sure you properly split your data into training, validation, and possibly testing sets. If your splits are incorrect, you might incorrectly feed data into your model.
Here’s an example of how you might divide your data,
xxxxxxxxxx
# if 'data' is your complete dataset
train_data = data.take(int(len(data)*0.7))
test_data = data.skip(int(len(data)*0.7))
Repeat Dataset:
Use the
xxxxxxxxxx
.repeat()
function on your dataset to ensure there is a consistent supply of data during model training. This function repeats your dataset for a specified number of times or indefinitely if no argument is provided. Please be careful while using it as indefinite repetition can cause the model to loop endlessly over the same set.
Here’s how to apply it on your data:
xxxxxxxxxx
data = data.repeat()
Multithreading and Queues:
If you use
xxxxxxxxxx
.prefetch(tf.data.AUTOTUNE)
, TensorFlow automatically loads batches of your training data ahead of time to help with performance, which can also resolve your error. In this manner, while your current batch is being used for training, the next batch is being loaded.
Example usage might look like this:
xxxxxxxxxx
data = data.prefetch(tf.data.AUTOTUNE)
Aside from these specific methods, always ensure your dataset has been correctly loaded before starting model training. You can do so by verifying a few instances using TensorFlow’s built-in
xxxxxxxxxx
take(n)
method, where “n” signifies the number of records to preview. Regular debug steps like this can potentially save significant effort and time.
In summary, understanding your data and carefully constructing your data pipeline will prevent ‘ran out of data’ issues. Furthermore, TensorFlow provides several flexible options like automatic batching, repeating, prefetching, and more, allowing developers to design a robust pipeline suited to their specific needs.
For more in-depth insights about handling datasets in TensorFlow, you can visit the official TensorFlow website here.
Absolutely, it’s quite critical to keep the data flowing into your TensorFlow model, ensuring there’s no interruption which might trigger an “Input Ran Out of Data” error. There are various strategic approaches that can be taken to ensure a consistent supply of data to your model during training or evaluation.
Overcoming the “Input Ran Out Of Data” Error
First and foremost, the “Input ran out of data” error in TensorFlow indicates that your model has run out of data during the training process. This usually happens during model.fit(), model.evaluate() or model.predict() calls. It often means that the dataset isn’t providing as many samples as expected, especially in scenarios where you’ve mentioned specific steps per epoch, set initially when calling fit() function.
Here, consider a scenario where you’ve a dataset of 100 samples and you’ve set batch size to 10. This implies that there are exactly 10 steps per epoch. However, if you mistakenly specify more than 10 (let’s say 15), TensorFlow will throw an exception after finishing the tenth step because it doesn’t have more data to offer, leading to the notorious “Input ran out of data” error.
xxxxxxxxxx
model.fit(train_dataset, epochs=number_of_epochs, steps_per_epoch=15)
To resolve these issues, consider the following tips:
Proper Setting of Steps Per Epoch
Ensure you’re setting an appropriate value for steps_per_epoch argument. Ideally, it should align with the count of available samples divided by batch size. As TensorFlow documentation highlights[^1^], this parameter lets the fit method know how many batches of data from your dataset will constitute one epoch. For instance:
xxxxxxxxxx
steps_per_epoch = len(dataset) / batch_size
model.fit(train_dataset, epochs=number_of_epochs, steps_per_epoch=steps_per_epoch)
The use of Repeat Function
Incorporate the use of .repeat() function[^2^] when preparing the dataset. When chained with other calls while building datasets, the function’s role is to repeat the dataset indefinitely. Consequently, even in cases of exhaustion, new data iteration starts automatically, offering continuous feed.
xxxxxxxxxx
dataset = dataset.repeat()
While using the repeat function, remember not to set the steps_per_epoch argument, letting TensorFlow calculate it on its own. The API is intelligent enough to compute it based on total count of available data samples and specified batch size.
Utilizing tf.data Dataset From Generator
You can also consider using
xxxxxxxxxx
tf.data.Dataset.from_generator()
[^3^] function when aiming to continuously feed data into your model. This enables reading data directly from a Python generator function, creating virtually endless data streams from finite datasets.
xxxxxxxxxx
def generator():
while True:
for x in X:
yield x
ds = tf.data.Dataset.from_generator(generator)
model.fit(ds)
In conclusion, persistent data feeding into TensorFlow models involves adopting well-suited strategies like appropriate steps_per_epoch setting, using .repeat() with dataset, or employing tf.data.Dataset.from_generator(). While these suggestions should handle most situations, always remember each case is unique. Therefore, adjustments may indeed be needed depending upon specific requirements or project constraints.
[^1^]: TensorFlow Model.fit Documentation
[^2^]: TensorFlow Dataset.repeat() Documentation
[^3^]: TensorFlow Dataset.from_generator() DocumentationWhen handling Neural Networks with Tensorflow, encountering the “Your Input Ran Out of Data” error can be an overwhelming experience. However, taking a closer look into the nature of this issue, it mainly roots in the incorrect set-up of one’s model or pipeline and is commonly associated with feeding insufficient data during the training process.
That said, understanding Tensorflow involves learning how to anticipate, detect, and respond to different kinds of errors, including the “Your Input Ran Out of Data” error. Here’s a quick overview on why it may occur:
- Not enough training samples: This happens when our network has run out of new information to learn from – essentially there isn’t enough data for the model to train.
- Mismatch of data and labels: Wherein, the amount of labels does not match with the available input data.
- Over-splitting of dataset: Too much data designated for validation and testing leaving sparse data for training can also generate this issue.
One simple way to rectify this error would be generating more data if possible. In Python, this involves adjusting the number of epochs using
xxxxxxxxxx
model.fit(epochs=x)
. It’s advisable not to set epochs too low since model training might stop before reaching an optimal accuracy score.
Alternatively, you could investigate the possibility of a mismatch between your inputs and targets (labels). Make sure that every input sample has an associated label. Following code snippet can help in verifying data-label association:
xxxxxxxxxx
print("Length of Inputs: ", len(input_data))
print("Length of Labels: ", len(labels))
Moreover, balancing your dataset distribution among training, validation, and test sets is also crucial. Properly allocate your data across these subsets to avoid depleting your training data prematurely.
Furthermore, there are tons of useful resources online like Stack Overflow, GitHub Issues and Tensorflow’s official forum that can provide extra guidance when dealing with the nuances of neural network training. Delving deeper into the workings of Tensorflow turns such hurdles into learning opportunities, enhancing your proficiency as a coder and contributing further to the AI revolution.
With persistent practice and intelligent tweaks, mastering Tensorflow’s sophisticated offerings can become second-nature, making machine learning challenges like “Your Input Ran Out of Data”, a breeze to overcome. Remember, as coders, we are not just solving problems, but continually refining our algorithms in the pursuit of optimization. And sometimes, running out of data can be just another stepping stone to better and cleaner code!